Program to Toggle Case of Each Character of String in C Language
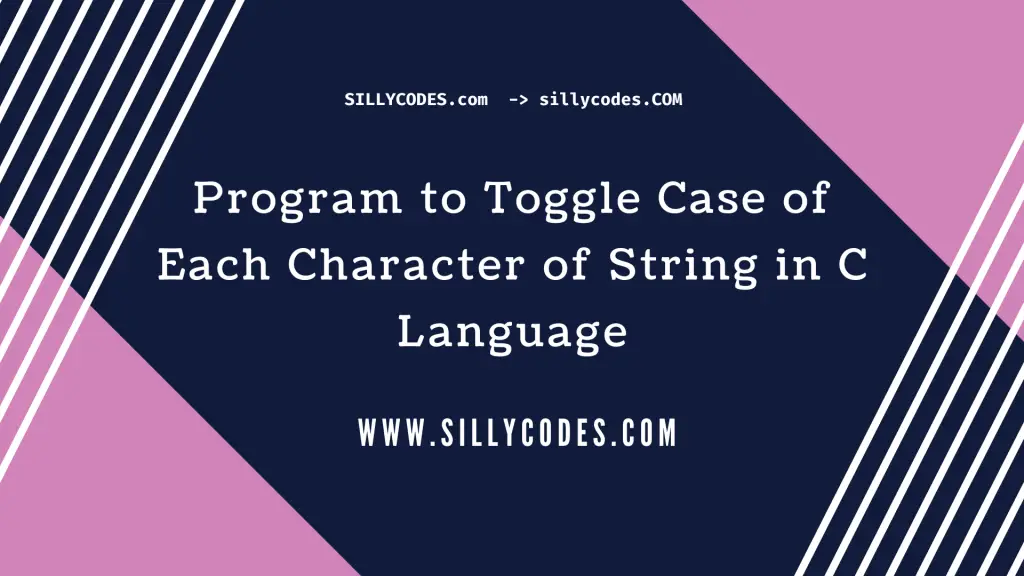
Program Description:
Write a Program to Toggle Case of Each character of String in C programming language. The program should accept a string from the user and Invert the case or Toggle the case of all characters in the string.
Excepted Program Input and Output:
Input:
Enter a string : May the Force be With YOU
Output:
String after toggling each character: mAY THE fORCE BE wITH you
If you observe the above input and output of the program, The case of all characters in the input string is inverted or toggled.
Prerequisites:
It is recommended to go through the following articles to learn more about the Strings.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
Algorithm to Toggle Case of Each Character of String in C:
We can toggle the character case by modifying the ASCII values of the string.
As we already discussed earlier ( Lowercase to Uppercase conversion, Uppercase to lowercase character conversion programs), characters in C language are stored in the memory with their ASCII Values.
For example, The character upper case A‘s ASCII Value is 65. Similarly lower case a‘s ASCII Value is 97.
You can check the list of ASCII Values by looking at the following post
To convert any Lowercase character to Upper Case character, We can subtract the value 32 from the ASCII number of the lowercase character.
For example,
- Lowercase ‘d’ – ASCII Value – 100.
- To convert to Upper case character – Subtract 32 from the lower case character. i.e 100 – 32 = 68
- The ASCII value of the Upper case ‘D’ is 68.
Similarly, To convert any Uppercase character to a Lowercase character, We can Add the number 32 to the ASCII number of the uppercase character.
For example,
- Uppercase ‘E’ – ASCII Value – 69.
- To convert Uppercase to a Lowercase character – Add 32 to the Uppercase character. i.e 69 + 32 = 101
- The ASCII value 101 is equal to the Lowercase character ‘e’
Toggle Case of Each Character of String in C Program Explanation:
Let’s look at the step-by-step explanation of the program.
- Start the program by declaring a string and naming it as inputStrwith the size SIZEelements. ( The SIZE is a constant with 100 value)
- Prompt the user to input a string and Update the inputStr with user-provided string.
- Now, To Toggle to the case of all characters, We will go through the all characters of the string using a For Loop, and based on the case of the string we either Add or Subtract the 32 from the character ASCII Value.
- Create a For loop to iterate over the string –
for( i = 0; inputStr[i]; i++)
- At Each Iteration, Check if the
inputStr[i] is a lowercase character use if(inputStr[i] >= ‘a’ && inputStr[i] <= ‘z’) condition.
- If the inputStr[i] is lower case character, Then Toggle it to upper case character by subtracting 32 from the ASCII value – inputStr[i] = inputStr[i] - 32;
- If the
inputStr[i] is an Upper case character(if(inputStr[i] >= ‘A’ && inputStr[i] <= ‘Z’)), Then Add the value
32 to it to make it a Lower case character.
- i.e inputStr[i] = inputStr[i] + 32;
- At Each Iteration, Check if the
inputStr[i] is a lowercase character use if(inputStr[i] >= ‘a’ && inputStr[i] <= ‘z’) condition.
- Once the above step 4 is completed, Then all the characters in the given string will be toggled.
- Print the resultant string on the console using printf function.
Program to Toggle Case of Each Character of String in C using Iterative Method:
Here is the program to toggle case of all characters in a string
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
/*     program to Toggle case of each character in a string.     sillycodes.com */  #include<stdio.h> #include<string.h>  // Max Size of string const int SIZE = 100;  int main() {     // declare a string with 'SIZE'     char inputStr[SIZE];     int i;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);      // Iterate over the string     // and Invert Each character of the string.     for( i = 0; inputStr[i]; i++)     {         // Check if the character is lower case         if(inputStr[i] >= 'a' && inputStr[i] <= 'z')         {             // Lower Case             // Ascii value of 'a' = 97             // Ascii value of 'A' = 65             // Subtract 32 (-32) to make it a Upper case character.             // Learn More - https://sillycodes.com/c-program-to-print-all-ascii-characters/             inputStr[i] = inputStr[i] - 32;         }         else if(inputStr[i] >= 'A' && inputStr[i] <= 'Z')         {             // Upper case character             // Add 32 (+32) to make it a Lower case character.             inputStr[i] = inputStr[i] + 32;         }     }      // Print the Resultant String.     printf("String after toggling each character: %s\n", inputStr);      return 0; } |
Program Output:
Let’s compile and run the program using GCC compiler (Any of your favorite compilers)
Test 1:
1 2 3 4 5 |
$ gcc toggle-string.c $ ./a.out Enter a string : May the Force be With YOU String after toggling each character: mAY THE fORCE BE wITH you $ |
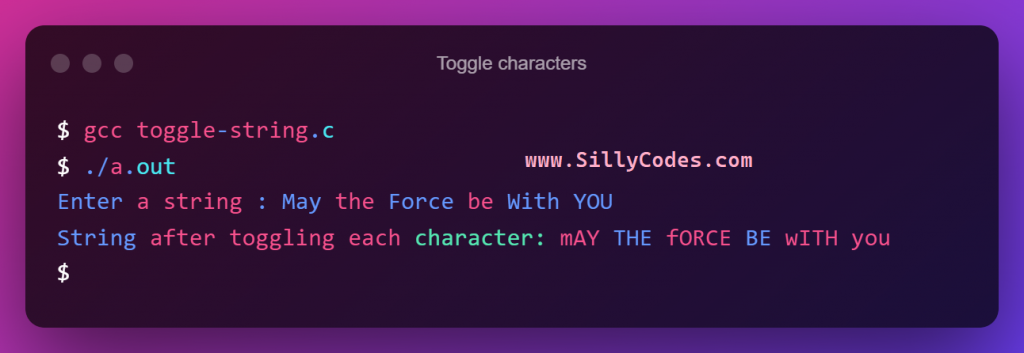
Let’s try another example
1 2 3 4 |
$ ./a.out Enter a string : Learn Programming at SillyCodes.com String after toggling each character: lEARN pROGRAMMING AT sILLYcODES.COM $ |
In the above example, The input string is Learn Programming at SillyCodes.com and the program toggled the all characters and provided the lEARN pROGRAMMING AT sILLYcODES.COM as output.
Test 2: When the input string contains numbers
1 2 3 4 |
$ ./a.out Enter a string : HELLO world 1234 String after toggling each character: hello WORLD 1234 $ |
As we are only changing the alphabets ( using the inputStr[i] >= ‘a’ && inputStr[i] <= ‘z’ conditions) other characters won’t be modified.
Toggle Case of Each Character of String in C using a user-defined function:
Let’s move the characters case toggle logic to a function, So that we can reuse the code. Here are few benefits of using the functions
In the following program, We defined a user defined function named toggleString to toggle all characters in the given string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 |
/*     program to Toggle case of each character in a string.     sillycodes.com */  #include<stdio.h>  // Max Size of string const int SIZE = 100;  /** * @brief  - Toggles or Inverts the each character in the string * * @param str - String to toggle the characters. */ void toggleString(char * str) {     int i;      // Iterate over the string     // and Invert Each character of the string.     for( i = 0; str[i]; i++)     {         // Check if the character is lower case         if(str[i] >= 'a' && str[i] <= 'z')         {             // Lower Case             // Subtract 32 (-32) to make it a Upper case character.             // Learn More - https://sillycodes.com/c-program-to-print-all-ascii-characters/             str[i] = str[i] - 32;         }         else if(str[i] >= 'A' && str[i] <= 'Z')         {             // Upper case character             // Add 32 (+32) to make it a Lower case character.             str[i] = str[i] + 32;         }     }  }  int main() {     // declare a string with 'SIZE'     char inputStr[SIZE];     int i;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);      // call 'toggelString' function with 'inputStr' to toggle all characters     toggleString(inputStr);      // Print the Resultant String.     printf("String after toggling each character: %s\n", inputStr);      return 0; } |
Here is the prototype details of the toggleString function.
void toggleString(char * str)
The toggleString function takes one formal argument named str and toggles or Inverts the case of all characters in the given string str. This function also modifies the ASCII values of the characters to convert them to upper case to lower case and vice versa.
Program Output:
Compile and Run the program.
1 2 3 4 5 6 7 8 9 |
$ gcc toggle-string.c $ ./a.out Enter a string : Howdy, How Are You? String after toggling each character: hOWDY, hOW aRE yOU? $ $ ./a.out Enter a string : SILLYCODES.com String after toggling each character: sillycodes.COM $ |
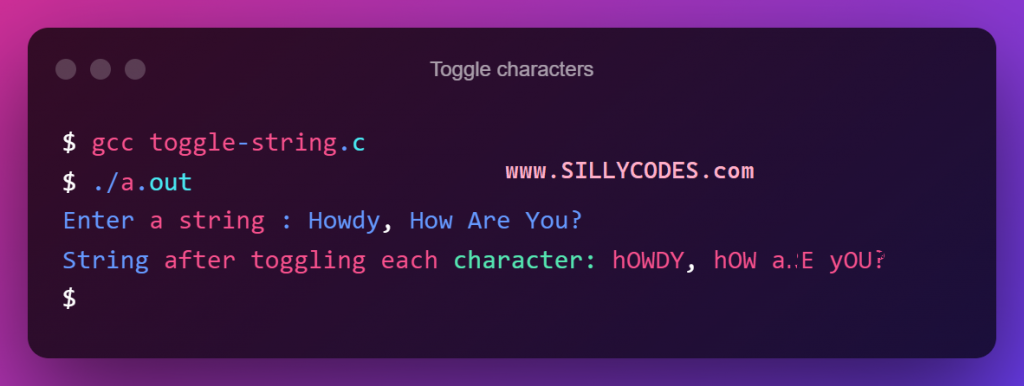
As we can see from the above output, The program is properly converting the character case.
Related String Practice Programs:
- C Tutorials Index
- C Programs Index
- C Program to Calculate Length of the string
- C Program to Copy a String to new string
- C Program to Concatenate Two strings
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
1 Response
[…] C Program to Toggle Case of All Characters in String […]