strcat in C language – String Library Functions
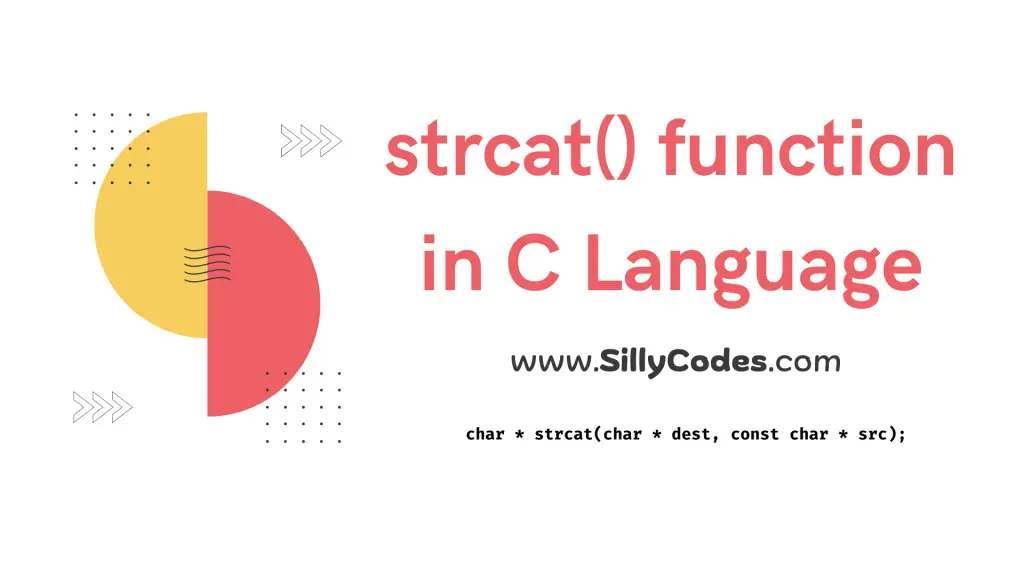
Introduction:
In earlier articles, We looked at the strlen function and strcpy functions. In today’s article, We will look at the strcat in C Programming language with example programs.
📢 This Program is part of the C String Practice Programs series
Prerequisites:
It is recommended to go through the following string tutorials to better understand this article.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
strcat in C Programming Language:
The strcat function is used to concatenate two strings. The strcat() function appends the source string at the end of the destination string. Let’s look at the strcat function syntax.
Syntax of strcat function in C:
1 |
char * strcat(char * dest, const char * src); |
Where
dest – Destination String
src – Source String
As we can see from the above syntax of the strcat function, The strcat function appends the source string ( src) at the end of the Destination string ( dest).
The strcat() function overwrites the NULL Character( \0) at the end of the destination string ( dst), Appends the src string and finally adds the terminating NULL Character( \0) at end of the appended string (final string).
The destination string( dest) size must be large enough to accommodate the concatenated string size, Otherwise the strcat() function behavior is undefined.
Function Parameters:
The strcat() function takes two strings as the parameters. The first argument is the Destination string (i.e dst) and the second argument is Source string (i.e src).
Function Return Type:
The strcat() function returns the destination string back to the caller.
📢 The resulting string of the strcat() function is always terminated with the NULL Character.
Program to concatenate two strings using strcat function in C:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
/* program to understand the strcat function in c sillycodes.com */ #include<stdio.h> #include<string.h> // for 'strcat' function const int MAXSIZE = 100; int main() { // declare two string with 'MAXSIZE' char src[MAXSIZE], dest[MAXSIZE]; // get the two strings from the user printf("Enter source string : "); // scanf("%s", src); gets(src); printf("Enter the destination string: "); gets(dest); // concatenate two strings 'src' to 'dest' strcat(dest, src); // print the results. printf("String after concatenation : %s \n", dest); return 0; } |
⚠️ Don’t forget to include the string.h header file.
Program Explanation:
In the above program, We declared two strings called src and dest with the size of MAXSIZE. The MAXSIZE is a global constant and it’s value is 100. So our strings at max can store 100 characters (including the terminating NULL Character)
We prompted the user to provide the two strings and stored the given strings in the src and dest strings respectively. We used the gets function to read the strings ( The gets function won’t do the boundary checks, so be cautious while using it)
Then we used the strcat() function to append the source string src to the destination string dest
strcat(dest, src);
Finally, We printed the resultant string on the console.
Program Output:
Let’s compile and run the program.
Test1:
1 2 3 4 5 |
$ ./a.out Enter source string : Sillycodes.com Enter the destination string: Welcome to String after concatenation : Welcome to Sillycodes.com $ |
In the above example, The user provided the source string as the Sillycodes.com and the destination string as the Welcome to (added extra space at after to). The strcat function concatenated two strings and returned the resulting result as Welcome to Sillycodes.com
Example 2:
1 2 3 4 5 |
$ ./a.out Enter source string : World Enter the destination string: Hello String after concatenation : Hello World $ |
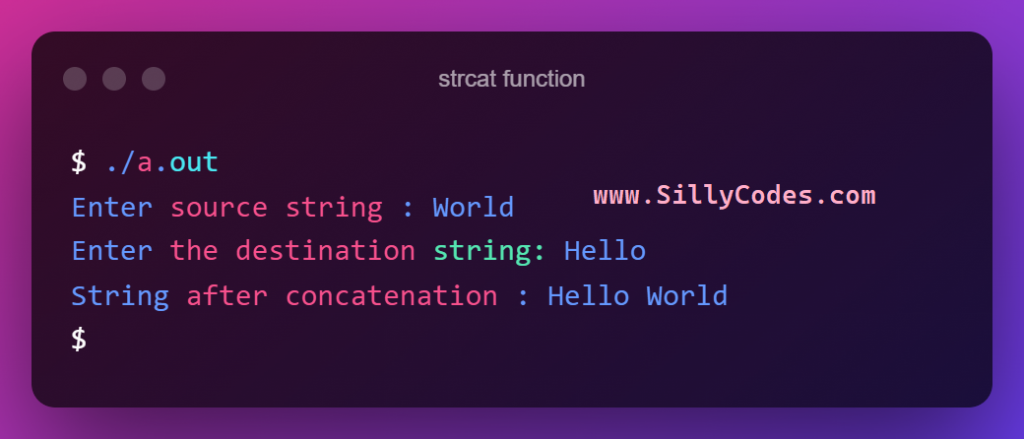
Similarly, The strcat function appended two strings World and Hellocreated the Hello World string.
One final thing to note is the strcat function also returns the resultant string to the caller. So we can use the nested strcat function and directly use it in the printf functions, etc.
strcat(str1, str(str2, str3));
and
printf("Resultant string is : %s\n", strcat(dest, src));
1 Response
[…] strcat library function in C […]