Program to Average of array elements in C Language
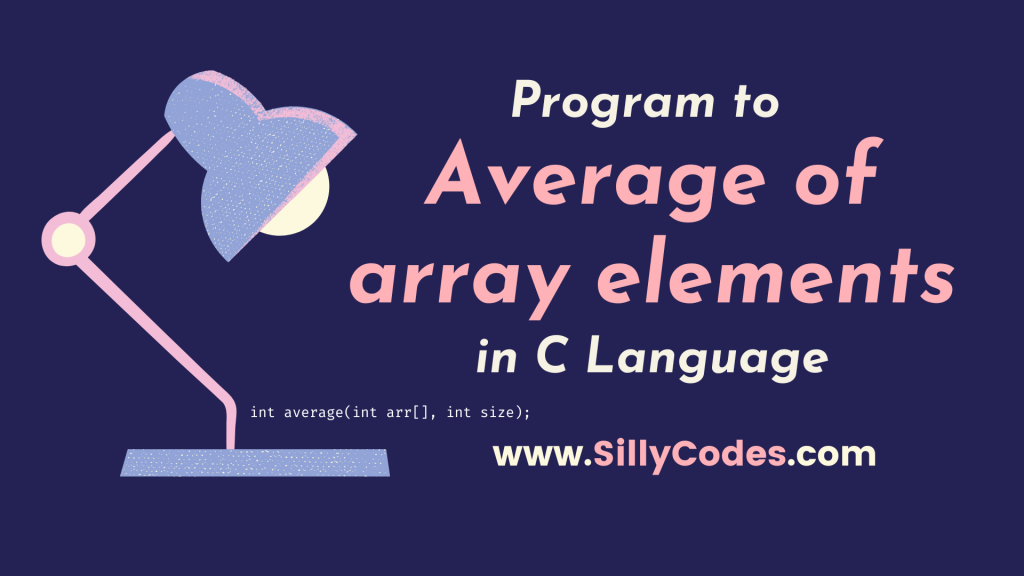
Program Description:
Write a program to calculate the Average of array elements in C programming language. The program should accept user input and update array elements and calculate the average of the array.
Example Input and Output:
Input:
1 2 3 4 5 6 |
Please enter the array elements arr[0] : 54 arr[1] : 21 arr[2] : 34 arr[3] : 54 arr[4] : 64 |
Output:
Average of all Array elements is : 45
Prerequisites:
We are going to use the arrays and functions in this program. Please go through the following articles to learn more about C Arrays and Functions.
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- 2D arrays in C with Example programs
- How to pass arrays to functions in C
- Functions in C language
Average of array elements in C Program Explanation:
- Create a Macro SIZE with value 5. #define SIZE 5
- In the main() function, Declare an array arr with the SIZE. Also, create variables i, sum, and avg. Initialize sum and avg with zero.
- Take the user input to update the array. To do that, Use a for loop to iterate over the array ( from
i = zero(0) to
SIZE-1 or i<SIZE ).
- At each iteration, Prompt the user for input and read the value using scanf function and store it in arr[i]
- The average of the array is equal to the Sum of all array elements divides by the number of elements in the array. ( avg = sum_of_elements / num_of_elements)
- First, calculate the sum of the array elements. To do that, Use a for loop to traverse the array at each iteration and add the sum to arr[i]. – sum += arr[i];
- Once we got the sum of all elements of the array( sum), Divide the sum with SIZE of the array to get the average of all array elements – avg = sum / SIZE
- Print the result on the standard output i.e console.
Algorithm of Average of array elements in C:
- Declare an integer array arr of size 5 and an integer variable i. Initialize the sum and avg with zero(0)
- Prompt the user to input the values for the arr
- FORÂ
i = 0 toÂ
SIZE-1Â ( Loop )
- Read the value for  arr[i]
- FORÂ
i = 0Â toÂ
SIZE-1Â ( Loop )
- sum = sum + arr[i];
- Calculate Average
- avg = sum / SIZE;
- Print the result on the console
Average of array elements in C Program:
Let’s convert the above algorithm into the C code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
/*     Program to calculate the average of array elements     Author: SillyCodes.com */ #include <stdio.h> #define SIZE 5  int main() {     int i, sum=0, avg=0;     // declare an array named 'arr'     int arr[SIZE];      printf("Please enter the array elements \n");     // Read the input from the user     for(i=0; i<SIZE; i++)     {         printf("arr[%d] : ", i);         scanf("%d", &arr[i]);     }      // calculate the 'sum' of elements     for(i = 0; i<SIZE; i++)     {         sum += arr[i];     }         // calculate average     avg = sum / SIZE;      // print the results     printf("Average of all Array elements is : %d \n", avg);      return 0; } |
Program Output:
Compile the program. We are using the GCC compiler in Linux OS.
gcc avg-arr-elements.c
The above command generates the executable file named a.out. Run the executable.
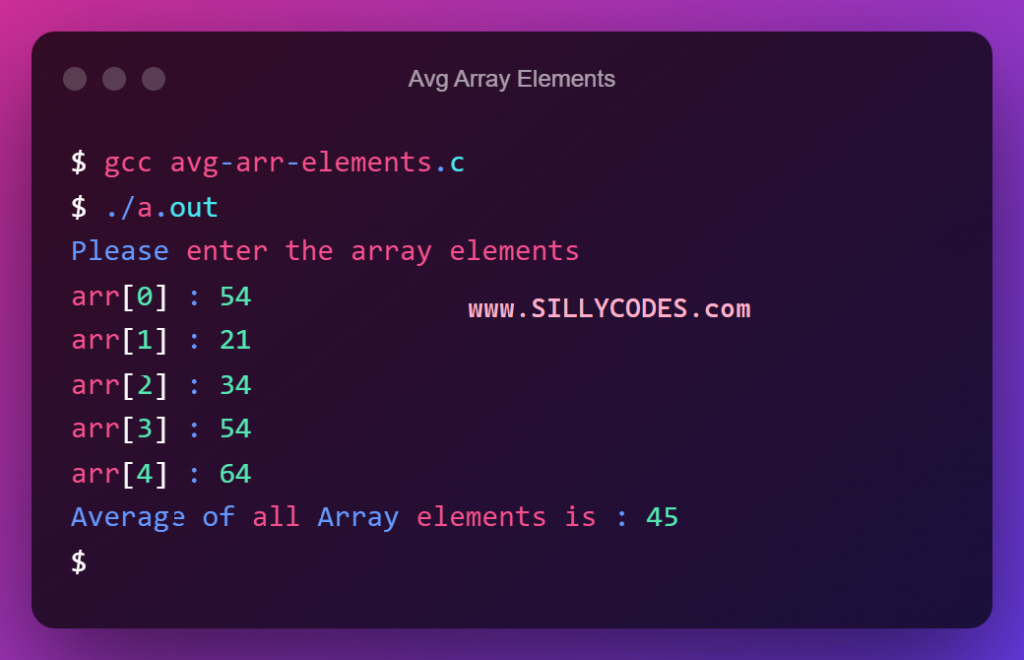
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$ ./a.out Please enter the array elements arr[0] : 54 arr[1] : 21 arr[2] : 34 arr[3] : 54 arr[4] : 64 Average of all Array elements is : 45 $ ./a.out Please enter the array elements arr[0] : 4 arr[1] : 2 arr[2] : 7 arr[3] : 8 arr[4] : 3 Average of all Array elements is : 4 $ |
As we can see from the above program, We are getting the expected results.
Program to calculate the Average of array elements using the function in C:
Let’s rewrite the above program to use the functions for Reading the numbers from user and Calculating the Average of elements.
The following program uses the read function to take user input and the average function to calculate the average of the array elements.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 |
/*     Program to calculate the average of array elements using functions     Author: SillyCodes.com */ #include <stdio.h> #define SIZE 5  /** * @brief  - Read the elements from INPUT * * @param arr- 'arr' Array * @param size - size of the array */ void read(int *arr, int size) {     int i;     printf("Please provide the Array elements\n");     // Read the input from the user     for(i=0; i<size; i++)     {         printf("arr[%d] : ", i);         scanf("%d", &arr[i]);     } }  /** * @brief  - Calculate the average of all elements in given array * * @param arr  - 'arr' array * @param size  - Size of 'arr' array * @return int  - Return Average of all elements */ int average(int arr[], int size) {     int i, sum = 0;     // calculate the 'sum' of elements     for(i = 0; i<size; i++)     {         sum += arr[i];     }         // calculate average and return it     return (sum / size); }  int main() {     int i, avg = 0;      // declare an array named 'arr'     int arr[SIZE];      // Read array from user     read(arr, SIZE);      // call the 'average' function     avg = average(arr, SIZE);      // print the results     printf("Average of all Array elements is : %d \n", avg);      return 0; } |
📢Note, As we already have the SIZE macro, We can use the SIZE macro directly instead of passing the size as a parameter to both functions.
Average of array elements using the function in C Program Explanation:
- Create arrarray with the size 5 and also create a avgvariable and initialize with zero(0).
- Define the
read() function to take the input numbers from the user. The read function takesÂ
*arr andÂ
size as formal arguments
- The read function uses a for loop to prompt the user for input and update the array elements.
- Create another functionÂ
average to calculate the average of all array elements.
- The averagefunctions take integer array arr[] and array size as the formal arguments and return an integer.
- This function iterates over the arr and at each iteration adds the sum and arr[i] – sum = sum + arr[i];
- Then calculate the average by dividing the sum by size. Return it. ( return sum / size;)
- In the main() function, Call the read() function to take user input.
- Call the average function to calculate the average of array elements. This function returns the average of elements. store it in avg variable.
- Print the avg variable on the console.
Program Output:
Let’s compile and run the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
$ gcc avg-arr-elements-func.c $ ./a.out Please provide the Array elements arr[0] : 6 arr[1] : 8 arr[2] : 3 arr[3] : 7 arr[4] : 1 Average of all Array elements is : 5 $ $ ./a.out Please provide the Array elements arr[0] : 34 arr[1] : 23 arr[2] : 87 arr[3] : 98 arr[4] : 23 Average of all Array elements is : 53 $ |
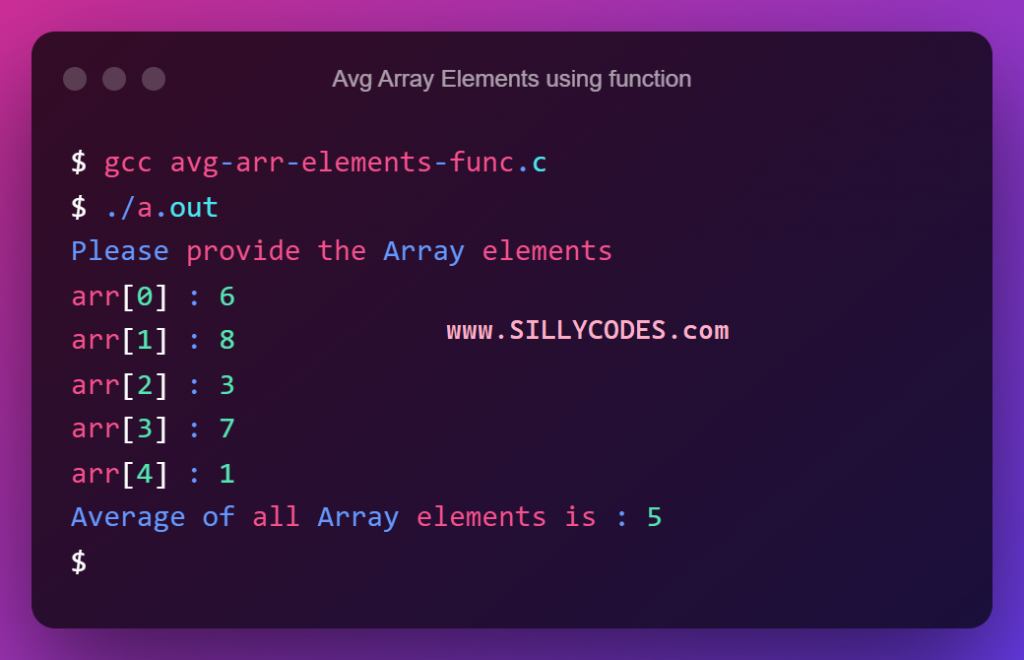
Related Programs:
- C Program to Perform Arithmetic Operations using functions
- Add Two Numbers using Functions in C
- C Program to calculate Fibonacci Series using function
- C Program to find Factorial of a Number using functions
- C Program to find Minimum and Maximum numbers using Functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C