Product of N Numbers using Recursion in C Language
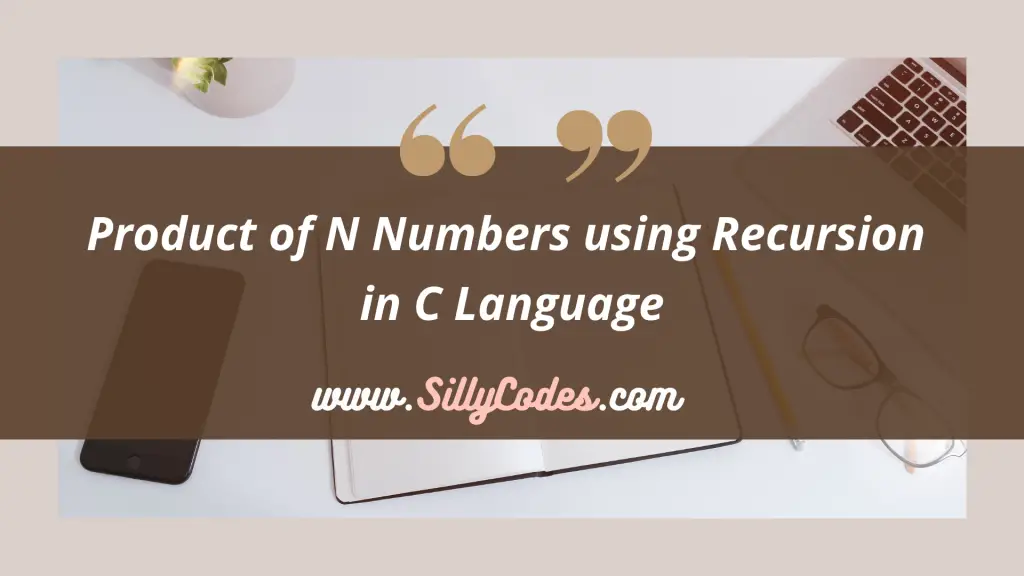
Product of N Numbers using Recursion in C Program Description:
Write a Program to calculate the Product of N Numbers using Recursion in C programming language. The program should accept a positive number and calculate the product of first n natural numbers using the recursive.
Example Input and Output:
Input:
Enter a Number (n): 10
Output:
Product of first 10 numbers is : 3628800
Pre-Requisites:
It is recommended to know the basics of the Functions in C Language, Recursion in Programming, and Relational Operators.
Product of N Numbers using Recursion in C Explanation:
- Take the input from the user and store it in a variable named n.
- Check if the number
n is a negative number, If so display the error message
ERROR! Enter a Valid Number. And ask for the user input again.
- We use the Goto Statement to jump back to the program start.
- use goto INPUT; and Label INPUT:
- The user will be prompted for the input until a valid number is provided.
- Create a Function named
productNNumbers. The
productNNumbers takes an integer
n as input and calculates the product of the first
n numbers using the recursion and returns the result back to the caller. Here are the details of the ProductNNumber function.
- As we are using recursion, We need to define the base condition to stop the recursive calls. Here function base condition is n == 1. So if the n reaches the 1, return 1
- Otherwise (if n > 1), Then make a recursive call to the productNNumbers with n-1. Multiply the result with n. – n * productNNumbers(n-1)
- The above recursive calls continue until the n value reaches 1, That is when the base condition will be evaluated as true and recursive functions will return one by one.
- Call the productNNumbers function from the main function. Pass the given number n to productNNumbers function. Store the return value in result variable. ( result = productNNumbers(n);)
- Finally, Display the results on the console.
Product of N Numbers using Recursion in C Program:
Here is the Program of Product of N Natural Numbers in C language using the recursive method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/* Program to calculate the product of first N Numbers using recursion in C */ #include <stdio.h> /** * @brief Calculate Product of first n natural numbers using recursion * * @param n - numbers * @return int - product of first n numbers */ int productNNumbers(int n) { // base condition if(n == 1) return 1; // recursive function call return(n * productNNumbers(n-1)); } int main() { // User Input INPUT: // goto label int n, result = 1; printf("Enter a Number (n): "); scanf("%d", &n); if(n <= 0) { printf("ERROR! Enter a Valid Number \n"); goto INPUT; } // call the productNNumbers function result = productNNumbers(n); printf("Product of first %d numbers is : %d\n", n, result); return 0; } |
The prototype details of the productNNumbers function
- Function_name: productNNumbers
- Argument_List: int
- Return_value: int
Program Output:
Compile the program
gcc product-n-numbers.c
Run the Program.
Test Case 1: Positive Numbers
1 2 3 4 5 6 7 |
$ ./a.out Enter a Number (n): 10 Product of first 10 numbers is : 3628800 $ ./a.out Enter a Number (n): 5 Product of first 5 numbers is : 120 $ |
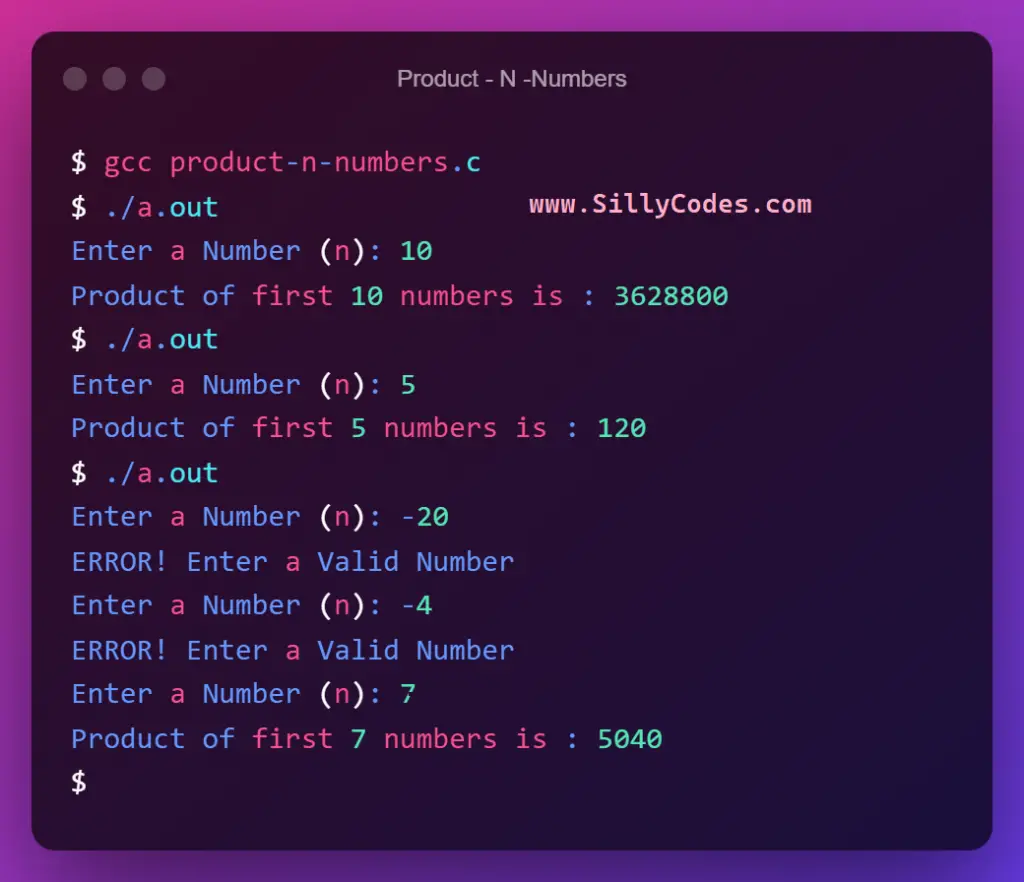
Test Case 2: Negative Numbers:
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Number (n): -20 ERROR! Enter a Valid Number Enter a Number (n): -4 ERROR! Enter a Valid Number Enter a Number (n): 7 Product of first 7 numbers is : 5040 $ |
We are getting the excepted results. Program is displaying the error message if the user enters a negative number and asking for the user input again.
📢 If you need to calculate the product for large numbers, Then use the
long int and
long long int for
result and
n variables.
Related: Limits and Sizes of Datatypes in C
Related Programs:
- Print First N Natural Numbers using Recursion
- Sum of First N Natural Numbers using Recursion
- C Program to Calculate Sum of First N natural numbers
- C Program to Reverse a Number
- C Program to Calculate Sum of Digits of a Number
- C Program to Calculate Product of Digits of a Number
- C Program to Count the number of Digits of a Number
- C Program to Multiply without using Multiplication operator (*)
- C Program to generate Fibonacci Series up to a given number
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- 100+ C Practice Programs
- C Tutorials Index
4 Responses
[…] Product of N natural Number using Recursion C Program […]
[…] C Program to calculate the Product of N Natural Numbers using Recursion […]
[…] C Program to calculate the Product of N Natural Numbers using Recursion […]
[…] C Program to calculate the Product of N Natural Numbers using Recursion […]