Add Two Numbers using Pointers in C Language
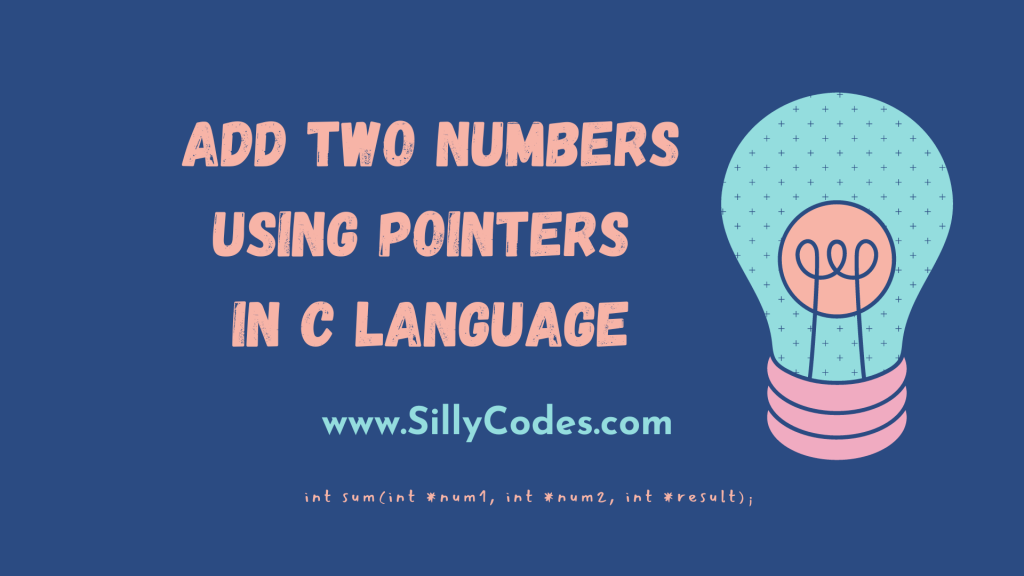
Add Two Numbers using Pointers in C Program Description:
Write a Program to add two numbers using pointers in c programming language. The program should accept two integers from the user and add the given integers by using the pointers.
📢This program is part of the Pointers Practice programs series.
Let’s look at the excepted input and output of the program
Excepted Input and Output:
Input:
Enter value for first number(num1): 44
Enter value for second number(num2): 63
Output:
Sum of 44+63 = 107
Prerequisites of the program:
It is recommended to go through the introduction to the pointers in C and how to use the address of operator and dereference operator in c to better understand the following program.
Add Two Numbers using Pointers in C Program Explanation:
We are going to create a function named sum, Which calculates the sum of the given numbers. Let’s look at the step-by-step instructions of the program.
- Start the program by creating a function called
sum. The
sum function takes three integer pointer variables as formal arguments, They are
num1,
num2, and
result.
- Here is the prototype of the sum() function – void sum( int *num1, int *num2, int *result)
- The sum function calculates the sum of the num1 and num2 and updates the calculated sum value in the result variable.
- As all three formal arguments are pointer variables, We need to use the Dereference or Indirection Operator(‘*’) to get the value at the pointer. For example, we need to use the *num1 to get the value of the num1.
- Finally, Update the result variable with the sumvalue using *result = *num1 + *num2;
- As the variables are passed by the address/reference, All changes made in the sum function will reflect in the caller. So we no need to return anything back to the caller.
- In the main() function, Take the two integer values from the user and store them in the variables num1 and num2 using scanf function.i.e use the address of operator
- Call the sum() function and pass the variables
num1,
num2, and
result with addresses. i.e use the address of operator(&)
- sum(&num1, &num2, &result);
- The above function call will calculate the sum of num1 and num2 and stores the result in result variable. So to display the sum of two numbers on the console using a printf function.
- Stop the program.
Let’s convert the above algorithm into the C program.
Program to Add Two Numbers using Pointers in C Language:
Here is the program to add two numbers using pointers in c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
/* Program to add two numbers using pointers in c sillycodes.com */ #include <stdio.h> /** * @brief - Add 'num1' and 'num2' and update 'result' * * @param num1 - first number * @param num2 - second number * @param result - result */ void sum(int *num1, int *num2, int * result) { // dereference the numbers to get values *result = *num1 + *num2; } int main() { // declare the variables int num1, num2, result; // Take two numbers from user printf("Enter value for first number(num1): "); scanf("%d", &num1); printf("Enter value for second number(num2): "); scanf("%d", &num2); // call the sum function // pass addresses of num1, num2, result sum(&num1, &num2, &result); // print the results printf("Sum of %d+%d = %d\n", num1, num2, result); return 0; } |
Note that we are calling the sum() function with addresses(sum(&num1, &num2, &result);). So all changes made in the sum() function will be visible in the main() function.
📢We’re passing num1 and num2 as addresses to the sum() function, but since we’re not changing them, we can also pass them as regular variables. That being said, in order to demonstrate and understand the pointer variables, we passed them as pointers.
Program Output:
Let’s compile and Run the program using GCC compiler (Any compiler)
Test 1:
1 2 3 4 5 6 |
$ gcc add-two-numbers-pointer.c $ ./a.out Enter value for first number(num1): 44 Enter value for second number(num2): 63 Sum of 44+63 = 107 $ |
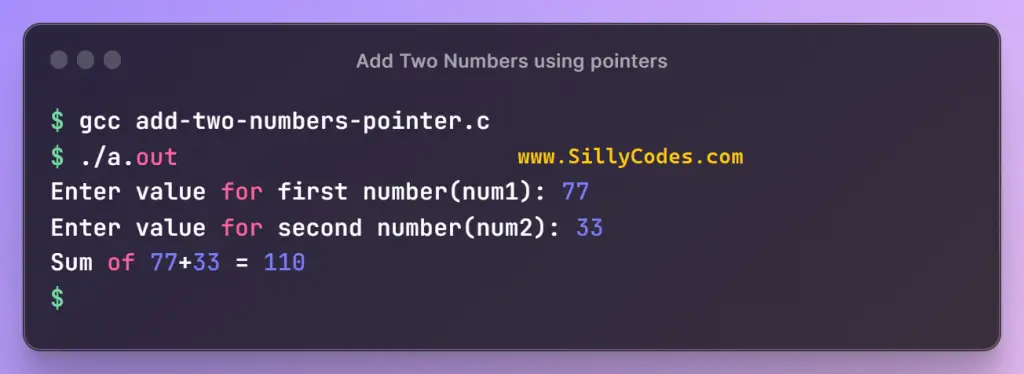
In this example, the user provided the numbers 44 and 63 and the program used pointers to perform the addition of two numbers and returned the result as 107.
Let’s try few more examples
Test 2:
1 2 3 4 5 6 7 8 9 10 11 |
$ $ ./a.out Enter value for first number(num1): 77 Enter value for second number(num2): 33 Sum of 77+33 = 110 $ $ ./a.out Enter value for first number(num1): -900 Enter value for second number(num2): 333 Sum of -900+333 = -567 $ |
As you can see the program is properly calculating the sum of given numbers and providing the output.
Related Programs:
- C Tutorials Index
- C Programs Index – 300+ Programs
- Size of Pointer variable in C Language
- C Program to generate Fibonacci series using Recursion
- C Program to print first N Natural Numbers using Recursion
- C Program to calculate the sum of N Natural Numbers using Recursion
- C Program to calculate the Product of N Natural Numbers using Recursion
- C Program to Count the Number of digits in a Number using Recursion
- C Program to Calculate the Sum of Digits using Recursion
- C Program to Product the Number of digits in a Number using Recursion
- C Program to Reverse a Number using Recursion.
- C Program to check whether the number is Palindrome using Recursion
- C Program to Calculate LCM using Recursion.
1 Response
[…] Program to Add Two Numbers using Pointers in C […]