Program to print first n natural numbers using recursion in C
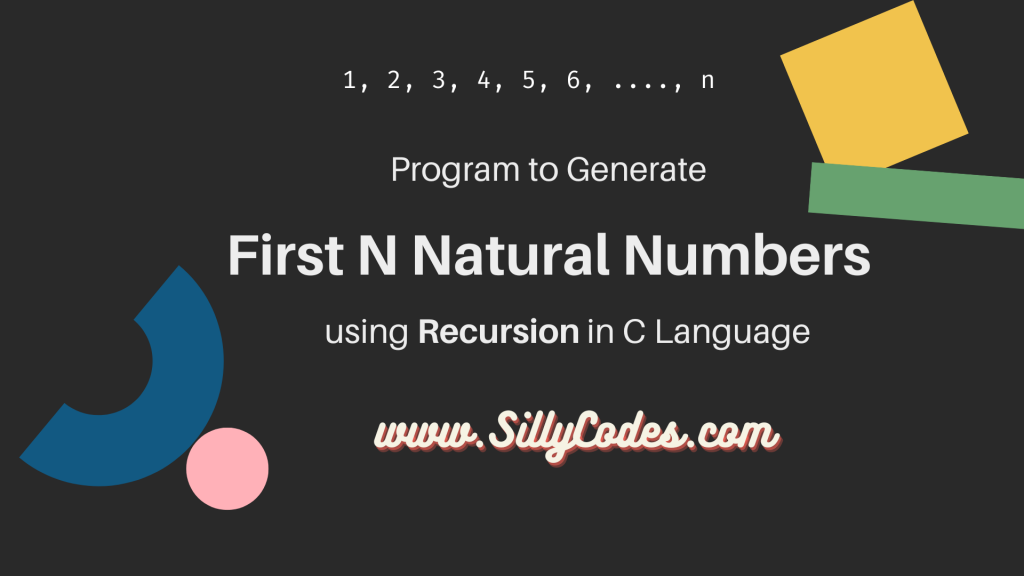
First N natural numbers using Recursion in C Program Description:
Write a program to print the first n natural numbers using recursion in C programming language. This program should take an integer number from the user ( n) and print the first n natural numbers up to n i.e given number.
📢 Related Program: We have already looked at the program to print n natural numbers using Loops.
Excepted Input and Output:
Input:
Enter a Number: 6
Output:
Numbers are : 1 2 3 4 5 6
Pre-Requisites:
It is recommended to know the basics of Functions and Recursion. Please go through the following articles learn more about them
First N Natural Numbers using Recursion in C Algorithm:
- Start the program by taking the user input and storing the input number in the variable n
- Check for a negative number and display the error message if the
n is a negative number. and allow the user to provide the input again using the Goto Statement
- We use the goto INPUT; statement to jump to the Label INPUT:. which prompts the user for input again.
- Create a function named
nNumbers, Which takes an integer number as input and uses recursion to print all numbers from
1 to
n.
- As the nNumbers function is using the recursion to print the natural numbers, We need to have the base condition. The nNumbers function base condition is if(n == 0). The recursion will stop once the value of the n becomes zero.
- If the n is not zero, Then we will call the nNumbers function with n-1. which is <strong>nNumbers</strong>(n-1);
- The above recursive calls (function calls) continue until we reach the base condition. And before returning, every function will print the n.
- Once the above recursive function finishes The n natural numbers will be printed on the console.
- Call the nNumbers function from the main function.
First N Natural Numbers using Recursion in C Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
/*     Program to print first n natural numbers using recursion in c */ #include <stdio.h>  /** * @brief - print first n natural numbers using recursion * * @param n - number */  void nNumbers(int n) {     // base condition     if(n == 0)         return;         // recursive call     nNumbers(n-1);     printf("%d ", n); }   int main() {     // User Input     INPUT:        // goto label     int n;     printf("Enter a Number: ");     scanf("%d", &n);      if(n <= 0)     {         printf("Error, Please enter positive number \n");         goto INPUT;     }      // call the nNumbers function     printf("Numbers are : ");     nNumbers(n);      printf("\n");     return 0; } |
Here are the prototype details of the nNumbers function.
- void nNumbers(int n);
- function_name: nNumbers
- arguments_list: int
- return_type: void
Program Output:
Let’s compile and run the program. To compile use the following command
gcc <filename.c>
$ gcc n-numbers-recursive.c
which generates an executable file named a.out. Run the executable using the ./a.out command like below.
Test Case 1: Positive Numbers:
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a Number: 15 Numbers are : 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 $ ./a.out Enter a Number: 6 Numbers are : 1 2 3 4 5 6 $ ./a.out Enter a Number: 2 Numbers are : 1 2 $ |
We are getting the excepted results When user provided the input number as 15, The program generated the first 15 natural numbers.
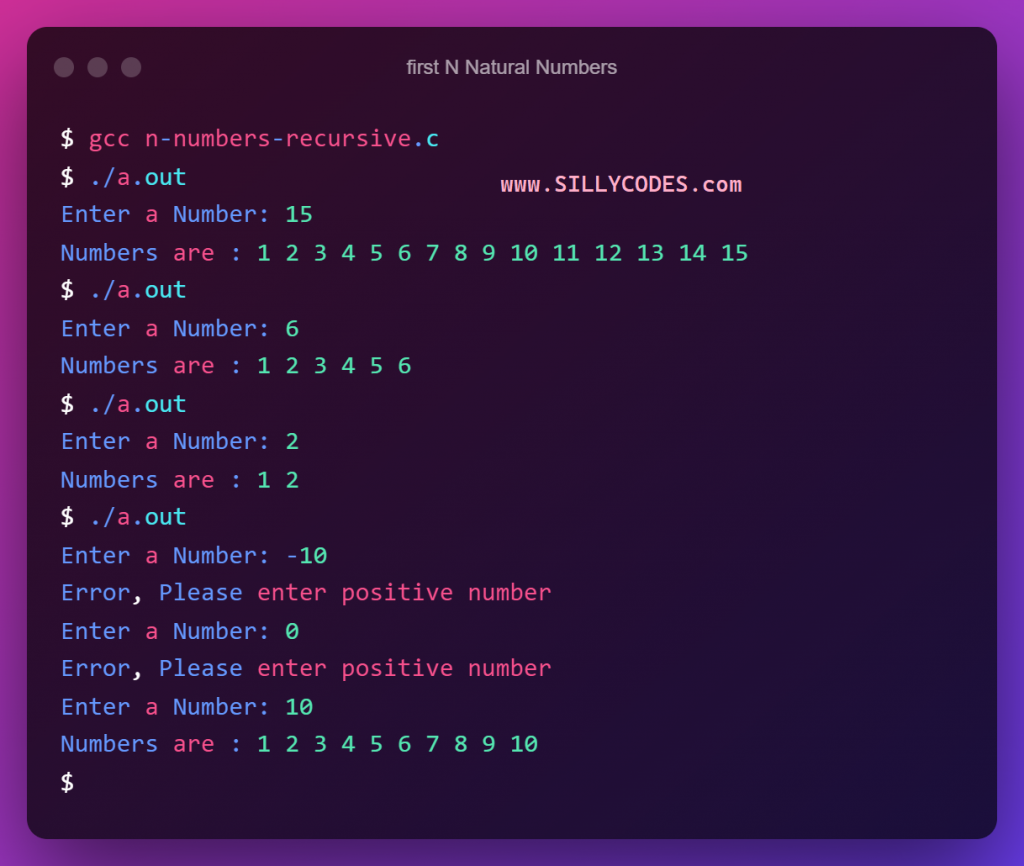
Test Case 2: Negative Numbers:
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Number: -10 Error, Please enter positive number Enter a Number: 0 Error, Please enter positive number Enter a Number: 10 Numbers are : 1 2 3 4 5 6 7 8 9 10 $ |
As we can see from the above output, Whenever a user enters a negative number, Program displays an error message Error, Please enter positive number and asks for the user’s input again. This process continues until valid input from the user.
4 Responses
[…] Print First N Natural Numbers using Recursion […]
[…] C Program to Print First N Natural Numbers using Recursion […]
[…] C Program to print first N Natural Numbers using Recursion […]
[…] C Program to print first N Natural Numbers using Recursion […]