Pattern 8: Diamond or Rhombus pattern program in C
Introduction:
We are going to discuss about the Rhombus Pattern program in this article, Sometimes we also call this as Diamond pattern. We are going to use C for loops to create the pattern.
Write a C Program to print below pattern ( Rhombus Pattern Program) :
Here is the Expected Output, When user specified the input as 5.
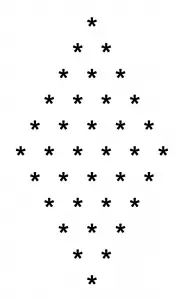
Rhombus (Diamond) Pattern Explanation :
This is one the series of pattern programs in C. This program is very similar to my previous pattern program “Pyramid Star pattern program in C Language“.
Here I am using only two for loops to create above pattern. First for loop is responsible for the number of rows. so it always starts with -n. The inner loop is for columns. I am using small logic(using variable ‘k’) to accomplish this pattern with only two loops.
Rhombus Pattern Program:
-
#include<stdio.h>
-
int main()
-
{
-
int i,j,k,n;
-
printf(“Enter the Value for n : “);
-
scanf(“%d”,&n);
-
-
for(i=-n; i<=n; i++) // Note that ‘i’ is starting from -n (negative n)
-
{
-
k=i;
-
if(k<0)
-
k= k*-1;
-
-
for(j=0;j<=n;j++)
-
{
-
if(k<=j)
-
printf(“* “);
-
else
-
printf(” “);
-
}
-
printf(“\n“);
-
}
-
return 0;
-
}
Output:
Similar Star pattern programs:
- Basic star pattern program (The square pattern with stars).
- Triangle star pattern program.
- Triangle star pattern program with Spaces.
- Pyramid star pattern.
- Reverse Pyramid program.
More C programs:
- Program to calculate Maximum of three numbers.
- What are Prime Number and C program to Check given number is Prime or Not
- Check given Number is Prime or not Using Square Root(sqrt) Function.(Efficient way)
- C Program to generate prime numbers between two numbers
- C Program to generate first N prime numbers.
- C program to Calculate percentage of student.
- C Program to check given year is the leap or not.
- C Program to convert Temperature.
- C program to understand type conversation.
- Finding Largest of two numbers using the conditional operator in C.
- C program to calculate the simple Interest,
- C program to understand Size of Operator.
- 200+ C Programs.
C Tutorials with simple Examples:
- Arithmetic Operators with Examples.
- Arithmetic operators priority and it’s Associativity.
- Modulus Operator and Hidden Concepts of Modulus Operator.
- Precedence Table or Operators Priority Table.
- Assignment Operator, Usage, Examples
- Increment Operator and Different types of Increment operators Usage with Examples.
- Decrement Operator and Different types of Decrement operators with Examples.
- Logical or Boolean Operators.
1 Response
[…] Pattern 8: Rhombus Star Pattern Program […]