Custom strstr function implementation in C Language
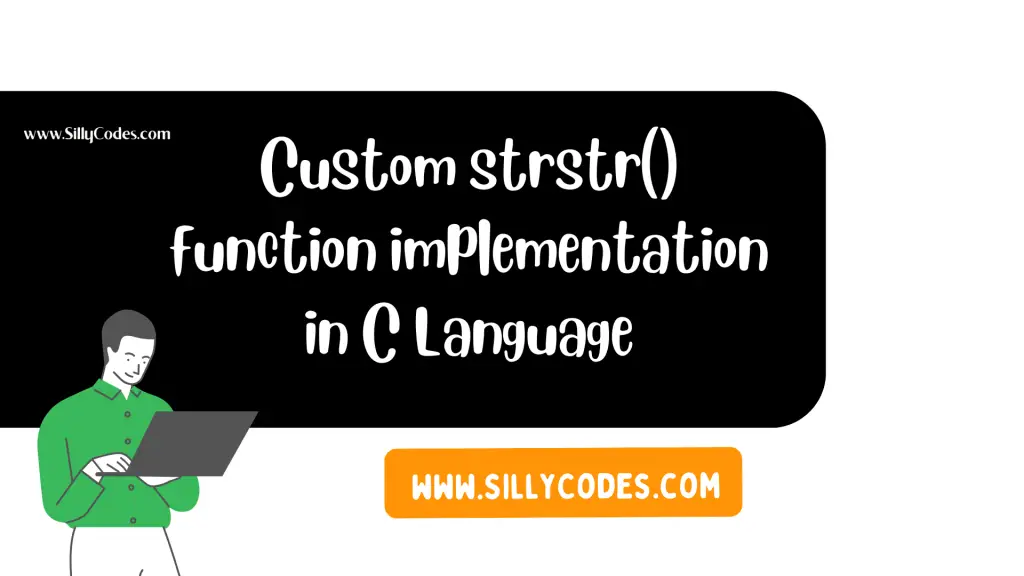
Program Description:
We are going to look at strstr function implementation in c programming language. As we already know the strstr()function is used to search for a substring in the string. The program will accept two strings from the user, one for the main string and one for the substring. It searches for the substring in the main string and returns the pointer to the first occurrence substring.
📢 This Program is part of the C String Practice Programs series
Here is the example input and output of the program.
Program Input and Output:
Input:
Enter a main string : we pass through this world but once
Enter the substring(String to search in main string): this
Output:
FOUND! Substring:'this' is found in mainString at 'this world but once'
The substring this is found in the main string and the program is returned the pointer to it.
Prerequisites:
We are going to use the String, Functions, and arrays in C language. So please go through the following articles to refresh your concepts.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
strstr() function syntax:
Before looking at the custom implementation of strstr() function, Here is the prototype of the strstr() library function
1 |
char * strstr( const char * mainStr, const char * subStr); |
The strstr() function is available as part of the string.h and The strstr() function searches for the substring( subStr) in the main string( mainStr) and returns the pointer to the first occurrence of the substring.
If the subStr is found in the mainStr, It returns the character pointer to the first occurrence of the subStr. If the subStr is not found, Then it returns a NULLpointer.
So we need to create a custom strstr() function which takes two character pointers as formal arguments and returns a character pointer.
Custom strstr function implementation in C Program Explanation:
Let’s look at the step-by-step instructions to implement the custom strstr() function
- Start the main() function by declaring the two strings and let’s say the main string as mainStr and the substring as subStr. The size of both strings is equal to SIZE macro.
- Read the two strings from the user and store them in mainStr and subStr respectively. We use the gets function to read the strings. The gets() function doesn’t do the boundary checks so be cautious while using the gets function.
- Now to search for the substring in the main string, We are going to create a function called mystrstr. The mystrstr() function takes two character pointers( char *mainStr, char *subStr) and returns a character pointer. – i.e char * mystrstr(const char * mainStr, const char * subStr)
- Within the
mystrstr() function, To search for the substring, Iterate over the main string(
mainStr) until the
*mainStr reaches the terminating NULL character(
NULL). – i.e
while( *mainStr != NULL)
- At each iteration, Check if the current character of mainStr is equal to the first character of the subStr ( i.e (*mainStr == *subStr))
- If the above condition is
true, Then search for all characters of the
subStr in the
mainStr. We need to use another while loop to iterate over the contents of the
mainStr and
subStr. We are using two temporary pointers
s1 and
s2 to compare the characters.
- Check if the *s1(s1 = mainStr)is not matching to *s2 ( s2 = substr), If so then the subString is not found. Otherwise, continue to check all elements of the subStr by incrementing the s1 and s2 pointers.
- Once the above while loop is completed, Check if we iterated over all elements of the substring ( use * s2 == NULL ). If we iterated the complete substring( subStr), then we found the substring in the main string. return the mainStr pointer( char *) back to the caller.
- Call the mystrstr() function from the main() function and pass the main string and substring and store the result on the result pointer – i.e char * result = mystrstr(mainStr, subStr);
- Finally check the result pointer and display the results on the console.
Program to implement custom strstr() function C Language:
Let’s convert the above logic into the C code.
Here is the custom implementation of the strstr() function in c.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 |
/*     program to implement the strstr function in c     sillycodes.com */  #include <stdio.h>  // Max Size of string #define SIZE 100   /** * @brief  - search for the 'subStr' in 'mainStr' and return the pointer to first occurrence * * @param mainStr  - main string - haystack * @param subStr  - sub string - needle * @return char*  - if 'subStr' found returns the pointer to first occurrence, Otherwise, Returns NULL */  char * mystrstr(const char * mainStr, const char * subStr) {     char *s1, *s2;      // Iterate over the main string     while( *mainStr != NULL)     {         // search for the first character in substring         if(*mainStr == *subStr)         {             // The first character of substring is matched.             // Check if all characters of substring.             s1 = mainStr;             s2 = subStr;              while (*s1 && *s2 )             {                 if(*s1 != *s2)                     break;      // not matched                  s1++;                 s2++;             }                         // we reached end of subStr             if(*s2 == NULL)                 return mainStr;         }          // go to next element         mainStr++;     }      return NULL; }    int main() {      // declare two strings with 'SIZE'     char mainStr[SIZE], subStr[SIZE];      // Take the main string from the user     printf("Enter a main string : ");     gets(mainStr);      // take the sub string     printf("Enter the substring(String to search in main string): ");     gets(subStr);      // call the custom strstr function (mystrstr() function)     char * result = mystrstr(mainStr, subStr);         // check the 'result'     if(result == NULL)     {         printf("NOT FOUND! Substring:'%s' is NOT found in mainString\n", subStr);     }     else     {         printf("FOUND! Substring:'%s' is found in mainString at '%s'\n", subStr, result);     }      return 0; } |
Here are the details of the custom strstr() function( mystrstr function).
- char * mystrstr(const char * mainStr, const char * subStr)
- Function Name – mystrstr
- Arguments list – (char *, char *)
- Return Value – char *
The mystrstr() function searches for the subStr in the mainStr and returns the pointer to the first occurrence of the subStr in the mainStr. If the subStr is not found, Then it returns the NULL.
Program Output:
Let’s compile and run the program.
Test Case 1: Positive case:
1 2 3 4 5 6 7 |
$ gcc mystrstr.c  $ ./a.out Enter a main string : we pass through this world but once Enter the substring(String to search in main string): this FOUND! Substring:'this' is found in mainString at 'this world but once' $ |
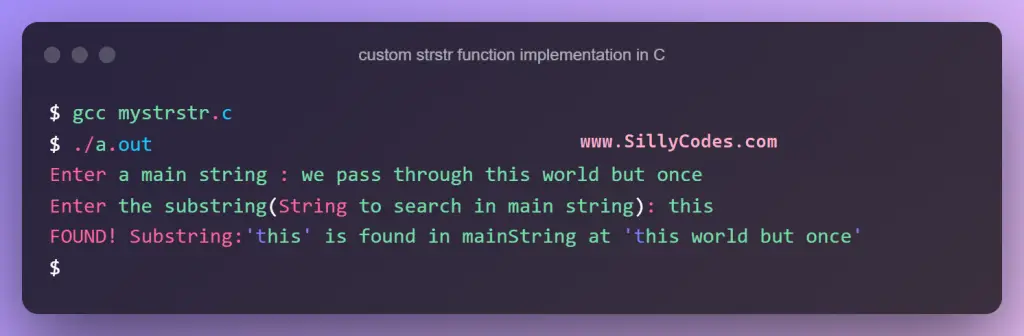
The substring this is found in the string and the mystrstr() function returned the character pointer to first occurrence of the substring this. So the resultant string is this world but once.
Let’s try another example.
1 2 3 4 5 |
$ ./a.out Enter a main string : learn programming online at sillycodes.com Enter the substring(String to search in main string): programming FOUND! Substring:'programming' is found in mainString at 'programming online at sillycodes.com' $ |
Here are also, The substring programming is found in the main string and the resultant string is programming online at sillycodes.com'
Test Case 2: Negative Cases:
1 2 3 4 5 |
$ ./a.out Enter a main string : Git rebase Enter the substring(String to search in main string): push NOT FOUND! Substring:'push' is NOT found in mainString $ |
In the above example, The substring push is not found in the main string Git rebase. So the mystrstr() function returned the NULL pointer back to the main() function.
Related String Practice Programs:
- C Tutorials Index
- C Programs Index
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
- C Program to Count Frequencies of each character in a string
- C Program to Find Highest Frequency character in a String
- C Program to Find Lowest Frequency Character in a String
- C Program to Remove Vowels from a String
- C Program to Remove Consonants from a String
- C Program to Sort String in Ascending order
- C Program to Sort String in Descending Order
- Sort String in Ascending and Descending order using Qsort()
- C Program to Find First Occurrences of a character in a String
- C Program to Find Last Occurrences of a character in a string
1 Response
[…] Custom strstr() function implementation in C […]