The strcmp in C with example programs
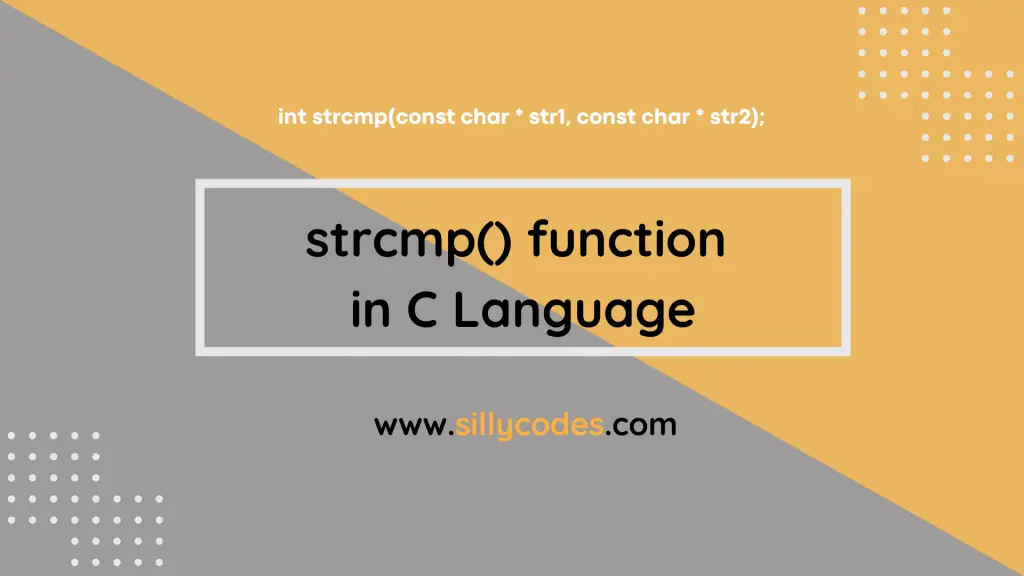
Introduction:
We covered the strlen, strcpy, and strcat library functions in earlier posts. In today’s article, We will look at the strcmp in C Programming language with example programs.
Before going any further, Here are the prerequisites for this article.
The strcmp in C Library Function:
The strcmp() function is used to compare the two strings in the C Programming language. strcmp() function is declared in the string.h header file.
strcmp() function takes two strings as input and compares the given strings in lexicographically. The lexicographically means the alphabetical order or dictionary order.
📢 This Program is part of the C String Practice Programs series
Let’s look at the syntax of the strcmp function.
strcmp Function Syntax:
1 |
int strcmp(const char * str1, const char * str2); |
As we can see from the above function prototype, The strcmp() function takes two strings and compare them using the ASCII Values of the characters of string.
strcmp Function Parameters
The strcmp() function must be called with the two parameters i.e Two strings. They are str1 and str2. The order of the string will affect the return type. This function compares the str1 and str2 and returns the results.
The strcmp function compares the strings by using the ASCII values of the each character. So to better understand the strcmp results, We recommend to check the ASCII values of the characters.
strcmp Return Value:
Return value of the strcmp() function is Integer ( int).
The strcmp() function returns
- Zero(0) – If both the string pointed by the str1 and str2 are equal.
- Negative Value – If the str1 is lexicographically smaller than the str2.
- Positive Value – If the str1 is lexicographically greater than the str2.
Let’s look at an example program to understand the strcmp function. We will explore all above return values in the following example.
Program to Compare two strings using strcmp in C Language:
Here is a program to demonstrate the strcmp string library function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/*     program to understand the strcmp function in c     sillycodes.com */  #include<stdio.h> #include<string.h>    // for 'strcmp' function  const int SIZE = 100;  int main() {      // declare two string with 'SIZE'     char str1[SIZE], str2[SIZE];      // get the two strings from the user     printf("Enter the first string : ");     scanf("%s", str1);     printf("Enter the second string : ");     scanf("%s", str2);      // compare two strings using `strcmp` function.     int result = strcmp(str1, str2);      // Print the results     if(result == 0)     {         printf("str1:%s and str2:%s are Equal\n", str1, str2);     }     else if (result < 0)     {         printf("str1:%s is lexicographically smaller than the str2:%s\n", str1, str2);     }     else     {         printf("str1:%s is lexicographically greater than the str2:%s\n", str1, str2);     }      return 0; } |
Don’t forget to include the string.h header file.
strcmp in C Program Explanation:
In the above program, We created two string str1 and str2 with the max size as the SIZE ( which is 100, Change this if you want to use large strings). Then we took the user input for the two strings str1 and str2 using the scanf function.
Once we got the two strings, We called the strcmp(str1, str2) function with the two input strings ( str1 and str2) to compare the strings.
int result = strcmp(str1, str2);
The strcmp() function compares the strings based on the ASCII Values. So we will get the result as Zero if both str1 and str2 are equal. If both strings are not equal then we will get the non-zero value. Print the results based on the result (i.e return value of the strcmp function).
1 2 3 4 5 6 7 8 9 10 11 12 |
    if(result == 0)     {         printf("str1:%s and str2:%s are Equal\n", str1, str2);     }     else if (result < 0)     {         printf("str1:%s is lexicographically smaller than the str2:%s\n", str1, str2);     }     else     {         printf("str1:%s is lexicographically greater than the str2:%s\n", str1, str2);     } |
Let’s compile and run the program and observe the output.
Program Output:
Compile the program.
$ gcc strcmp-func.c
above command generates an executable file named a.out or .exe based your operating system.
Run the executable file.
Test Case 1: When both strings are equal:
1 2 3 4 5 |
$ ./a.out Enter the first string : Roger Enter the second string : Roger str1:Roger and str2:Roger are Equal $ |
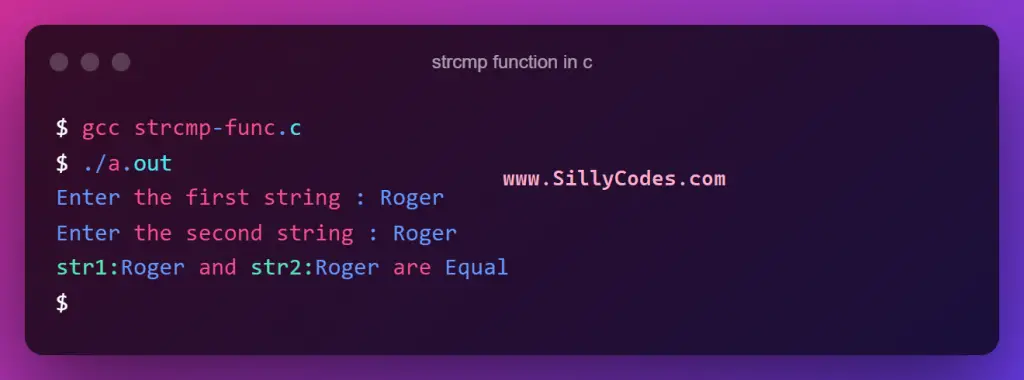
In the above example, The first string (i.e str1) and the Second string (i.e str2) are Roger. So they are equal. The strcmp() function compares the strings element by element using the ASCII Values of the characters. So as the all characters are same, We got the result as Zero(0).
Also note that, The two strings also have the same case characters, As C is case sensitive language, The case mismatch will also result in to the un-equal strings.
Test Case 2: When the str1 is smaller than the str2:
1 2 3 4 5 |
$ ./a.out Enter the first string : Rafa Enter the second string : Roger str1:Rafa is lexicographically smaller than the str2:Roger $ |
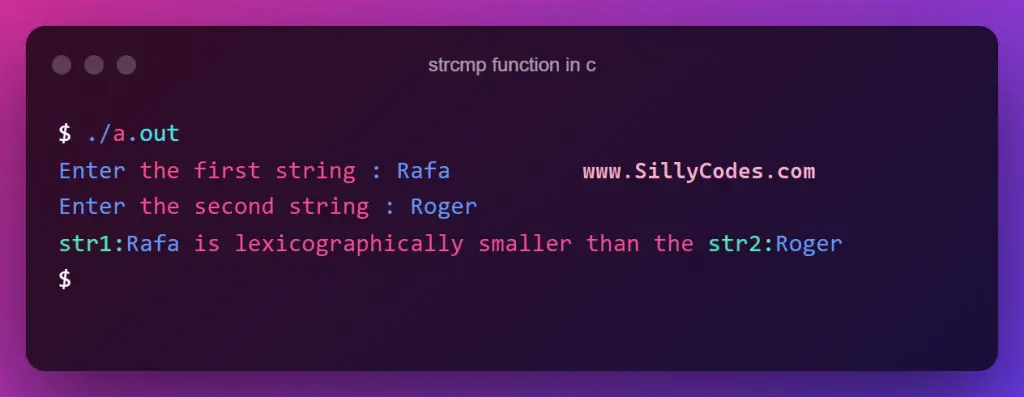
In this example, The first string Rafa is smaller than the Roger.
- If you observe the first character R is equal in both strings,
- But the second character a ( Rafa) is smaller than the character o (Roger).
- So the result will be difference between the ASCII Characters a - o, which is 97 - 111 equal to -14 (i.e a Negative number)
Test Case 3: When the str1 is greater than the str2:
1 2 3 4 5 |
$ ./a.out Enter the first string : Max Enter the second string : Lewis str1:Max is lexicographically greater than the str2:Lewis $ |
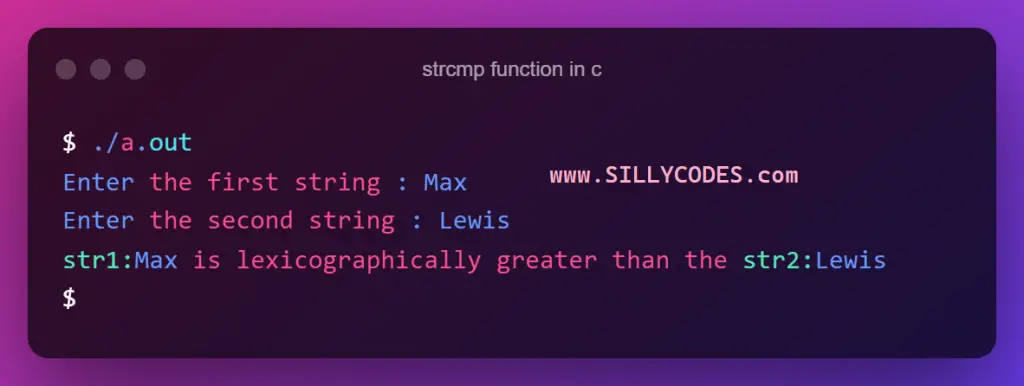
As we can see from the above output, The M(Max) is grether than the L(Lewis), So the first string str1(Max) is lexicographically greater than the second string str2(Lewis)
3 Responses
[…] have covered the strlen, strchr, strcpy, strcat, and strcmp library functions in our earlier posts. Today’s post will look at the strstr() in C programming […]
[…] strcmp library function in C […]
[…] are using the strcmp library function to compare the strings. Call the strcmp function with two strings firstStr and […]