Program to print first n prime numbers in C Language
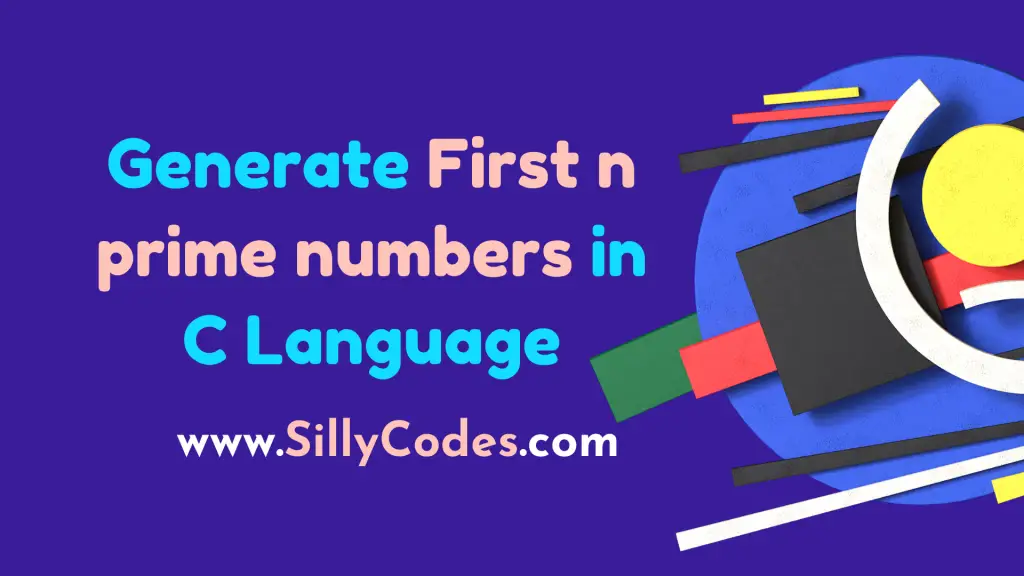
Introduction:
Write a Program to print first n prime numbers in C programming language. We are going to use two for loops (Nested Loops) to calculate the first n prime numbers. The program is going to accept a positive number ( n) from the user and generates that many(first n) prime numbers.
If the user enters a Negative number program should display an error message – Please enter positive number
Example Inputs and Output:
Input:
Enter how many prime numbers do you want to print : 10
Output:
2 3 5 7 11 13 17 19 23 29
Related Prime Numbers Programs:
This article is one of the articles in the Series of Prime number programs in C language. In our previous article, We discussed
- What is Prime Number and C program to Check given number is Prime or Not
- Check given Number is Prime or not Using Square Root(sqrt) Function.(Efficient way)
- C Program to generate prime numbers between two numbers
- C program to print prime numbers up to n
- C Program to Print nth Prime Number in C
First n prime numbers in C Program Explanation :
This Program accepts one integer from the user and prints first n prime numbers. If the user enters a Negative number, We display an error message and Ask for the user’s input again using the Goto Statement.
Here we are using two loops one outer loop and one inner loop.
The Outer loop will help us to track the number of prime numbers we printed onto the console, The Outer loop starts from the number 2 ( as prime numbers start from 2) and goes until we find n (user provided number) Prime Numbers. and The Inner loop is used to check if the given number is a prime number or not ( basic prime number checking logic).
As a result, the outer loop will determine whether or not any number between 2 and n are prime numbers.
We will also use a FLAG variable, The FLAG variable initialized with Zero (0) and We will update the FLAG variable inside of the Inner Loop with 1 whenever we found any number, which is not a prime ( i.e which is perfectly divisible by the other number)
Once we came out of the Inner loop, We will check if the FLAG is equal to zero(0). If so, Then the number i will be a prime number. We will print it onto the console and go to the next number.
We also maintain a Counter variable named cnt, The cnt variable will be incremented whenever we found any prime numbers. Our OuterLoop termination condition depends on the cnt variables which is cnt < n (Outer loop condition).
Here are the first 10 prime numbers:
n | nth Prime Number |
---|---|
1 | 2 |
2 | 3 |
3 | 5 |
4 | 7 |
5 | 11 |
6 | 13 |
7 | 17 |
8 | 19 |
9 | 23 |
10 | 29 |
Program to generate First n prime numbers in C Language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
#include<stdio.h> #include<math.h> void main() { int i,j,cnt,FLAG,n; cnt = 0; // we will increase this count by 1 for each prime LABEL: // goto statement label // Take the input from the user printf("Enter how many prime numbers do you want to print : "); scanf("%d",&n); if(n > 0) { for(j=2; cnt<n ; j++) { FLAG=0; // set this flag to zero for each iteration for(i=2;i <= j/2; i++) { if(j%i == 0) { // Set FLAG to 1 if given number is evenly // divisible by any other number b/w 2 & j/2 FLAG = 1; break; } } // if flag is still zero, that means Given Number is Prime. if(FLAG == 0) {// Number is primes, so let's print it now. cnt++ ; // increase count for each prime number printf("%d ",j); } } } else { printf("Please enter positive number \n"); goto LABEL; } printf("\n"); } |
Program Output:
We are compiling the program using the GCC compiler.
$ gcc first-n-primes.c
The above gcc command generates an executable file a.out. Run the executable file.
1 2 3 4 |
$ ./a.out Enter how many prime numbers do you want to print : 10 2 3 5 7 11 13 17 19 23 29 $ |
As you can see user entered n as 10, So the program generated 10 prime numbers.
Similarly, try a few more examples
1 2 3 4 5 6 7 |
$ ./a.out Enter how many prime numbers do you want to print : 8 2 3 5 7 11 13 17 19 $ ./a.out Enter how many prime numbers do you want to print : 100 2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97 101 103 107 109 113 127 131 137 139 149 151 157 163 167 173 179 181 191 193 197 199 211 223 227 229 233 239 241 251 257 263 269 271 277 281 283 293 307 311 313 317 331 337 347 349 353 359 367 373 379 383 389 397 401 409 419 421 431 433 439 443 449 457 461 463 467 479 487 491 499 503 509 521 523 541 $ |
Let’s try an example with a Negative number
1 2 3 4 5 6 7 8 |
$ ./a.out Enter how many prime numbers do you want to print : -3 Please enter positive number Enter how many prime numbers do you want to print : -12 Please enter positive number Enter how many prime numbers do you want to print : 5 2 3 5 7 11 $ |
As you can see from the above output, The program is displaying an Error message. and asking the user for the new Input number.
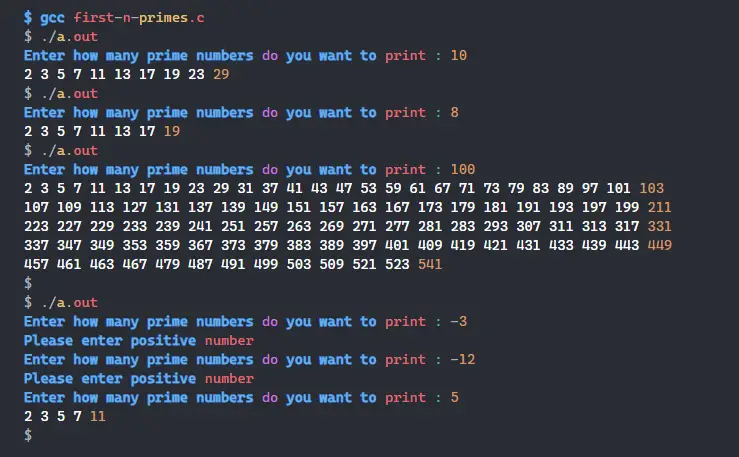
4 Responses
[…] C Program to print First N Prime numbers […]
[…] C Program to print First N Prime numbers […]
[…] C Program to print First N Prime numbers […]
[…] C Program to print First N Prime numbers […]