Nested if else and if else ladder in C
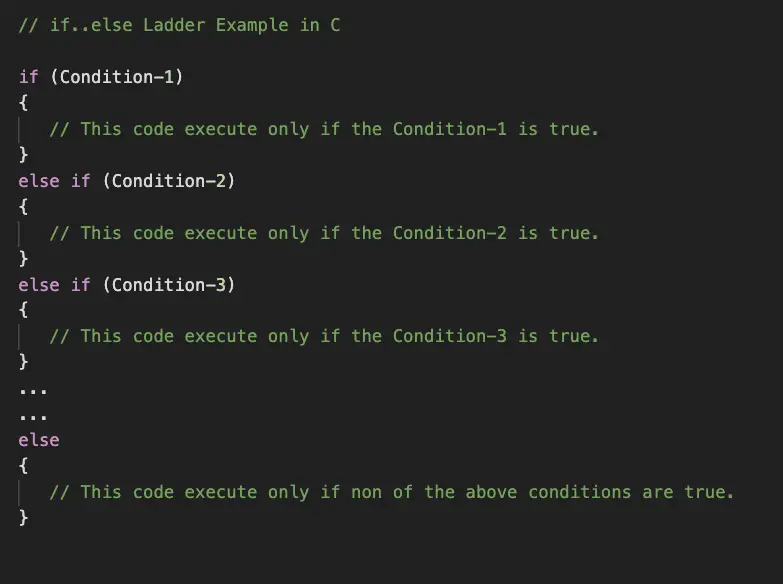
In our previous post, we discussed the if and if…else statements. Today we will discuss Nested if…else and if…else ladder.
Sometimes we need to use if statement inside the existing if statement.
Syntax of Nested if…else statement :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
if(condition1) { // This code execute only if the condition1 is true. // some other logic if (condition2) { // This codes executes only if the condition1 and condition2 is true // some code } } else { // If the condition1 is false we come here if(condition3) { // We can also have if here. // This will execute only if the condition3 is true. } } |
In the above code if statement with condition2 is nested if statement.
Example to demonstrate nested if…else :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
#include<stdio.h> int main(int argc, char const *argv[]) { int A,B,C; printf("Enter 3 number : "); scanf("%d%d%d", &A, &B, &C); // Control statement if if(A>B) { // A is bigger than B, So lets see if A is bigger than the C if(A>C) { // A is larger than the B and C. printf("Given number %d is the largest \n", A); } } else { // B is bigger than the A if(B>C) { // B is also bigger than the C, So B is largest among all printf("Number %d is the largest \n", B); } else { // B is smaller than the C, So C is the largest among all printf("Number %d is the largest \n", C); } } return 0; } |
if…else ladder :
if we have a chain of if and else blocks then we call it as if…else ladder. only one block among all if..else blocks executed and remaining all blocks will be ignored.
Syntax of if..else ladder
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
if (Condition-1) { // This code execute only if the Condition-1 is true. } else if (Condition-2) { // This code execute only if the Condition-2 is true. } else if (Condition-3) { // This code execute only if the Condition-3 is true. } ... ... else { // This code execute only if non of the above conditions are true. } |
📢 Only one of the all if and else..if and else blocks will be executed
If any of the conditions are true then that particular block of code will be executed if none of the conditions are true then final else block will be executed. Whenever we need to make a decision based on multiple conditions we use the if..else ladder.
If you have too many conditions if..else ladder will becomes difficult to maintain and code readability also degrades. So when you have too many conditions use the switch case instead of if..else ladder
if..else ladder example program:
We can calculate the student grades using the if..else ladder. Because we need to compare the different marks to calculate the grade.
Here is the code for the student grades program
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
#include <stdio.h> int main(void) { // Take the input from the user int num; printf("Enter student marks: "); scanf("%d",&num); // Calculate the grades using the if..else ladder if(num >= 80){ printf("You got A grade \n"); } else if ( num >=60) { printf("You got B grade \n"); } else if ( num >=40) { printf("You got C grade \n"); } else if ( num < 40) { printf("You Failed in this exam \n"); printf("Better Luck Next Time \n"); } return 0; } |
Output:
We are using the gcc compiler to run the program. you can use any C-lang compiler.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
$ gcc if-else-ladder.c $ ./a.out Enter student marks: 90 You got A grade $ ./a.out Enter student marks: 70 You got B grade $ ./a.out Enter student marks: 50 You got C grade $ ./a.out Enter student marks: 30 You Failed in this exam Better Luck Next Time $ |
We have explained the student’s grade program in detail in the following article, Please check it for details.
Conclusion:
We have discussed the Nested if else and if..else ladder.
8 Responses
[…] Nested if else and if..else ladder in C – SillyCodes […]
[…] Nested if else and if..else ladder in C-Language […]
[…] have discussed the Nested-if-else and if else ladder in an earlier article. In today’s article, we will look at the Switch Statement in C […]
[…] Nested if else and if..else ladder in C-Language […]
[…] Nested if else and if..else ladder in C-Language […]
[…] Nested if else and if..else ladder in C-Language […]
[…] Nested if else and if..else ladder in C-Language […]
[…] Nested if else and if..else ladder in C-Language […]