Program to convert octal to decimal in C Language
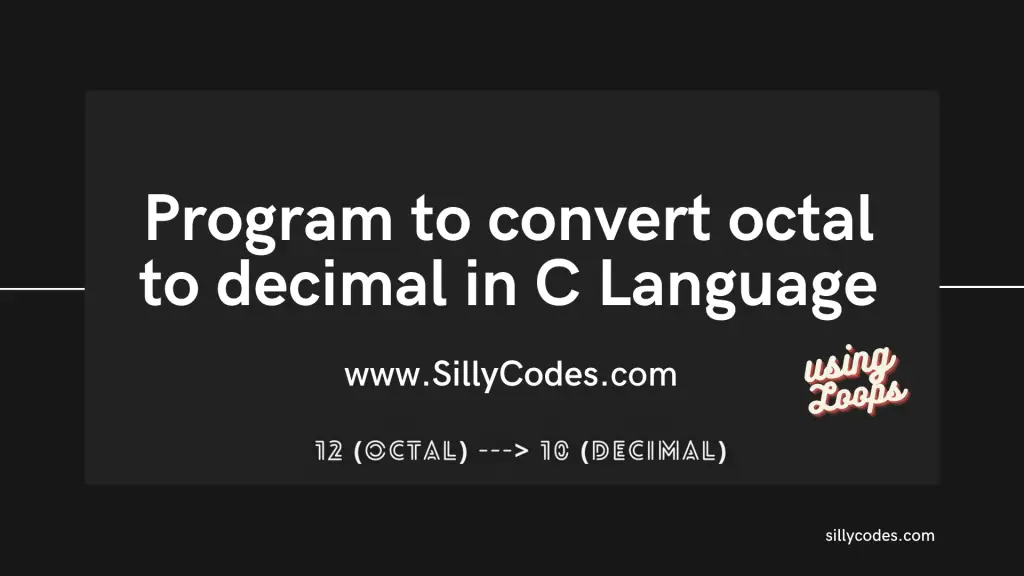
Program Description:
Write a Program to convert octal to decimal in C programming language. The program should accept an Octal number and converts the provided octal number to a decimal number using the while loop.
What are Octal Numbers?
Here is the definition Octal number from Wikipedia,
The octal numeral system, or oct for short, is the base-8 number system and uses the digits 0 to 7, that is to say 10octal represents eight and 100octal represents sixty-four. However, English, like most languages, uses a base-10 number system, hence a true octal system might use different vocabulary.
In the decimal system, each place is a power of ten. For example:
In the octal system, each place is a power of eight. For example:
Octal Number to Decimal Number conversion:
To convert an Octal number to a Decimal number. We need to get each digit of the Octal number and apply the power function.
Here is an example of an Octal number to Decimal conversion.
Convert Octal 20 to decimal number
20 = (2 × 8¹) + (0 × 8⁰) = 16
As you can see Octal 20 is equal to decimal 16
Here are the few Octal Numbers and their equivalent decimal numbers.
Octal Number | Decimal Number |
---|---|
0 | 0 |
1 | 1 |
2 | 2 |
3 | 3 |
4 | 4 |
5 | 5 |
6 | 6 |
7 | 7 |
10 | 8 |
11 | 9 |
12 | 10 |
13 | 11 |
14 | 12 |
15 | 13 |
16 | 14 |
17 | 15 |
20 | 16 |
Program to Convert Octal to Decimal in C Language Algorithm:
- Take the input Octal number from the user. And we store the input value in variable n
- If the number is negative or zero, Display the error and ask for the input again ( We use the goto statement to take the program control back to the start)
- The main idea is to get each digit of the Octal number and use the power function to calculate the power of 8 (as it is an octal number) with respect to the digit position.
- The loop run until the n becomes zero or less – Condition – (n > 0). We start from the last digit and go til the first digit.
- At Iteration of Loop
- We get the last digit of the number. for the first iteration, we get the last digit using the modulus operator – rem = n%10;
- Then we calculate the rem * pow(8, i); – At the first Iteration, which is equal to pow(8, 0) and at the second iteration, It will be pow(8, 1), and so on.
- Then add the above result to our decimal variable – decimal = decimal + rem * pow(8, i);
- As we processed the last digit, We need to remove it from the n. To do that, We can use the Division Operation like this – n = n/10;
- Finally, Increment the variable i. which is used in the calculation of pow
- The above loop continues until the n value is greater than the Zero
- Once the loop is terminated, The decimal variable contains the Decimal value of the provided octal number ( n). Then display the result on the console.
📢 We are going to use the pow function from the math.h header file to calculate the power. If you don’t want to use the predefined pow function, you can write a custom function as well.
Program to Convert Octal to Decimal in C Language:
Let’s convert the above algorithm into the C program.
We are going to use the pow function from the math.h library. The prototype of the pow function is
double pow(double x, double y)
Here is the program to convert the Octal number to a Decimal number in C language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/* Program: Octal to Decimal Convertion Program in C Author: Sillycodes.com */ #include<stdio.h> #include<math.h> int main () { // Declare variables int decimal=0, i=0, rem=0,n,temp; // goto label. INPUT: // Take input from the user. printf("Enter the octal number : "); scanf("%d", &n); if(n <= 0) { printf("Invalid Input, Please try again \n"); // using goto statement to go back to take input again. goto INPUT; } // Take backup of n temp=n; // Loop until the n becomes equal to zero. while (n > 0) { // Get the last digit of number rem = n%10; // calculate the decimal decimal = decimal + rem * pow(8, i); n = n/10; i++; } printf("%d in octal is equal to %d in decimal \n", temp, decimal); return 0; } |
Convert Octal to Decimal in C program Output:
As we have specified above, We used the pow function to calculate the power value. And pow function is not part of the default C library, So we need to use the -lm option to include the math library functions.
So to Compile the program use the following command ( In Linux based systems)
1 |
gcc octal-to-decimal.c -lm |
The above command generates the executable file, which is a.out, Run the executable.
1 2 3 4 |
$ ./a.out Enter the octal number : 20 20 in octal is equal to 16 in decimal $ |
Let’s test few more examples
1 2 3 4 5 6 7 |
$ ./a.out Enter the octal number : 456 456 in octal is equal to 302 in decimal $ ./a.out Enter the octal number : 78 78 in octal is equal to 64 in decimal $ |
As you can see we are getting the expected decimal values.
What if the user enters a negative number?
1 2 3 4 5 6 7 8 |
$ ./a.out Enter the octal number : -3 Invalid Input, Please try again Enter the octal number : -192 Invalid Input, Please try again Enter the octal number : 234 234 in octal is equal to 156 in decimal $ |
If the user enters a negative number, The program displayed an error notice ( Invalid Input, Please try again) and asked for the user input again.
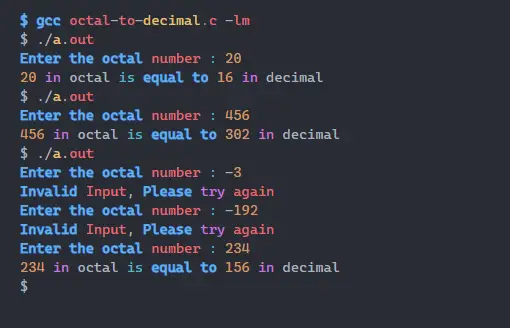
3 Responses
[…] C Program to Convert Octal Number to Decimal Number […]
[…] C Program to Convert Octal Number to Decimal Number […]
[…] C Program to Convert Octal Number to Decimal Number […]