Program to Reverse string in C Language
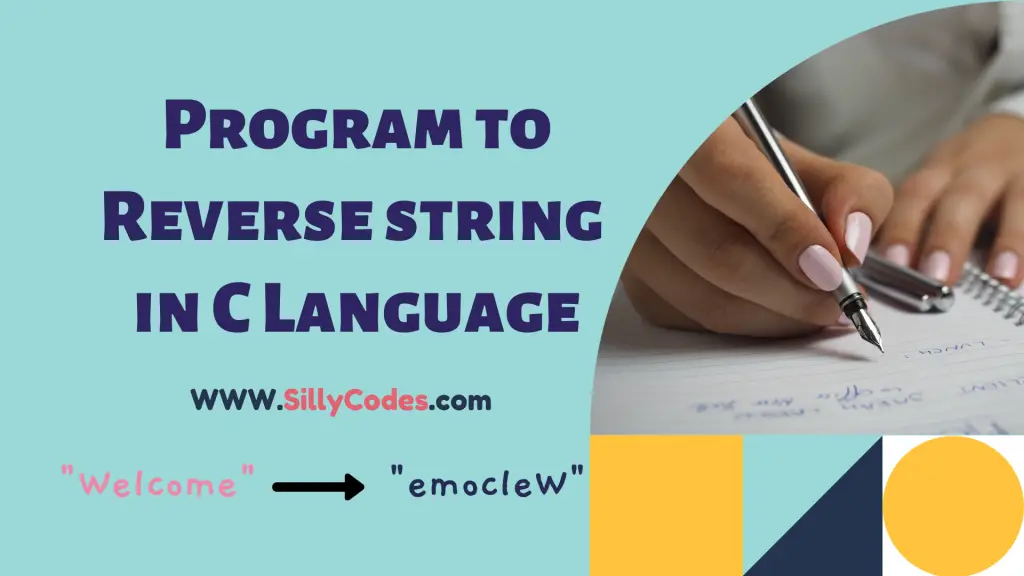
Program Description:
Write a Program to Reverse String in C programming language. The program should accept a string from the user and reverse its characters.
📢 This Program is part of the C String Practice Programs series
Here is the example input and output of the program.
Example Input and Output:
Input:
Enter a string : learn programming
Output:
Reversed String: gnimmargorp nrael
As you can see from the above input and output, The output string is the exact reverse of the input string.
Prerequisites:
It is recommended to have knowledge of the C-Strings and Arrays. Please go through the following articles to learn more about it.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
Algorithm to Reverse string in C:
We can Reverse a String by using Two pointer approach.
- Create two variables called start and end. Which stores the indices.
- The start variable stores the first element in the string(Zeroth Index). The end variable stores the last element in the string.
- Create a Loop to iterate over the string.
- Swap the start index and end index elements. Now The first character and last character are swapped. We are using a temporary variable to swap the elements, But you can use other methods to Swap as well.
- Then increment the start element, so that it pointed to the next element in the string. Similarly, Decrement the end, So that it points to the second last element.
- Repeat the above step until the start index becomes equal to or greater than the end index. (start < end)
- Once the above loop is completed, The input strings will be Reversed.
Reverse string in C Using Iterative Method:
In the following program, We used the above string reverse algorithm. Note, That We used the variables i and j in place of the start and end in the above example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/*     program to Reverse a String in C     sillycodes.com */  #include<stdio.h> #include<string.h>  // Max Size of string const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     int i, j, temp;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);      // maintain two indices, one(i) at start, second(j) at end     i = 0;     j = strlen(inputStr)-1;         // Iterate over the string     // swap the characters at indices i, j and increment 'i' and decrement 'j's     for( ; i < j; i++, j--)     {         // swap characters         temp = inputStr[i];         inputStr[i] = inputStr[j];         inputStr[j] = temp;     }      // print the reversed string     printf("Reversed String: %s\n", inputStr);      return 0; } |
We declared a string called inputStr and took the user input and updated it. Then we used the above String Reverse Two Pointer approach to Reverse the string.
Finally, We displayed the Reversed string on the console.
Program Output:
Let’s compile and Run the Program
1 2 3 4 5 6 7 8 |
$ gcc reverse-string.c $ ./a.out Enter a string : Welcome Reversed String: emocleW $ ./a.out Enter a string : sillycodes.com Reversed String: moc.sedocyllis $ |
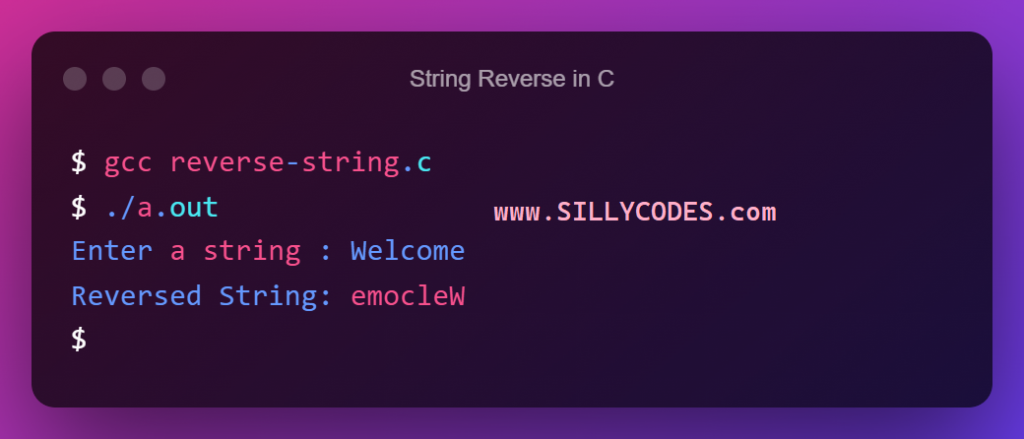
As we can see from the above output When the user entered the input string as Welcome, The program reversed the string and provided the output as emocleW.
Program to Reverse string in C using User-defined functions:
In this method, We are going to move the string reverse logic to a user-defined function called stringReverse.
📢 Related: Advantages of the Functions
The stringReverse function takes a string(i.e character pointer) as a formal argument and reverses the given string and returns it back to the caller.
Prototype of the stringReverse function:
1 |
char * stringReverse(char * inputStr) |
Here is the program to reverse a string in the C language using a custom function called stringReverse.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
/*     program to Reverse a String in C     sillycodes.com */  #include<stdio.h> #include<string.h>  // Max Size of string const int SIZE = 100;  /** * @brief - Reverse the given string * * @param inputStr - input string * @return char*  - Returns the reversed string */  char * stringReverse(char * inputStr) {     // maintain two indices, one(i) at start, second(j) at end     int i = 0;     int j = strlen(inputStr)-1;     int temp;         // Iterate over the string     // swap the characters at indices i, j and increment 'i' and decrement 'j's     for( ; i < j; i++, j--)     {         // swap characters         temp = inputStr[i];         inputStr[i] = inputStr[j];         inputStr[j] = temp;     }      return inputStr; }  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     int i, j, temp;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);      // call the 'strinReverse' function     printf("Reversed String: %s\n", stringReverse(inputStr));      return 0; } |
The stringReverse function uses the above two-pointer approach to reverse the string. It uses two variables called i and j to maintain the start and end indices and Swaps the characters pointed by the i and j indices, Then it increments the i and decrements j. This process continues until the i<j condition becomes false. Finally, stringReverse function returns the resultant string back to the main() function.
📢 We used the
strlen string library function in the above program. But if you don’t want to use any library functions, Then you can implement and use custom strlen function also.
Here is the article, Where we implemented a custom strlen function( string length function )
Program Output:
Let’s compile and Run the program using GCC.
1 2 3 4 5 6 7 8 9 |
$ gcc stringReverse.c $ ./a.out Enter a string : learn programming Reversed String: gnimmargorp nrael $ $ ./a.out Enter a string : SillyCodes.com Reversed String: moc.sedoCylliS $ |
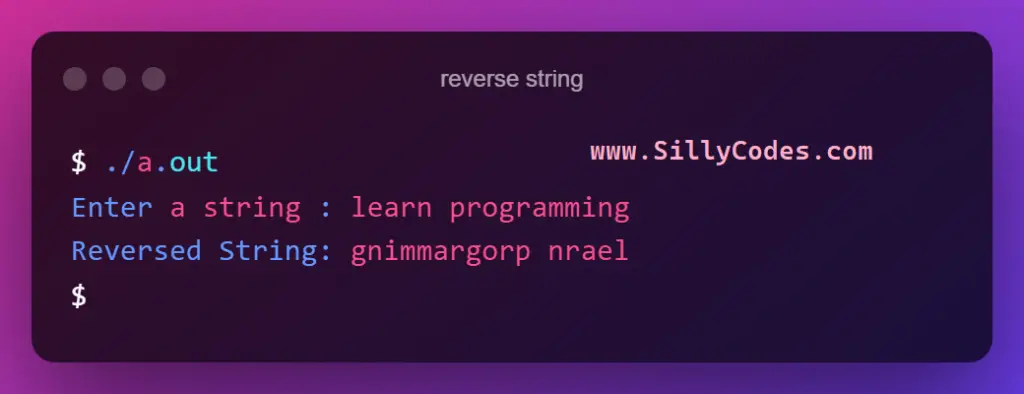
Program is generating the desired results.
Reverse String using strrev function in C Language:
We can also use the strrev function to reverse a string in C language. But the strrev() function is a non-standard C function. It is a non-standard function from Microsoft’s C-Lang library. So it might not work on all implementations (compilers/environments)
The strrev(str) function takes a string as input and reverses its characters.
You can use the following program on compatible C Implementations (Compilers) to Reverse the string using the strrev function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
/*     program to Reverse a String in C     sillycodes.com */  #include<stdio.h> #include<string.h>  // Max Size of string const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     int i, j, temp;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);      // Call the 'strrev' function to reverse the string     printf("Reversed String: %s\n", strrev(inputStr));      return 0; } |
Related String Practice Programs:
- C Tutorials Index
- C Programs Index
- C Program to Calculate Length of the string
- C Program to Copy a String to new string
- C Program to Concatenate Two strings
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
1 Response
[…] C Program to Reverse a String […]