Program to Calculate length of String in C Language
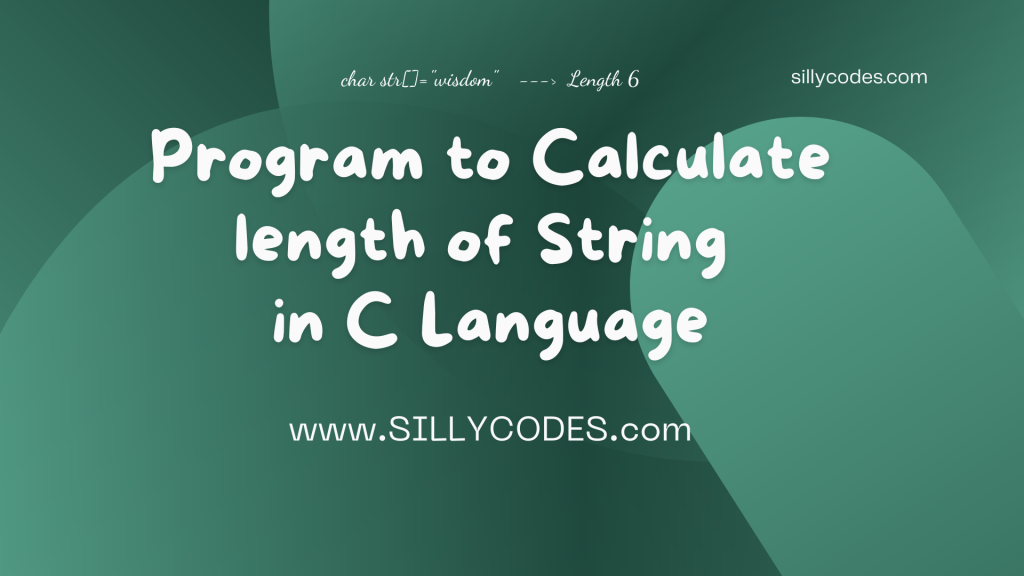
Program Description:
Write a Program to Calculate the Length of String in C programming language. We are going to look at different methods to calculate the length of the strings. They are
- Manual Method – Calculating the Length of the string using the iterative method
- User-defined function – Calculating the length of the string using a user-defined function
- strlen function – Using the strlen library function.
Example Input and Output:
Input:
Enter a string : Welcome
Output:
Length of the Given string 'Welcome' is : 7
Prerequisites:
It is recommended to have knowledge of C strings. Please go through the following articles to learn more about the strings.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
Calculate Length of String without using the strlen function (Iterative Way):
We can calculate the length of the string by iterating over the elements of the string and counting the number of characters until we reach the end of the string. As we already know the strings are terminated with the NULL characters. So we need to count the number of characters in the string till we reach the terminating NULL character.
Here is a step-by-step explanation of the program.
Calculate String Length using Iterative Method Program Explanation:
- Start the program by declaring a string and let’s say it is as str with the size SIZE(i.e 100).
- Prompt the user to provide the string for str and read it using the gets function. (The gets function won’t check the boundaries, so use it with caution).
- Initialize a variable named strLength with Zero(0). This variable contains the length of the given string ( str).
- Create a For loop to iterate over the string. Start the loop from index zero and go till we reach the terminating NULL character
\0.
- At each iteration, Increment the strLength variable – strLength++;
- Print the result on the console.
📢 This Program is part of the C String Practice Programs series
Program (Iterative Method ):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
/*     program to calculate the length of the string in c     sillycodes.com */  #include<stdio.h>  const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char str[SIZE];      // get the string from the user     printf("Enter a string : ");     // scanf("%s", mainStr);     gets(str);         // initialize the 'strLength' variable with zero     int strLength = 0;      // Iterate through the string and count characters     for(int i=0; str[i] != '\0'; i++)     {         // increment the 'strLength' variable         strLength++;     }      // Print the result     printf("Length of the Given string '%s' is : %d\n", str, strLength);      return 0; } |
Program Output:
Compile and Run the program using your favorite compiler. We are using GCC compiler in this example.
1 2 3 4 5 |
$ gcc string-length.c $ ./a.out Enter a string : Welcome Length of the Given string 'Welcome' is : 7 $ |
The input string Welcome has 7 characters
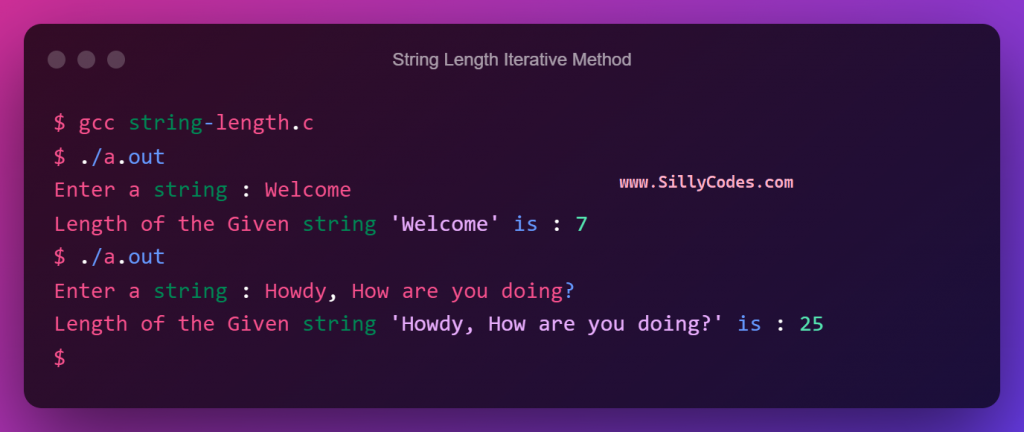
Let’s try a few more examples
1 2 3 4 5 6 7 |
$ ./a.out Enter a string : Howdy, How are you doing? Length of the Given string 'Howdy, How are you doing?' is : 25 $ ./a.out Enter a string : i Length of the Given string 'i' is : 1 $ |
The program is generating the excepted results.
Calculate Length of String using User-defined function(without strlen) in C:
Let’s look at the second method, Calculating the string Length using a custom user-defined function ( without strlen library function).
Let’s rewrite the above program and create a function named mystrlen. The mystrlen function takes a character pointer str (which points to the input string) and returns the length of the string ( str)
Here is the prototype of the custom string length function
int mystrlen(char *str)
The mystrlen function also uses a for loop to iterate over the string( str) and calculates the length of the string and returns the results back to the caller.
Here is the string length program with user-defined string length ( mystrlen) function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
/*     program to calculate the length of the string using user defined function in c     sillycodes.com */  #include<stdio.h> const int SIZE = 100;  /** * @brief  - 'mystrlen' function calculate the length of the given string 'str' * * @param str - input string * @return int - length of the 'str' string */ int mystrlen(char *str) {     // initialize the 'strLength' variable with zero     int strLength = 0;      // Iterate through the string and count characters     while(str[strLength] != '\0')     {         strLength++;     }      // return the string length     return strLength; }  int main() {      // declare a string with 'SIZE'     char str[SIZE];      // get the string from the user     printf("Enter a string : ");     // scanf("%s", mainStr);     gets(str);         // call the 'mystrlen' function with 'str' to calculate the length     int result = mystrlen(str);       // Print the result     printf("Length of the Given string '%s' is : %d\n", str, result);      return 0; } |
Program Output:
Let’s compile and Run the program
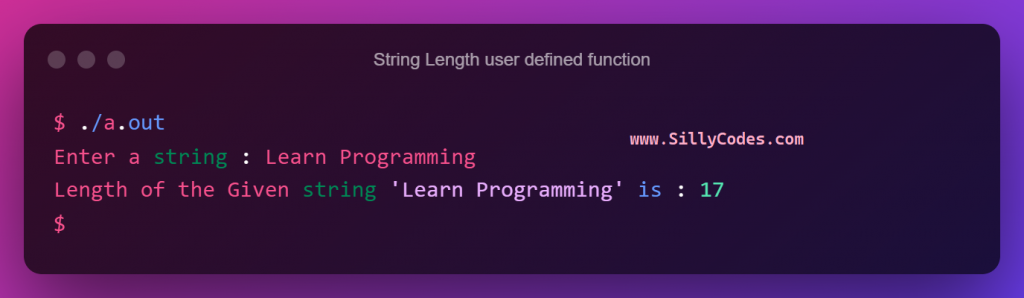
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a string : Learn Programming Length of the Given string 'Learn Programming' is : 17 $ ./a.out Enter a string : Bitcoin Length of the Given string 'Bitcoin' is : 7 $ ./a.out Enter a string : k8s Length of the Given string 'k8s' is : 3 $ |
The program is properly calculating the string length.
Program to Calculate Length of the string using strlen function in C:
The standard C Library provides the in-built function to calculate the length of the string, which is strlen() function. The strlen() function is declared in the string.h header file.
Let’s look at a program to calculate the string length using the strlen library function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/*     program to calculate the length of the string in c     sillycodes.com */  #include <stdio.h> #include <string.h>  // for 'strlen' function  // Max size of the array const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char str[SIZE];      // get the string from the user     printf("Enter a string : ");     // scanf("%s", mainStr);     gets(str);      // call the 'strlen' function with 'str'      int strLength = strlen(str);      // Print the result     printf("Length of the Given string '%s' is : %d\n", str, strLength);      return 0; } |
Program Output:
Compile and Run the program
1 2 3 4 5 6 7 8 |
$ gcc len-strlen.c $ ./a.out Enter a string : Wisdom Length of the Given string 'Wisdom' is : 6 $ ./a.out Enter a string : It always seems impossible until it’s done Length of the Given string 'It always seems impossible until it’s done' is : 44 $ |
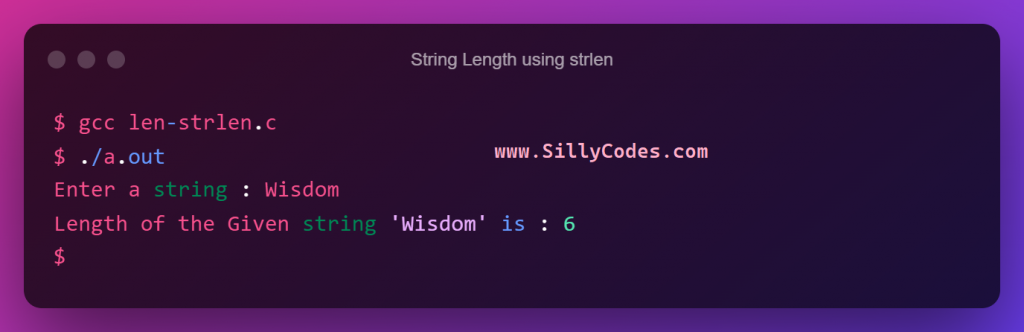
📢We have covered the strlen() library function in detail in the following article
Call the strlen() function with the input string and strlen() function calculates and returns the string length.
int strLength = strlen(str);
📢 It is recommended to use the strlen library function to calculate the string length. It is generally faster and more efficient than our custom implementations. So it is better to use the library functions.
Related Programs:
- C Tutorials Index
- C Programs Index
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
- C Program to Merge Two Arrays
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
1 Response
[…] C Program to Calculate Length of the String […]