Program to Generate Random Array in C Language
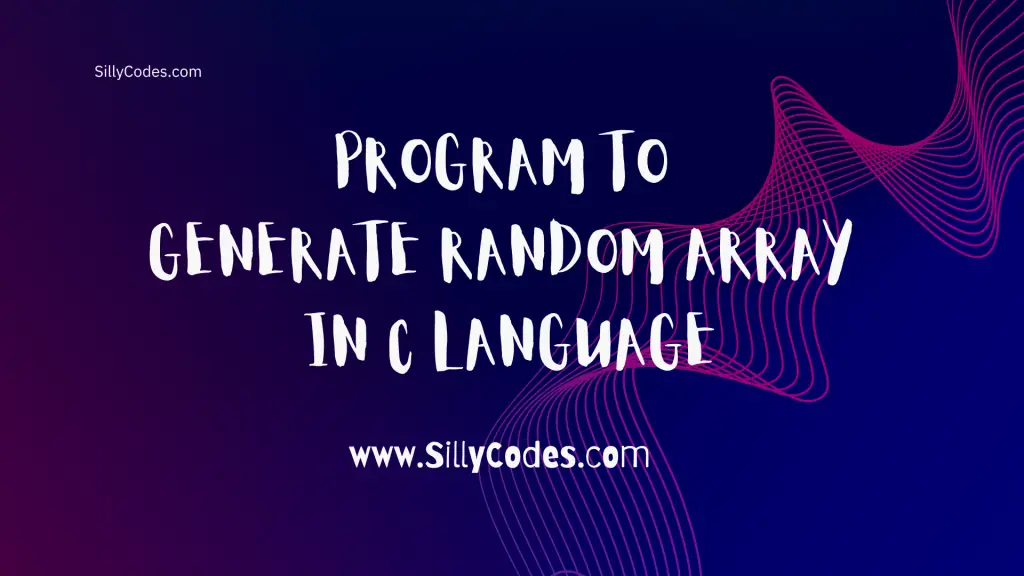
Program Description:
Write a Program to generate a random array in c programming language. The program should accept the desired array size from the user and generate an array with random values of specified size.
📢 This program is part of the Array Practice Programs Series.
let’s look at the program example input and output
Example Input and Output:
Input:
Enter the desired size for Array(1-1000): 5
Output:
Generated Random Array:
Value of randPtr[0]=37
Value of randPtr[1]=89
Value of randPtr[2]=56
Value of randPtr[3]=71
Value of randPtr[4]=93
Prerequisites:
Please go through the following articles to better understand the program.
Explanation to Generate Random Array in C Programming:
How to generate a Random array in C:
Create a function called generateRandomArray() to generate a random array. Here is the prototype of the generateRandomArray() function.
int * generateRandomArray(int size)
The generateRandomArray() function takes size as the formal argument and generates the random integer array of size elements.
This function also returns the generated array(pointer to the generated array – int *) back to the caller.
We use the rand() function from the stdlib.h header file to generate the random elements. Here is the syntax of the rand() function.
int rand(void)
We pass the time(0) to the srand() function to generate random numbers. The time(0) is equal to the number of seconds elapsed from the epoch ( 00:00:00 UTC, January 1, 1970.). If you don’t pass the random seed to the srand() function, Then there is a possibility of getting the same values every time you run the program.
srand(time(0));
Store the random values on a static integer array called randomArray.
Why We need to use a Static array:
The default storage class for local variables is automatic, and they will be destroyed once the function returns. Because we need our random array ( randomArray) to be available even after the generateRandomArray()function returns, So we must use a static integer array that will be alive throughout the program’s life.
Finally, Return the randomArray back to the caller.
Program Explanation:
Start the program by defining a macro named MAXSIZE. This macro holds the maximum allowed size of the array. Please change this value if you want to use large arrays.
Take the user input and store it in size variable. and check if the size variable is within the present array bounds ( 1-MAXSIZE)
Call the generateRandomArray() function and pass the size(Desired size) as an argument. The generateRandomArray() function returns an integer pointer that holds the random array. So store the return value in an integer pointer( randPtr).
Now, The random array is stored in the randPtr. Iterate over it and display the contents on the console using a for loop.
Program to Generate Random Array in C Language:
Here is the program to generate random array elements in c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
/*     Program to Generate Random Array in C     www.sillycodes.com */  #include <stdio.h> #include <time.h> #include <stdlib.h>  // Max Size of Array #define MAXSIZE 1000  /** * @brief - Generate a Random Array and return it * * @param size - Desired Size of the array * @return int* - Generated Random Array */ int * generateRandomArray(int size) {     // create a static Array with 'MAXSIZE'     // static as we need 'randomArray' to stay alive after this function returned.     static int randomArray[MAXSIZE];      // seed the time(0) to rand function     srand(time(0));      // create random int and store in randomArray     for(int i=0; i<size; i++){         randomArray[i] = rand()%100+1;     }      // return the generated random array     return randomArray; }  int main() {       // Declare an integer pointer variable to hold random array     int *randPtr;      // desired size of array     int size;     printf("Enter the desired size for Array(1-%d): ", MAXSIZE);     scanf("%d", &size);      // check for array bounds     if(size >= MAXSIZE || size <= 0)     {         // display error and return         printf("Invalid Array Size(%d), Please try again \n", size);         return 0;     }      // call the 'generateRandomArray()' function     randPtr = generateRandomArray(size);      // print the random array     printf("Generated Random Array: \n");     for(int i=0; i<size; i++)     {         printf("Value of randPtr[%d]=%d\n", i, randPtr[i]);     }      return 0; } |
📢 Make sure to include the necessary header files to use the time and rand and srand functions.
Program Output:
Let’s compile and run the program using your compiler. We are using the GCC compiler to compile the program.
Test case 1:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
$ gcc randArray.c $ ./a.out Enter the desired size for Array(1-1000): 15 Generated Random Array: Value of randPtr[0]=60 Value of randPtr[1]=68 Value of randPtr[2]=79 Value of randPtr[3]=36 Value of randPtr[4]=72 Value of randPtr[5]=62 Value of randPtr[6]=67 Value of randPtr[7]=61 Value of randPtr[8]=3 Value of randPtr[9]=40 Value of randPtr[10]=33 Value of randPtr[11]=29 Value of randPtr[12]=27 Value of randPtr[13]=77 Value of randPtr[14]=100 $ |
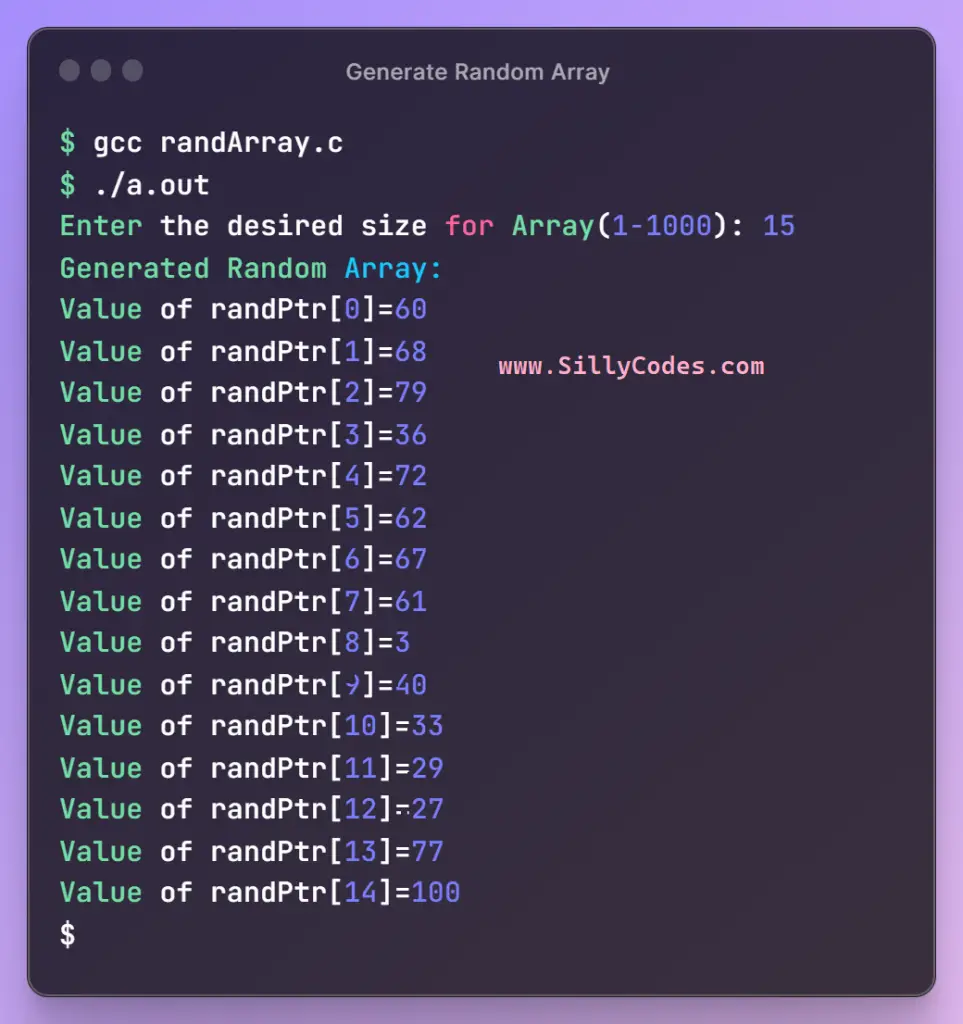
As you can see from the above output, the User provided the desired size as the 15 elements and the generateRandomArray() function generated the array with 15 random values.
Let’s try another example
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter the desired size for Array(1-1000): 5 Generated Random Array: Value of randPtr[0]=37 Value of randPtr[1]=89 Value of randPtr[2]=56 Value of randPtr[3]=71 Value of randPtr[4]=93 $ |
As expected, the program generated a random array with a size 5.
Test case 2: Negative Cases:
1 2 3 4 |
$ ./a.out Enter the desired size for Array(1-1000): -5 Invalid Array Size(-5), Please try again $ |
If the user enters a negative value, the program will display an error message. In the above example, the user entered the size as the
-5, So the program displayed the error message. (
Invalid Array Size(-5), Please try again)
1 2 3 4 |
$ ./a.out Enter the desired size for Array(1-1000): 1481 Invalid Array Size(1481), Please try again $ |
Similarly, If the input size is greater than the MAXSIZE (i.e 1000), Then the program displays an error message.
Related Programs:
- C Tutorials Index
- C Programs Index – 300+ Programs
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
- C Program to Merge Two Arrays
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
- C Program to Delete Duplicate Elements in an Array
- C Program to Left Rotate Array by ‘N’ Times