Delete Element from Array in C Language
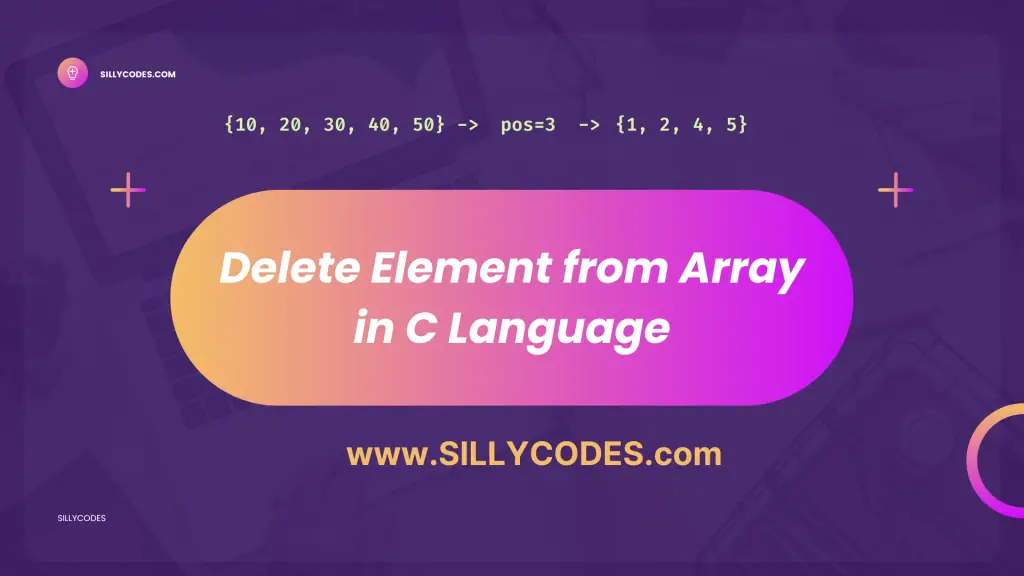
Program Description:
In our earlier article, We have looked at the program to Insert an element in an array and In today’s article, we will write a Program to Delete Element from Array in C Langauge. The program accepts an array and element position from the user and deletes the element at the given position.
Example Input and Output:
Input:
Enter desired array size(1-1000): 10
Please enter array elements(10) : 34 82 98 23 45 67 12 34 56 76
Enter the position(1-10) of element to be deleted : 5
Output:
Original Array: 34 82 98 23 45 67 12 34 56 76
Array after deleting element: 34 82 98 23 67 12 34 56 76
If you observe, The element at the position 5 ( position starts from 1, so position = index + 1) which is 45 is removed from the resultant array.
Prerequisites:
It is recommended to know the basics of the C Arrays and Functions. Please read the following articles to learn more about them.
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- How to pass arrays to functions in C
- Functions in C Language
- 2D arrays in C with Example programs
Algorithm to Delete Element from Array in C:
Take the array values( numbers) and the element position to be deleted from the user.
Let’s say the array name is numbers array. And the element position to be deleted is position, and the Array size is size.
To Delete the element at the position from the numbers array of size size.
- Shift all the elements in the numbers array that has an index greater than ( position-1) to the left by one position.
- This will overwrite the element at the index ( position-1) and removes it from the array.
- Finally, Reduce the array size by 1. decrement size variable – size– ;
This way we can Remove or Delete an element from the array in the C programming language.

📢This program is part of the Array Practice Programs in C language.
Delete Element from Array in C Program Explanation:
- Declare the numbers array with 1000 elements, Also declare other variables like i, size, iNum, and position.
- Take the desired array size from the user and store it in size variable.
- Define a
read() function to update the array elements with user-provided values.
- Prompt the user to provide the array elements and update the array.
- Call the read() function from the main() function and pass the numbers array and its size as the actual arguments. The read() function updates the numbers array with user values.
- Take the position of the element to be deleted from the user. The position is not equal to index, ( index = position-1)
- Define another function called
display() to print the array elements on the standard output.
- Use a For Loop to traverse the array and print the elements
- To delete element at the
position. Shift all elements greater than the
position to left by one position.
- Use For loop to traverse from the position-1 till the size-1 – for(i=position-1; i<size; i++)
- Replace the element at the i with i+1. – numbers[i] = numbers[i+1];
- Repeat the above step-7 till we reach the end of the array.
- As we deleted an element, Reduce the array size by 1 – Decrement the size variable – size--;
- Print the array after deleting the element at position on the console by calling the display() function.
Program to Delete Element from Array in C:
Here is the program to delete an element from an array in c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
/*     Program to delete an element in array     Author: SillyCodes.com */  #include <stdio.h>  /** * @brief  - Read the user input and update the 'numbers' array * * @param numbers - 'numbers' array * @param size    - size of the 'numbers' array */ void read(int numbers[], int size) {     int i;     // User input for array elements     printf("Please enter array elements(%d) : ", size);     for(i = 0; i < size; i++)     {         // printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     } }  /** * @brief  - Display the 'numbers' array * * @param numbers - Array * @param size    - Array size */ void display(int numbers[], int size) {     int i;     for(i = 0; i < size; i++)     {         printf("%d ", numbers[i]);        }     printf("\n"); }    int main() {      // declare the 'numbers' array     int numbers[1000];     int i, size, iNum, position;      printf("Enter desired array size(1-1000): ");     scanf("%d", &size);      // User input for array elements     read(numbers, size);      // Take the position(delete) from the user     printf("Enter the position(1-%d) of element to be deleted : ", size);     scanf("%d", &position);      // check for array bounds     if(position>size || position<1)     {         printf("Invalid Position, Position should be 1-%d, Try again \n", size);         return 0;     }      // Print the original array     printf("Original Array: ");     display(numbers, size);      // Shift all elements >position to left by one position     // start from 'position-1'(index = position-1) go till 'size'     for(i=position-1; i<size; i++)     {         // move 'i+1' to 'i'         numbers[i] = numbers[i+1];     }      // decrement the size     size--;      // Print the sorted array     printf("Array after deleting element: ");     display(numbers, size);      return 0; } |
Program Output:
Let’s compile and run the program
$ gcc delete-elem-arr.c
Run the program.
Test Case 1: Normal Cases:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter desired array size(1-1000): 5 Please enter array elements(5) : 1 2 3 4 5 Enter the position(1-5) of element to be deleted : 2 Original Array: 1 2 3 4 5 Array after deleting element: 1 3 4 5 $ ./a.out Enter desired array size(1-1000): 10Â Â Â Â Â Â Please enter array elements(10) : 34 82 98 23 45 67 12 34 56 76 Enter the position(1-10) of element to be deleted : 5 Original Array: 34 82 98 23 45 67 12 34 56 76 Array after deleting element: 34 82 98 23 67 12 34 56 76 $ |
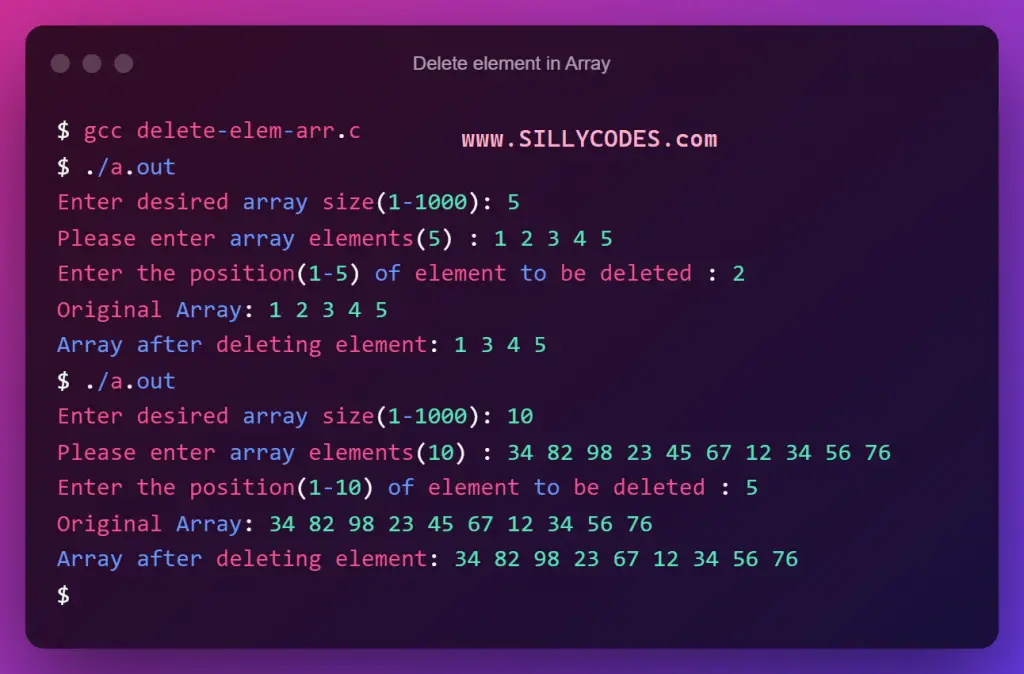
As we can see from the above output, The program is deleted the element from the array.
For example, If the input array is 1 2 3 4 5 and we want to delete the element at the position 2, Then the resultant array is 1 3 4 5. ( index = position -1)
Test Case 2: Boundary Cases:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter desired array size(1-1000): 5 Please enter array elements(5) : 90 80 70 60 50 Enter the position(1-5) of element to be deleted : 1 Original Array: 90 80 70 60 50 Array after deleting element: 80 70 60 50 $ ./a.out Enter desired array size(1-1000): 4 Please enter array elements(4) : 88 55 44 11 Enter the position(1-4) of element to be deleted : 4 Original Array: 88 55 44 11 Array after deleting element: 88 55 44 $ |
Test Case 3: Negative Cases:
1 2 3 4 5 6 7 8 9 10 11 12 |
// Negative Cases $ ./a.out Enter desired array size(1-1000): 5 Please enter array elements(5) : 10 20 30 40 50 Enter the position(1-5) of element to be deleted : 7 Invalid Position, Position should be 1-5, Try again $ ./a.out Enter desired array size(1-1000): 4 Please enter array elements(4) : 1 2 3 4 Enter the position(1-4) of element to be deleted : 0 Invalid Position, Position should be 1-4, Try again $ |
If the user enters the wrong position, Then the program displays an error message saying the Invalid Position.
Delete Element from Array using a user-defined function in C Language:
In the above program, We used two functions read() and display() to read and print the array elements. Let’s create another function named deleteAt to delete an element from the array.
The deleteAt the function should take an input array, the position, Then deletes the element at the position and provides the resultant array back to the caller.
Here is the rewritten version of the above program, Where we defined the deleteAt function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 |
/*     Program to delete an element in array using function     Author: SillyCodes.com */  #include <stdio.h> int deleteAt(int numbers[], int size, int position);   /** * @brief  - Read the user input and update the 'numbers' array * * @param numbers - 'numbers' array * @param size    - size of the 'numbers' array */ void read(int numbers[], int size) {     int i;     // User input for array elements     printf("Please enter array elements(%d) : ", size);     for(i = 0; i < size; i++)     {         // printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     } }  /** * @brief  - Display the 'numbers' array * * @param numbers - Array * @param size    - Array size */ void display(int numbers[], int size) {     int i;     for(i = 0; i < size; i++)     {         printf("%d ", numbers[i]);        }     printf("\n"); }    int main() {      // declare the 'numbers' array     int numbers[1000];     int i, size, iNum, position;      printf("Enter desired array size(1-1000): ");     scanf("%d", &size);      // User input for array elements     read(numbers, size);      // Take the position(delete) from the user     printf("Enter the position(1-%d) of element to be deleted : ", size);     scanf("%d", &position);      // check for array bounds     if(position>size || position<1)     {         printf("Invalid Position, Position should be 1-%d, Try again \n", size);         return 0;     }      // Print the original array     printf("Original Array: ");     display(numbers, size);      // call 'deleteAt'     size = deleteAt(numbers, size, position);      // Print the sorted array     printf("Array after deleting element: ");     display(numbers, size);      return 0; }  /** * @brief  - Delete element at 'position' from 'numbers' array * * @param numbers  - Array * @param size    - size of 'numbers' array * @param position - element position to delete * @return int    - Array size after deletion */ int deleteAt(int numbers[], int size, int position) {     int i;     // Shift all elements >position to left by one position     // start from 'position-1'(index = position-1) go till 'size'     for(i=position-1; i<size; i++)     {         // move 'i+1' to 'i'         numbers[i] = numbers[i+1];     }      // decrement the size and return     return --size; } |
📢 As the deleteAt function is defined after calling it from the main() function, We need to declare the function at the start of the program ( Forward declaration of the function).
- int deleteAt(int numbers[], int size, int position);
Delete an Array Element Program Output:
Compile and Run the program using any compiler. We are using the GCC Compiler on ubuntu Linux system.
Test Case 1: Positive Cases:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
$ gcc delete-elem-arr-func.c $ ./a.out Enter desired array size(1-1000): 10 Please enter array elements(10) : 43 87 98 78 23 12 34 54 65 76 Enter the position(1-10) of element to be deleted : 3 Original Array: 43 87 98 78 23 12 34 54 65 76 Array after deleting element: 43 87 78 23 12 34 54 65 76 $ ./a.out Enter desired array size(1-1000): 5 Please enter array elements(5) : 111 222 333 444 555 Enter the position(1-5) of element to be deleted : 1 Original Array: 111 222 333 444 555 Array after deleting element: 222 333 444 555 $ ./a.out Enter desired array size(1-1000): 5 Please enter array elements(5) : 0 0 0 1 0 Enter the position(1-5) of element to be deleted : 4 Original Array: 0 0 0 1 0 Array after deleting element: 0 0 0 0 $ |
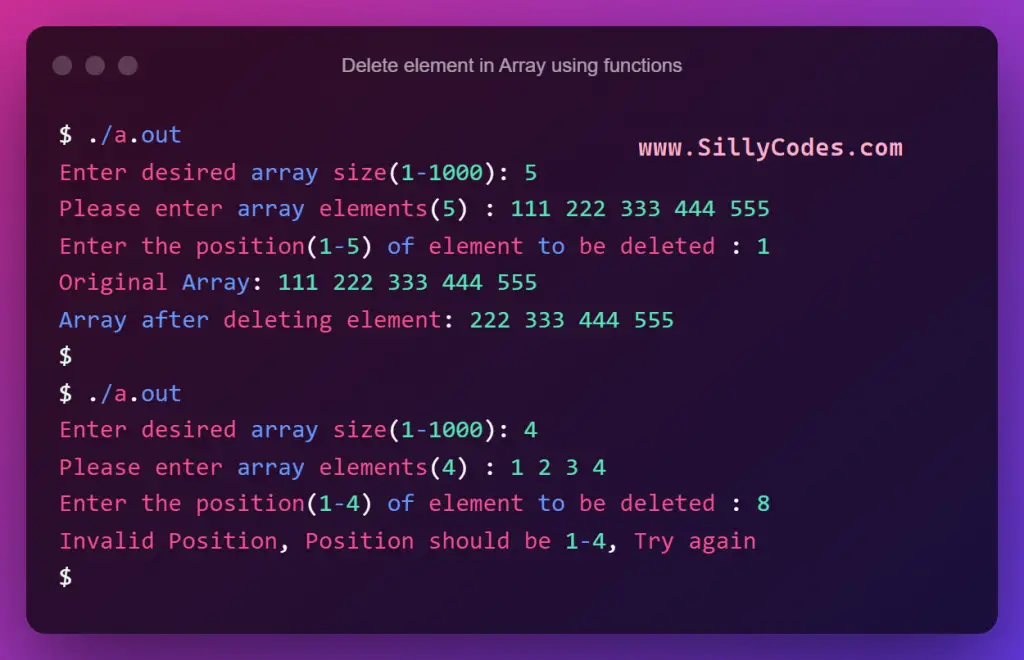
Test case 2: Negative Cases:
1 2 3 4 5 6 7 8 9 10 11 12 |
// Negative $ ./a.out Enter desired array size(1-1000): 4 Please enter array elements(4) : 1 2 3 4 Enter the position(1-4) of element to be deleted : 8 Invalid Position, Position should be 1-4, Try again $ ./a.out Enter desired array size(1-1000): 7 Please enter array elements(7) : 1 1 1 1 1 1 1 Enter the position(1-7) of element to be deleted : 0 Invalid Position, Position should be 1-7, Try again $ |
As you can see from the above output, The program is generating the desired results.
Related Programs:
- C Program to find minimum element in Array
- C Program to Insert an element in Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Find Maximum Element in Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- Index – C Language Practice Programs
- C Tutorials Index – Step by step tutorials to master C Langauge
2 Responses
[…] Delete an element from an Array […]
[…] C Program to Delete an Element in Array […]