strstr() in C Language – String library Function
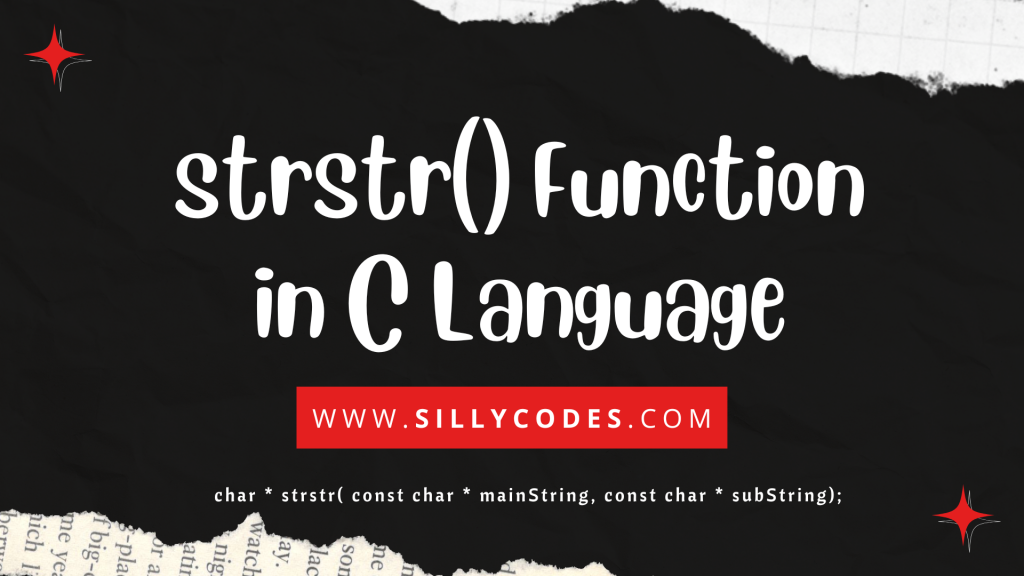
Introduction:
We have covered the strlen, strchr, strcpy, strcat, and strcmp library functions in our earlier posts. Today’s post will look at the strstr() in C programming language.
Prerequisites:
In order to better understand the article, Please go through the following tutorials regarding the Strings in C programming.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
The strstr() Function in C:
The strstr() function is used to search a substring in a string. So if we want to search for a string in another string, we can use the strstr() function.
The strstr() functiron searches for the substring in the main string and returns the pointer to the first occurrence of the substring.
Syntax of strstr() function in C Language:
Here is the syntax of the strstr() in c language:
1 |
char * strstr( const char * mainString, const char * subString); |
The strstr() function searched for the subString in the mainString.
Parameters:
The strstr() function takes two strings as the parameter.
- mainString – The main string where the search happens.
- substring – String to be searched in the mainString.
Return type:
The return type of the strstr() function is char *.
If the substring is found in the mainString, Then the strstr() function returns the character pointer pointing to the first occurrence of the substring in the mainString.
If the substring is not found in the mainString, Then strstr() function returns NULL.
📢 This Program is part of the C String Practice Programs series
Let’s look at an example program about strstr() function.
Program to search for a sub-string in a string using the strstr() function in C Language:
Here is the program to search for a sub string in a string using strstr() function in C.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
/* program to understand the strstr function in c sillycodes.com */ #include<stdio.h> #include<string.h> // for 'strstr' function const int SIZE = 100; int main() { // declare two strings with 'SIZE' char mainStr[SIZE], subStr[SIZE]; char *res; // get the string from the user printf("Enter the main string : "); // scanf("%s", mainStr); gets(mainStr); printf("Enter the string to Search(subString): "); // scanf("%s", subStr); gets(subStr); // search for the 'subStr' in 'mainStr' string res = strstr(mainStr, subStr); // display the results if(res == NULL) { printf("NOT FOUND: subString:%s is not found mainString:%s \n", subStr, mainStr); } else { printf("FOUND: subString:%s is found mainString:%s \n", subStr, mainStr); } return 0; } |
⚠️We need to include the string.h header file to use the strstr() function.
Program Explanation:
Here is the step-by-step explanation of the finding a substring program
- Declare two strings
mainStr and
subStr.
- The mainStr is the main string, This is string where we are going to search for the subStr.
- The subStr is the sub string. String to be searched in the mainStr
- Take the user input for the mainStr and subStr strings. We are using the gets() function. but gets() function won’t do the boundary checks, so use it with causion. Please look at the Different Ways to Read and Print strings in C programming to learn about other ways to read the strings.
- Now we got the Main string ( mainStr) and the Sub string ( subStr). To search for the string subStr in the mainStr string we use the strstr() function.
- Call the strstr() function and pass the mainStr as first argument and the subStr as the second argument. The strstr() function returns the pointer to the first occurrence subStr string in the Main string( mainStr). – res = strstr(mainStr, subStr);
- If the subStr is found in the mainStr, Then res will contain some address (Won’t be NULL). So print the “FOUND” message on console.
- If the subStr is not found in the mainStr, Then the return value res will contain the NULL. So display the “NOT FOUND” message on the console
Program Output:
let’s compile and RUn the program using the GCC compiler ( Any compiler)
Test Case 1: When the substring is found in the main string.
1 2 3 4 5 6 |
$ gcc strstr-func.c $ ./a.out Enter the main string : Who likes formula One? Enter the string to Search(subString): formula FOUND: subString:formula is found mainString:Who likes formula One? $ |
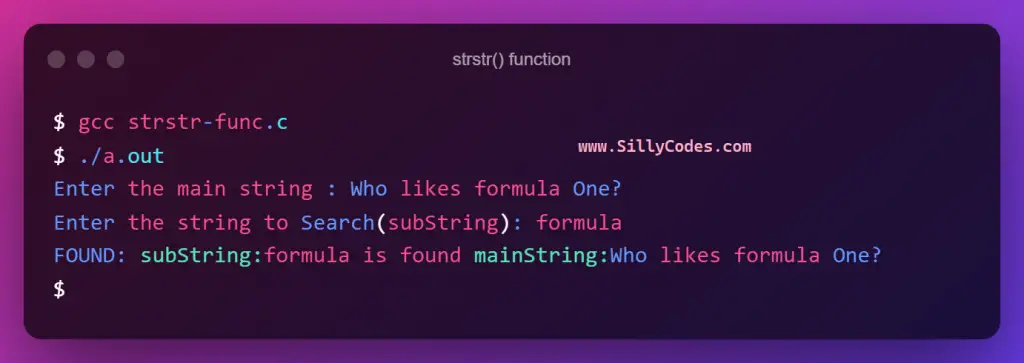
In the above example, The main string is Who likes formula One? and the sub string is formula. As the sub string formula is found in the main string. The strstr() function returned the valid address pointing to the first occurrence of the formula in the main string.
Test 2: When the sub string is not found in the Main string
1 2 3 4 5 |
$ ./a.out Enter the main string : Howdy, Welcome to Sillycodes.com Enter the string to Search(subString): Hello NOT FOUND: subString:Hello is not found mainString:Howdy, Welcome to Sillycodes.com $ |
In the above example, The substring Hello is not found in the main string Howdy, Welcome to Sillycodes.com. So the strstr() function returned the NULL as the return value.
Program to understand the return value of the strstr() function:
Let’s modify the above program to print the result if we find the substring in the main string. This will prove us that the strstr function is returning the address of first occurrence of the sub string in the main string.
Her is the rewritten version of the above program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/* program to understand the strstr function in c sillycodes.com */ #include<stdio.h> #include<string.h> // for 'strstr' function const int SIZE = 100; int main() { // declare two strings with 'SIZE' char mainStr[SIZE], subStr[SIZE]; char *res; // get the string from the user printf("Enter the main string : "); // scanf("%s", mainStr); gets(mainStr); printf("Enter the string to Search(subString): "); // scanf("%s", subStr); gets(subStr); // search for the 'subStr' in 'mainStr' string res = strstr(mainStr, subStr); // display the results if(res == NULL) { printf("NOT FOUND: subString:%s is not found mainString:%s \n", subStr, mainStr); } else { printf("FOUND: subString:%s is found mainString:%s \n", subStr, mainStr); printf("Result is : %s\n", res); } return 0; } |
Program Output:
Compile and Run the program
1 2 3 4 5 6 |
$ ./a.out Enter the main string : One Two One Two One Two Enter the string to Search(subString): Two FOUND: subString:Two is found mainString:One Two One Two One Two Result is : Two One Two One Two $ |
The main string is One Two One Two One Two and the substring is Two.
The strstr() function found the first occurrence of the substring( Two) in the main string and returned the character pointer pointing to it (i.e res pointer)
If we print the resultant pointer, We will get Two One Two One Two as the string. Which confirms us the strstr() function returned the first occurrence of the Two substring.
1 Response
[…] strstr library function in C […]