Program to Generate Multiplication Table in C Language
- Multiplication Table in C Program Description:
- Multiplication Table in C Program Algorithm:
- Generate Multiplication Table in C using for loop ( Up to 10):
- Multiplication Table in C up to given range (Times):
- Using Do while or Goto statement to ask for user input again in case of an Invalid range number:
- Request input until the user provides a valid number using the goto statement:
- Request input until the user provides a valid number using the Do-While Loop:
- Generate Multiplication Table up to Range using while loop in C:
- Generate Multiplication Table of a Number up to Range using while loop in C:
- Math (Multiplication) Tables in C language using the Goto Statement:
- Program to Print All Multiplication Tables from 1 to 20 numbers in C:
- Generate Multiplication Tables with Custom number of Tables and Custom Range:
- Related Programs:
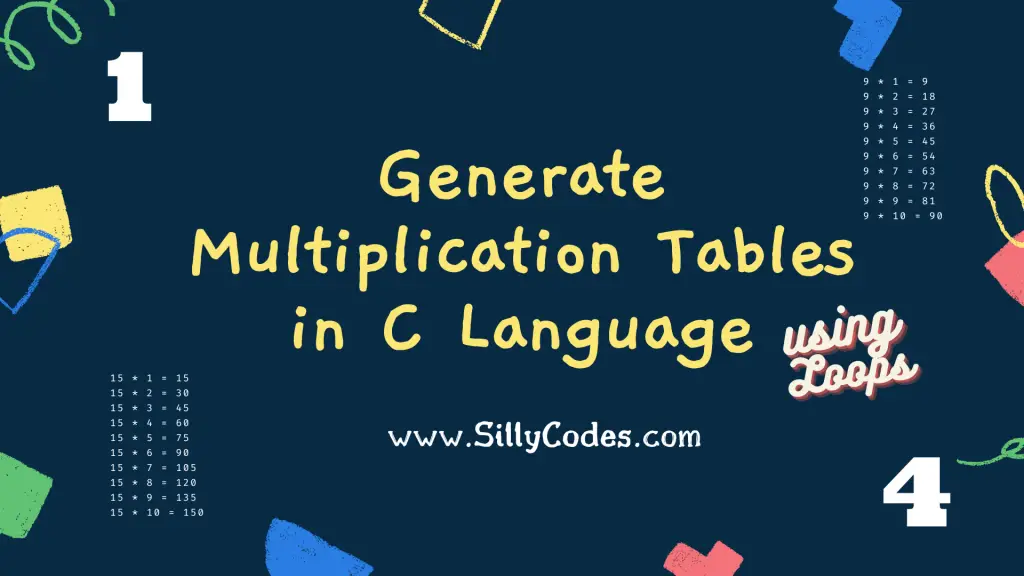
Multiplication Table in C Program Description:
We will write a program to generate the multiplication table in C Programming Language. The program will ask for a number and generates the multiplication table for the given number up to 10. Then we will customize the program to take the range from the user and finally we look at a program where we generate a multiplication table for all numbers from 1 to 20. So let’s get started.
Example Input and Output of the Program:
Input:
Enter number to generate the table: 15
Output:
1 2 3 4 5 6 7 8 9 10 11 |
Multiplication Table of 15: 15 * 1 = 15 15 * 2 = 30 15 * 3 = 45 15 * 4 = 60 15 * 5 = 75 15 * 6 = 90 15 * 7 = 105 15 * 8 = 120 15 * 9 = 135 15 * 10 = 150 |
Program Pre-Requisites:
It will be good to know the C Loops and the Arithmetic Operators. So please go through the following articles to learn more about them
- Arithmetic Operators.
- While Loop in C Language
- For Loop in C Language with Example Programs
- do while loop in Language with example programs
- Goto Statement in C
Multiplication Table in C Program Algorithm:
- The program starts by asking for the input number and we store it in num variable.
- Then we use the a C loop (for, while, etc) to print the multiplication of the num.
- We use variable i to control the Loop. Initialize the counter i with 1
- Start the Loop. Our Loop condition is i <= 10, So the loop will continue until
i value reaches 10. At Each Iteration
- Print the value of num * i.
- Then update the counter variable i by 1
- So the above loop iterates 10 times and prints one line (by multiplying the variable i and variable num) onto the console at each iteration. Thus generating the multiplication table up to 10.
We will explore several variations of the Multiplication table program. All of these methods are based on the above algorithm.
Let’s start with the most basic one. Printing Multiplication Table of given number up to 10.
Generate Multiplication Table in C using for loop ( Up to 10):
We are using the for loop to create the multiplication table in this program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
/*     Program : Generate Multiplication Table using for loop */ #include<stdio.h> int main() {     // We will use `i` as the counter     int i;     int num;     // Take the input from the user and store in 'num'     printf("Enter number to generate the table: ");     scanf("%d", &num);      // if(num <= 0) {     //    printf("Error: Invalid Input. Please try again \n");     //    return 0;     // }     printf("Multiplication Table of %d: \n", num);     /*     1. Initialization: 'i = 1', We set our counter 'i' to 1     2. Condition: 'i<=10', This is our loop condition - loop will continue until condition becomes false     3. Update  : 'i++', Our loop control variable update statement     */     for (i = 1; i<= 10; i++) {         // Create the table         printf("%d * %d = %d\n", num, i, num * i);     }     return 0; } |
Program Output:
Save the above program using the .c extension. Compile and run the program using your favorite compiler. We are using the GCC compiler here.
$ gcc multiplicatioTable.c
Run the program
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
$ ./a.out Enter number to generate the table: 9 Multiplication Table of 9: 9 * 1 = 9 9 * 2 = 18 9 * 3 = 27 9 * 4 = 36 9 * 5 = 45 9 * 6 = 54 9 * 7 = 63 9 * 8 = 72 9 * 9 = 81 9 * 10 = 90 $ ./a.out Enter number to generate the table: 15 Multiplication Table of 15: 15 * 1 = 15 15 * 2 = 30 15 * 3 = 45 15 * 4 = 60 15 * 5 = 75 15 * 6 = 90 15 * 7 = 105 15 * 8 = 120 15 * 9 = 135 15 * 10 = 150 $ |
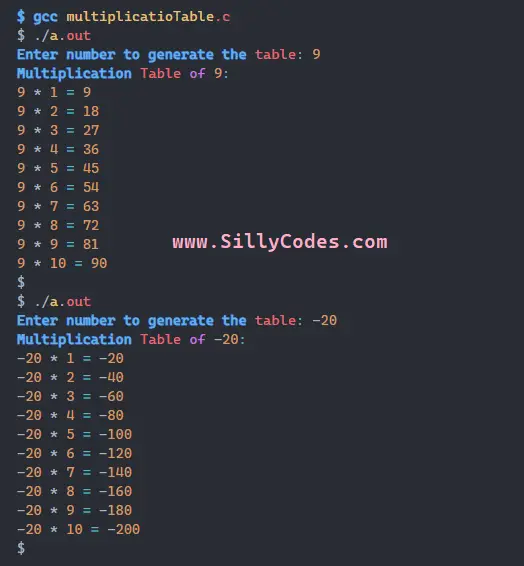
What if the user enters a negative number, Here is an example of a negative number multiplication table.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// For Negative Numbers. $ ./a.out Enter number to generate the table: -20 Multiplication Table of -20: -20 * 1 = -20 -20 * 2 = -40 -20 * 3 = -60 -20 * 4 = -80 -20 * 5 = -100 -20 * 6 = -120 -20 * 7 = -140 -20 * 8 = -160 -20 * 9 = -180 -20 * 10 = -200 $ |
📢 If you don’t want to accept the Negative numbers, Then you can add the condition to display an error message based on user input.
Multiplication Table in C up to given range (Times):
How many times do you want to print the table?
In the above example, We are printing the table up to the number 10 (times). Let’s customize it and ask for the user input for it as well ( Number of times or up to the given range – times)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/*     Program : Generate Multiplication Table upto Range */ #include<stdio.h> int main() {     // We will use `i` as the counter     int i;     int num;      // range variable.     int times;     // Take the input from the user and store in 'num'     printf("Enter number to generate the table: ");     scanf("%d", &num);      printf("How many times you want to print (upto range): ");     scanf("%d", ×);      // Don't allow the Negative number for range. (times.)     if(times <= 0) {         printf("Error: Invalid Input. Please try again \n");         return 0;     }     printf("Multiplication Table of %d: \n", num);     /*     1. Initialization: 'i = 1', We set our counter 'i' to 1     2. Condition: 'i<=times', Iterate upto range 'times'     3. Update  : 'i++', Our loop control variable update statement     */     for (i = 1; i<= times; i++) {         // Create the table         printf("%d * %d = %d\n", num, i, num * i);     }     return 0; } |
As you can see from the above program, We also added a new variable times and asking for the user input and storing the given range value in the variable times
Program Output:
Let’s compile and run the program.
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc multiplicatioTable.c $ ./a.out Enter number to generate the table: 8 How many times you want to print (upto range): 5 Multiplication Table of 8: 8 * 1 = 8 8 * 2 = 16 8 * 3 = 24 8 * 4 = 32 8 * 5 = 40 $ |
Here the program asked for a second number, which defines the range. and We entered the range as 5. So the program displayed the 8 table up to 5 times.
As the range is not going to be a negative number. So our program won’t accept the negative number for times the variable. Let’s check it.
1 2 3 4 5 |
$ ./a.out Enter number to generate the table: 15 How many times you want to print (upto range): -3 Error: Invalid Input. Please try again $ |
As you can see from the above output, The program is displaying the error message if the user provides a negative number for times variables ( range variables)
Here is one more example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
$ ./a.out Enter number to generate the table: 15 How many times you want to print (upto range): 20 Multiplication Table of 15: 15 * 1 = 15 15 * 2 = 30 15 * 3 = 45 15 * 4 = 60 15 * 5 = 75 15 * 6 = 90 15 * 7 = 105 15 * 8 = 120 15 * 9 = 135 15 * 10 = 150 15 * 11 = 165 15 * 12 = 180 15 * 13 = 195 15 * 14 = 210 15 * 15 = 225 15 * 16 = 240 15 * 17 = 255 15 * 18 = 270 15 * 19 = 285 15 * 20 = 300 $ |
Using Do while or Goto statement to ask for user input again in case of an Invalid range number:
In the above example program, we are simply terminating the program in case of a negative number( Invalid number). So instead of stopping the program. Let’s ask the user input again using the goto statement or do-while loop.
Let’s start with the goto statement.
Request input until the user provides a valid number using the goto statement:
In this program, We are going to use the goto statement to jump from one statement in the program to another statement.
Whenever a user enters a negative number, The goto START; ( at line 26) will be executed and This will make the program go back to the goto label which is START ( at line 18). So The program displays the How many times you want to print (upto range): message again to accept the new input.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/*     Program : Generate Multiplication Table upto Range with goto */ #include<stdio.h> int main() {     // We will use `i` as the counter     int i;     int num;     // range variable.     int times;         // Take the input from the user and store in 'num'     printf("Enter a number to generate the table: ");     scanf("%d", &num);      START:    // goto statement label.     printf("How many times you want to print (upto range): ");     scanf("%d", ×);      // Don't allow the Negative number for range. (times.)     if(times <= 0) {         printf("Error: Invalid Input. Please try again \n");         // goto label to jump from one statement to another.         goto START;     }     printf("Multiplication Table of %d: \n", num);     /* Loop from '1' to range ('times')*/     for (i = 1; i<= times; i++) {         // Create the table         printf("%d * %d = %d\n", num, i, num * i);     }     return 0; } |
Program Output:
Let’s compile and run the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
$ gcc multiplicatioTable.c $ ./a.out Enter a number to generate the table: 12 How many times you want to print (upto range): 3 Multiplication Table of 12: 12 * 1 = 12 12 * 2 = 24 12 * 3 = 36 $ ./a.out Enter a number to generate the table: 8 How many times you want to print (upto range): -4 Error: Invalid Input. Please try again How many times you want to print (upto range): -10 Error: Invalid Input. Please try again How many times you want to print (upto range): 0 Error: Invalid Input. Please try again How many times you want to print (upto range): 5 Multiplication Table of 8: 8 * 1 = 8 8 * 2 = 16 8 * 3 = 24 8 * 4 = 32 8 * 5 = 40 $ |
As you can see from the above output, the Program is working as expected in normal cases, But when the user enters a Negative number like -4, It displayed the error message – How many times you want to print (up to range): -4. and asked for input again. This process continues until the user enters a valid number.
Request input until the user provides a valid number using the Do-While Loop:
In this program, We are going to use the do while loop instead of the goto statement to ask for the user input again.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
/*     Program : Generate Multiplication Table upto Range with do while */ #include<stdio.h> int main() {     // We will use `i` as the counter     int i;     int num;     // range variable.     int times;         // Take the input from the user and store in 'num'     printf("Enter a number to generate the table: ");     scanf("%d", &num);      // take range from user.     do {         printf("How many times you want to print (upto range): ");         scanf("%d", ×);     } while (times <= 0);     printf("Multiplication Table of %d: \n", num);     /* Loop from '1' to range ('times')*/     for (i = 1; i<= times; i++) {         // Create the table         printf("%d * %d = %d\n", num, i, num * i);     }     return 0; } |
As you can see from the above code, We wrapped the second printf function using the do while loop.
1 2 3 4 |
do { Â Â Â Â Â Â Â Â printf("How many times you want to print (upto range): "); Â Â Â Â Â Â Â Â scanf("%d", ×); } while (times <= 0); |
So the do while loop continues executes until the condition times<= 0, becomes False. Which will become False when the user enters a positive number.
Program Output:
Save the program. Then compile and run the program.
Normal Case:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ gcc multiplicatioTable-do-while.c $ ./a.out Enter a number to generate the table: 3 How many times you want to print (upto range): 7 Multiplication Table of 3: 3 * 1 = 3 3 * 2 = 6 3 * 3 = 9 3 * 4 = 12 3 * 5 = 15 3 * 6 = 18 3 * 7 = 21 $ |
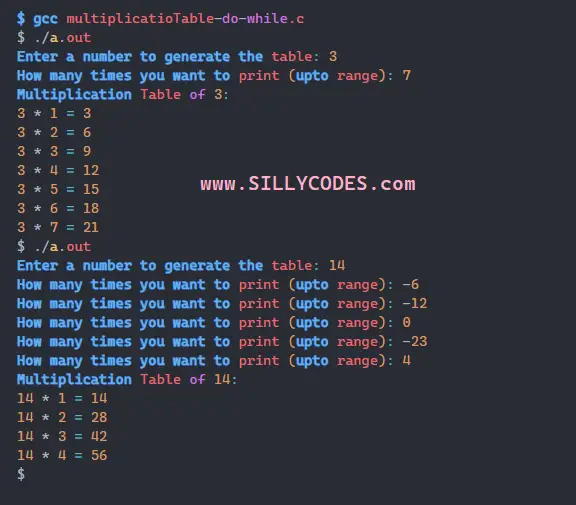
When the user enters a negative number:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter a number to generate the table: 14 How many times you want to print (upto range): -6 How many times you want to print (upto range): -12 How many times you want to print (upto range): 0 How many times you want to print (upto range): -23 How many times you want to print (upto range): 4 Multiplication Table of 14: 14 * 1 = 14 14 * 2 = 28 14 * 3 = 42 14 * 4 = 56 $ |
As you can see from the above output When the user enters a negative value -6 for the range(times variable), The do-while loop condition times<= 0 returned True, So we asked for the input again, Then user entered the another negative number -12, Still, the condition is True. It will become False only if the user enters a positive number. So the do-while loop won’t terminate until the user enters a valid number.
Generate Multiplication Table up to Range using while loop in C:
So far we have used the for loop to generate the table, Let’s use the while loop to generate the multiplication table. The program algorithm is not going to change.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
/*     Program : Generate Multiplication Table upto Range using while loop */ #include<stdio.h> int main() {     // We will use `i` as the counter     int i;     int num;     // range variable.     int times;         // Take the input from the user and store in 'num'     printf("Enter a number to generate the table: ");     scanf("%d", &num);      START:    // goto statement label.     printf("How many times you want to print (upto range): ");     scanf("%d", ×);      // Don't allow the Negative number for range. (times.)     if(times <= 0) {         printf("Error: Invalid Input. Please try again \n");         // goto label to jump from one statement to another.         goto START;     }     printf("Multiplication Table of %d: \n", num);     /*     1. Initialization: start with 'i = 1'     2. Condition: 'i<=times',     3. Update  : 'i++',     */     i = 1;      // using while loop.     while(i <= times) {         printf("%d * %d = %d\n", num, i, num * i);         i++;     }     return 0; } |
If you observe, When a user enters a negative number, we are using the goto statement method described above to ask the user again for the input.
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
$ gcc multiplicatioTable.c $ ./a.out Enter a number to generate the table: 11 How many times you want to print (upto range): 8 Multiplication Table of 11: 11 * 1 = 11 11 * 2 = 22 11 * 3 = 33 11 * 4 = 44 11 * 5 = 55 11 * 6 = 66 11 * 7 = 77 11 * 8 = 88 $ ./a.out Enter a number to generate the table: 17 How many times you want to print (upto range): -4 Error: Invalid Input. Please try again How many times you want to print (upto range): -8 Error: Invalid Input. Please try again How many times you want to print (upto range): 3 Multiplication Table of 17: 17 * 1 = 17 17 * 2 = 34 17 * 3 = 51 $ $ ./a.out Enter a number to generate the table: -5 How many times you want to print (upto range): 5 Multiplication Table of -5: -5 * 1 = -5 -5 * 2 = -10 -5 * 3 = -15 -5 * 4 = -20 -5 * 5 = -25 $ |
We can see from the above output, We are getting the expected results.
Generate Multiplication Table of a Number up to Range using while loop in C:
Here is the program to generate the multiplication table using the do while loop in C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
/*     Program : Generate Multiplication Table upto Range using do while loop */ #include<stdio.h> int main() {     // We will use `i` as the counter     int i;     int num;     // range variable.     int times;         // Take the input from the user and store in 'num'     printf("Enter a number to generate the table: ");     scanf("%d", &num);      START:    // goto statement label.     printf("How many times you want to print (upto range): ");     scanf("%d", ×);      // Don't allow the Negative number for range. (times.)     if(times <= 0) {         printf("Error: Invalid Input. Please try again \n");         // goto label to jump from one statement to another.         goto START;     }     printf("Multiplication Table of %d: \n", num);     /*     1. Initialization: start with 'i = 1'     2. Condition: 'i<=times',     3. Update  : 'i++',     */     i = 1;      // Let's use the do while loop.     do {         printf("%d * %d = %d\n", num, i, num * i);         i++;     } while(i <= times);     return 0; } |
Program Output:
Compile and run the program using your favorite compiler, We are using the GCC compiler to Compile and Run the C Program under Linux operating system
Test Case 1: Positive numbers for num and times
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
$ gcc multiplicatioTable.c $ ./a.out Enter a number to generate the table: 6 How many times you want to print (upto range): 7 Multiplication Table of 6: 6 * 1 = 6 6 * 2 = 12 6 * 3 = 18 6 * 4 = 24 6 * 5 = 30 6 * 6 = 36 6 * 7 = 42 $ $ ./a.out Enter a number to generate the table: 2 How many times you want to print (upto range): 1 Multiplication Table of 2: 2 * 1 = 2 $ |
When the user enters a negative number for range( times variable)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ ./a.out Enter a number to generate the table: 22 How many times you want to print (upto range): -5 Error: Invalid Input. Please try again How many times you want to print (upto range): -9 Error: Invalid Input. Please try again How many times you want to print (upto range): 5 Multiplication Table of 22: 22 * 1 = 22 22 * 2 = 44 22 * 3 = 66 22 * 4 = 88 22 * 5 = 110 $ |
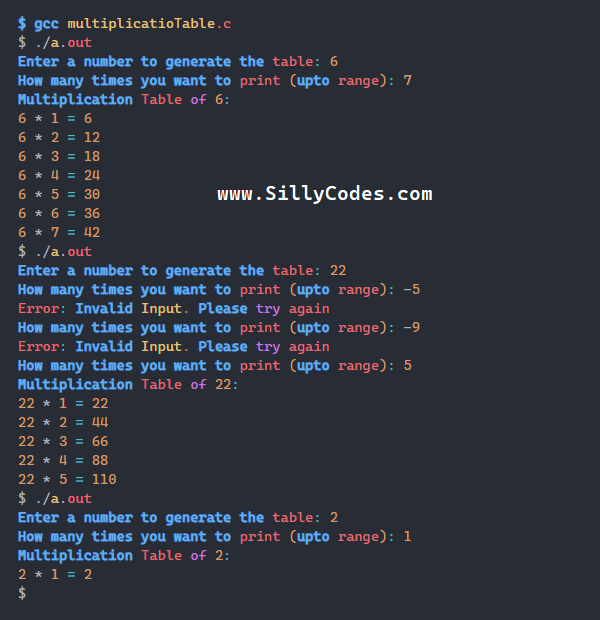
Math (Multiplication) Tables in C language using the Goto Statement:
We can also create the loop-like behavior using the goto statement. This means we can use the Goto statement to iterate over a block of code. So let’s use the goto statement to print the multiplication table in C language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
/*     Program : Generate Multiplication Table upto Range using goto statement loop */ #include<stdio.h> int main() {     // We will use `i` as the counter     int i;     int num;     // range variable.     int times;         // Take the input from the user and store in 'num'     printf("Enter a number to generate the table: ");     scanf("%d", &num);      START:    // goto statement label.     printf("How many times you want to print (upto range): ");     scanf("%d", ×);      // Don't allow the Negative number for range. (times.)     if(times <= 0) {         printf("Error: Invalid Input. Please try again \n");         // goto label to jump from one statement to another.         goto START;     }     printf("Multiplication Table of %d: \n", num);     /*     1. Initialization: start with 'i = 1'     2. Condition: 'i<=times',     3. Update  : 'i++',     */     i = 1;      // Let's use the goto statement to create loop.     LOOP:     printf("%d * %d = %d\n", num, i, num * i);     i++;     if(i <= times)     {         goto LOOP;     }     return 0; } |
Here is the main block of code, which creates the loop using the goto statement.
1 2 3 4 5 6 7 |
LOOP: printf("%d * %d = %d\n", num, i, num * i); i++; if(i <= times) { Â Â Â Â goto LOOP; } |
Program Output:
Compile and run the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
$ gcc multiplicatioTable.c $ ./a.out Enter a number to generate the table: 10 How many times you want to print (upto range): 7 Multiplication Table of 10: 10 * 1 = 10 10 * 2 = 20 10 * 3 = 30 10 * 4 = 40 10 * 5 = 50 10 * 6 = 60 10 * 7 = 70 $ ./a.out Enter a number to generate the table: -5 How many times you want to print (upto range): 2 Multiplication Table of -5: -5 * 1 = -5 -5 * 2 = -10 $ ./a.out Enter a number to generate the table: 8 How many times you want to print (upto range): -4 Error: Invalid Input. Please try again How many times you want to print (upto range): -2 Error: Invalid Input. Please try again How many times you want to print (upto range): 8 Multiplication Table of 8: 8 * 1 = 8 8 * 2 = 16 8 * 3 = 24 8 * 4 = 32 8 * 5 = 40 8 * 6 = 48 8 * 7 = 56 8 * 8 = 64 $ |
Program to Print All Multiplication Tables from 1 to 20 numbers in C:
Now let’s generate all multiplication tables from the number 1 to number 20 using the for loop.
We are going to use the two loops,
- The Outer-Loop, Which will loop start from 1 to 20. We use cnt variable to control the outer loop.
- The Inner Loop Creates the table. Which starts from 1 to up to the given range times. (the range must be a positive number).
Here is the program to print math tables from 1 to 20 numbers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
/*     Program : Generate Multiplication Tables from 1 to 20 */ #include<stdio.h> int main() {     // We will use `i` as the counter     int i;     int num;     // range variable.     int times;         // // Take the input from the user and store in 'num'     // printf("Enter a number to generate the table: ");     // scanf("%d", &num);      START:    // goto statement label.     printf("How many times you want to print (Range of each table): ");     scanf("%d", ×);      // Don't allow the Negative number for range. (times.)     if(times <= 0) {         printf("Error: Invalid Input. Please try again \n");         // goto label to jump from one statement to another.         goto START;     }         int cnt;     // 'et's print 20 Tables.     num = 20;      for(cnt = 1; cnt <= num; cnt++)     {         printf("Multiplication Table of %d: \n", cnt);         /* Loop from '1' to range ('times')*/         for (i = 1; i<= times; i++) {             // Create the table             printf("%d * %d = %d\n", cnt, i, cnt * i);         }         printf("-------------\n");     }     return 0; } |
Program Output:
Let’s compile and run the program. This output is going to generate all tables from 1 to 20. So the output will be very large.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 |
$ gcc multiplicatioTable.c $ ./a.out How many times you want to print (Range of each table): 10 Multiplication Table of 1: 1 * 1 = 1 1 * 2 = 2 1 * 3 = 3 1 * 4 = 4 1 * 5 = 5 1 * 6 = 6 1 * 7 = 7 1 * 8 = 8 1 * 9 = 9 1 * 10 = 10 ------------- Multiplication Table of 2: 2 * 1 = 2 2 * 2 = 4 2 * 3 = 6 2 * 4 = 8 2 * 5 = 10 2 * 6 = 12 2 * 7 = 14 2 * 8 = 16 2 * 9 = 18 2 * 10 = 20 ------------- Multiplication Table of 3: 3 * 1 = 3 3 * 2 = 6 3 * 3 = 9 3 * 4 = 12 3 * 5 = 15 3 * 6 = 18 3 * 7 = 21 3 * 8 = 24 3 * 9 = 27 3 * 10 = 30 ------------- Multiplication Table of 4: 4 * 1 = 4 4 * 2 = 8 4 * 3 = 12 4 * 4 = 16 4 * 5 = 20 4 * 6 = 24 4 * 7 = 28 4 * 8 = 32 4 * 9 = 36 4 * 10 = 40 ------------- Multiplication Table of 5: 5 * 1 = 5 5 * 2 = 10 5 * 3 = 15 5 * 4 = 20 5 * 5 = 25 5 * 6 = 30 5 * 7 = 35 5 * 8 = 40 5 * 9 = 45 5 * 10 = 50 ------------- Multiplication Table of 6: 6 * 1 = 6 6 * 2 = 12 6 * 3 = 18 6 * 4 = 24 6 * 5 = 30 6 * 6 = 36 6 * 7 = 42 6 * 8 = 48 6 * 9 = 54 6 * 10 = 60 ------------- Multiplication Table of 7: 7 * 1 = 7 7 * 2 = 14 7 * 3 = 21 7 * 4 = 28 7 * 5 = 35 7 * 6 = 42 7 * 7 = 49 7 * 8 = 56 7 * 9 = 63 7 * 10 = 70 ------------- Multiplication Table of 8: 8 * 1 = 8 8 * 2 = 16 8 * 3 = 24 8 * 4 = 32 8 * 5 = 40 8 * 6 = 48 8 * 7 = 56 8 * 8 = 64 8 * 9 = 72 8 * 10 = 80 ------------- Multiplication Table of 9: 9 * 1 = 9 9 * 2 = 18 9 * 3 = 27 9 * 4 = 36 9 * 5 = 45 9 * 6 = 54 9 * 7 = 63 9 * 8 = 72 9 * 9 = 81 9 * 10 = 90 ------------- Multiplication Table of 10: 10 * 1 = 10 10 * 2 = 20 10 * 3 = 30 10 * 4 = 40 10 * 5 = 50 10 * 6 = 60 10 * 7 = 70 10 * 8 = 80 10 * 9 = 90 10 * 10 = 100 ------------- Multiplication Table of 11: 11 * 1 = 11 11 * 2 = 22 11 * 3 = 33 11 * 4 = 44 11 * 5 = 55 11 * 6 = 66 11 * 7 = 77 11 * 8 = 88 11 * 9 = 99 11 * 10 = 110 ------------- Multiplication Table of 12: 12 * 1 = 12 12 * 2 = 24 12 * 3 = 36 12 * 4 = 48 12 * 5 = 60 12 * 6 = 72 12 * 7 = 84 12 * 8 = 96 12 * 9 = 108 12 * 10 = 120 ------------- Multiplication Table of 13: 13 * 1 = 13 13 * 2 = 26 13 * 3 = 39 13 * 4 = 52 13 * 5 = 65 13 * 6 = 78 13 * 7 = 91 13 * 8 = 104 13 * 9 = 117 13 * 10 = 130 ------------- Multiplication Table of 14: 14 * 1 = 14 14 * 2 = 28 14 * 3 = 42 14 * 4 = 56 14 * 5 = 70 14 * 6 = 84 14 * 7 = 98 14 * 8 = 112 14 * 9 = 126 14 * 10 = 140 ------------- Multiplication Table of 15: 15 * 1 = 15 15 * 2 = 30 15 * 3 = 45 15 * 4 = 60 15 * 5 = 75 15 * 6 = 90 15 * 7 = 105 15 * 8 = 120 15 * 9 = 135 15 * 10 = 150 ------------- Multiplication Table of 16: 16 * 1 = 16 16 * 2 = 32 16 * 3 = 48 16 * 4 = 64 16 * 5 = 80 16 * 6 = 96 16 * 7 = 112 16 * 8 = 128 16 * 9 = 144 16 * 10 = 160 ------------- Multiplication Table of 17: 17 * 1 = 17 17 * 2 = 34 17 * 3 = 51 17 * 4 = 68 17 * 5 = 85 17 * 6 = 102 17 * 7 = 119 17 * 8 = 136 17 * 9 = 153 17 * 10 = 170 ------------- Multiplication Table of 18: 18 * 1 = 18 18 * 2 = 36 18 * 3 = 54 18 * 4 = 72 18 * 5 = 90 18 * 6 = 108 18 * 7 = 126 18 * 8 = 144 18 * 9 = 162 18 * 10 = 180 ------------- Multiplication Table of 19: 19 * 1 = 19 19 * 2 = 38 19 * 3 = 57 19 * 4 = 76 19 * 5 = 95 19 * 6 = 114 19 * 7 = 133 19 * 8 = 152 19 * 9 = 171 19 * 10 = 190 ------------- Multiplication Table of 20: 20 * 1 = 20 20 * 2 = 40 20 * 3 = 60 20 * 4 = 80 20 * 5 = 100 20 * 6 = 120 20 * 7 = 140 20 * 8 = 160 20 * 9 = 180 20 * 10 = 200 ------------- $ |
As you can see from the above output, The program printed all multiplication tables.
Generate Multiplication Tables with Custom number of Tables and Custom Range:
In the above program, We generated 20 tables. So let’s customize it.
Now the program will ask the user how many tables you want to print. (Number of tables from 1). and what is the range of each table.
For Example,
1 2 |
How many tables you want to print(From 1): 5 How many times you want to print (Range of each table): 5 |
If the user enters the number( num) as 5, and range/times as 5, Then We generate the tables from the number 1 to number 5 and Each table will have a range up to 5.
Note: This program doesn’t allow negative numbers for num and range/ times variables.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
/*     Program : Generate Multiplication Table with custom tables and range */ #include<stdio.h> int main() {     // We will use `i` as the counter     int i;     int num;     // range variable.     int times;      START:    // goto statement label.      // Take the input from the user and store in 'num'     printf("How many tables you want to print(From 1): ");     scanf("%d", &num);      printf("How many times you want to print (Range of each table): ");     scanf("%d", ×);      // Don't allow the Negative number for range. (times.)     if(num <= 0 || times <= 0 ) {         printf("Error: Invalid Input. Please try again \n");         // goto label to jump from one statement to another.         goto START;     }         int cnt;      for(cnt = 1; cnt <= num; cnt++)     {         printf("Multiplication Table of %d: \n", cnt);         /* Loop from '1' to range ('times')*/         for (i = 1; i<= times; i++) {             // Create the table             printf("%d * %d = %d\n", cnt, i, cnt * i);         }         printf("-------------\n");     }     return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 |
$ gcc multiplicatioTable.c $ ./a.out How many tables you want to print(From 1): 10 How many times you want to print (Range of each table): 5 Multiplication Table of 1: 1 * 1 = 1 1 * 2 = 2 1 * 3 = 3 1 * 4 = 4 1 * 5 = 5 ------------- Multiplication Table of 2: 2 * 1 = 2 2 * 2 = 4 2 * 3 = 6 2 * 4 = 8 2 * 5 = 10 ------------- Multiplication Table of 3: 3 * 1 = 3 3 * 2 = 6 3 * 3 = 9 3 * 4 = 12 3 * 5 = 15 ------------- Multiplication Table of 4: 4 * 1 = 4 4 * 2 = 8 4 * 3 = 12 4 * 4 = 16 4 * 5 = 20 ------------- Multiplication Table of 5: 5 * 1 = 5 5 * 2 = 10 5 * 3 = 15 5 * 4 = 20 5 * 5 = 25 ------------- Multiplication Table of 6: 6 * 1 = 6 6 * 2 = 12 6 * 3 = 18 6 * 4 = 24 6 * 5 = 30 ------------- Multiplication Table of 7: 7 * 1 = 7 7 * 2 = 14 7 * 3 = 21 7 * 4 = 28 7 * 5 = 35 ------------- Multiplication Table of 8: 8 * 1 = 8 8 * 2 = 16 8 * 3 = 24 8 * 4 = 32 8 * 5 = 40 ------------- Multiplication Table of 9: 9 * 1 = 9 9 * 2 = 18 9 * 3 = 27 9 * 4 = 36 9 * 5 = 45 ------------- Multiplication Table of 10: 10 * 1 = 10 10 * 2 = 20 10 * 3 = 30 10 * 4 = 40 10 * 5 = 50 ------------- $ |
Related Programs:
- C Program to Convert Decimal Number to Binary Number
- C Program to Convert Binary Number to Decimal Number
- C Program to Convert Octal Number to Decimal Number
- C Program to Calculate the Factorial of Number
- C Program to Find all Factors of a Number
- C Program to Calculate the GCD or HCF of Two Number
- C Program to Calculate the LCM of two Numbers
- C Program to Check 3-Digit and N-Digit Armstrong Number
- Collection of All Loops Programs
1 Response
[…] C Program to Generate Multiplication Tables […]