Find All Occurrences of substring in string in C Program
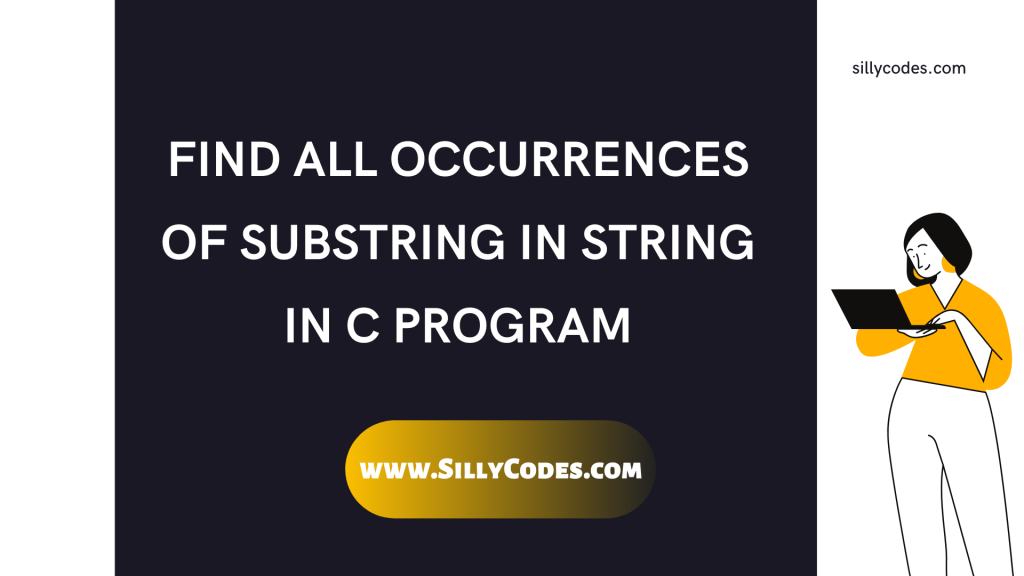
Program Description:
Write a Program to Find All Occurrences of substring in string in C Programming language. The program should accept two strings from the user, one for the main string and one for the substring. The program needs to find all occurrences of the substring within the main string.
We are going to look at a couple of methods to find all occurrences of substring and each program will be followed by a detailed step-by-step explanation of the program and program output. We also included the instructions to compile and run the program.
📢 This Program is part of the C String Practice Programs series
Input and Output:
Input:
Enter a main string : music is bliss and music is soothing
Enter the substring(String to search in main string): music
Output:
FOUND! The Substring:'music' is found at 0 index
FOUND! The Substring:'music' is found at 19 index
If you observe the above output, The substring music is found in two places in the main string.
📢 The program is case-sensitive. As a result, upper-case characters will differ from lower-case characters.
Prerequisites of the program:
It is recommended to go through the following articles to better understand the program.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Functions in C – How to Create and Use functions in C
We will look at two methods to find all occurrences of the substring in the main string. They are
- Manual Approach(Iterative) – Where we use an iterative method to find the sub-string in the main string
- User-defined function – We use a custom user-defined to find all occurrences of the target string in the main string.
Find All Occurrences of substring in string in C Program Explanation:
Let’s look at the instructions to find all occurrences of a string in a string.
- Create a Macro called SIZE with the value 100. The SIZE holds the max size of the strings. please change this if you want to use large strings.
- Start the main() function by declaring the two strings and naming them as mainStr and subStr. The size of both strings is equal to SIZE macro.
- Initialize a variable called isFound with the value -1. This variable is used verify if we found the sub string( subStr) in the main string( mainStr).
- Take two strings from the user and store them in mainStr and subStr respectively. We use the gets function to read the strings.
- To find all occurrences of substring( subStr) in the main string( mainStr), Iterate through the main string( mainStr), looking for the first character of the substring( subStr[0]). If we found the first character of the substring, we should then determine whether all other characters of the substring match. If that’s the case, we found the substring( subStr) in the main string( mainStr). Similar way we need to look for all occurrences of the substring.
- Create a For loop by initializing variable i with 0 to iterate over the
mainStr. It will continue looping as long as the current character (
mainStr[i]) is not a
NULL character (
'\0').
- At each iteration, Check if the current character( mainStr[i]) is equal to the first character of the substring( subStr[0]) - i.e if(mainStr[i] == subStr[0])
- If the above condition is
true, Then search for all characters of the
subStr in the
mainStr. We need to use another for loop to iterate over the contents of the
subStr – i.e
for(j=0; subStr[j]; j++).
- Check if the mainStr[k] (where k = i) is not matching to subStr[j], If so then the subString is not found. Otherwise, continue to check all elements of the subStr.
- Once the above loop is completed, Check if we iterated complete subStr ( use j == strlen(substr) ). If we iterated the complete substring( subStr), then we found the match and print the index on the screen. So update the isFoundvariable with the current index( i). – i.e lastStrIdx = i;
- Continue to the next iteration to search for remaining occurrences of the substring ( subStr).
- If step 5 is completed, Check the value of the
lastStrIdx variable.
- If it is equal to the -1, Then we haven’t found the substring( str) in the main string( mainStr). Display the NOT FOUND message on the console.
- Stop the program.
Program to Find All Occurrences of substring in string in C – Iterative Method:
Here is the program to find all occurrences of substring in the main string in c programming language using loops.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
/*     program to Search for a all occurrences substring in a string in C     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string #define SIZE 100  int main() {      // declare two strings with 'SIZE'     char mainStr[SIZE], subStr[SIZE];     int i, j, k;     int isFound = -1;      // Take the main string from the user     printf("Enter a main string : ");     gets(mainStr);      // take the sub string     printf("Enter the substring(String to search in main string): ");     gets(subStr);      // Iterate over the main string     for(i = 0; mainStr[i]; i++)     {         // search for the first character in substring         if(mainStr[i] == subStr[0])         {             // The first character of substring is matched.             // Check if all characters of substring matching.             k = i;             for(j=0; subStr[j]; j++)             {                 // printf("mainStr[%d]=%c, subStr[%d]=%c \n", i, mainStr[i],j, subStr[j]);                 if(mainStr[k] != subStr[j])                 {                     // Not matched                     break;                 }                 k++;             }              // check if the 'j' is equal to length of the 'subString', Then we found the match             if(j == strlen(subStr))             {                 printf("FOUND! The Substring:'%s' is found at %d index\n", subStr, i);                 isFound = i;             }          }     }      // check the 'isFound'     if(isFound == -1)     {         // 'subStr' is not found         printf("NOT FOUND! Substring:'%s' is NOT found in main string\n", subStr);     }         return 0; } |
As we are using the strlen() library function, We need to include the string.h header file.
Program Output:
Compile the program using the following command.
$ gcc search-str-all.c
Run the executable file. ( i.e a.out is our executable file name)
Example 1:
1 2 3 4 5 6 |
$ ./a.out Enter a main string : music is bliss and music is soothing Enter the substring(String to search in main string): music FOUND! The Substring:'music' is found at 0 index FOUND! The Substring:'music' is found at 19 index $ |
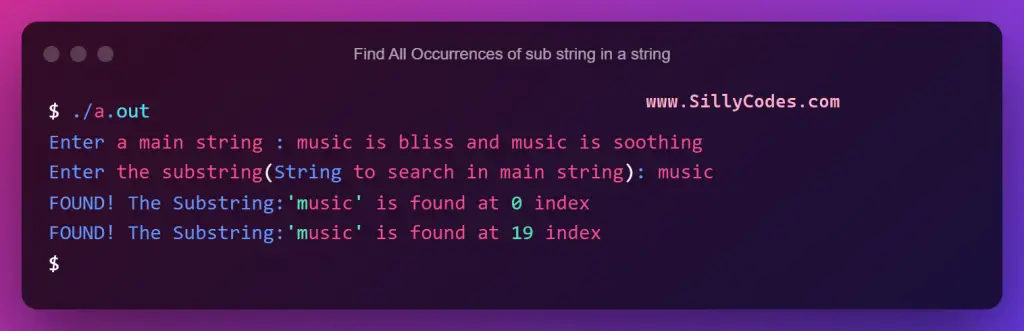
In the above example, The main string is music is bliss and music is soothing and the substring is music. The program is able to find all occurrences of the music in the main string and displayed the indices of the substring.
Example 2:
1 2 3 4 5 |
$ ./a.out Enter a main string : Happy and Cheerful Enter the substring(String to search in main string): sad NOT FOUND! Substring:'sad' is NOT found in main string $ |
In this example, The substring sad is not present in the main string Happy and Cheerful.
Find All Occurrences of substring in string using a user-defined function in C:
In the above method, We have written the complete program inside the main() function. Let’s rewrite the above program to use a function to find all occurrences of a sub string in a string.
By using the functions we can define a function once and can call it as many times as we want. Functions also increase the readability of the program and make it easier to debug issues. Here are the benefits using the functions in programming.
In the following program, We have defined a function called printAllSubStr() to find and print indices of all occurrences of the given substring in the main string.
The prototype of the printAllSubStr() function is – int printAllSubStr(char *mainStr, char * subStr)
As we can see, It takes two formal arguments, They are mainStr and subStr. Both these arguments are character pointers pointing to the main string and substring respectively.
This function searches for the subStr in the mainStr and prints the indices of the subStr. The return value of the function is Integer ( int). It returns > 0 if the subStr is found in the mainStr, otherwise, it returns -1.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 |
/*     program to Search for a all occurrences substring in a string in C     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string #define SIZE 100  /** * @brief  - Print all occurrences of the 'subStr' in the 'mainStr' * * @param mainStr - Main String * @param subStr  - Sub String - String to be searched in the main string * @return int    - Return > 0 if the 'subStr' is found in 'mainStr' Otherwise returns -1. */ int printAllSubStr(char *mainStr, char * subStr) {     int i, j, k;     int isFound = -1;      // Iterate over the main string     for(i = 0; mainStr[i]; i++)     {         // search for the first character in substring         if(mainStr[i] == subStr[0])         {             // The first character of substring is matched.             // Check if all characters of substring matching.             k = i;             for(j=0; subStr[j]; j++)             {                 // printf("mainStr[%d]=%c, subStr[%d]=%c \n", i, mainStr[i],j, subStr[j]);                 if(mainStr[k] != subStr[j])                 {                     // Not matched                     break;                 }                 k++;             }              // check if the 'j' is equal to length of the 'subString', Then we found the match             if(j == strlen(subStr))             {                 printf("FOUND! The Substring:'%s' is found at %d index\n", subStr, i);                 isFound = i;             }          }     }      // return isFound     return isFound;  }  int main() {      // declare two strings with 'SIZE'     char mainStr[SIZE], subStr[SIZE];      // Take the main string from the user     printf("Enter a main string : ");     gets(mainStr);      // take the sub string     printf("Enter the substring(String to search in main string): ");     gets(subStr);      // Call the 'printAllSubStr' and pass the mainStr, subStr     int isFound = printAllSubStr(mainStr, subStr);      // check the 'isFound'     if(isFound == -1)     {         // 'subStr' is not found         printf("NOT FOUND! Substring:'%s' is NOT found in main string\n", subStr);     }         return 0; } |
Call the printAllSubStr() function from the main() function with mainStr and subStr as arguments.
1 2 |
// Call the 'printAllSubStr' and pass the mainStr, subStr int isFound = printAllSubStr(mainStr, subStr); |
The isFound variable contains the return value of the function.
Program Output:
Let’s compile and run the program.
Example 1:
1 2 3 4 5 6 7 |
$ gcc search-str-all-func.c $ ./a.out Enter a main string : Live today like there is no other day Enter the substring(String to search in main string): day FOUND! The Substring:'day' is found at 7 index FOUND! The Substring:'day' is found at 34 index $ |
The substring day found two times in the main string at the index 7 and 34.
Example 2:
1 2 3 4 5 |
$ ./a.out Enter a main string : Learn Programming Enter the substring(String to search in main string): language NOT FOUND! Substring:'language' is NOT found in main string $ |
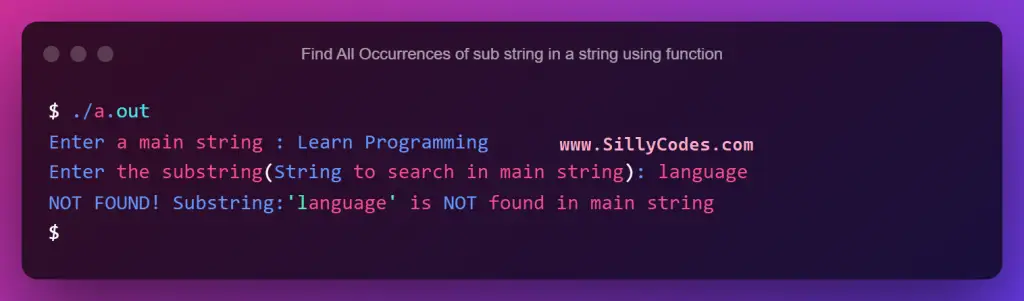
The string language is not found in the main string Learn Programming.
As we can see from the above outputs, The program is properly finding the all occurrences of the substring in the main string.
More String Programs:
- C Tutorials Index
- C Programs Index
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence