Return Multiple Values from a Function in C Language
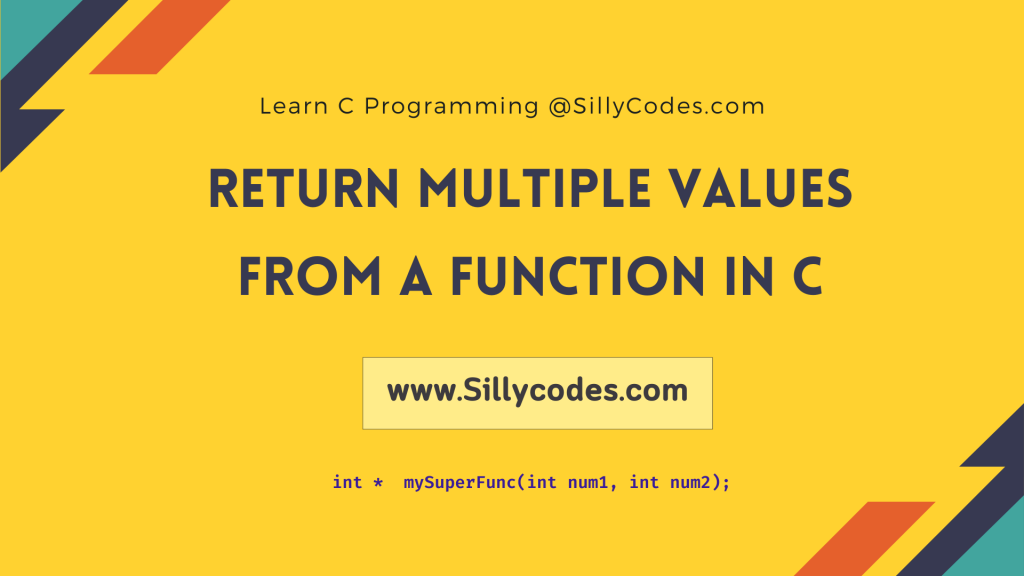
Return multiple values from a Function in C Program Description:
Write a Program to return multiple values from a function in c programming language. The program should define a function that updates/returns multiple values to the caller.
📢 This Program is part of the Pointers Practice Programs series
We are going to look at a couple of methods to return more than one value from a function. They are
- Passing variables as References to Function
- Returning an Array of values from a function.
Prerequisites:
It is recommended to go through the Pointers and Functions tutorials to better understand the program.
- Pointers in C Programming Language – Declare, Initialize, and use pointers
- Pointer Arithmetic in C Language
- Pointers and Arrays in C with Example Programs
- C Arrays – How to Create and use arrays
- Functions in C
Let’s look at the above two methods in detail.
Method 1: By Passing variables as References/Addresses to Function:
We can get multiple values from a function by passing the desired variables with a call by address/call by reference.
For example, You want to get multiple values like x and y from a function, Then pass the x and y with addresses to the function call. Use the address of operator(&) to pass the variables with addresses.
void myFunc(int var, int &x, int &y);
Also, make sure to define the function which accepts the above arguments as the pointers. In the above case, The myFun should accept three formal arguments and the last two formal arguments should be integer pointers. Here is the example prototype of the function.
void myFunc(int var, int * xPtr, int *yPtr);
Now, We can modify the variables x and y by modifying the xPtr and yPtr in the myFunc function. Use the dereference operator or Indirection operator to update the variables x and y.
Let’s look at an example to get multiple values from a function ( by passing variables as references)
Program to Get Multiple Values from a function by Passing Variables as Addresses:
In the following program, We have defined a function called performArithOps. The function performs all arithmetic operations like addition, subtraction, product, division, and modulo division on given two integers. Here is the prototype of the function.
void performArithOps(int num1, int num2, int *sumPtr, int *subPtr, int *productPtr, int *divisionPtr, int *moduloDivPtr)
The performArithOps function takes seven formal arguments. First two arguments are integer numbers ( num1 and num2), and the remaining five arguments are integer pointers( int *).
- sumPtr- sum pointer
- subPtr- subtraction pointer
- productPtr- product pointer
- divisionPtr- division pointer
- moduloDivPtr- modulo division pointer
This function calculates the sum of num1 and num2 and updates the sumPtr by using the indirection operator.
*sumPtr = num1 + num2;
Similarly, the function will perform the remaining arithmetic operations and updates the respective pointer variable.
Inside the main() function, Take two integer numbers from the user and store it in num1 and num2. Initialize five variables to hold the results( int sum=0, sub=0, product=0, division=0, moduloDiv=0;).
Call the performArithOps() function and pass the numbers( num1, num2) as values and result variables as addresses(use the address of operator ‘&’ ).
1 |
performArithOps(num1, num2, &sum, &sub, &product, &division, &moduloDiv); |
The performArithOps() function will update the sum, sub, product, division, and moduloDiv variables. As we passed them as the reference any changes made in the function will be reflected in the main() function.
Finally, display the results on the console.
Here is the program to return multiple values from a function in c language by passing variables with addresses.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
/* program to return morethan one value from function in C sillycodes.com */ #include<stdio.h> /** * @brief - Perform arithmetic operations * * @param num1 - number 1 * @param num2 - number 2 * @param sumPtr - sum pointer * @param subPtr - subtraction pointer * @param productPtr - product pointer * @param divisionPtr - division pointer * @param moduloDivPtr - modulo division pointer */ void performArithOps(int num1, int num2, int *sumPtr, int *subPtr, int *productPtr, int *divisionPtr, int *moduloDivPtr) { // dereference and update values // sum *sumPtr = num1 + num2; // sub *subPtr = num1 - num2; // product *productPtr = num1 * num2; // division *divisionPtr = num1 / num2; // modulo division *moduloDivPtr = num1 % num2; } int main() { // declare two variables int num1, num2; // intiailize variables to hold results. int sum=0, sub=0, product=0, division=0, moduloDiv=0; // get the numbers from user printf("Enter two integer numbers: "); scanf("%d%d", &num1, &num2); // call the 'performArithOps' function performArithOps(num1, num2, &sum, &sub, &product, &division, &moduloDiv); // print the result printf("Addition is %d+%d = %d\n", num1, num2, sum); printf("Substraction is %d-%d = %d\n", num1, num2, sub); printf("Multiplication is %d*%d = %d\n", num1, num2, product); printf("Division is %d/%d = %d\n", num1, num2, division); printf("Modulo Division is %d%%%d = %d\n", num1, num2, moduloDiv); return 0; } |
Program Output:
Compile and Run the Program using GCC compiler.
$ gcc return-multiple-values.c
Run the program.
1 2 3 4 5 6 7 8 |
$ ./a.out Enter two integer numbers: 200 100 Addition is 200+100 = 300 Substraction is 200-100 = 100 Multiplication is 200*100 = 20000 Division is 200/100 = 2 Modulo Division is 200%100 = 0 $ |
Let’s look at another example.
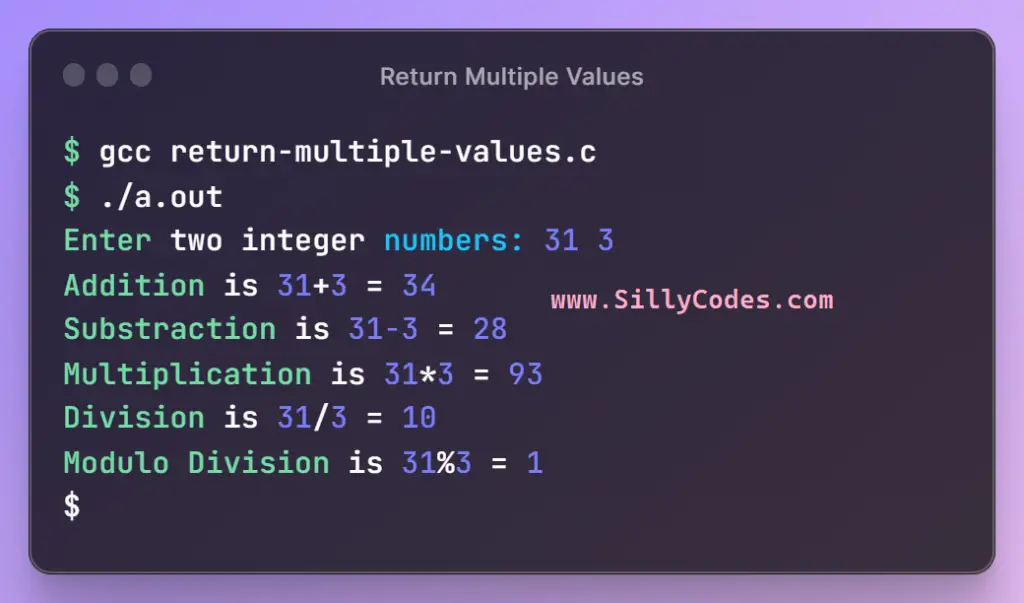
As we can see from the above output, The program is able to calculate the arithmetic operations properly. The performArithOps performed the arithmetic operations and updated the resultant variables.
Method 2: Return an Array of Values to get multiple values from a Function:
In this method, We are going to return an array of values from a function instead of passing the list of variables as references.
Let’s modify the above program and return an array with Five elements from the function to the caller.
Here is the program to return multiple values from a function in c language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
/* Program to return mutliple values from function in c www.sillycodes.com */ #include <stdio.h> #include <time.h> #include <stdlib.h> // Max Size of Array #define MAXSIZE 5 /** * @brief - perform arithemtic operations and returns an array * * @param num1 - number 1 * @param num2 - number 2 * @return int* - Array with results */ int * calcArithmeticOps(int num1, int num2) { // create a static Array with 'MAXSIZE' // static as we need 'results' to stay alive after this function returned. static int results[MAXSIZE]; int i = 0; // update 'sum' at index '0' and increment 'i' results[i++] = num1 + num2; // update 'sub' results[i++] = num1 - num2; // product results[i++] = num1 * num2; // division results[i++] = num1 / num2; // modulo division results[i++] = num1 % num2; // return the array return results; } int main() { // declare two variables int num1, num2; // get the numbers from user printf("Enter two integer numbers: "); scanf("%d%d", &num1, &num2); // create a pointer variable to hold results array int *results; // call the 'generateRandomArray()' function results = calcArithmeticOps(num1, num2); printf("Results after performing arthimetic operations on %d and %d are(sum, sub, product, div, mod): \n", num1, num2); // print the results. Note size is '5' for(int i=0; i<MAXSIZE; i++) { printf("%d\n", results[i]); } return 0; } |
In the above program, We have defined a function called calcArithmeticOps(). This function takes two integers as formal arguments and returns an integer pointer(array) as a return value. Here is the prototype of the function.
int * calcArithmeticOps(int num1, int num2)
Create an array called results with MAXSIZE. The MAXSIZE is 5 as we are performing five arithmetic operations. First element of the results array( results[0]) will hold the result of the addition operation, the second element( results[1]) holds the results of the subtraction operation, and so on so. The final element of the array results[4] will store the results of the modulo-division operation.
Because we are returning an array, we must ensure that the array is accessible after the calcArithmeticOps() function returns. To accomplish this, we must use the static array.
The default storage class for local variables is automatic, and they will be destroyed once the function returns. Because we need our results array ( results) to be available even after the calcArithmeticOps() function returns, So we must use a static integer array that will be alive throughout the program’s life.
Finally, Call the calcArithmeticOps from main() function and store the return value in the results pointer and display the results using a for loop.
1 |
results = calcArithmeticOps(num1, num2); |
Program Output:
Compile and Run the program and observe the output.
1 2 3 4 5 6 7 8 9 10 |
$ gcc return-multiple-values-with-pointer.c $ ./a.out Enter two integer numbers: 40 15 Results after performing arthimetic operations on 40 and 15 are(sum, sub, product, div, mod): 55 25 600 2 10 $ |
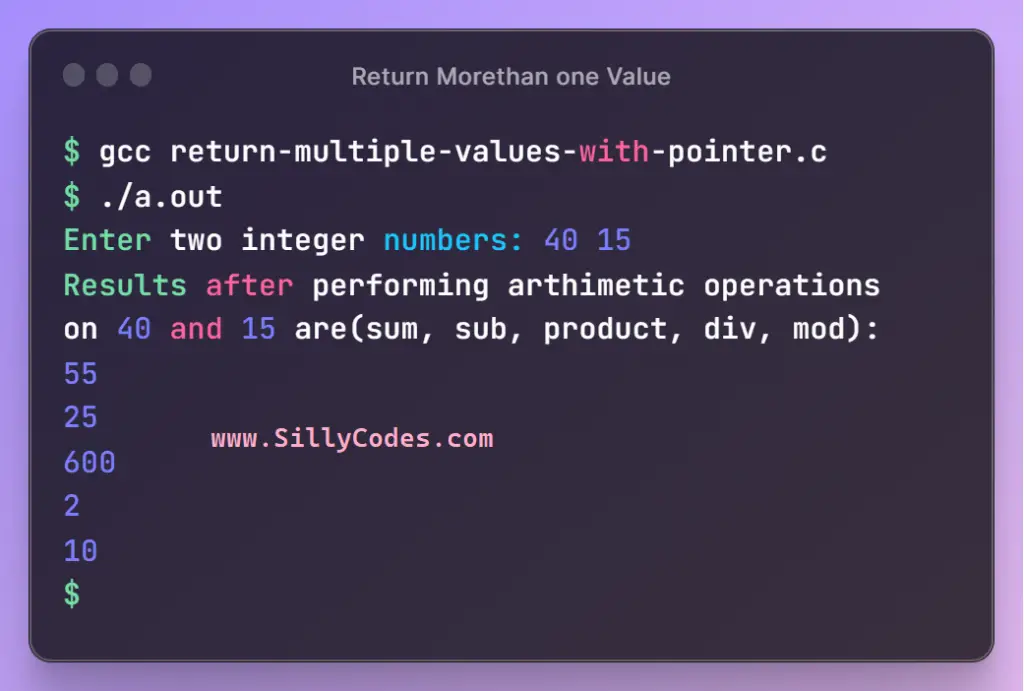
As we can see from the above output, The calcArithmeticOps() function properly calculated the arithmetic operations and populated the resultsarray.
Related Programs:
- C Tutorials Index
- C Programs Index – 300+ Programs
- Add Two Numbers using Pointers in C Language
- Program to perform Arithmetic Operations using Pointers in C
- Swap Two Numbers using Pointers in C Language
- Pointer Arithmetic in C Language
- Pointer to Pointer in C – Double Pointer in C
- Pointers and Arrays in C Language with Example Programs
- Accessing Array Elements using Pointers in C
- Print String Elements using Pointers in C Language