Prime number in C using function
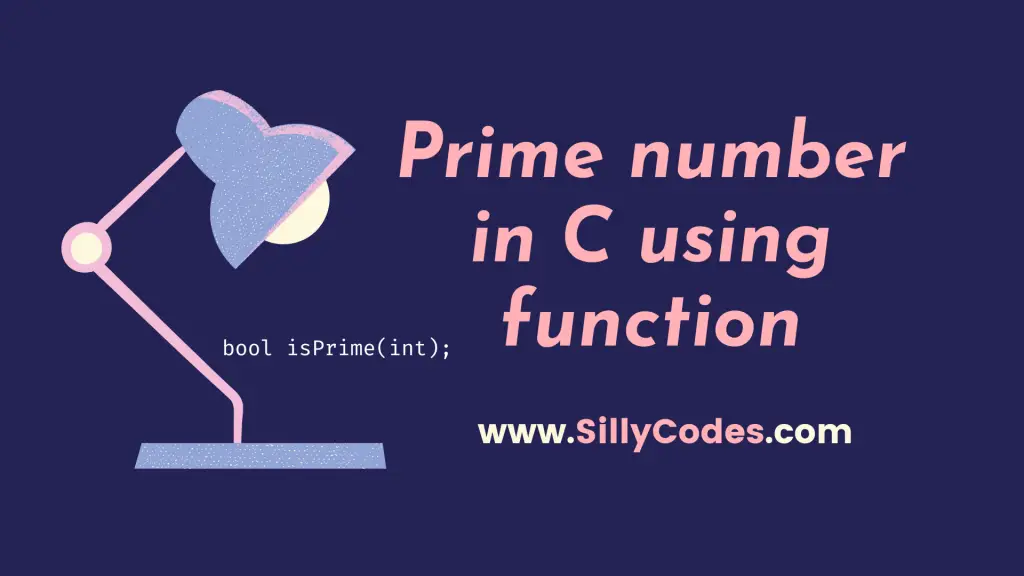
Program Description:
Write a program to check the given number is a Prime number in C using function, We will create a function called isPrime(), The function accepts a number as input and returns true if the number is a prime number. Otherwise, The function should return false.
Excepted Input and Output:
Input:
Enter a positive numbers: 89
Output:
Number 89 is a Prime Number
Pre-Requisites:
It is recommended to go through the C functions and Loops to understand the following program.
Prime number in C using function Algorithm:
- Accept a number from the user and store it in a variable named num
- Check if the num is a Negative number if (num <= 0) display an error message if num is a negative number.
- Create a function called
isPrime. The
isPrime function is used to check the prime number.
- Prototype of isPrime – bool isPrime(int);
- The isPrime accepts an integer number as input ( n)
- Returns true – if the n is prime number
- Returns false – if the n is not a prime number.
- This function returns a Boolean, So we need to include the stdbool.h header file. (We can also return int)
- Call the isPrime function from the main function and pass the input number num.
- The isPrime function will check to see if the given integer n is evenly divisible by any number by going through all numbers from 2 to num/2 ( n/2). The number n is not a prime number if it can be divided evenly by any of the aforementioned numbers. Otherwise, the number n is a prime number.
- Display the results on console based on the isPrime function return value.
Prime number in C using function Program:
Let’s write the program
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
/* Program: Check Prime Number in C using a function Author: Sillycodes.com */ #include<stdio.h> #include<stdbool.h> bool isPrime(int n); /* main() function */ int main() { // Take the input from the user int num; printf("Enter a positive numbers: "); scanf("%d", &num); // check for negative numbers if (num <= 1) { printf("Error: Invalid Input, Try again \n"); return 0; } // call the 'isPrime' with one integer if(isPrime(num)) { printf("Number %d is a Prime Number \n", num); } else { printf("Number %d is not a Prime Number \n", num); } return 0; } /* function_name: isPrime arguments_list : (int n) return_value : bool */ bool isPrime(int n) { int i; // Iterate through 2 to n/2 // We can also iterate only upto sqrt(n) for(i=2; i<=n/2; i++) { // Check if 'i' is factor of 'n' if(n%i == 0) { // 'i' is factor of `n` // so `n` is not a Prime Number return false; } } return true; } |
Prototype Details of the isPrime function
- bool isPrime(int n);
- Function_name: isPrime
- Arguments_list : (int n)
- Return_value : bool
📢 As the isPrime function is called before defining, So we need to declare the function using function declaration or forward declaration.
bool isPrime(int n);
Program Output:
Compile and Run the program.
$ gcc isPrime.c
Test Case 1: Check Prime Numbers – Positive Numbers
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter a positive numbers: 7 Number 7 is a Prime Number $ ./a.out Enter a positive numbers: 27 Number 27 is not a Prime Number $ ./a.out Enter a positive numbers: 12 Number 12 is not a Prime Number $ ./a.out Enter a positive numbers: 89 Number 89 is a Prime Number $ |
Test Case 2: Negative Numbers
1 2 3 4 5 6 7 |
$ ./a.out Enter a positive numbers: -45 Error: Invalid Input, Try again $ ./a.out Enter a positive numbers: -2 Error: Invalid Input, Try again $ |
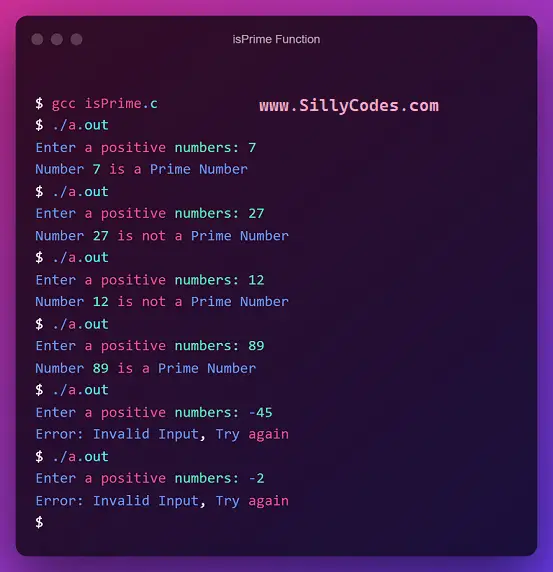
Programs Related to Prime number in c using a function:
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- C Program Check Prime Number [Mutliple Methods]
- C Program to Convert Decimal Number to Binary Number
- C Program to Convert Binary Number to Decimal Number
- C Program to Convert Octal Number to Decimal Number
- C Program to Calculate the Factorial of Number
- C Program to Calculate the Power of Number
- 100+ C Practice Programs
- C Tutorials Index
1 Response
[…] Prime number program using Function […]