Enter week number and print day of week program in C Language
- Day of Week Program Description:
- Excepted Input and Output:
- Prerequisites:
- Method 1: Day of Week Program Explanation or Algorithm:
- Program: Day of week program in C language:
- Program Output:
- Method 2: Day of Week Program using the Switch statement in C programming
- Program Output:
- Related Programs:
- C Tutorials Index:
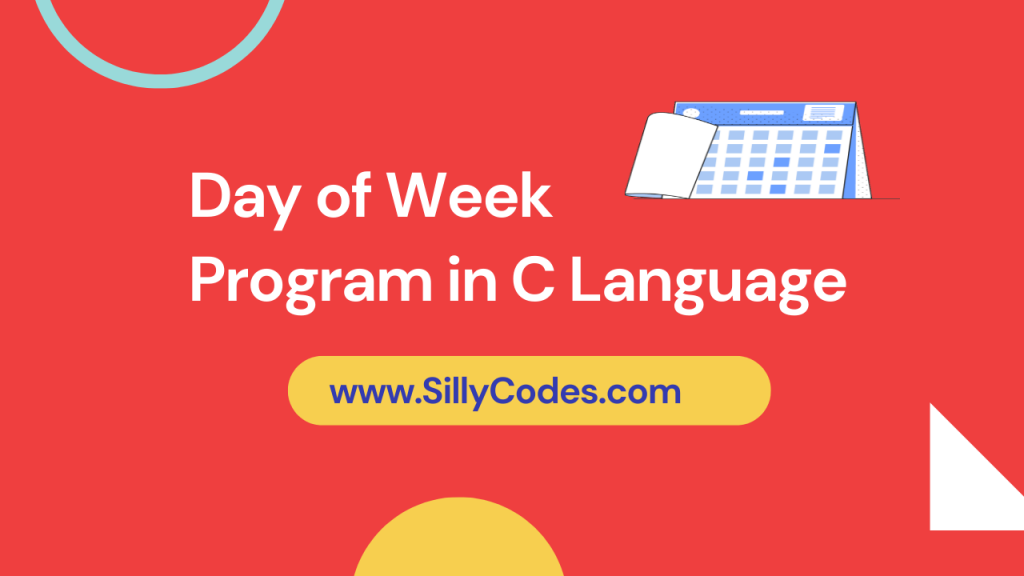
Day of Week Program Description:
Write a day of week Program in C language, Which takes the week Number from the user and print the day of Week using the if else condition. Day of the week program will take an Integer from the user and display the week day. And Program should do the error checking and display the Error message if the user enters an Invalid number.
If the user enters 1, The Program should display the Week day as Sunday, for 2 it should display Monday, and so on. Here is the table for the same.
Day Number | Day of Week |
---|---|
1 | Sunday |
2 | Monday |
3 | Tuesday |
4 | Wednesday |
5 | Thursday |
6 | Friday |
7 | Saturday |
Here are the excepted Input and output of the program
Excepted Input and Output:
Example1:
Input:
Enter day Number [1-7]: 4
Output:
Wednesday
Example1:
Input:
Enter day Number [1-7]: 6
Output:
Friday
Example1:
Input:
Enter day Number [1-7]: 9
Output:
ERROR: Invalid Input, Please provide valid input
Prerequisites:
We need to know the basics of the decision-making statements like the if..else statement, if else ladder, and switch case. please go through the following articles to learn more.
- Decision making statements if and if…else in C
- Nested if else and if..else ladder in C-Language
- Switch Statement in C Language
- Relational Operators.
We can write the Day of week program in different ways, Let’s look at the following two methods
- Method 1: Day of week using if else ladder
- Method 2: Day of week using switch statement or switch case.
Method 1: Day of Week Program Explanation or Algorithm:
We will use the if else ladder along with the relational operators to check the day of week of the given number.
- We take the week number from the user and store the week number to a variable day.
- Then we start the if else ladder and we will compare the given week number i.e day with the numbers 1, 2, 3, … 7.
- If the day is equal to 1, Then day of week is Sunday.
- Similarly, If the day is equal to 2, Then the day of week is Monday, Like this we check the week number ( day) with all the numbers up to 7.
- If the day doesn’t match any number from 1 to 7, Then User provided an Invalid Number. So at the final else statement, We are displaying the Error message saying – ERROR: Invalid Input, Please provide valid input
Program: Day of week program in C language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
/* Program: Check the weekday based on week Number Author: www.SillyCodes.com */ #include<stdio.h> int main() { int day; // Take the input from the user // Number 1 - for Sunday // Number 2 - Monday,.. so on. printf("Enter day Number [1-7]: "); scanf("%d", &day); // We are going to use the if..else ladder // and compare the 'day' with each value. if(day == 1) { // Sunday printf("Sunday\n"); } else if (day == 2) { printf("Monday \n"); } else if (day == 3) { printf("Tuesday \n"); } else if (day == 4) { printf("Wednesday \n"); } else if (day == 5) { printf("Thursday \n"); } else if (day == 6) { printf("Friday \n"); } else if (day == 7) { printf("Saturday \n"); } else { // Invalid Input, Display error printf("ERROR: Invalid Input, Please provide valid input \n"); } return 0; } |
Program Output:
We are using the GCC Compiler to compile and run the program. By GCC compiler names the executable file as the a.out. ( As I am not renaming the executable file during the compilation, we also get the executable file as a.out)
You can learn more about the C program compilation on Linux in the following article
Example 1:
1 2 3 4 5 |
$ gcc weekday.c $ ./a.out Enter day Number [1-7]: 4 Wednesday $ |
Example 2:
1 2 3 4 |
$ ./a.out Enter day Number [1-7]: 1 Sunday $ |
More Examples:
1 2 3 4 5 6 7 |
$ ./a.out Enter day Number [1-7]: 7 Saturday $ ./a.out Enter day Number [1-7]: 6 Friday $ |
Invalid Input Example:
1 2 3 4 5 6 7 |
$ ./a.out Enter day Number [1-7]: 9 ERROR: Invalid Input, Please provide valid input $ ./a.out Enter day Number [1-7]: -1 ERROR: Invalid Input, Please provide valid input $ |
As you can see if the user enters any other value other than the 1 to 7, The program will display the Error message.
Method 2: Day of Week Program using the Switch statement in C programming
The program logic is almost the same, Except in this case we are using the Switch case instead of the if else ladder.
📢 If the number of comparisons is increasing then, If else ladder can cause some confusion, So it is advised to use the Switch statement whenever you feel it appropriate.
Here is the Day of week program using the switch statement. This code looks more readable and easily maintainable.
We used the switch statement‘s Default Case to handle the Invalid Inputs.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
/* Program: Check the weekday based on week Number Author: www.SillyCodes.com */ #include<stdio.h> int main() { int day; // Take the input from the user // Number 1 - for Sunday // Number 2 - Monday,.. so on. printf("Enter day Number [1-7]: "); scanf("%d", &day); // We will use the switch statement/case // and compare the 'day' with each value. switch (day) { case 1: printf("Sunday \n"); break; case 2: printf("Monday \n"); break; case 3: printf("Tuesday \n"); break; case 4: printf("Wednesday \n"); break; case 5: printf("Thursday \n"); break; case 6: printf("Friday \n"); break; case 7: printf("Saturday \n"); break; default: // Invalid Input, Display error printf("ERROR: Invalid Input, Please provide valid input \n"); break; } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
$ gcc weekday.c $ ./a.out Enter day Number [1-7]: 5 Thursday $ ./a.out Enter day Number [1-7]: 2 Monday $ ./a.out Enter day Number [1-7]: 3 Tuesday $ ./a.out Enter day Number [1-7]: -5 ERROR: Invalid Input, Please provide valid input $ ./a.out Enter day Number [1-7]: 8 ERROR: Invalid Input, Please provide valid input $ ./a.out Enter day Number [1-7]: 1 Sunday $ ./a.out Enter day Number [1-7]: 7 Saturday $ |
As you can see we got the excepted results and also handles the Invalid Inputs.
Related Programs:
- Roots of Quadratic Equation Program
- C Program to calculate remainder and Quotient in C
- C Program to given year is Check Leap year or Not
- Minimum of three number
- C Program to check character is Alphabet or Number or Special Character
- 200 + C Programs
2 Responses
[…] C Program to check Day of week using Switch Case […]
[…] Enter the week number and print the day of week program Using Switch Statement […]