Delete Duplicate Elements in Array in C Programming
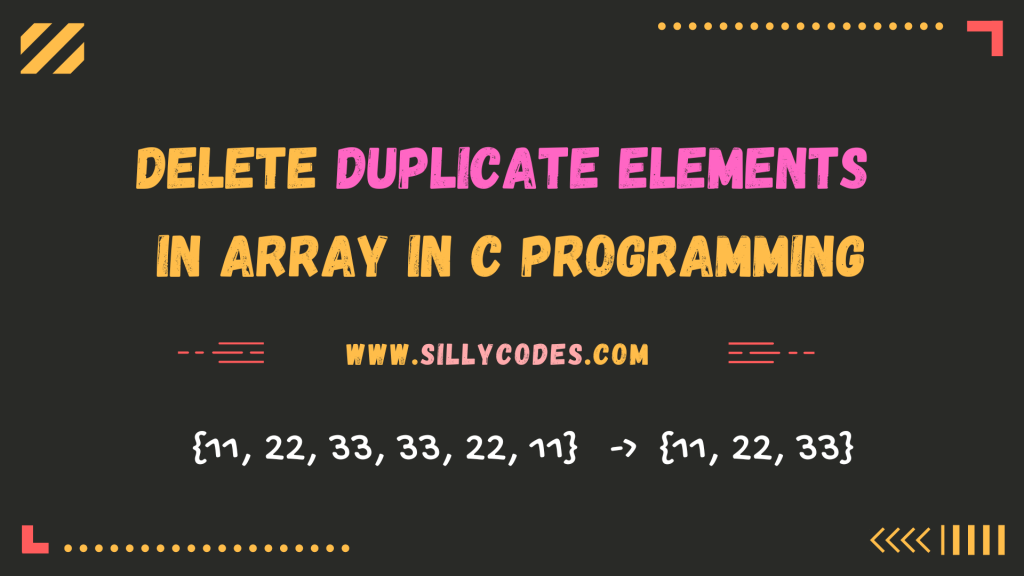
Program Description:
Write a Program to Delete Duplicate Elements in Array in C programming language. The program should accept an input array from the user, Input array may contain duplicate elements, The program should remove all duplicate elements from the array and provide the results.
Here is the example input and output of the program.
Example Input and Output:
Input:
Enter desired array size(1-1000): 8
Please enter array elements(8) : 1 2 1 2 1 2 3 3
Output:
Original Array: 1 2 1 2 1 2 3 3
Array (without Duplicates): 1 2 3
The input array 1 2 1 2 1 2 3 3 has few duplicate elements, So the result after removing the duplicate elements is 1 2 3
📢 Related Programs:
This program is part of the Series of Array Practice Programs in C Language
Prerequisites:
It is recommended to have a basic knowledge of the Arrays, and Functions in C language. Please go through the following articles to learn more about them.
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- 2D arrays in C with Example programs
- How to pass arrays to functions in C
- Functions in C Language
Delete Duplicate Elements in Array in C Algorithm:
To delete the duplicate elements in the input array, We are going to create a new array that is going to store distinct elements of the original array. This is equal to deleting all duplicate elements from the array.
So we need to go through the original array element by element and Insert the element into the new array only if the current element is not present in the new array.
Once the above loop is completed, The new array will contain only the distinct elements (i.e without duplicate elements)
In the end, If we want to replace the original array, We can replace the original array elements with the new array elements.

Now let’s look at the step-by-step explanation of the delete duplicate elements in the array program.
Delete Duplicate Elements in Array in C Program Explanation:
- Declare the numbers array, with the size of 1000. Also, create an array named noDups with 1000 elements. The noDups array is going to store the distinct elements of the numbers array.
- Take the desired array size from the user and update the size variable.
- Check for the array bounds using the if(size >= 1000 || size <= 0), Display an error message if the size goes beyond the bounds.
- Request input from the user for the array’s elements, and update the array.
- To get the distinct elements of the array, Traverse through the original array (i.e numbers) and Update the noDups array only if the current element( numbers[i]) is not found in the noDups array.
- Start a For loop from i=0 to i<size to iterate over the original array.
- Use isFound variable to check if the element is found in the noDups array. Set isFound=0 at the start of each iteration.
- Create another for loop from j=0 to j<noDupsSize
- check if the present number number[i] is present in the noDups array.
- if the number[i] == <code>noDups[j] condition is true, then update the isFound=1. and break out of the loop.
- Check the isFound is still zero(0), If so, Then the number[i] element is not found in the noDups array, So update the noDups array with the number[i] – noDups[noDupsSize] = numbers[i];
- Increment the noDupsSize – noDupsSize++
- Once the above two loops are completed, The noDups array will not contain any duplicate elements. So we are able to delete duplicate elements from the array.
- Display the results. First display the input array by using a For loop, Then display the noDups array using another for loop.
📢We can also replace the elements of the input array( numbers) with the new array( noDups) elements.
Program to Delete Duplicate Elements in Array in C Language:
Let’s convert above algorithm into the C Program, which deletes all duplicate elements from the input array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 |
/*     Program to delete duplicates from the array     Author: SillyCodes.com */  #include <stdio.h>  int main() {      // declare the 'numbers' array and Initialize 'noDups' array     int numbers[1000], noDups[1000] = {0};     int i, j, size, noDupsSize = 0, isFound = 0;      printf("Enter desired array size(1-1000): ");     scanf("%d", &size);      // check for array bounds     if(size >= 1000 || size <= 0)     {         printf("Invalid Size, Try again \n");         return 0;     }      // User input for array elements     printf("Please enter array elements(%d) : ", size);     for(i = 0; i < size; i++)     {         // printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     }      // Iterate over the original array 'numbers'     // and update the 'noDups' array only if the element is not found in the 'noDups' array     for(i=0; i<size; i++)     {         // update the isFound with '0' at the start of each iteration         isFound = 0;         for(j=0; j<noDupsSize; j++)         {             if(numbers[i] == noDups[j])             {                 // 'numbers[i] is found in 'noDups' array                 // so mark the 'isFound' as 1                 isFound = 1;                 break;             }         }          // update the 'noDups' array if 'isFound' is equal to zero         if(isFound == 0)         {             // 'number[i]' is not found in the 'noDups' array             // 'noDupsSize' starts from '0'             noDups[noDupsSize] = numbers[i];             noDupsSize++;         }     }         // Print the original array     printf("Original Array: ");     for(i = 0; i < size; i++)     {         printf("%d ", numbers[i]);        }     printf("\n");      // If you want to replace, you can replace the original array like below     // Replace the original Array with new array.     // for(i=0; i<noDupsSize; i++)     // {     //    numbers[i] = noDups[i];     // }     // size = noDupsSize;      // Print the 'noDups'  array     printf("Array (without Duplicates): ");     for(i = 0; i < noDupsSize; i++)     {         printf("%d ", noDups[i]);        }     printf("\n");      return 0; } |
Program Output:
Now, We need to compile and Run the Program.
Compile the program using GCC compiler (Any compiler)
$ gcc delete-duplicates.c
The above command generates an executable file named a.out (we are using GCC compiler on ubuntu Linux, If you are using a windows system, you will get .exe file).
Run the Executable file using the following command ( a.out)
Test Case 1: Normal Cases:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
$ gcc delete-duplicates.c $ ./a.out Enter desired array size(1-1000): 10 Please enter array elements(10) : 1 2 1 1 1 3 4 5 4 5 Original Array: 1 2 1 1 1 3 4 5 4 5 Array (without Duplicates): 1 2 3 4 5 $ gcc delete-duplicates.c $ ./a.out Enter desired array size(1-1000): 8 Please enter array elements(8) : 1 2 1 2 1 2 3 3 Original Array: 1 2 1 2 1 2 3 3 Array (without Duplicates): 1 2 3 $ ./a.out Enter desired array size(1-1000): 5 Please enter array elements(5) : 18 98 34 98 76 Original Array: 18 98 34 98 76 Array (without Duplicates): 18 98 34 76 $ |
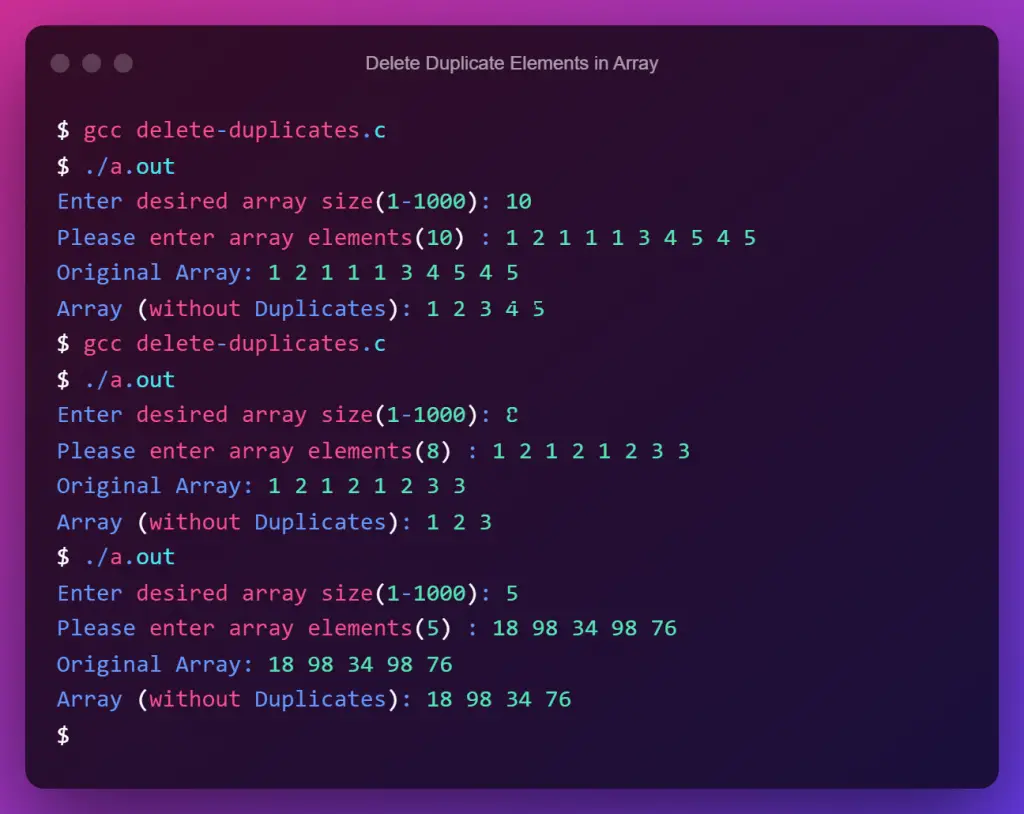
As we can see from the above output, The program is properly removing the duplicate elements.
For example, If the input array is 18 98 34 98 76, Then the program removed the duplicate element 98 and provided the output array as 18 98 34 76.
Test Case 2: Check the Array Bounds:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
// Out of bounds $ ./a.out Enter desired array size(1-1000): 0 Invalid Size, Try again $ ./a.out Enter desired array size(1-1000): 1234 Invalid Size, Try again $ ./a.out Enter desired array size(1-1000): 8 Please enter array elements(8) : 1 2 3 4 1 8 2 3 Original Array: 1 2 3 4 1 8 2 3 Array (without Duplicates): 1 2 3 4 8 $ |
As excepted, If the given array size is out of bounds, Then the program is displaying an error message which is Invalid Size, Try again.
Related Array Programs:
- Index – C Language Practice Programs
- C Tutorials Index – Step by step tutorials to master C Langauge
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
- C Program to Insert an element in Array
- C Program to Delete an Element in Array
- C Program to Find Unique Elements in an Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Find Maximum Element in Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
1 Response
[…] C Program to Delete Duplicate Elements in an Array […]