Factorial using Recursion in C Language
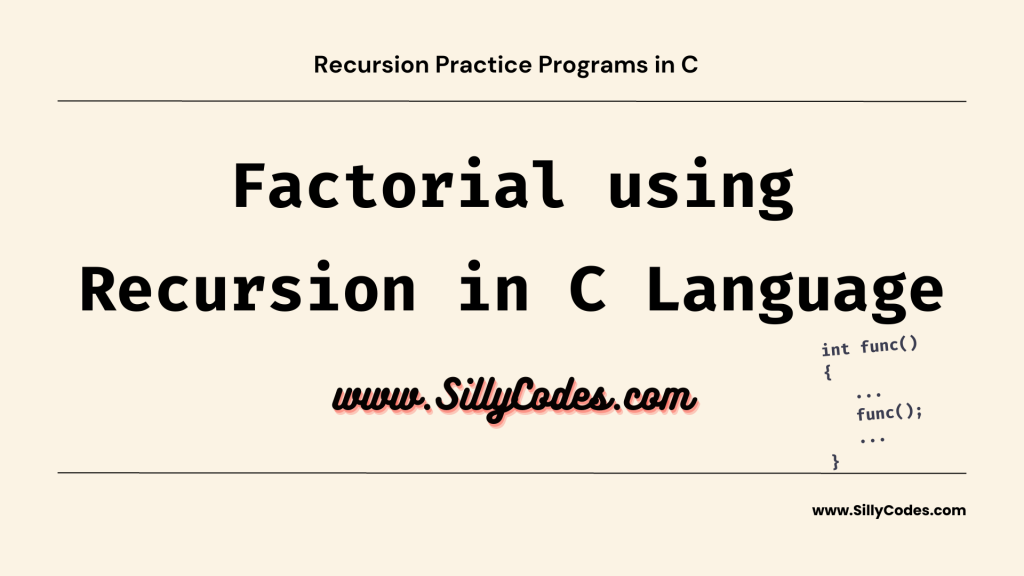
Factorial using recursion in C Program Description:
Write a Program to calculate the Factorial using recursion in C programming language. The program will accept a number from the user and calculate the factorial using recursion (shouldn’t use the loops)
Example Input and Output:
Input:
Enter a Number to calculate factorial : 5
Output:
Factorial of 5 is : 120
Related Program: Calculate Factorial Program using iterative method ( Loops )
Pre-Requisites:
It is recommended to know the basics of the Functions in C language and how recursion works. Please go through the following articles to learn more about them.
Factorial using recursion in C Algorithm/Explanation:
- Start the program by accepting a number from the user and storing it in num variable.
- Don’t allow negative numbers, So check for negative numbers using num <= 0 condition and display an error message if the user enters a negative number.
- Create a function to calculate the factorial recursively named
fact function. The
fact function takes a number
num as input and calculates the factorial of
num recursively.
- The base condition for recursion is num == 1. Whenever the num becomes one, The fact function will return 1.
- If the num is not zero, Then we will make a recursive call to the fact function and pass the num-1 as the input. fact(num-1)
- Also, Multiply the result of fact(num-1) with num. – i.e num * fact(num-1)
- The above process continues until the value of num reaches the 1. (The base condition)
- Call the fact function from the main function and pass the num as the input value. The fact function calculates the factorial of num and returns it. Print the result on the console using printf function.
Factorial using Recursion in C Program:
Let’s write the program using above algorithm.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
/*     Program: Calculate Factorial using recursion in C */  #include<stdio.h>  // function declaraion int fact(int);  // main function int main() {     int num;      // Take user input     printf("Enter a Number to calculate factorial : ");     scanf("%d", &num);      // Negative Number check     if(num <= 0)     {         printf("Error: Invalid Input, Try again\n");         return 0;     }      // call the fact() function     printf("Factorial of %d is : %d \n", num, fact(num));      return 0; }  /*     arguments_list: int     return_type: int     Description: The fact function calculates factorial recursively. */ int fact(int num) {     // base condition     if(num == 1)         return 1;     else     {         // recursive call with 'num-1'         return ( num * fact(num-1));     } } |
Here we defined the fact function after calling it from the main function, So we have to specify the function declaration ( forward declaration of function).
int fact(int num);
Here are the prototype details of the factfunction.
- function_name: fact
- Arguments_list: (int)
- Return_Value: int
- Description: The fact function calculates the factorial of a given number recursively and returns the result.
Program Output:
We are using the GCC compiler to compile the program.
📢Related Tutorial: Compile and Run Programs Using GCC
$ gcc factorial-recursive.c
Run the program.
Test Case 1: Positive Numbers:
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a Number to calculate factorial : 5 Factorial of 5 is : 120 $ ./a.out Enter a Number to calculate factorial : 7 Factorial of 7 is : 5040 $ ./a.out Enter a Number to calculate factorial : 10 Factorial of 10 is : 3628800 $ |
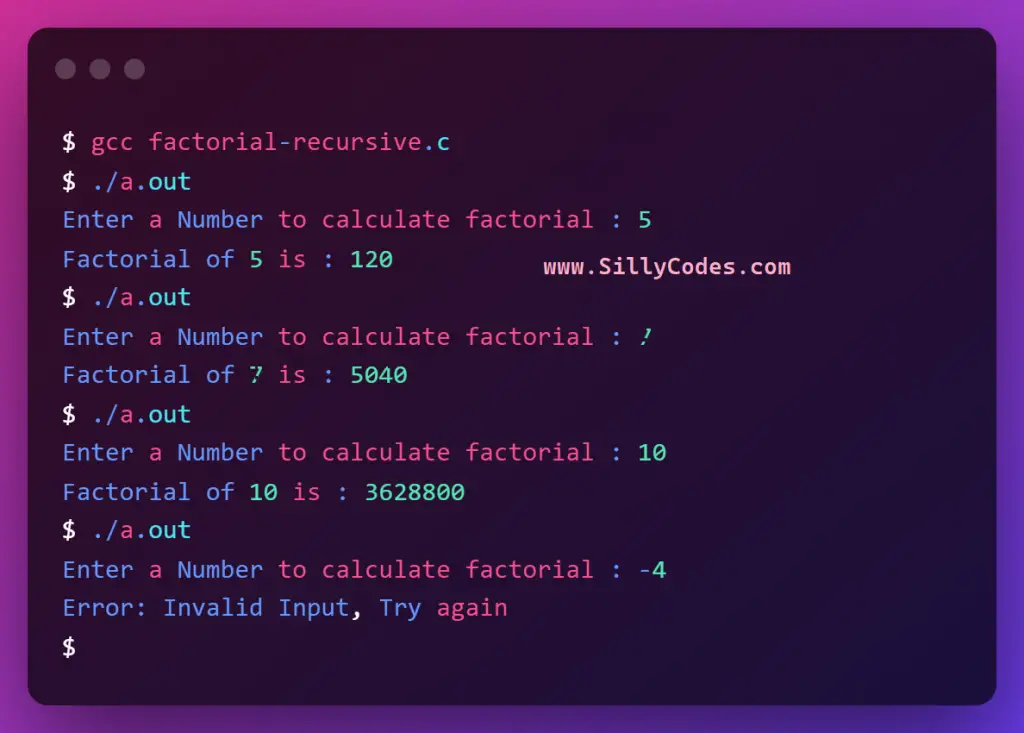
Test Case 2: Negative Numbers
1 2 3 4 |
$ ./a.out Enter a Number to calculate factorial : -4 Error: Invalid Input, Try again $ |
As we can see from the above output, The program is providing the valid output and displaying the error message Error: Invalid Input, Try again on a negative number.
Related Recursion Programs:
- Program to print first n natural numbers using recursion in C
- Calculate Sum of N Natural Numbers in C using Recursion
- Fibonacci Number in C using Recursion
Related Functions Practice Programs:
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
- 100+ C Practice Programs
- C Tutorials Index
1 Response
[…] C Program to Calculate the Factorial of a Number using Recursion […]