Check Palindrome Number in C program
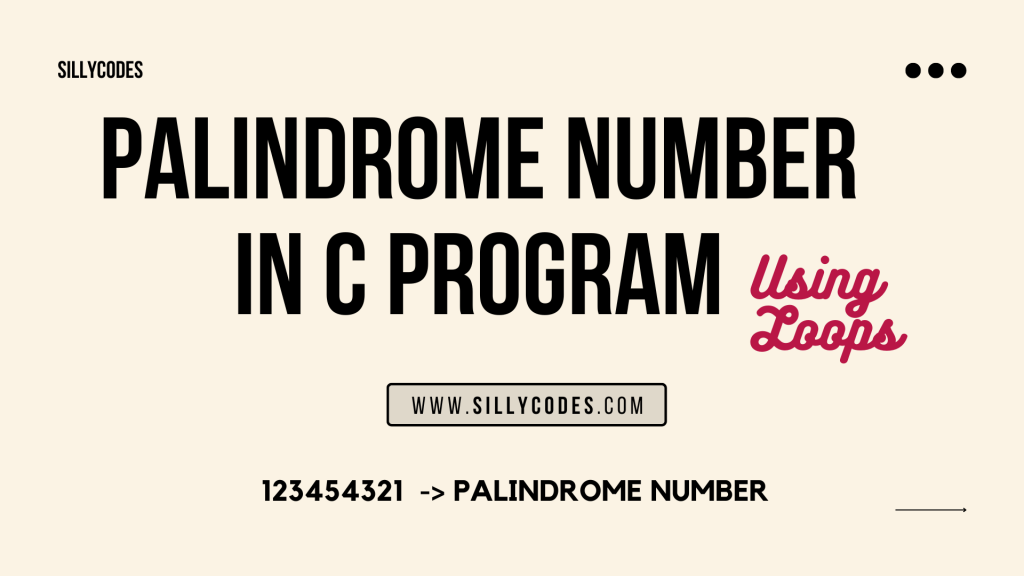
Program Description:
We have looked at the LCM of two numbers program in our earlier articles, In today’s article, We are going to write a program to check Palindrome Number in C programming language. The program needs to accept a number from the user and check it is a palindrome number.
What is a Palindrome Number?
The Palindrome Number is a number that is equal to the original number when its digits are Reversed.
Here is an example.
Number 1234321, The Number 1234321 is a Palindrome number, As if you reverse the number, It will be the same as the Original number.
Similarly, The Number 89098 is a Palindrome number.
Now, We know what are the palindrome numbers, So let’s get started with the program.
Example Inputs and Outputs of the Program:
Example 1: Input:
Enter a positve numbers: 76967
Output:
76967 is a Palindrome Number
Example 2: Input:
Enter a positve numbers: 237384
Output:
237384 is not a Palindrome Number
Program Pre-Requisites:
We are going to use the C Loops and the Arithmetic Operators. So it is recommended to have the knowledge of them. Please go through the following blogs to learn more about the above topics.
- Arithmetic Operators.
- While Loop in C Language
- For Loop in C Language with Example Programs
- do while loop in Language with example programs
Palindrome number in C Program Algorithm:
The main Idea is to Reverse the Original number and Then check the original number and reversed number. If both are Equal, Then the number is Palindrome. If both are not equal, Then the number is not a Palindrome number.
Here is the step-by-step algorithm:
- Start the program by taking the user input. Take the number from the user and store it in a variable named num
- We only accept positive numbers, So check if the number is positive, If not display the error message – Invalid Input. Please provide valid number
- Take a backup of the num for later comparison. temp = num
- Now use the Reverse Number in C Program logic to reverse the number using the Modulus and division operators
- We use the Loop to iterate over each digit from the last to first
- Perform the Modulus operation on the number to get the last digit – rem = temp % 10;
- Then update the reverse number using rev_num = (rev_num * 10) + rem ;
- Finally, remove the last digit of the number using the division operator using temp = temp / 10;
- This above step-4 continues until the temp is greater than zero. temp > 0.
- Once step 4 is completed, We will get the reversed number in the variables rev_num.
- Compare the reversed number
rev_num with the original number
num using the num == rev_num
- If the above comparison is True, Then both numbers i.e Original number and Reversed numbers are equal, and the Given number is a Palindrome Number. So display the result on the console.
- If the above comparison is False, Then the number num is not a Palindrome number.
- STOP.
Palindrome number in C Program using while loop:
Here is the Check or Detect Palindrome number program using the while loop.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
/* Program: Palindrome number in C using while loop Author: SillyCodes.com */ #include<stdio.h> int main() { int num, temp, rev_num, rem; // User Input printf("Enter a positve numbers: "); scanf("%d", &num); // check for negative number and display error if(num <= 0) { // got negative number. Display Error printf("Invalid Input. Please provide valid number\n"); return 0; } // Take back of 'num'. temp = num; rev_num = 0; // Use while loop to Iterate over the number. // We are going to go through each digit by using the modulus operator // and division operator. while(temp > 0) { // get the last digit using modulus rem = temp % 10; rev_num = (rev_num * 10) + rem ; // remove the last digit temp = temp / 10; } // compare given number and reverse if(num == rev_num) { // Both numbers are same. // So it is palindrome printf("%d is a Palindrome Number\n", num); } else { // Not palindrome printf("%d is not a Palindrome Number\n", num); } return 0; } |
Program Output:
Compile and run the program using your IDE. we are using the GCC compiler under the Ubuntu Operating system.
$ gcc palindrome_number.c
Test the program.
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a positve numbers: 12121 12121 is a Palindrome Number $ ./a.out Enter a positve numbers: 1234 1234 is not a Palindrome Number $ ./a.out Enter a positve numbers: 45654 45654 is a Palindrome Number $ |
As expected, Program is giving the desired results.
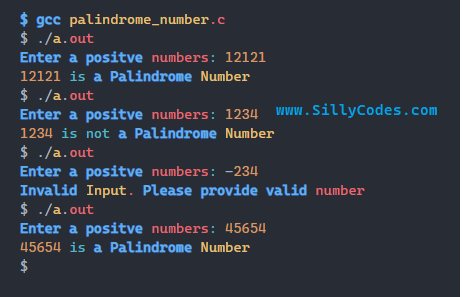
Let’s try to input the negative numbers to the program
1 2 3 4 |
$ ./a.out Enter a positve numbers: -234 Invalid Input. Please provide valid number $ |
As we can see from the above output, The program is displaying the error message on the Negative number.
Palindrome number in C using for loop:
Let’s rewrite the above program using the for loop instead of the while loop. The program algorithm is going to be the same but the program syntax is going to change.
Here is the C code with for loop.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
/* Program: Palindrome number in C using for loop Author: SillyCodes.com */ #include<stdio.h> int main() { int num, temp, rev_num, rem; // User Input printf("Enter a positve numbers: "); scanf("%d", &num); // check for negative number and display error if(num <= 0) { // got negative number. Display Error printf("Invalid Input. Please provide valid number\n"); return 0; } // Number is Positive Number. // Take back of 'num' temp = num; // Use for loop to Iterate over the number. // We are going to go through each digit by using the modulus operator // and division operator. for(rev_num = 0; temp > 0; temp = temp/10) { // get the last digit using modulus rem = temp % 10; // update reverse number - rev_num rev_num = (rev_num * 10) + rem ; } // compare given number and reverse if(num == rev_num) { // Both numbers are same. // So it is palindrome printf("%d is a Palindrome Number\n", num); } else { // Not palindrome printf("%d is not a Palindrome Number\n", num); } return 0; } |
Program Output:
Compile and run the program.
Here is the output of the program for positive numbers
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc palindrome_number.c $ ./a.out Enter a positve numbers: 19791 19791 is a Palindrome Number $ ./a.out Enter a positve numbers: 2359 2359 is not a Palindrome Number $ ./a.out Enter a positve numbers: 13031 13031 is a Palindrome Number $ ./a.out Enter a positve numbers: 123454321 123454321 is a Palindrome Number $ |
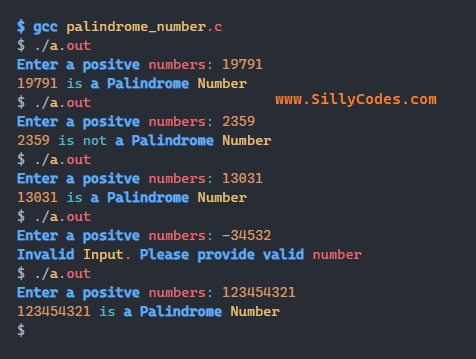
Program output for the negative numbers.
1 2 3 4 |
$ ./a.out Enter a positve numbers: -34532 Invalid Input. Please provide valid number $ |
Related Programs:
- If else practice Programs
- Switch Case Practise Programs
- All Factors of Number Program
- GCD of two numbers Program in C Language
- LCM of two numbers program in C
- C program to test Leap year
- C program to Find Area and Perimeter of Rectangle.
- Calculate the Grade of Student.
- Type Conversion Operators on Assignment operator.
- Prime Numbers up to n
- Calculate Sum of Digits Program in C
- Octal to Decimal Number Conversion
3 Responses
[…] C Program to Check Palindrome Number […]
[…] C Program to Check Palindrome Number […]
[…] C Program to Check Palindrome Number […]