Reverse Array in C Langauge | Reverse Array Elements without using extra memory
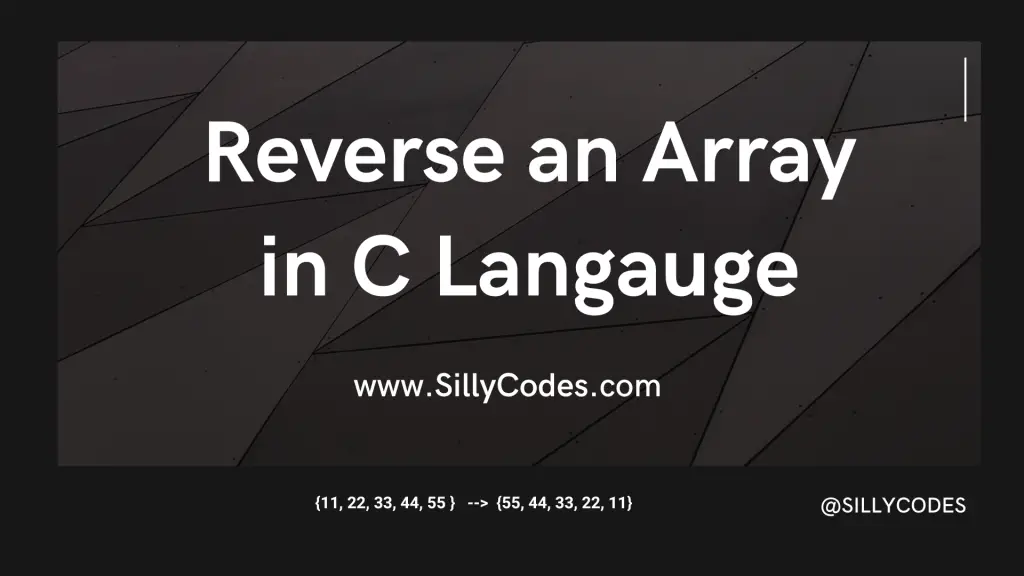
- Reverse Array in C without using extra memory Program Explanation:
- Algorithm to Reverse Array in C (without extra memory):
- Program to Reverse Array in C language:
- Method 2: Reverse Array elements in C using a function (reverseArray):
- Method 3: Reverse the Array by creating a copy of the original Array (Using Extra space):
Program Description:
Write a Program to Reverse Array in C programming language. The program should accept an input array from the user and reverse the array elements.
We are going to look at a few methods in this article.
- Reverse an Array without using extra memory
- Using reverseArray function to reverse the array elements
- Copying Original Array to new Array using Extra Memory (Not Recommended).
Here are the expected input and output of the program.
Example Input and Output:
Input:
1 2 |
Enter desired array size(1-100): 5 Please enter array elements(5) : 82 37 23 74 99 |
Output:
1 2 |
Original Array: 82 37 23 74 99 Reversed Array: 99 74 23 37 82 |
Prerequisites:
We need to know the basics of the Arrays, and Functions in C to better understand the following program.
- Arrays in C Language with Example Programs
- Functions in C Langauge – How to create, call functions
- C Arrays with Functions – How to Pass arrays to functions
Reverse Array in C without using extra memory Program Explanation:
- Create an array named numbers with size of 100. And few other supporting variables like i, size, start, end, and temp variables
- Take the desired array size from the user and store it in size variable.
- We need to take the input values for the array. So prompt the user to provide the array elements.
- Create a for loop and start with the element zero(index) to size-1 index.
- Prompt the user for input and read it using scanf function and update the numbers[i] element.
- Once we got the array, We can display it as the Original Array. ( We need to use another for loop to display the array elements)
- To Reverse the array, update the variables start with zero(0) and end with size-1. So the start variable is pointing to the first element in the array and the end element is pointing to the last element in the array.
- The fundamental concept is to swap the first element for the final element, the second element for the second-to-last element, and so forth. In this manner, the array’s elements will be reversed when the loop is completed.
- So start the loop
start=0 and
end=size-1. At each iteration,
- Swap the numbers[start] with numbers[end].
- Here we are using a temp variable to do the swapping, But we can also use Swapping without using the temporary variable method. for simplicity, we are using the temporary variable to swap the elements.
- Then increment the start variable (i.e start++) and decrement the end variable (i.e end--)
- Once the above loop is finished, The array elements will be Reversed.
- Display the reversed array on the console.
Algorithm to Reverse Array in C (without extra memory):
Here is the algorithm for reversing an array in the C language without using any extra space.
- Start the program
- Declare numbers[100], i, size, start, end, and temp variables.
- Take the size from the user
- Prompt the user and Update the
numbers array
- FOR i=0 to i=size-1
- Update the &numbers[i] element
- Display the original array.
- FOR i=0 to i=size-1
- display the numbers[i] element
- Reverse the Array
- FOR start=0; end=size-1 till (start < end)
- Swap the numbers[start] and numbers[end]
- Update start++ and end-- at each iteration
- Display the Reversed Array
- FOR i=0 to i=size-1
- display the numbers[i] element
- Stop the program
Program to Reverse Array in C language:
Let’s convert the above algorithm into the C program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
/*     Program to Reverse Array in c without using extra space     sillycodes.com */ #include <stdio.h>  int main() {     // declare the 'numbers' array     int numbers[100];     int i, size, start, end, temp;      printf("Enter desired array size(1-100): ");     scanf("%d", &size);      // User input for array elements     printf("Please enter array elements(%d) : ", size);     for(i = 0; i < size; i++)     {         // printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     }      printf("Original Array: ");     for(i = 0; i < size; i++)     {         printf("%d ", numbers[i]);        }      // update 'start' and 'end'     start=0, end=size-1;      // Reverse array by swaping elements in-place     for(; start < end; start++, end--)     {         // swap the 'start' and 'end' index elements         temp = numbers[start];         numbers[start] = numbers[end];         numbers[end] = temp;     }      printf("\nReversed Array: ");     for(i = 0; i < size; i++)     {         printf("%d ", numbers[i]);        }     printf("\n");      return 0; } |
Reverse Array Program Output:
Compile and run the program using your favorite compiler.
Compile the program
gcc reverse-array.c
The above command generates an executable file ( a.out in Linux). Run the executable file.
Test 1:
1 2 3 4 5 6 |
$ ./a.out Enter desired array size(1-100): 10 Please enter array elements(10) : 9 8 7 6 5 4 3 2 1 0 Original Array: 9 8 7 6 5 4 3 2 1 0 Reversed Array: 0 1 2 3 4 5 6 7 8 9 $ |
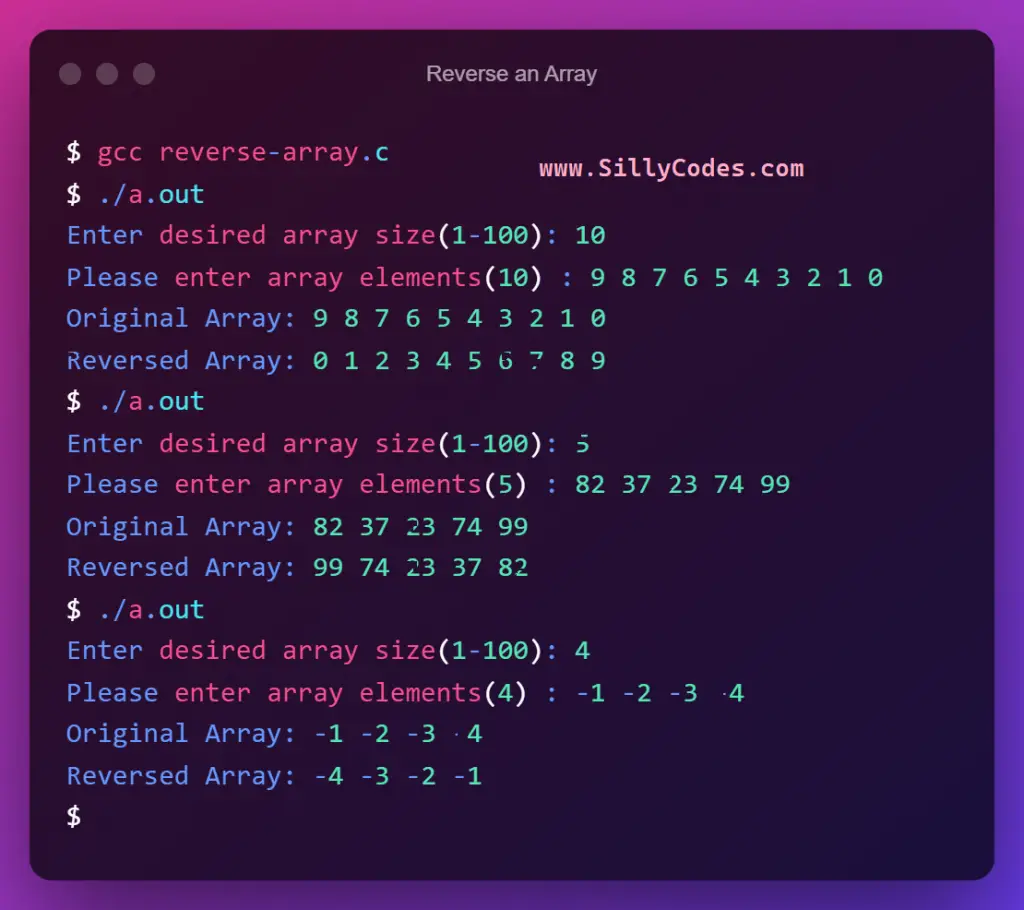
Let’s try a few more examples.
Test 2:
1 2 3 4 5 6 7 8 9 10 11 |
$ ./a.out Enter desired array size(1-100): 5 Please enter array elements(5) : 82 37 23 74 99 Original Array: 82 37 23 74 99 Reversed Array: 99 74 23 37 82 $ ./a.out Enter desired array size(1-100): 4 Please enter array elements(4) : -1 -2 -3 -4 Original Array: -1 -2 -3 -4 Reversed Array: -4 -3 -2 -1 $ |
Method 2: Reverse Array elements in C using a function (reverseArray):
Let’s convert the above program into small functions, where each function does a specific task. In the above program, We used only one function which is main() to perform all the tasks.
Here is the program with three user-defined functions(except main()) which are read, display, and reverseArray. Each function does a specific task.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
/*     Program to Reverse an Array using function     sillycodes.com */ #include <stdio.h>  /** * @brief  - Read the user input and update the 'numbers' array * * @param numbers - 'numbers' array * @param size    - size of the 'numbers' array */ void read(int numbers[], int size) {     int i;     // User input for array elements     printf("Please enter array elements(%d) : ", size);     for(i = 0; i < size; i++)     {         // printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     } }  /** * @brief  - Display the 'numbers' array * * @param numbers - Array * @param size    - Array size */ void display(int numbers[], int size) {     int i;     for(i = 0; i < size; i++)     {         printf("%d ", numbers[i]);        }     printf("\n"); }  /** * @brief  - Reverse the given 'numbers' array in-place by swapping elements * * @param numbers - 'numbers' array (to be swapped) * @param size    - size of the 'numbers' array */  void reverseArray(int numbers[], int size) {     int temp;     // update 'start' and 'end'     int start=0, end=size-1;      // Reverse array by swaping elements in-place     for(; start < end; start++, end--)     {         // swap the 'start' and 'end' index elements         temp = numbers[start];         numbers[start] = numbers[end];         numbers[end] = temp;     } }  int main() {     // declare the 'numbers' array     int numbers[100];     int i, size, start, end, temp;      printf("Enter desired array size(1-100): ");     scanf("%d", &size);      // Take user input and update array     read(numbers, size);      // print the original array     printf("Original Array: ");     display(numbers, size);      //Reverse Array elements by using 'reverseArray' function     reverseArray(numbers, size);      // print the reversed array     printf("Reversed Array: ");     display(numbers, size);      return 0; } |
In the above program, We have defined four functions including the main() function.
- The
read() function –
void read(int numbers[], int size)
- This function takes two formal arguments. The numbers array and the size
- The read() is used to take the user input and update the array elements.
- The
display() function –
void display(int numbers[], int size)
- This function also takes two formal arguments, which are numbers[] array and its size
- The display() function is used to display the array on the standard output.
- The
reverseArray() function –
void reverseArray(int numbers[], int size)
- This is the function, which actually reverse the array. The reverseArray() function takes two formal arguments, they are numbers array and size and it doesn’t return anything( void)]
- The reverseArray modifies the input array numbers and reverses the elements in place by swapping the array elements.
- As the numbers array is passed by the address, so all the changes made in the reverseArray will be reflected in the main() function’s numbers array.
Program Output:
Let’s compile and run the program and observe the output.
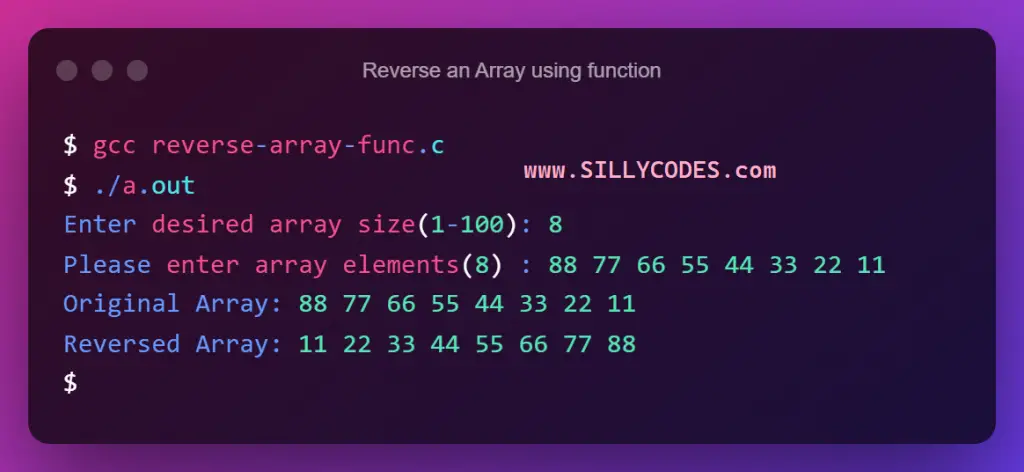
1 2 3 4 5 6 7 8 9 10 11 12 |
$ gcc reverse-array-func.c $ ./a.out Enter desired array size(1-100): 8 Please enter array elements(8) : 88 77 66 55 44 33 22 11 Original Array: 88 77 66 55 44 33 22 11 Reversed Array: 11 22 33 44 55 66 77 88 $ ./a.out Enter desired array size(1-100): 5 Please enter array elements(5) : 0 9 2 4 -4 Original Array: 0 9 2 4 -4 Reversed Array: -4 4 2 9 0 $ |
Method 3: Reverse the Array by creating a copy of the original Array (Using Extra space):
We can reverse the array by copying all the elements of the array in the reverse order to a new array. So here is the program to copy the elements to new array in reverse order.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
/*     Program to Reverse Array bycopying elements of the original array     sillycodes.com */ #include <stdio.h>  /** * @brief  - Read the user input and update the 'numbers' array * * @param numbers - 'numbers' array * @param size    - size of the 'numbers' array */ void read(int *numbers, int size) {     int i;     // User input for array elements     printf("Please enter array elements(%d) : ", size);     for(i = 0; i < size; i++)     {         // printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     } }  /** * @brief  - Display the 'numbers' array * * @param numbers - Array * @param size    - Array size */ void display(int *numbers, int size) {     int i;     for(i = 0; i < size; i++)     {         printf("%d ", numbers[i]);        }     printf("\n"); }  int main() {     // declare the 'numbers' array     int numbers[100], revArr[100] = {0};     int i, j, size, start, end, temp;      printf("Enter desired array size(1-100): ");     scanf("%d", &size);      // Take user input and update array     read(numbers, size);      // print the original array     printf("Original Array: ");     display(numbers, size);         // copy the array elements in reverse order     for(i=size-1, j=0; (i>=0 || j<size); i--, j++)     {         revArr[i]=numbers[j];     }      printf("Reversed Array: ");     display(revArr, size);      return 0; } |
Here we have created a new array revArr with the same size of the numbers array (i.e 100).
And copied the numbers array in reverse order to the revArr.
1 2 3 4 5 |
// copy the array elements in reverse order for(i=size-1, j=0; (i>=0 || j<size); i--, j++) { Â Â Â Â revArr[i]=numbers[j]; } |
We need to use the two control variables i and j. Where i goes from size-1 to zero(0) and j goes from zero(0) to size-1. At each iteration we simply copy the numbers[j] to the revArr[i] element.
Once the above loop is completed, The revArr array contains the Reversed Array.
Program Output:
Compile and Run the program
1 2 3 4 5 6 7 8 9 10 11 12 |
$ gcc reverse-array-method-2.c $ ./a.out Enter desired array size(1-100): 10 Please enter array elements(10) : 10 20 30 40 50 60 70 80 90 99 Original Array: 10 20 30 40 50 60 70 80 90 99 Reversed Array: 99 90 80 70 60 50 40 30 20 10 $ ./a.out Enter desired array size(1-100): 5 Please enter array elements(5) : 56 37 82 91 23 Original Array: 56 37 82 91 23 Reversed Array: 23 91 82 37 56 $ |
Related Array Programs:
- Index – C Language Practice Programs
- C Tutorials Index – Step by step tutorials to master C Langauge
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Find Maximum Element in Array
2 Responses
[…] Array using Recursion in C programming language. In an earlier tutorial, We have looked at the Reversing array elements using the Iterative method. In today’s article, we will use Recursion to reverse the array […]
[…] C Program to Reverse the Array Elements […]