Find Max Element in Array in C Language
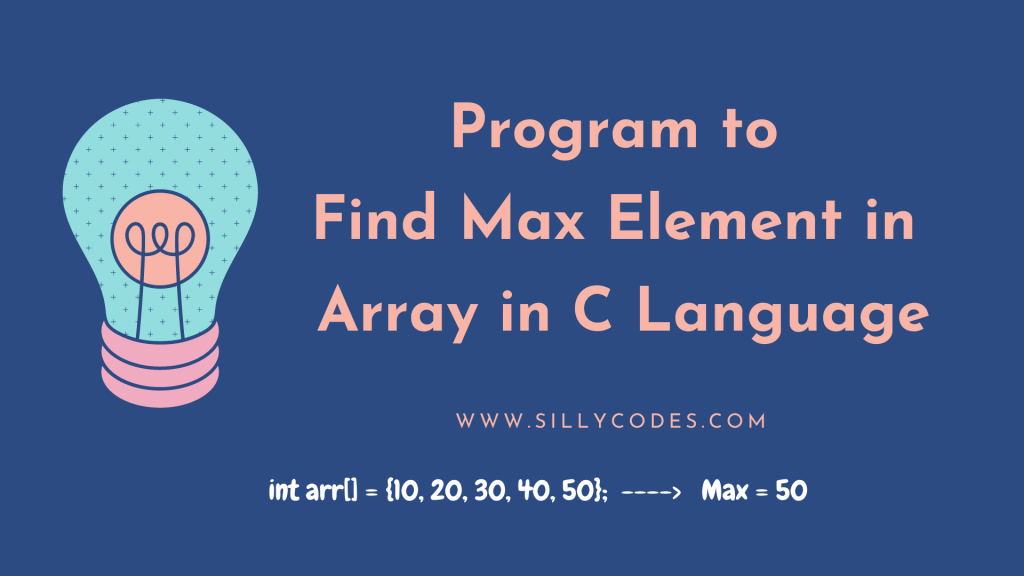
Max Element in Array in C Program Description:
Program to find the Max Element in Array in C programming language. We ask the user to enter the elements of the array and find the Maximum element.
Expected Input and Output:
Input:
1 2 3 4 5 6 |
Please enter array elements numbers[0] : -24 numbers[1] : 98 numbers[2] : 0 numbers[3] : 45 numbers[4] : 72 |
Output:
1 |
Maximum element in array is : 98 |
Prerequisites:
We need to know the basics of the C Array, Functions, and Passing Arrays to Function.
Find Max Element in Array in C Program Explanation:
- Declare an integer array named numbers. The numbers array holds 5 elements ( size of the array) and also declares a variable i to traverse the array.
- Take the input from the user and update the numbers array. Use a For Loop to iterate over the array and take the input from the user using standard input and output functions ( printf and scanf) and update the array elements.
- Create a variable called max. Initialize the max with INT_MIN. The value of the INT_MIN is -2147483648. Learn more about the INT_MIN at the Size and Limits of datatypes article.
- To find the Maximum element(max) we need to traverse the array element by element and update the max variable if any element is larger than the max. which is numbers[i] > max
- Continue above loop till size-1. and once the loop is terminated the variable max contains the Max element in the array.
- Display the max on the console.
Find Max Element in Array in C Algorithm:
- Declare array int values[5]; and int i
- Prompt the user to input the values for the array
- FOR
i = 0 to
SIZE-1 ( Loop )
- Read the value for values[i]
- Initialize max variable i.e max = INT_MIN;
- FOR
i = 0 to
SIZE-1 ( Loop )
- update max( max = data[i]) if the data[i] > max is true
- Print the max value on the console
Program to Find Max Element in Array in C:
Here is the C Code for the above algorithm
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
/* Program to find Maximum number of Array sillycodes.com */ #include <stdio.h> #include <limits.h> int main() { // declare the 'numbers' array int numbers[5]; int i; // User input for array elements printf("Please enter array elements\n"); for(i = 0; i < 5; i++) { printf("numbers[%d] : ", i); scanf("%d", &numbers[i]); } // initialize the 'max' variable with 'INT_MIN' from limits.h file // Value of INT_MIN = -2147483648 // Look at - https://sillycodes.com/size-and-limits-datatypes-in-c-programming/ for info. int max = INT_MIN; // find the Maximum of array elements for(i = 0; i < 5; i++) { // check for 'max' if( numbers[i] > max) { // update the 'max' value max = numbers[i]; } } // print the result printf("Maximum element in array is : %d\n", max); return 0; } |
Program Output:
Now compile the program
$ gcc max-array.c
We used the GCC compiler to run the program. The above command generates the a.out file, which is an executable file. Run the executable file.
1 2 3 4 5 6 7 8 9 |
$ ./a.out Please enter array elements numbers[0] : -24 numbers[1] : 98 numbers[2] : 0 numbers[3] : 45 numbers[4] : 72 Maximum element in array is : 98 $ |
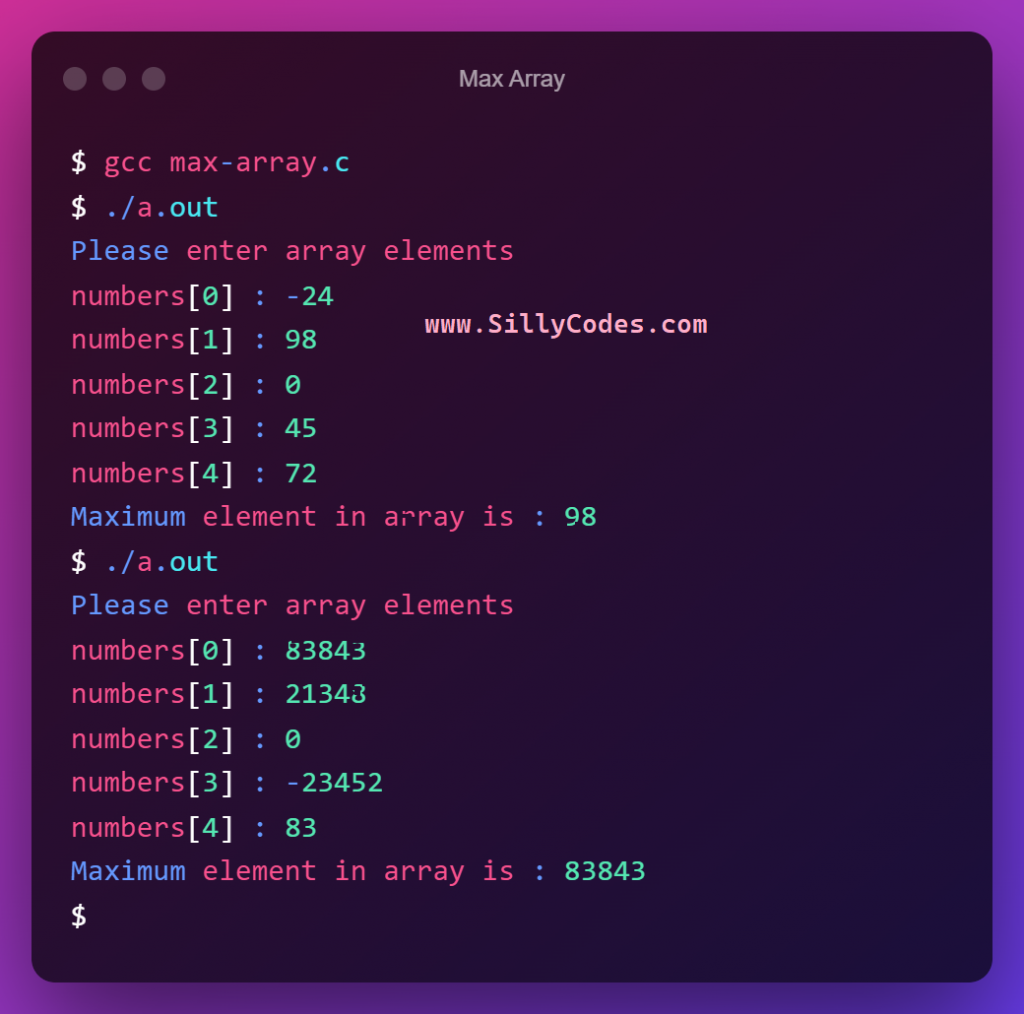
Try another example
1 2 3 4 5 6 7 8 9 |
$ ./a.out Please enter array elements numbers[0] : 83843 numbers[1] : 21348 numbers[2] : 0 numbers[3] : -23452 numbers[4] : 83 Maximum element in array is : 83843 $ |
Method 2: Maximum Element in an Array in C using Functions:
Let’s divide the above program into the functions and each and every function will perform a specific task like reading the values from the user and calculating the maximum value.
The following program uses two functions
- The
read() function.
- Prototype of the read() function – void read(int numbers[], int size);
- The read function takes two arguments – numbers array and its size. The read() function prompts the user for array elements and updates the number array.
- The
maximum() function.
- Prototype of the maximum() function – int maximum(int numbers[], int size);
- Similar to the read() function, The maximum function also takes two formal arguments one is the input array numbers[] and the sizeof the number’s array.
- The maximum() function finds the maximum element in the array by comparing all elements.
- Finally, It returns the maximum element in the array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
/* Program to find Maximum number of Array sillycodes.com */ #include <stdio.h> #include <limits.h> /** * @brief - Read the elements from INPUT * * @param numbers - 'numbers' Array * @param size - size of the array */ void read(int numbers[], int size) { int i; printf("Please enter array elements\n"); // Read the input from the user for(i = 0; i < size; i++) { printf("numbers[%d] : ", i); scanf("%d", &numbers[i]); } } /** * @brief - Find the maximum element of the array * * @param numbers - Input Array * @param size - Size of 'numbers' array * @return int - Maximum element of 'numbers' array */ int maximum(int numbers[], int size) { // initialize the 'max' variable with 'INT_MIN' from limits.h file // Value of INT_MIN = -2147483648 // Look at - https://sillycodes.com/size-and-limits-datatypes-in-c-programming/ for info. int i, max = INT_MIN; // find the Maximum of array elements for(i = 0; i < 5; i++) { // check for 'max' if( numbers[i] > max) { // update the 'max' value max = numbers[i]; } } return max; } int main() { // declare the 'numbers' array int numbers[5]; // Take the array elements from the user read(numbers, 5); // Call 'maximum' function to get the max element int max = maximum(numbers, 5); // print the result printf("Maximum element in array is : %d\n", max); return 0; } |
Program Output:
Here is the output of the program.
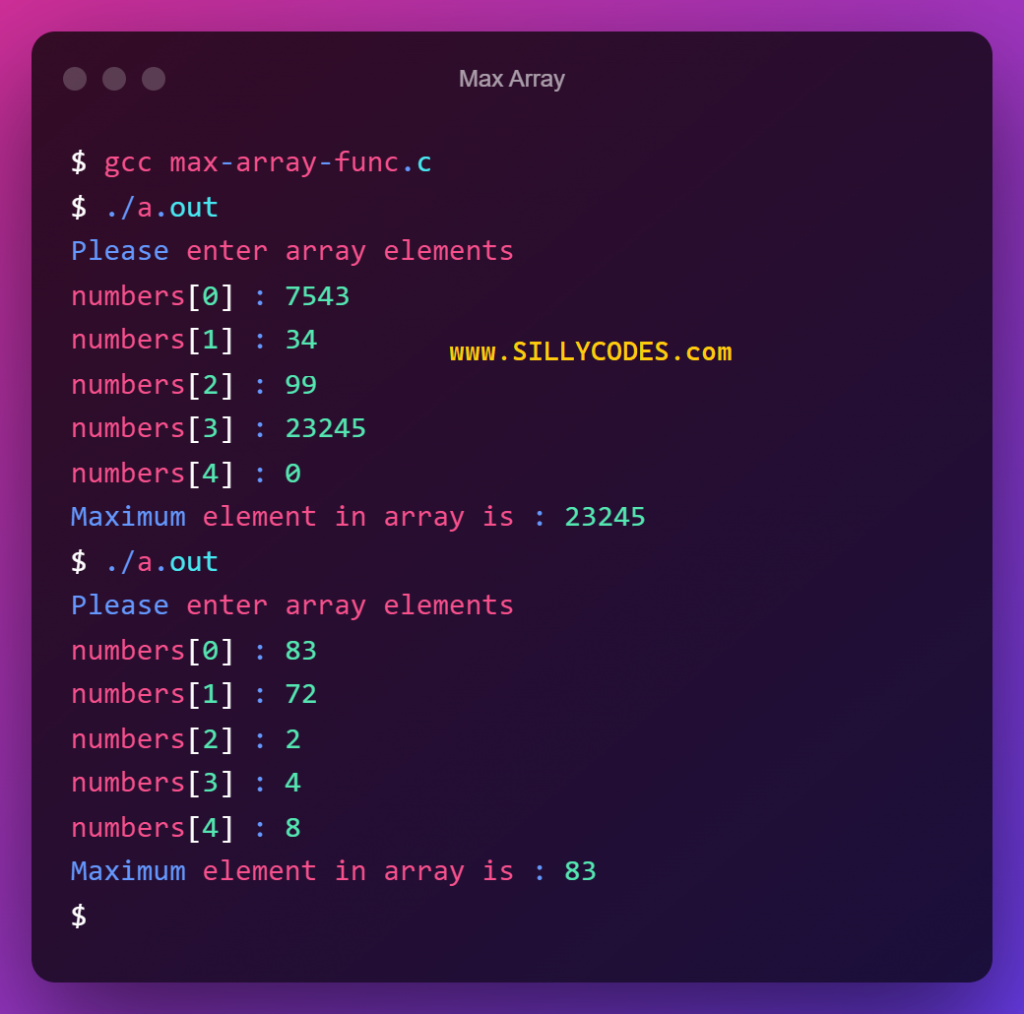
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
$ gcc max-array-func.c $ ./a.out Please enter array elements numbers[0] : 7543 numbers[1] : 34 numbers[2] : 99 numbers[3] : 23245 numbers[4] : 0 Maximum element in array is : 23245 $ ./a.out Please enter array elements numbers[0] : 83 numbers[1] : 72 numbers[2] : 2 numbers[3] : 4 numbers[4] : 8 Maximum element in array is : 83 $ |
We are getting the expected results.
Related Array Programs:
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
1 Response
[…] C Program to Find Maximum Element in Array […]