Program to Remove Trailing Whitespace in String in C Language
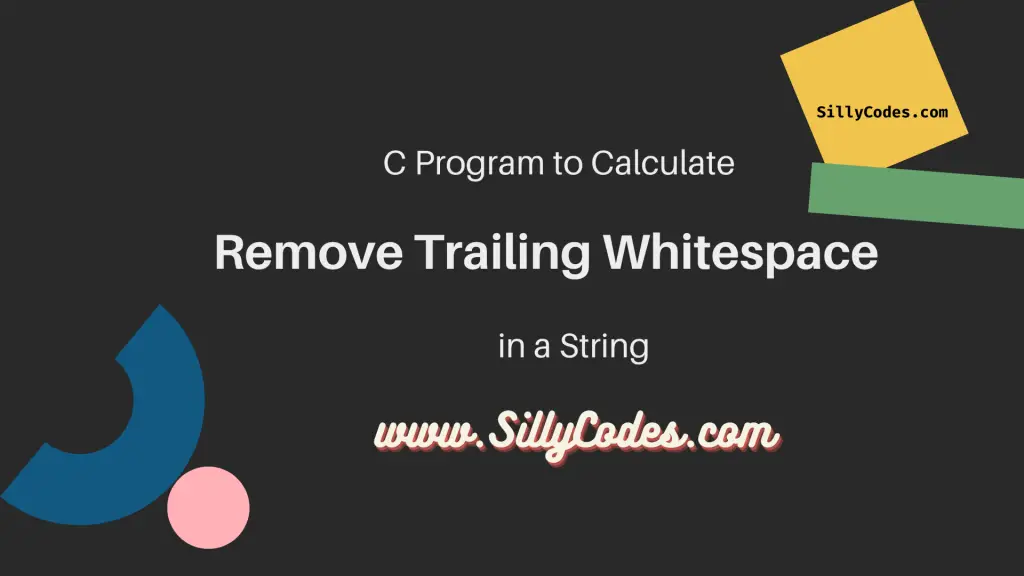
Program Description:
Write a Program to Remove Trailing Whitespace in C programming language. The program will accept a string from the user and removes or trims all the trailing white spaces from the input string.
📢 This Program is part of the C String Practice Programs series
Excepted Input and Output:
Input:
Enter a string : Programming is Fun
Output:
Before removing Trailing Whitespaces:Programming is Fun (Actual string)
After removing Trailing Whitespaces:Programming is Fun(Resultant string)
In the above example, The input string has trailing spaces, The program should remove the trailing spaces and return the valid NULL-terminated string.
Program Prerequisites:
It is recommended to have knowledge of the C Strings, Arrays, and Functions. Please go through the following article to learn more about them.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
Remove Trailing Whitespace in a String in C Program Explanation:
Let’s look at the step-by-step instructions of the program.
- Create an integer constant named SIZEwith the value 100. This is the max size of the input string. ( Please change this value if you want to use large strings)
- Create a character array i.e string to store the user input. Let’s say we created a inputStr string with the size of SIZE elements.
- Take the input string( inputStr) from the user and Read it using the gets function. We can also use other functions like scanf, fgets, etc. Also, note that the gets function doesn’t check boundaries.
- Create a variable called endand initialize it with the last character in the string i.e strlen(inputStr) - 1. This variable is used to hold the index of the last non-whitespace character in the inputStr
- Create a While Loop and Iterate over the input string. Start the loop from the
endindex (the last character in the string) and check if the character at the
endindex is a white space. We use
isspace() function from the
ctype.h header file.
- If the character at the index endis a white space, Then decrement the endby 1 – i.e end--;
- Repeat this step till we find a non-whitespace character in the string.
- Once Step 5 is completed, The endindex will be pointing to the last non-whitespace character in the string. So this will be the actual end of the string.
- To Remove the Trailing whitespace from the string, Simply, Add the Terminating NULL Character ( '\0') at the end+1 index. – i.e inputStr[end+1] = '\0';
- Print the Resultant string on Console.
Program to Remove Trailing Whitespace in String in C:
Here is the program to Remove the Trailing white spaces from a string in C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
/* program to Remove Trailing white spaces in a String in C sillycodes.com */ #include<stdio.h> #include<ctype.h> #include<string.h> // Max Size of string const int SIZE = 100; int main() { // declare a string with 'SIZE' char inputStr[SIZE]; int i; // get the string from the user printf("Enter a string : "); gets(inputStr); printf("Before removing Trailing Whitespaces:%s(Actual string)\n", inputStr); // Create a variable 'end' and assign 'len-1' int end = strlen(inputStr) - 1; // Create a loop and check for trailing white spaces while(isspace((unsigned char)inputStr[end])) { // found trailing whitespace // decrement 'end' end--; } // 'end' contains the actual end of the string. // If there are any trailing white spaces. if(end != (strlen(inputStr) - 1)) // we can even remove this check { // add terminating NULL Character at 'end+1' inputStr[end+1] = '\0'; } // Print Results printf("After removing Trailing Whitespaces:%s(Resultant string)\n", inputStr); return 0; } |
Program Output:
Compile and Run the Program.
1 2 3 4 5 6 |
$ gcc trailing-whitespaces.c $ ./a.out Enter a string : Programming is Fun Before removing Trailing Whitespaces:Programming is Fun (Actual string) After removing Trailing Whitespaces:Programming is Fun(Resultant string) $ |
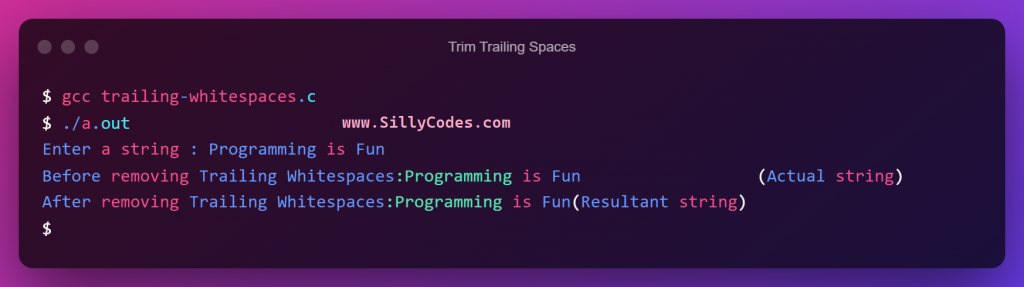
Let’s try one more example with Tabs:
1 2 3 4 5 |
$ ./a.out Enter a string : Learn Programming at sillycodes.com Before removing Trailing Whitespaces:Learn Programming at sillycodes.com (Actual string) After removing Trailing Whitespaces:Learn Programming at sillycodes.com(Resultant string) $ |
As we can see from the above output, The program is properly removing the trailing white spaces from the input string. Note that, We intentionally added the (Actual String) at the end of the string to represent the spaces.
Remove Trailing Whitespace in String in C using a user-defined function:
In this method, We are going to create a user-defined function to remove the trailing white spaces in the input string.
Related: Advantages of the Functions
Here is the program to remove the Trailing whitespace using a function named trimTrailingWSpaces. The trimTrailingWSpaces() function takes a string as the input and removes the all trailing or ending white spaces.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
/* program to Remove Trailing White spaces in a String in C using functions sillycodes.com */ #include<stdio.h> #include<ctype.h> #include<string.h> // Max Size of string const int SIZE = 100; /** * @brief - Trim / Remove Trailing white spaces in the given string. * * @param str - Input String * @return char* - Returns resultant string */ char * trimTrailingWSpaces(char * str) { int i; // Create a variable 'end' and assign 'len-1' int end = strlen(str) - 1; // Create a loop and check for trailing white spaces while(isspace((unsigned char)str[end])) { // found trailing whitespace // decrement 'end' end--; } // 'end' contains the actual end of the string. // Add terminating NULL Character at 'end+1' str[end+1] = '\0'; return str; } int main() { // declare a string with 'SIZE' char inputStr[SIZE]; int i; // get the string from the user printf("Enter a string : "); gets(inputStr); printf("Before removing Trailing Whitespaces:%s(Actual string)\n", inputStr); // call the 'trimTrailingWSpaces' with 'inputStr' to remove the the Trailing spaces trimTrailingWSpaces(inputStr); // Print Results printf("After removing Trailing Whitespaces:%s(Resultant string)\n", inputStr); return 0; } |
We have called the trimTrailingWSpaces(inputStr) function with the inputStr from the main() function to remove the trailing whitespace from the input string ( inputStr).
The Prototype of the trimTrailingWSpaces() function is char * trimTrailingWSpaces(char * str). This function takes the input string as the formal argument and removes the trailing spaces and returns the resultant string back to the caller.
Program Output:
Let’s compile and Run the Program and observe the output.
1 2 3 4 5 6 7 8 9 10 |
$ gcc trailing-whitespaces-func.c $ ./a.out Enter a string : C Programming Language Before removing Trailing Whitespaces:C Programming Language (Actual string) After removing Trailing Whitespaces:C Programming Language(Resultant string) $ ./a.out Enter a string : Graph Theroy Before removing Trailing Whitespaces:Graph Theroy (Actual string) After removing Trailing Whitespaces:Graph Theroy(Resultant string) $ |
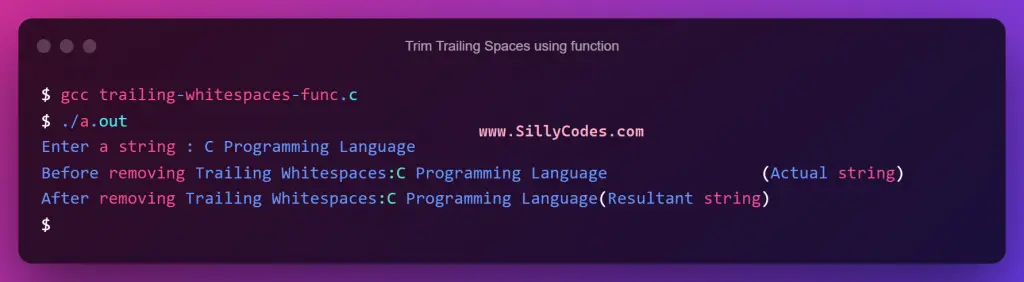
The function is properly removing the trailing white spaces.
Related Articles:
- C Tutorials Index
- C Programs Index
- C Program to Calculate Length of the string
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
3 Responses
[…] C Program to Remove Trailing Whitespaces in a String […]
[…] String with trailing spaces […]
[…] C Program to Remove Trailing Whitespaces in a String […]