Program to check Palindrome String in C Language
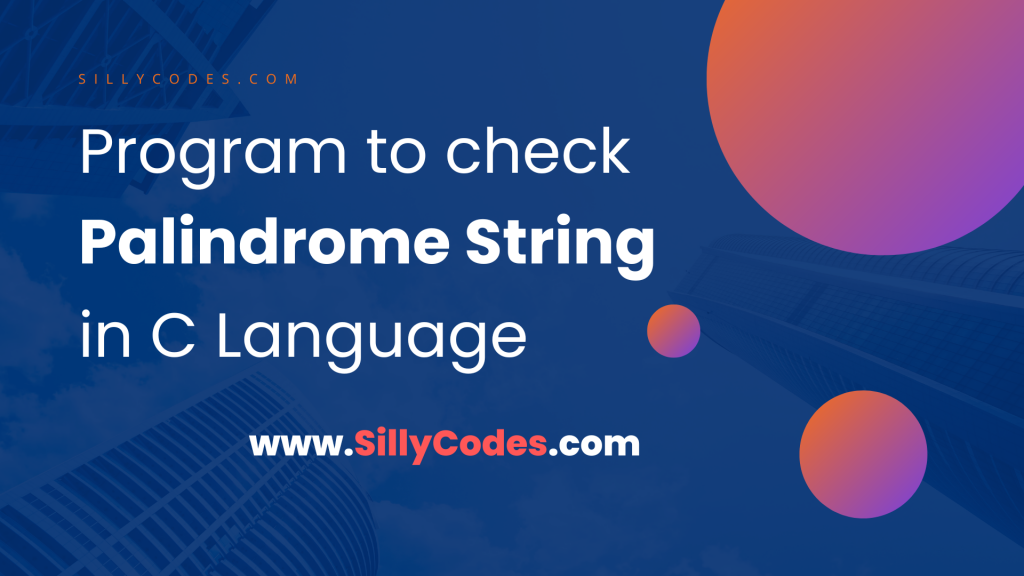
Program Description:
Write a Program to Check Palindrome String in C Programming language. The Program will accept a string from the user and check whether the string is a palindrome string.
📢 This Program is part of the C String Practice Programs series
What is a Palindrome string?
If the Reverse of a String is equal to the Original String, Then the string is a Palindrome.
Example Input and Output of the Program:
Input:
Enter a string : racecar
Output:
String 'racecar' is a Palindrome
Prerequisites:
We need to know the basics of the C-Strings, C-Arrays, and Functions in C in order to understand the following program better.
We are going to look at the a few methods to check the palindrome in this article.
- Two-Pointer Approach(Iterative Method): Check a Palindrome string using the two-pointer approach(Without using extra space)
- User-Defined Functions: Check Palindrome String using a user-defined function.
- Reversing the string: Compare the Original String and Reverse String to detect if the string is a palindrome.
Palindrome String in C Program Explanation:
We are going to use the Two Pointer approach to check if the given string is a Palindrome string. We create two variables start and end. The start variable points to the first character in the string and the end variable points to the last character in the string.
Then go through the array and compare the characters at the start and end indices and if the characters at these indices are matched(or Equal), Then go to the next elements, Increment the start Index and Decrement the end Index. If didn’t find any mismatch, Then the string is a palindrome string.
Here is a step-by-step explanation of the program.
- Declare a string named inputStr with the SIZE ( The SIZE is a constant with 100 value).
- Take the user input and update the inputStr string.
- Create two variables, The start and end variables. The start variable points to the first character in the string and the end variable points to the last character in the string. i.e start = 0, end = strlen(inputStr)-1
- Iterate over the string and compare the characters pointed by the
start and
end indices.
- At each iteration, Check if the inputStr[start] is not equal to inputStr[end], If it is true, Then the inputStr is Not a Palindrome string. Print the results and break the loop – i.e if(inputStr[start] != inputStr[end])
- If the above condition is false, Then continue to the next iteration.
- Increment the start index – start++
- Decrement the end index – end--
- Once step 4 is completed successfully, Then we didn’t find any character anomalies, so the string is a Palindrome string.
- Print the results on the console.
Check a String is a Palindrome using the Iterative Method:
Let’s convert the above algorithm to C program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
/*     program to check a string is a Palindrome     sillycodes.com */  #include<stdio.h> #include<string.h>  // Max Size of string const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     int start, end, temp;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);      // maintain two indices, one(start) at start, second(end) at end     start = 0;     end = strlen(inputStr)-1;         // Iterate over the string     // Check the characters at indices start, end and increment 'start' and decrement 'end'     for( ; start < end; start++, end--)     {         // Check if the characters at index 'start' and 'end' are equal         if(inputStr[start] != inputStr[end])         {             // Not Matched. Not a palindrome             printf("String '%s' is not a Palindrome \n", inputStr);             return 0;         }     }      // if we reached here, The string is palindrome     printf("String '%s' is a Palindrome \n", inputStr);      return 0; } |
Program Output:
Compile and Run the Program.
Test 1: When the string is a Palindrome
1 2 3 4 5 |
$ gcc palindrome-string.c $ ./a.out Enter a string : tenet String 'tenet' is a Palindrome $ |
1 2 3 4 |
$ ./a.out Enter a string : racecar String 'racecar' is a Palindrome $ |
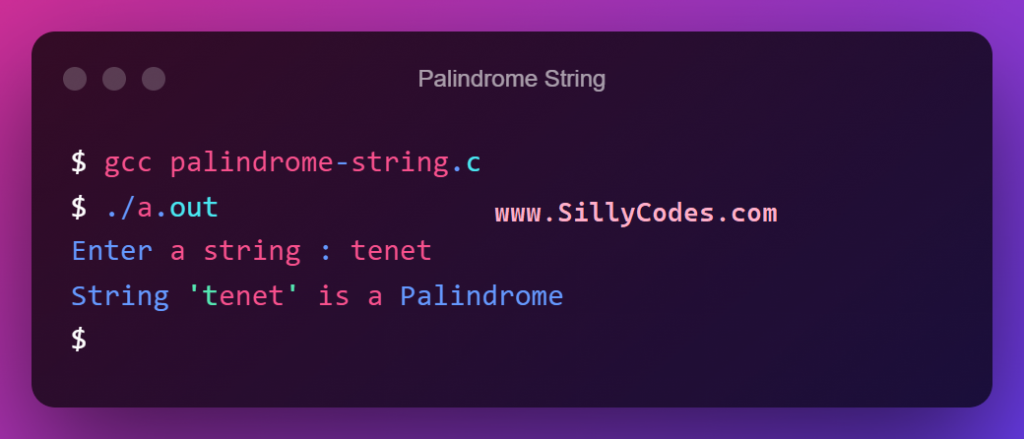
As we can see from the above output, The strings tenet and racecar are palindromes.
Test 2: When the string is not a palindrome.
1 2 3 4 |
$ ./a.out Enter a string : CProgramming String 'CProgramming' is not a Palindrome $ |
The CProgramming string is not a Palindrome.
Palindrome String in C using user-defined functions:
Let’s move the Palindrome check logic to a function named isPalindrome() so that we can reuse and take all advantage of the functions.
Here is the modified program to check palindrome string in C using a user-defined function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 |
/*     program to check a string is a Palindrome     sillycodes.com */  #include<stdio.h> #include<string.h>  // Max Size of string const int SIZE = 100;  /** * @brief  - Verifies if the 'inputStr' is a Palindrome string. * * @param inputStr - Input string * @return int  - Returns 1 if the 'inputStr' is Palindrome, Otherwise returns Zero. */ int isPalindrome(char * inputStr) {     // maintain two indices, one(i) at start, second(j) at end     int i = 0;     int j = strlen(inputStr)-1;         // Iterate over the string     // Check the characters at indices i, j and increment 'i' and decrement 'j's     for( ; i < j; i++, j--)     {         // Check if the characters at index 'i' and 'j' are equal         if(inputStr[i] != inputStr[j])         {             // Not Matched. Not a palindrome             return 0;         }     }      // Palindrome string.     return 1; }  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     int i, j, temp;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);      if(isPalindrome(inputStr))     {         // The string is palindrome         printf("String '%s' is a Palindrome \n", inputStr);     }     else     {         // String is not a Palindrome         printf("String '%s' is not a Palindrome \n", inputStr);     }      return 0; } |
We defined a function named isPalindrome() function. with the prototype int isPalindrome(char * inputStr). The isPalindrome() function accepts formal arguments and checks if it is a palindrome. Function returns One(1) if the inputStr is a palindrome, Otherwise function returns Zero(0).
Program Output:
Let’s Compile and Run the program.
1 2 3 4 5 6 7 8 |
$ gcc palindrome-string.c $ ./a.out Enter a string : madam String 'madam' is a Palindrome $ ./a.out Enter a string : sillycodes String 'sillycodes' is not a Palindrome $ |
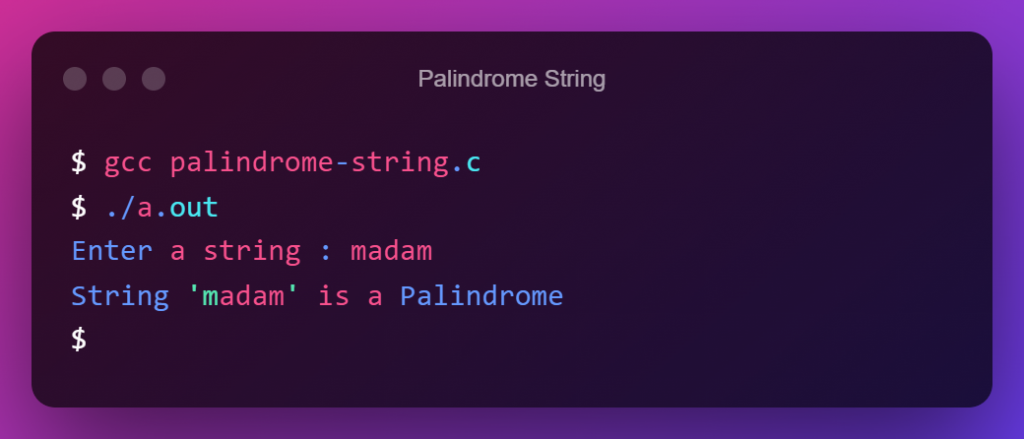
Method 2: Check Palindrome String in C by Reversing String:
By comparing the original and reversed strings, we can determine if the given string is a palindrome string.
Here is a brief explanation of the program.
- Copy the input string to a New string let’s say it as Reverse string( revStr)
- Call the stringReverse() function with revStr to get the reverse of the input string.
- Compare the input string(
inputStr) and reversed string(
revStr) using the strcmp() function.
- If both strings are the same, Then the input string( inputStr) is a palindrome string.
- If both strings are not equal, Then the input string ( inputStr) is not a palindrome.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 |
/*     program to check a string is a Palindrome     sillycodes.com */  #include<stdio.h> #include<string.h>  // Max Size of string const int SIZE = 100;  /** * @brief - Reverse the given string * * @param inputStr - input string * @return char*  - Returns the reversed string */  char * stringReverse(char * inputStr) {     // maintain two indices, one(i) at start, second(j) at end     int i = 0;     int j = strlen(inputStr)-1;     int temp;         // Iterate over the string     // swap the characters at indices i, j and increment 'i' and decrement 'j's     for( ; i < j; i++, j--)     {         // swap characters         temp = inputStr[i];         inputStr[i] = inputStr[j];         inputStr[j] = temp;     }      return inputStr; }  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     int i, j, temp;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);       // Make a backup of the original string.     char revStr[SIZE];     strcpy(revStr, inputStr);      // Reverse the string     // call 'stringReverse' function with 'revStr'     stringReverse(revStr);           // Compare the 'inputStr' and 'revStr'     if(strcmp(inputStr, revStr) == 0)     {         // 'inputStr' and 'revStr' are same         // so string is palindrome         printf("String '%s' is a Palindrome \n", inputStr);     }     else     {         // String is not a Palindrome         printf("String '%s' is not a Palindrome \n", inputStr);     }      return 0; } |
📢 It is recommended to use the first method, As we are using the extra space in the second method.
Program Output:
Compile and run the program using GCC (Any compiler)
1 2 3 4 5 6 7 8 |
$ gcc palindrome-string.c $ ./a.out Enter a string : rotator String 'rotator' is a Palindrome $ ./a.out Enter a string : filter String 'filter' is not a Palindrome $ |
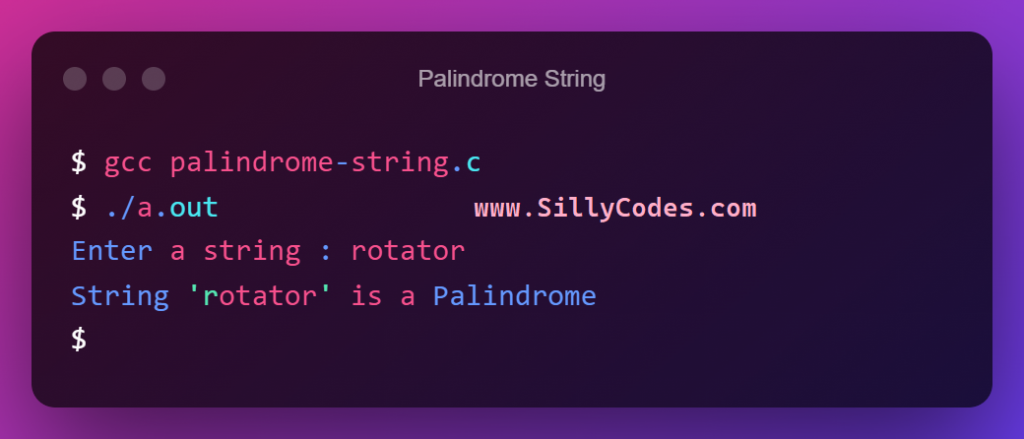
As we can see from the above output, The program is providing the desired results.
Related String Programs:
- C Tutorials Index
- C Programs Index
- C Program to Calculate Length of the string
- C Program to Copy a String to new string
- C Program to Concatenate Two strings
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
1 Response
[…] C Program to Check Palindrome String […]