Program to calculate Perimeter and Area of Rectangle in C Language
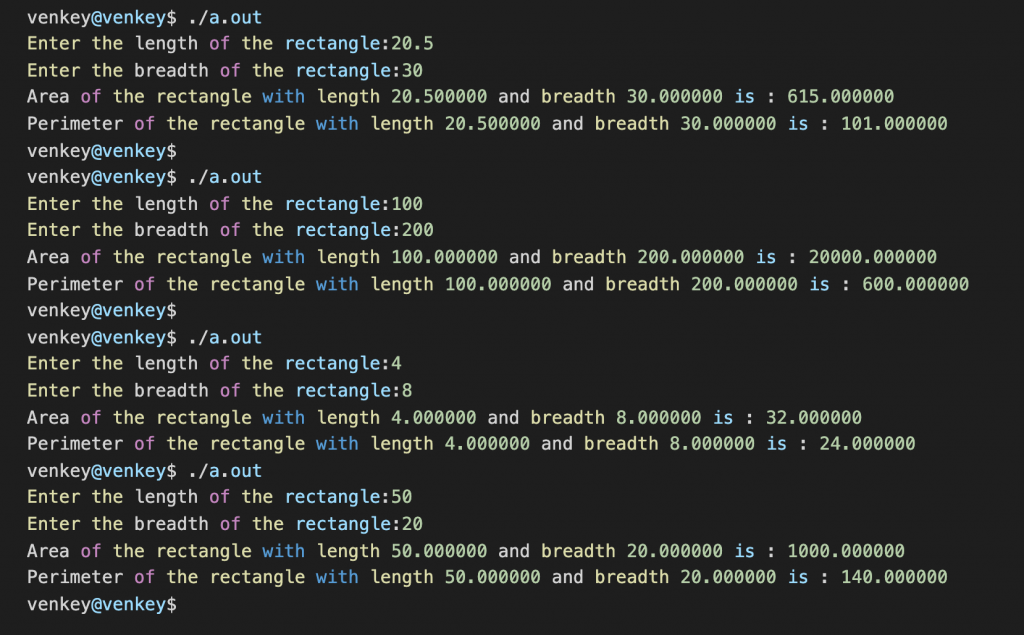
Introduction:
This program is for Calculating the Perimeter and Area of a Rectangle in C programming Langauge.
most of us already know the formula’s to calculate the Perimeter and Area of the Rectangle.
Anyway, here are the formulas for Perimeter and Area.
Area of Rectangle Formula:
Area of Rectangle = b × h.Â
Where b = breadth and h = Height
Perimeter of Rectangle Formula:
The Perimeter of Rectangle = 2(b) + 2(h)
Where b = breadth and h = Height
Calculate Perimeter and Area of Rectangle in C Program :
We are going to take the user input for the Length and Breadth values. We are considering the length and Breadth as the Float values, So you can give the float and integer values.
Once we got the user input, We are going to apply the above Area and Perimeter formulas to Calculate the results.
Finally, We are going to print the results onto the console.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#include<stdio.h> int main() {     // Create desired Variables, Like length, breath, area and perimeter     float length, breadth, area, perimeter;      // Take input from the user     // Take Length from the user and store it in 'length' variable     printf("Enter the length of the rectangle:");     scanf("%f",&length);      // Take the 'breadth' from user and store it in 'breadth' variable.     printf("Enter the breadth of the rectangle:");     scanf("%f",&breadth);      // Use the Rectangle Area Formula to calculate the Area.     // Store result in 'area' variable     area = length * breadth;      // Calculate the Perimeter, using the Rectangle Perimeter formula     // Store the result in 'perimeter' variable     perimeter = 2*(length + breadth);      // Print the results     printf("Area of the rectangle with length %f and breadth %f is : %f \n", length, breadth, area);     printf("Perimeter of the rectangle with length %f and breadth %f is : %f \n", length, breadth, perimeter);         return 0; } |
Program Output:
Here is the output for the program for different Length and Breadth values.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
venkey@venkey$ ./a.out Enter the length of the rectangle:20.5 Enter the breadth of the rectangle:30 Area of the rectangle with length 20.500000 and breadth 30.000000 is : 615.000000 Perimeter of the rectangle with length 20.500000 and breadth 30.000000 is : 101.000000 Â venkey@venkey$ ./a.out Enter the length of the rectangle:100 Enter the breadth of the rectangle:200 Area of the rectangle with length 100.000000 and breadth 200.000000 is : 20000.000000 Perimeter of the rectangle with length 100.000000 and breadth 200.000000 is : 600.000000 Â venkey@venkey$ ./a.out Enter the length of the rectangle:4 Enter the breadth of the rectangle:8 Area of the rectangle with length 4.000000 and breadth 8.000000 is : 32.000000 Perimeter of the rectangle with length 4.000000 and breadth 8.000000 is : 24.000000 Â venkey@venkey$ ./a.out Enter the length of the rectangle:50Â Â Enter the breadth of the rectangle:20 Area of the rectangle with length 50.000000 and breadth 20.000000 is : 1000.000000 Perimeter of the rectangle with length 50.000000 and breadth 20.000000 is : 140.000000 venkey@venkey$ |
1 Response
[…] C Program to calculate Perimeter and Area of Rectangle […]