Program to Copy Array to Another Array in C Language
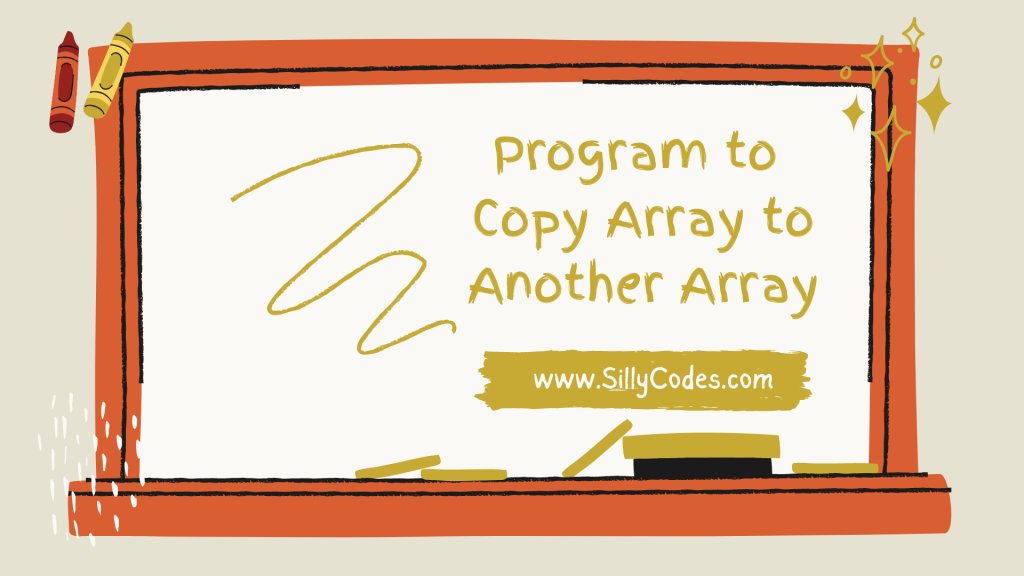
Program Description:
Write a Program to Copy Array to Another Array in C programming language. The program will take an input array from the user and copy all elements of the array to another array. So it is a deep copy.
Example Input and Output:
Input:
1 2 |
Enter desired array size(1-1000): 10 Please enter array elements(10) : 99 88 00 22 33 44 77 88 11 22 |
Output:
1 2 |
Original Array: 99 88 0 22 33 44 77 88 11 22 Copied(dup) Array: 99 88 0 22 33 44 77 88 11 22 |
Prerequisites:
We are going to use the arrays and functions in C language in the array copy program. So it is recommended to go through the following articles, where we explained about the arrays and functions.
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- Functions in C Langauge with Example Programs
- 2D arrays in C with Example programs
- How to pass arrays to functions in C
Copy Array to Another Array in C Program Explanation:
- Start the program by declaring two arrays numbers and dup with the max size of 1000.
- Take the desired Array size from the user and store the provided value in the size variable.
- Check the size. As we support max of 1000 elements in the array. Display an error message if the provided size is out of bounds.
- Now, Prompt the user to provide the values for array elements.
- Use a For Loop to iterate over the array from to size-1 and accept the user input and update the &number[i] element using scanf function.
- Display the Original Array on the console.
- To copy the elements of the
numbersarray to another array, We need to iterate over the elements of
numbers array and copy each element to the new array. We have already created a new array
dup at the start of the program.
- So Start the loop from the first element and traverse till the size-1 and copy each element. – dup[i] = numbers[i];
- Once the above For loop is completed, The dup array will contain a copy of the numbers array.
- Display the dup array onto the console using another loop.
Copy Array to Another Array in C Algorithm:
- Declare numbers and dup array with size 1000 and also declare variables i and size
- Take the max size of the array from the user and update the size variable.
- Check the size, if it is out of bounds – if(size<=0 || size>1000)
- Update the array with user input.
- Loop from 0 to size-1 using FOR.
- update &numbers[i]
- Display the Original Array i.e
numbers array.
- Loop from 0 to size-1 using FOR.
- Display the numbers[i]
- Copy the
numbers array elements one by one to
dup array.
- Loop from 0 to size-1 using FOR.
- Â dup[i] = numbers[i];
- Display the Duplicate Array i.e
dup array.
- Loop from 0 to size-1 using FOR.
- Display the dup[i]
- Stop the program.
Program to Copy Array to Another Array in C Language:
Let’s convert above algorithm into the code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
/*     Program COpy an array to another array     Author: SillyCodes.com */  #include <stdio.h>  int main() {      // declare the 'numbers' array and 'dup' array     int numbers[1000], dup[1000] = {0};     int i, size;      printf("Enter desired array size(1-1000): ");     scanf("%d", &size);      // check size     if(size<=0 || size>1000)     {         printf("Invalid Size, Please try again\n");         return 0;     }      // User input for array elements     printf("Please enter array elements(%d) : ", size);     for(i = 0; i < size; i++)     {         // printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     }         // Print the original array     printf("Original Array: ");     for (i = 0; i < size; i++)     {         printf("%d ", numbers[i]);     }     printf("\n");      // Copy the array     for(i=0; i<size; i++)     {         // copy 'number[i]' to 'dup[i]'         dup[i] = numbers[i];     }      // Print the copied array - 'dup'     printf("Copied(dup) Array: ");     for (i = 0; i < size; i++)     {         printf("%d ", numbers[i]);     }     printf("\n");      return 0; } |
Program Output:
Compile the program.
$ gcc copy-array.c
Run the Program.
Test 1: Copy Array to Another Array.
1 2 3 4 5 6 7 8 9 10 11 |
$ ./a.out Enter desired array size(1-1000): 10 Please enter array elements(10) : 99 88 00 22 33 44 77 88 11 22 Original Array: 99 88 0 22 33 44 77 88 11 22 Copied(dup) Array: 99 88 0 22 33 44 77 88 11 22 $ ./a.out Enter desired array size(1-1000): 5 Please enter array elements(5) : 1 2 3 4 5 Original Array: 1 2 3 4 5 Copied(dup) Array: 1 2 3 4 5 $ |
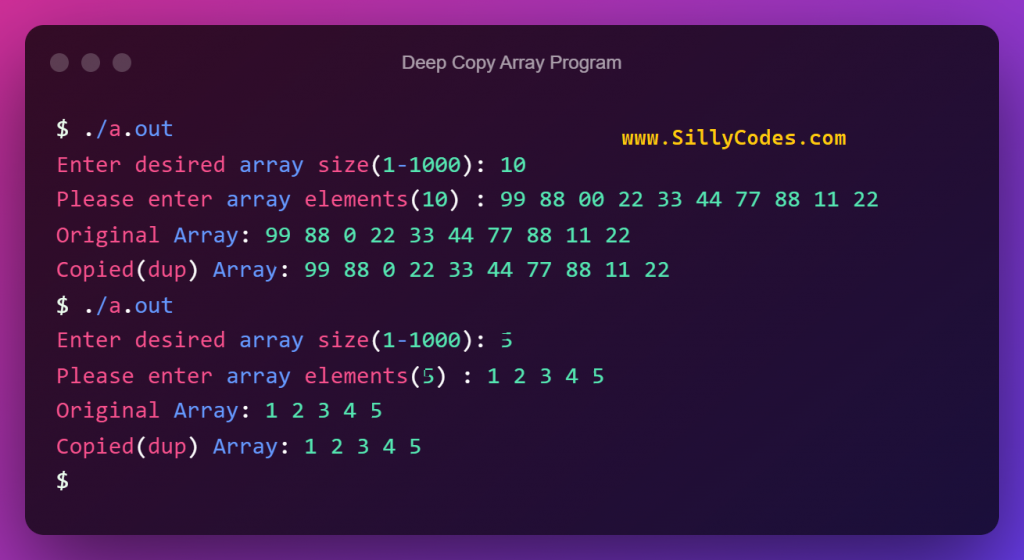
As we can see from the above output, We are getting the expected results.
Test 2: Array Out of bounds :
1 2 3 4 5 6 7 |
$ ./a.out Enter desired array size(1-1000): -1 Invalid Size, Please try again $ ./a.out Enter desired array size(1-1000): 1100 Invalid Size, Please try again $ |
As expected, The program is displaying the error message Invalid Size, Please try again.
Copy Array to Another Array in C using Functions:
In the above program we used only one function to write the program. Let’s divide the above program into the set of functions, So that each function will do a specific task.
Here is the re written version of the above program. This program uses three functions read, display and copyArray.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 |
/*     Program to copy array using function     Author: SillyCodes.com */  #include <stdio.h> void copyArray(int source[], int destination[], int size);  /** * @brief  - Read the user input and update the 'numbers' array * * @param numbers - 'numbers' array * @param size    - size of the 'numbers' array */ void read(int numbers[], int size) {     int i;     // User input for array elements     printf("Please enter array elements(%d) : ", size);     for(i = 0; i < size; i++)     {         // printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     } }  /** * @brief  - Display the 'numbers' array * * @param numbers - Array * @param size    - Array size */ void display(int numbers[], int size) {     int i;     for(i = 0; i < size; i++)     {         printf("%d ", numbers[i]);        }     printf("\n"); }  int main() {      // declare the 'numbers' array and 'dup' array     int numbers[1000], dup[1000] = {0};     int i, size;      printf("Enter desired array size(1-1000): ");     scanf("%d", &size);      // check size     if(size<=0 || size>1000)     {         printf("Invalid Size, Please try again\n");         return 0;     }      // User input for array elements     read(numbers, size);      // Print the original array     printf("Original Array: ");     display(numbers, size);      // Call the 'copyArray' function with 'source' and 'destination' arrays     copyArray(numbers, dup, size);      // Print the copied array - 'dup'     printf("Copied(dup) Array: ");     display(dup, size);      return 0; }  /** * @brief    - Copy 'source' to 'destination' array * * @param source  - 'source' array * @param destination - 'destination' array * @param size      - size of arrays */ void copyArray(int source[], int destination[], int size) {     int i;     // Copy the array     for(i=0; i<size; i++)     {         // copy 'source[i]' to 'destination[i]'         destination[i] = source[i];     } } |
Here we have created three functions.
- The
read() function.
- Prototype of the read function – void read(int numbers[], int size)
- read() function takes two formal arguments which are numbers array and it’s size.
- The read() function is used to take the input numbers from the user and update the numbers array.
- The
display function
- Prototype of the display() function – void display(int numbers[], int size)
- The display() function also takes two formal arguments, which are numbers array and it’s size.
- display() function is used to display all array elements on the standard output i.e console.
- The
copyArray() Function.
- Here is the prototype of the copyArray() function – void copyArray(int source[], int destination[], int size)
- The copyArray() function takes three formal arguments, which are source array and destination array and size parameter.
- The copyArray() function will copy the elements of the source[] array to the destination[] array.
Program Output:
Let’s compile and run the program using GCC( any compiler)
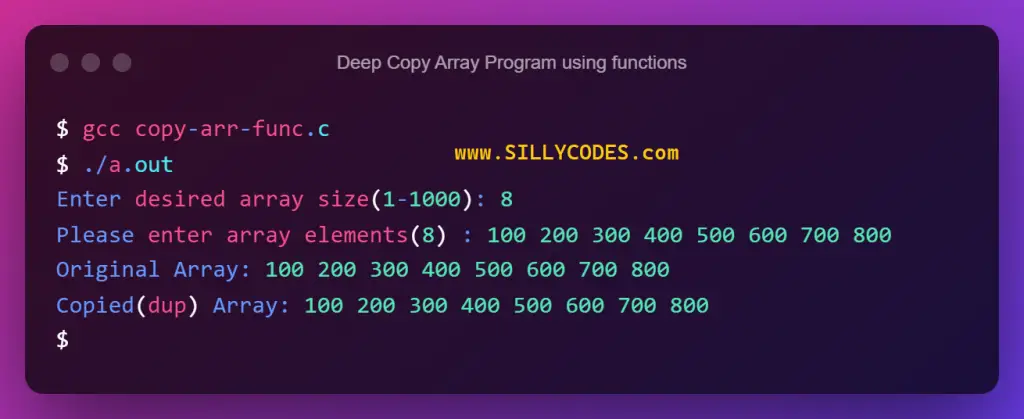
Test 1:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$ gcc copy-arr-func.c $ ./a.out Enter desired array size(1-1000): 8 Please enter array elements(8) : 100 200 300 400 500 600 700 800 Original Array: 100 200 300 400 500 600 700 800 Copied(dup) Array: 100 200 300 400 500 600 700 800 $ ./a.out Enter desired array size(1-1000): 4 Please enter array elements(4) : 1 2 3 4 Original Array: 1 2 3 4 Copied(dup) Array: 1 2 3 4 $ ./a.out Enter desired array size(1-1000): 5 Please enter array elements(5) : 12 87 -98 0 34 Original Array: 12 87 -98 0 34 Copied(dup) Array: 12 87 -98 0 34 $ |
Test 2:
1 2 3 4 5 6 7 |
$ ./a.out Enter desired array size(1-1000): -100 Invalid Size, Please try again $ ./a.out Enter desired array size(1-1000): 100000 Invalid Size, Please try again $ |
As we can see from the above outputs, The program is providing the desired output.
Related Programs:
- Index – C Language Practice Programs
- C Tutorials Index – Step by step tutorials to master C Langauge
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
- C Program to Find Maximum Element in Array
- C Program to Find Second Largest Element in Array
- C Program to Find Second Smallest Element in Array
1 Response
[…] C Program to Copy array to Another Array […]