Program to find min and max using functions in c language
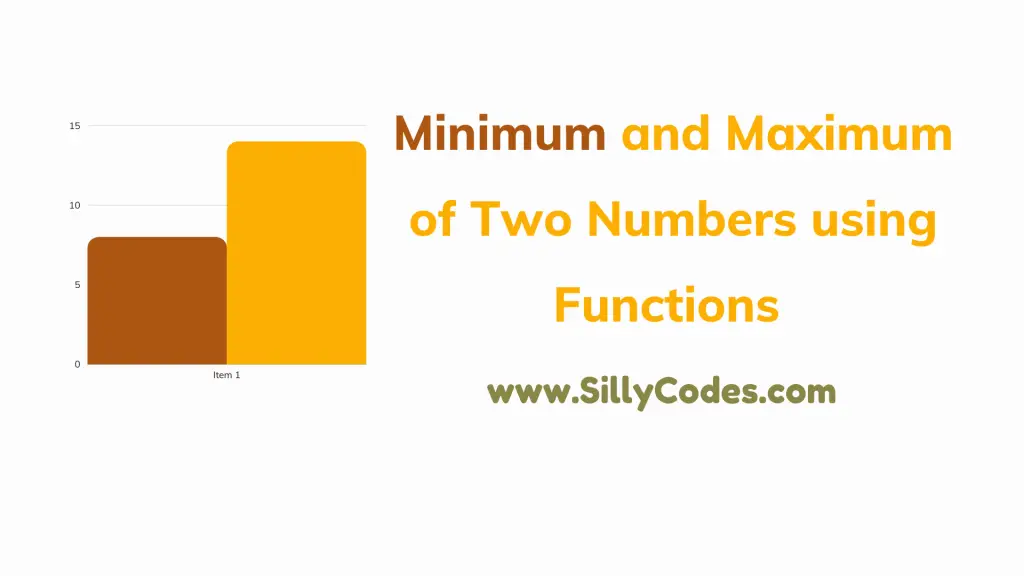
Min and Max using functions in C Program Description:
Write a program to find the min and max using functions in C language. We are going to write two programs, One for calculating the minimum and another for calculating the maximum.
Pre-Requisites:
Find Min using a function in C:
Input:
Enter two numbers: 92 31
Output:
The minimum of 92 and 31 is : 31
Find the Minimum using a function in C language Algorithm:
- Take two numbers from the user and store them in variables num1 and num2 respectively.
- We defined a function called
min, The
min function accepts two integer numbers as input and calculates the minimum of the given numbers. Here is the prototype of the
min function
- int min(int num1, int num2);
- function_name: min
- arguments_list : (int, int)
- return_type: int
- The min function uses the conditional variable to calculate the minimum of num1 and num2(i.e (num1 > num2) ? num2 : num1 ) and returns a minimum value.
- Call the min function passing input numbers num1 and num2. and store the return value in variable minimum
- Print the results on the console.
Program to Find the Minimum using a function in C language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
/*     Program: Find Minimum of two numbers in c using function     Author: SillyCodes.com */  #include<stdio.h>  /*     Description: 'min' function finds min of 'num1' and 'num2'     arguments_list: (int, int)     return_type: (int) */ int min(int num1, int num2) {     // using the conditional operator to calcualte the min     return (num1 > num2) ? num2 : num1; }  int main() {     int num1, num2, minimum;      // Take user input     printf("Enter two numbers: ");     scanf("%d%d", &num1, &num2);      // call the 'min' function and store the result in 'minimum' variable     minimum =  min(num1, num2);      // print result     printf("The minimum of %d and %d is : %d\n", num1, num2, minimum);      return 0; } |
Minimum Program output:
Save the above program with .c extension. Compile and run the program.
1 2 3 4 5 |
$ gcc min-function.c $ ./a.out Enter two numbers: 92 31 The minimum of 92 and 31 is : 31 $ |
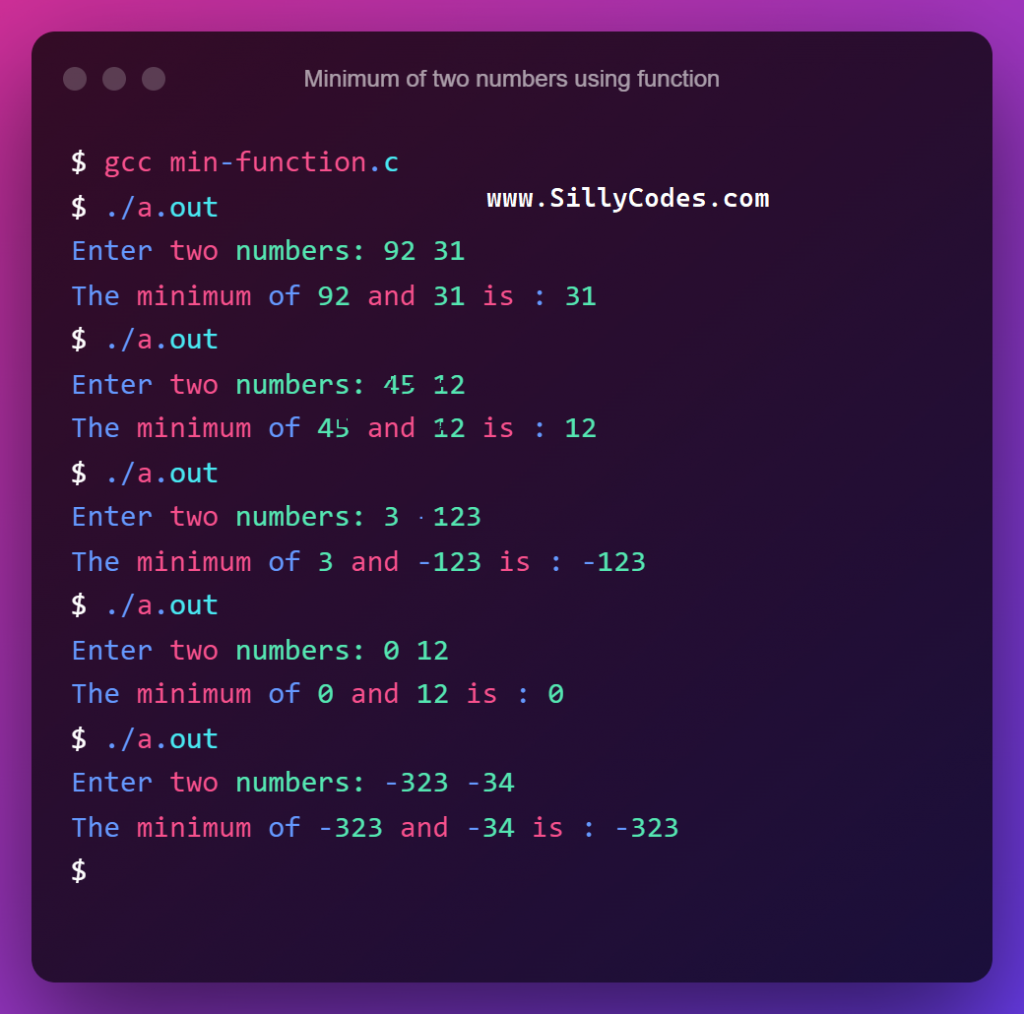
Let’s try a few more examples.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter two numbers: 45 12 The minimum of 45 and 12 is : 12 $ ./a.out Enter two numbers: 3 -123 The minimum of 3 and -123 is : -123 $ ./a.out Enter two numbers: 0 12 The minimum of 0 and 12 is : 0 $ ./a.out Enter two numbers: -323 -34 The minimum of -323 and -34 is : -323 $ |
Find a Maximum of Two numbers using a function in C:
Example Input:
Enter two numbers: 4 2
Output:
The maximum of 4 and 2 is : 4
Find a Max of two numbers using a function Program algorithm:
- Accept two integers from the user and store them in variables num1 and num2 respectively.
- We have created a function called
max, The
max function accepts two integer numbers as input and calculates the maximum of the given numbers. Here is the prototype of the
max function
- int max(int num1, int num2);
- function_name: min
- arguments_list : (int, int)
- return_type: int
- The max function uses the conditional variable to calculate the maximum of num1 and num2 (i.e (num1 > num2) ? num2 : num1 ) and returns a maximum value.
- Call the max function from the main function and pass two variables num1 and num2. Store the return value in maximum variable.
- We got the max value in maximum variable, Print the result on the console.
Program to find max of Two numbers using function in C:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
/*     Program: Find Maximum of two numbers in c using function     Author: SillyCodes.com */  #include<stdio.h>  /*     Description: 'max' function finds maximum of 'num1' and 'num2'     arguments_list: (int, int)     return_type: (int) */ int max(int num1, int num2) {     // using the conditional operator to calcualte the max     return (num1 > num2) ? num1 : num2; }  int main() {     int num1, num2, maximum;      // Take user input     printf("Enter two numbers: ");     scanf("%d%d", &num1, &num2);      // call the 'max' function and store the result in 'maximum' variable     maximum =  max(num1, num2);      // print result     printf("The maximum of %d and %d is : %d\n", num1, num2, maximum);      return 0; } |
Program Output:
Compile and Run the program.
$ gcc max-function.c
The above gcc command, Generates the a.out executable file. Run the executable using ./a.out command.
1 2 3 4 |
$ ./a.out Enter two numbers: 44 77 The maximum of 44 and 77 is : 77 $ |
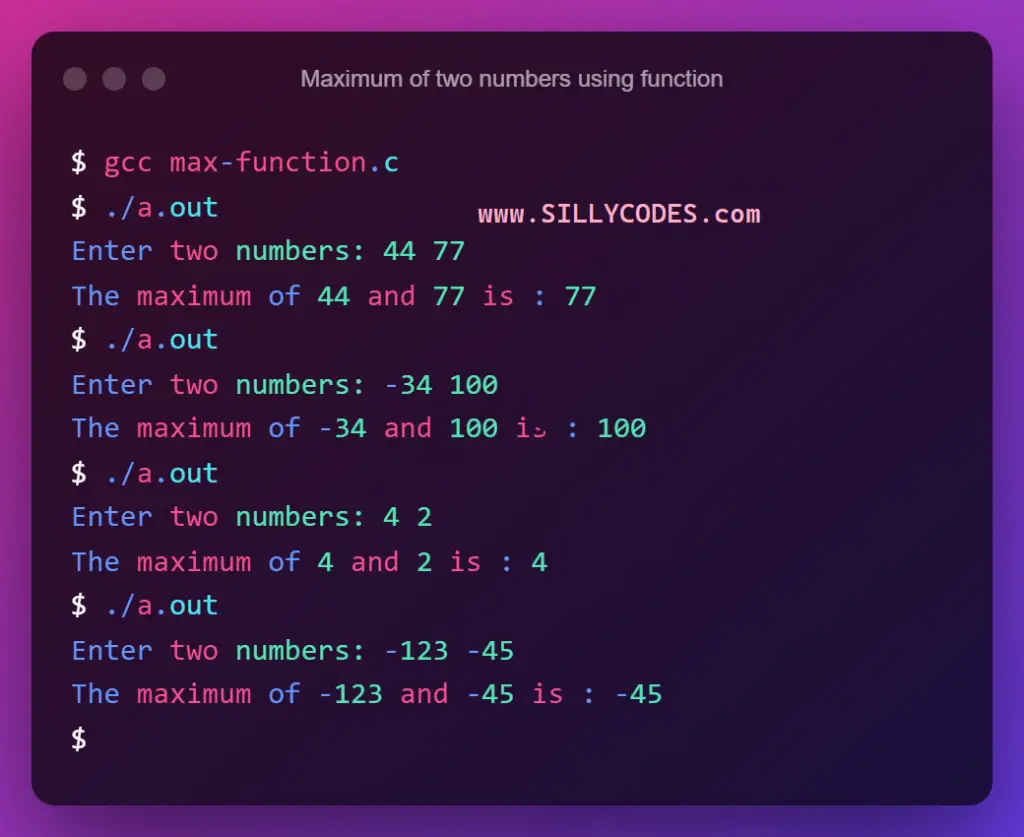
Let’s try a few more examples.
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter two numbers: -34 100 The maximum of -34 and 100 is : 100 $ ./a.out Enter two numbers: 4 2 The maximum of 4 and 2 is : 4 $ ./a.out Enter two numbers: -123 -45 The maximum of -123 and -45 is : -45 $ |
As expected, We are getting the results correctly.
Related Programs:
- All C Programs Index [List]
- C Program to Calculate Sum of Digits of a Number
- C Program to Calculate Product of Digits of a Number
- C Program to Count the number of Digits of a Number
- C Program to Multiply without using Multiplication operator (*)
- C Program to generate Fibonacci Series up to a given number
- C Program to Print First n Fibonacci numbers
- C Program to get Nth Fibonacci number