Functions with Variable Arguments in C Language
- Introduction:
- Functions with Variable Arguments in C Language:
- Types and Macros to create functions with Variable Arguments:
- Steps to create and access the variable arguments functions in C:
- Program 1: Function to Calculate Sum of variable number arguments in C:
- Example 2: Program to accept the variable number of arguments from the user and calculate the average:
- Conclusion:
- Related Articles:
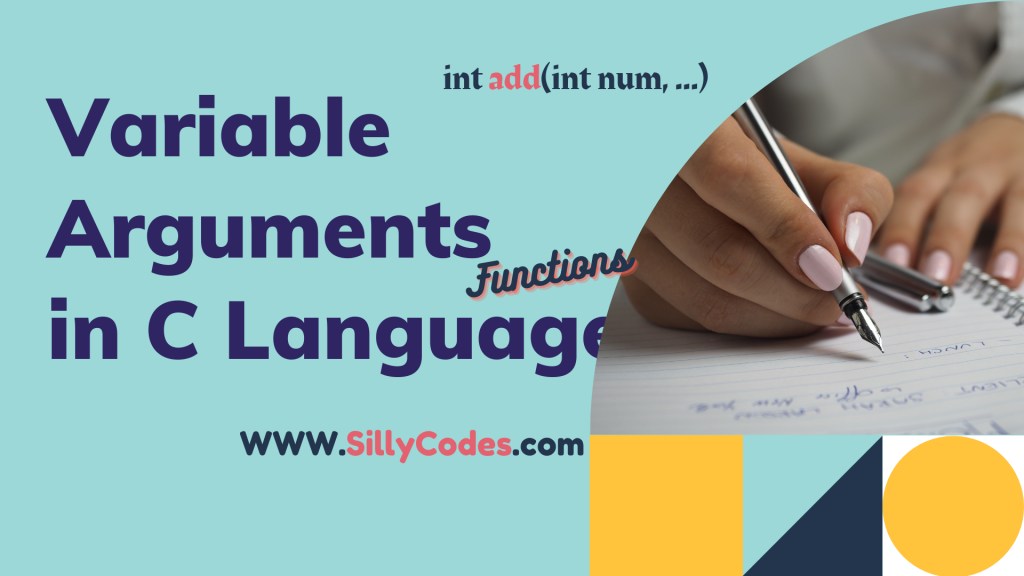
Introduction:
We have discussed the Recursion and Call by value and Call by Reference in our Earlier articles, In today’s article, we are going to look at the Functions with variable arguments in C programming language with Example Programs.
Functions with Variable Arguments in C Language:
So far We have looked at the functions with a fixed number of arguments, but there will be times when you don’t know the number of arguments beforehand, In such cases, we need to use the variable arguments for the functions. The C Programming language allows developers to create functions with variable arguments. Let’s look at them in detail.
We have already used the variable arguments functions many times during our practice programs, The standard input and output functions like scanf and printf are variable argument functions. These functions can accept a single variable or multiple variables depending on the situation.
Syntax of Variable Arguments function in C:
To define a variable argument function, Pass the mandatory arguments followed by the ellipsis ( …) symbol at the end.
Here is the syntax of the function with variable arguments.
1 2 3 4 5 |
// variable argument function return_type function_name(int num, ...) { // Function Body } |
Please note that We added three dots or ellipsis (…) at the end of the argument list or parameters list, which tells the compiler that the function_name function is a variable argument function.
Example of Variable Argument function:
1 2 3 4 |
int product(int num1, ...) { // Function body } |
The above function product takes one mandatory or fixed argument num1 which is of the integer type, the rest of the arguments can be vary depending upon the requirement. We can have one, two, or any number of arguments. That is the reason the above product function is called the variable argument function.
📢The variable argument function should contain the ellipsis (…) and The ellipsis should always be the last argument of the argument list. We can’t add the ellipsis in between the argument list.
Valid: func(int n, …); ✅
Invalid: func(int n, …, float k, int a); ❌
Variable Argument Library Functions:
If we look at the use cases of the printf or scanf functions, We will get a good idea of how variable arguments are working.
The printf function with a single argument:
printf("Hello World !\\n");
above printf function has only one argument, which is of type string.
The printf function with multiple arguments:
printf("The given Number is : %d \\n", number);
The above printf function has two arguments, One string argument with a format specifier and an integer variable number
printf("The LCM of %d and %d is : %d \\n", number1, number2, lcm);
Similarly, Here is a printf function with four arguments, One string with format specifiers and variables number1, number2, and lcm.
This way we can pass any number of arguments to the printf function. Similarly, The scanf function is also a variable argument function, which Can accept the variable length of arguments.
Types and Macros to create functions with Variable Arguments:
The C Programming language provides a few types and macros to create and access the variable arguments functions. The above types and macros are available as part of the stdarg.h header file. So we need to include the stdarg.h header file to create and use the variable argument functions.
Let’s look at the types and macros provided by the stdarg.h header file.
- va_list – type:
- The va_list type is used to declare the variable-argument list pointer. By using this va_list variable we can access the variable arguments
- va_start:
- The va_start macro is used to Initialize the va_list type variable ( variable-argument pointer variable initialization)
- va_arg:
- The va_arg macro is used to Retrieve the present argument from the va_list type variable and increment it.
- va_end:
- The va_end macro is used to Invalidate the va_list type variable by assigning the NULL to it.
Steps to create and access the variable arguments functions in C:
1. Include the stdarg.h header file to the program
1 |
#include <stdarg.h> |
2. Create the variable argument function by specifying the ellipsis symbol
1 |
int sum(int num, ...); |
3. To access the variable length arguments inside the function, We need to create the variable argument pointer with the type va_list
1 |
va_list args_list; |
4. Initialize the variable argument pointer using the va_start macro.
- The va_start function takes two variables, First one is the variable of type va_list that we created in the above step( step 4) and the second argument specifies the number of arguments in the variable argument list.
- This num should be equal to the number of variable arguments passed to the function. If you pass 3 arguments(excluding num), The num should be 3.
- The va_start points the args_list to the first value of the variable argument list.
- As the num specifies the total number of variable arguments, We can use it to get the values from the variable argument list.
1 |
va_start(args_list, num); |
5. Retrieve the variable argument value using the va_arg macro.
- The va_arg macro takes two parameters, They are args_list of va_list type and the data type of the variable being retrieved. Here we are retrieving the integer variable so we used the int as the second parameter.
- The va_arg macro, Retrieves the present value and increments the args_list variable so that it will be pointed to the next variable in the variable-argument list.
- We can call the va_arg the num number of times to get all values passed to the function.
1 |
value = va_arg(args_list, int); |
6. Once we accessed all the variable arguments, Call the va_end to release the resources. Pass the args_list variable to va_end function.
1 |
va_end(args_list); |
Let’s look at a couple of example programs to understand how functions with variable length arguments work in C programming language.
Program 1: Function to Calculate Sum of variable number arguments in C:
In the following program, We are going to create a function called add, The add function accepts variable length arguments and calculates the sum of them, and returns it.
As we have specified earlier, The add function accepts the variable number of arguments by using the ellipsis( …) syntax.
int add(int num, ...)
- The first argument of the
add function is
num, which is of integer type. The
num specifies the total number of variable arguments which are going to be passed to the function.
- To get the values from the va_list we use this num.
- Call the va_arg macro num number of times to get all values in the variable argument list (the values passed as arguments to the function)
- The second argument is ellipsis ...
- add function calculates the sum and returns it. So the return type of add is int.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
/* Program to understand variable argument in C */ #include <stdio.h> #include <stdarg.h> // to access variable length arguments /** * @brief Calculate the sum of variable number of arguments passed to function. * * @param num - Total number of variable arguments. * @param ... - variable argument list * @return int - sum of input numbers */ int add(int num, ...) { int i, sum = 0; // declare the 'va_list' variable va_list args_list; // Initialize the `va_list' type variable va_start(args_list, num); // The 'num' specifies the number of variable arguments passed. // Loop until we reach 'num' and get each value using 'va_arg' for(i = 0; i<num; i++) { // get the variable argument value using 'va_arg' sum = sum + va_arg(args_list, int); } // finished the operation. set the 'args_list' to NULL. va_end(args_list); return sum; } int main() { // call the 'add' function with 3 arguments - 1 fixed, 2 variable arguments printf("Result : %d \n", add(2, 20, 30)); // call the 'add' function with 5 arguments - 1 fixed, 4 variable arguments printf("Result : %d \n", add(4, 20, 30, 80, 70)); // call the 'add' function with 11 arguments - 1 fixed, 10 variable arguments printf("Result : %d \n", add(10, 20, 30, 80, 70, 4, 8, 3, 40, 71, 23)); return 0; } |
Program Output:
Compile and Run the program using GCC compiler(any compiler)
1 2 3 4 5 6 |
$ gcc variable_args-1.c $ ./a.out Result : 50 Result : 200 Result : 349 $ |
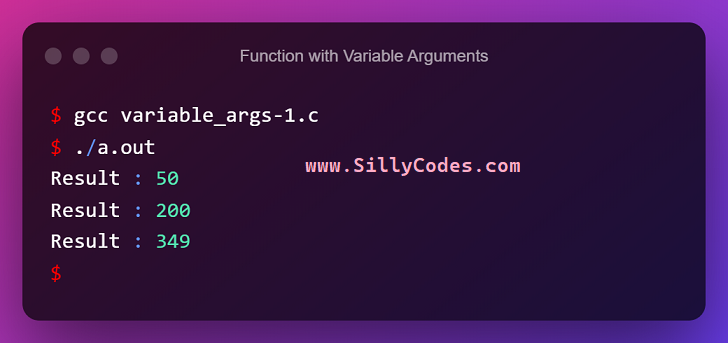
As we can see from the above output, The add function is able to calculate the sum of variable length arguments, We have made three calls to the add function with a different number of arguments. In the first call we passed two arguments(variable arguments), in the second call, We passed 4 arguments, and in the Third call, We passed 10 variable arguments, Still the add function was able to calculate the sum.
First Call:
printf("Result : %d \\n", **add**(2, 20, 30));
Here we passed 3 arguments to the add function,
- The first argument 2 specifies the total number of variable arguments.
- As we are passing the two variable arguments( 20, 30) we specified 2 as the first argument.
Output:
Result : 50
Second Call:
printf("Result : %d \\n", **add**(4, 20, 30, 80, 70));
Here we passed 5 arguments,
- The first argument specifies the length of the variable argument list, which is 4
- The variable argument list is 20, 30, 40, and 50.
Output:
Result : 200
Third Call:
printf("Result : %d \\n", **add**(10, 20, 30, 80, 70, 4, 8, 3, 40, 71, 23));
Here we passed 11 arguments
- The first argument contains the length of the argument list – i.e 10
- The next 10 arguments are variable arguments.
Output:
Result : 349
Example 2: Program to accept the variable number of arguments from the user and calculate the average:
This program going to accept variable length input values from the user and makes a call to the average function to calculate the average of the given numbers.
The average function is a variable argument function and can calculate the average of different length arguments using the stdarg.h headerfile’s va_list type.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
/* Program to understand variable argument in C */ #include <stdio.h> #include <stdarg.h> // to access variable length arguments /** * @brief - Caclulate Avg of variable arguments passed to function * * @param num - Total number of variable arguments * @param ... - variable argument list * @return int - average of given variable arguments */ int average(int num, ...) { int i, sum = 0; // declare the 'va_list' variable va_list args_list; // Initialize the `va_list' type variable va_start(args_list, num); // The 'num' specifies the number of variable arguments passed. // Loop until we reach 'num' and get each value using 'va_arg' for(i = 0; i<num; i++) { // get the variable argument value using 'va_arg' sum = sum + va_arg(args_list, int); } // finished the operation. set the 'args_list' to NULL. va_end(args_list); // return the average - which is sum/num return sum/num; } int main() { // First Input int n1, n2, n3, n4, n5, n6, result; printf("Enter 3 values to calculate the average: "); scanf("%d%d%d", &n1, &n2, &n3); // call the 'average' function with 4 arguments - 1 fixed, 3 variable arguments result = average(3, n1, n2, n3); printf("Average of %d,%d,%d is : %d\n", n1, n2, n3, result); // Second Input n1 = n2 = n3 = result = 0; printf("Enter 6 values to calculate the average: "); scanf("%d%d%d%d%d%d", &n1, &n2, &n3, &n4, &n5, &n6); // call the 'average' function with 7 arguments - 1 fixed, 6 variable arguments result = average(6, n1, n2, n3, n4, n5, n6); printf("Average of %d,%d,%d,%d,%d,%d is : %d\n", n1, n2, n3, n4, n5, n6, result ); return 0; } |
Here is the prototype of the average function:
- int average(int num, ...);
- Function_name: average
-
Argument_list: variable argument list
- First argument num – contains the total number of variable arguments passed to average function.
- Second argument ... – specifies the variable argument list
- Return_type: int – calculates the average of the given variable length arguments.
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 |
$ gcc avg-var-arg.c $ ./a.out Enter 3 values to calculate the average: 10 20 30 Average of 10,20,30 is : 20 Enter 6 values to calculate the average: 60 20 10 90 30 70 Average of 60,20,10,90,30,70 is : 46 $ ./a.out Enter 3 values to calculate the average: 9 2 4 Average of 9,2,4 is : 5 Enter 6 values to calculate the average: 1 3 9 2 8 1 Average of 1,3,9,2,8,1 is : 4 $ |
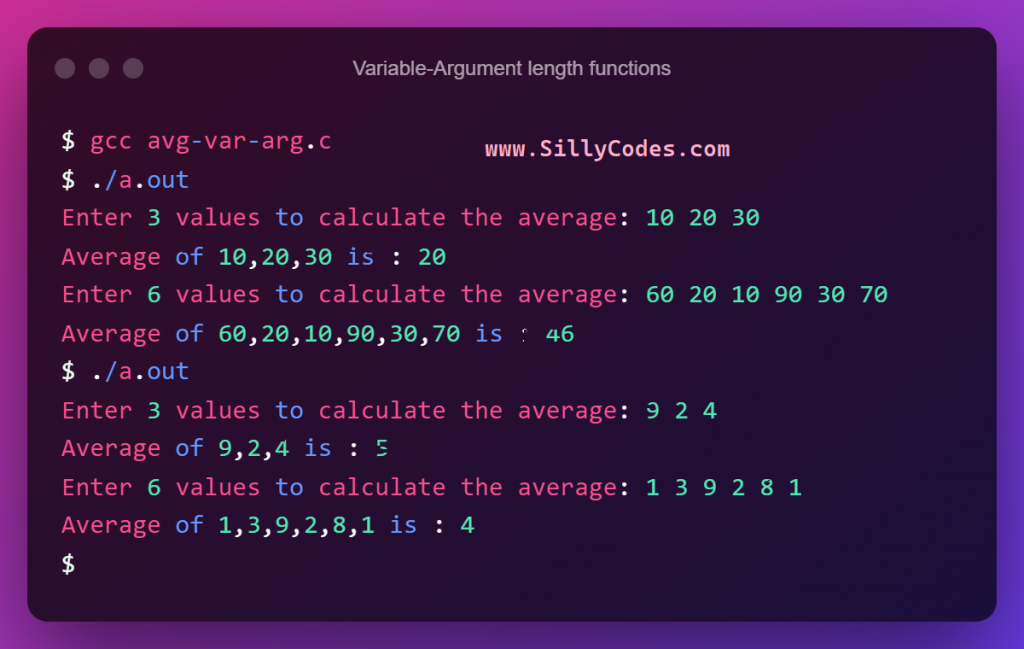
As you can see from the above output, The program is able to calculate the average of the variable arguments. In the first function call to the average function, We passed 3 variable arguments, In the second call, We passed the 6 variable arguments.
To calculate the average of variable length arguments, The average function used the va_list type variable with the va_start, va_arg and va_end macros.
Conclusion:
In this article, We have looked at the functions with variable length arguments in C language – How to create and use the variable argument functions. Finally we looked at a couple of example program to make understanding concrete.
2 Responses
[…] Variable Argument functions in C Language […]
[…] Variable Argument functions in C Language […]