Find Highest Frequency Character in a String in C Language
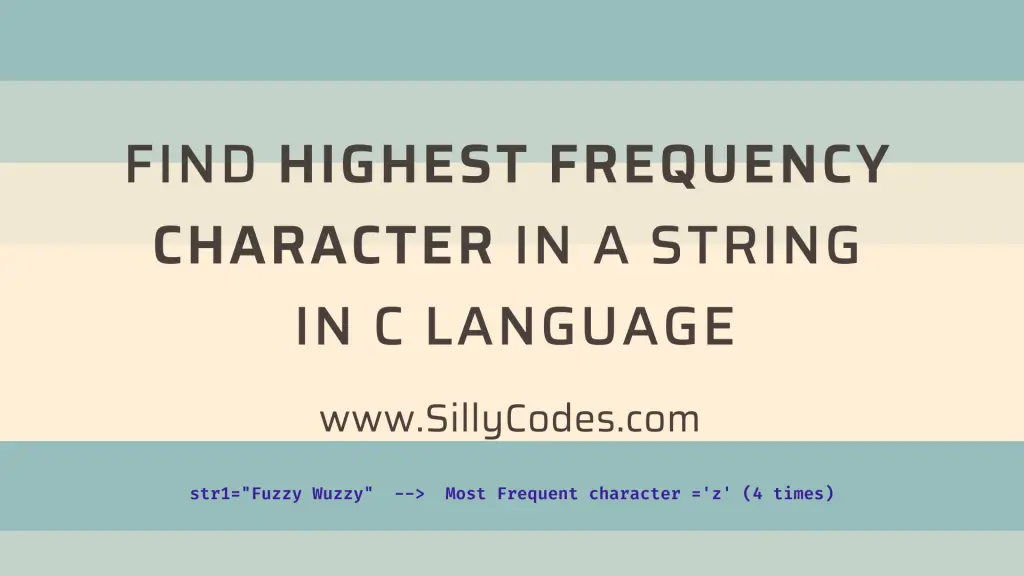
Program Description:
Write a Program to Find highest frequency character in a string in C programming language. The program will accept a string from the user and prints the most frequent character in the given string along with the character frequency.
We are going to look at a few methods to find the highest frequency character or most frequent character in a string. Each program will be followed by a detailed step-by-step explanation and program output. We also provided instructions for compiling and running the program using your compiler.
📢 This Program is part of the C String Practice Programs series
Let’s look at the program input and output.
Example Input and Output:
Input:
Enter a string : Fuzzy Wuzzy
Output:
Frequency of Characters in given string :
Character : ' ' - Frequency: 1
Character : 'F' - Frequency: 1
Character : 'W' - Frequency: 1
Character : 'u' - Frequency: 2
Character : 'y' - Frequency: 2
Character : 'z' - Frequency: 4
Character 'z' is the High Frequency Character(Frequency: 4) in the given string
In the above example, The character z is the most frequent character in the word Fuzzy Wuzzy.
Program Prerequisites:
It is recommended to know the basics of the string, and arrays in the c programming language. Please go through the following articles to refresh your concepts.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
We will look at the two different methods to find the highest frequency character in a string in C Language.
- Iterative Method
- Using User-defined Functions
Algorithm to Find Highest Frequency Character in a String in C:
Step 1: Create two macros to store the string SIZE and ASCIISIZE
Step 2: Declare a string to store the input with size of SIZE – char inputStr[SIZE];
Step 3: Create another string to store the frequencies and initialize it with zeroes. – char frequency[ASCIISIZE] = {0};
Step 4: Prompt the user for the input string and read it using the gets function and update the inputStr string.
Step 5: Iterate over the inputStr and update the frequency array with the frequency of each character in the string. Please look at the Count Frequency of characters in the string program for a detailed explanation.
Step 6: Use another loop to iterate over the frequency array and find the highest frequency character by updating the highFreq variable – if(highFreq < frequency[i]) update highFreq = frequency[i]; and ch = i ( Note: We can use above step 5 loop to get the highFreq and ch but for brevity we used two loops)
Step 7: Once the above loop is completed, The highFreq variable will contain the highest frequency and ch character will contain the most frequent character in the string.
Step 8: Print the results on the console.
Program to Find highest frequency character in a string in C – Iterative Method:
Let’s covert the above algorithm into a working C program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 |
/* program to find highest frequency character in a string in c sillycodes.com */ #include<stdio.h> // Max Size of string #define SIZE 100 #define ASCIISIZE 256 int main() { // declare a string with 'SIZE' char inputStr[SIZE]; int i, highFreq = 0, ch = 0; // Create a frequency string - Stores count based on ASCII number of characters char frequency[ASCIISIZE] = {0}; // get the string from the user printf("Enter a string : "); gets(inputStr); // Iterate through all characters of string // update the frequency array for(i = 0; inputStr[i]; i++) { // Update the frequency Array. // frequency['a'] = frequency[97] = element at 97th index will be incremented. frequency[inputStr[i]]++; } // Print the Frequency of characters printf("Frequency of Characters in given string : \n"); for(i = 0; i<ASCIISIZE; i++) { if(frequency[i] != 0) { printf("Character : '%c' - Frequency: %d\n", i, frequency[i]); // check if the present frequency is higher than 'highFreq' if(highFreq < frequency[i]) { // update 'highFreq' highFreq = frequency[i]; ch = i; } } } printf("Character '%c' is the High Frequency Character(Frequency: %d) in the given string\n", ch, highFreq); return 0; } |
Program Output:
Compile the program using your favorite compiler. We are using the GCC compiler here.
1 |
$ gcc high-freq.c |
The above command generates an executable file named a.out. Run the executable file.
Test 1:
1 2 3 4 5 6 7 8 9 10 11 |
$ ./a.out Enter a string : Fuzzy Wuzzy Frequency of Characters in given string : Character : ' ' - Frequency: 1 Character : 'F' - Frequency: 1 Character : 'W' - Frequency: 1 Character : 'u' - Frequency: 2 Character : 'y' - Frequency: 2 Character : 'z' - Frequency: 4 Character 'z' is the High Frequency Character(Frequency: 4) in the given string $ |
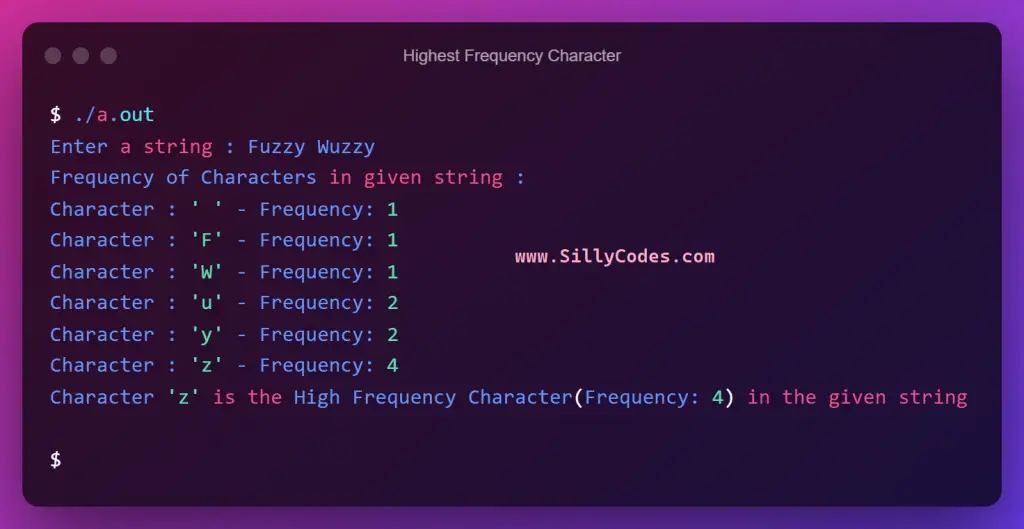
Let’s try a few more examples.
Test 2:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
$ ./a.out Enter a string : www.SillyCodes.com Frequency of Characters in given string : Character : '.' - Frequency: 2 Character : 'C' - Frequency: 1 Character : 'S' - Frequency: 1 Character : 'c' - Frequency: 1 Character : 'd' - Frequency: 1 Character : 'e' - Frequency: 1 Character : 'i' - Frequency: 1 Character : 'l' - Frequency: 2 Character : 'm' - Frequency: 1 Character : 'o' - Frequency: 2 Character : 's' - Frequency: 1 Character : 'w' - Frequency: 3 Character : 'y' - Frequency: 1 Character 'w' is the High Frequency Character(Frequency: 3) in the given string $ |
Test 3:
1 2 3 4 5 6 7 8 9 10 11 |
$ ./a.out Enter a string : AABBCCCUUU KKKAA BBBAAACC BBB Frequency of Characters in given string : Character : ' ' - Frequency: 3 Character : 'A' - Frequency: 7 Character : 'B' - Frequency: 8 Character : 'C' - Frequency: 5 Character : 'K' - Frequency: 3 Character : 'U' - Frequency: 3 Character 'B' is the High Frequency Character(Frequency: 8) in the given string $ |
As we can see from the above program, The program is properly calculating the most frequent character in the string with it’s frequency.
Find Highest Frequency Character in a String in C using a user-defined function:
In the above program, We have written all the code inside the main() function. Which is not a recommended method. So lets rewrite the above program to use a user-defined function to find the highest frequency character in the string.
We will define a user-defined function called getHighFreqChar to find the highest frequency character in the input string.
Here is the prototype of the getHighFreqChar function
char getHighFreqChar(char * inputStr)
This function takes the input string( inputStr) as the formal argument. It computes the most frequent character in the string( inputStr) and returns that character to the caller.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 |
/* program to find highest frequency character in a string in c sillycodes.com */ #include<stdio.h> // Max Size of string #define SIZE 100 #define ASCIISIZE 256 /** * @brief Get the High Frequency Character in 'inputStr' * * @param inputStr - Input String * @return char - Returns Max or High frequency character in inputstr */ char getHighFreqChar(char * inputStr) { // Create a frequency string - Stores count based on ASCII number of characters char frequency[ASCIISIZE] = {0}; int i, ch = 0, highFreq = 0; // Iterate through all characters of string // update the frequency array for(i = 0; inputStr[i]; i++) { // Update the frequency Array. // frequency['a'] = frequency[97] = element at 97th index will be incremented. frequency[inputStr[i]]++; } // Get High Frequency character for(i = 0; i<ASCIISIZE; i++) { if(frequency[i] != 0) { // check if the present frequency is higher than 'highFreq' if(highFreq < frequency[i]) { // update 'highFreq' and 'ch' characters highFreq = frequency[i]; ch = i; } } } // Return the high or Max frequency character return ch; } int main() { // declare a string with 'SIZE' char inputStr[SIZE]; int i, ch = 0; // get the string from the user printf("Enter a string : "); gets(inputStr); ch = getHighFreqChar(inputStr); printf("Character '%c' is the High Frequency Character in the given string\n", ch); return 0; } |
We can even combine Two for loops and check the highFreq number within the first for loop itself.
Program Output:
Let’s compile and Run the program.
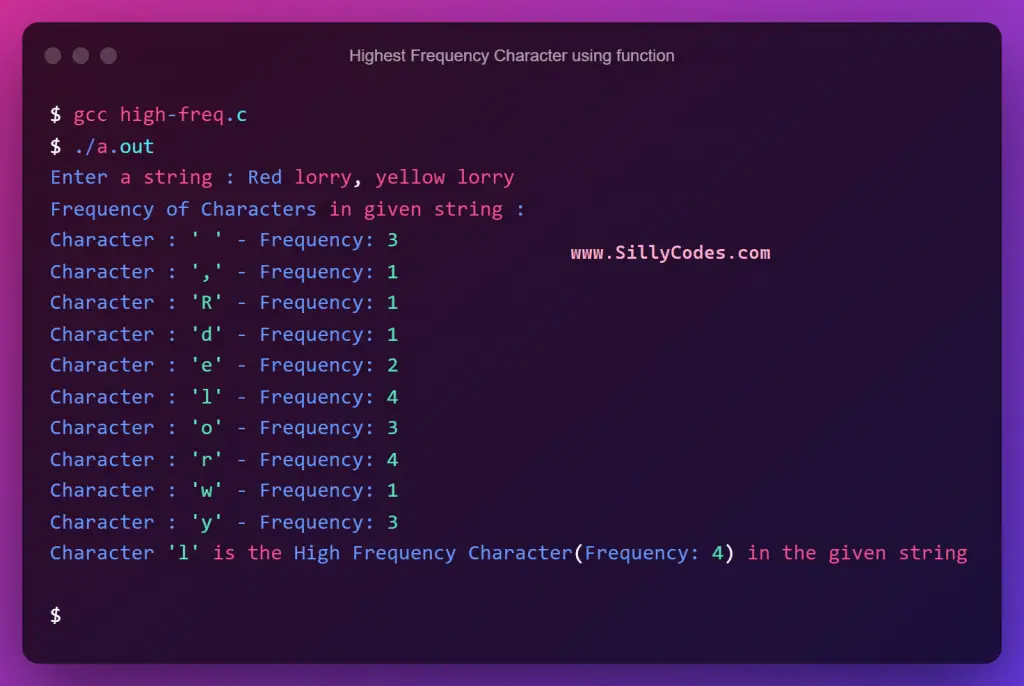
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
$ gcc high-freq.c $ ./a.out Enter a string : Red lorry, yellow lorry Frequency of Characters in given string : Character : ' ' - Frequency: 3 Character : ',' - Frequency: 1 Character : 'R' - Frequency: 1 Character : 'd' - Frequency: 1 Character : 'e' - Frequency: 2 Character : 'l' - Frequency: 4 Character : 'o' - Frequency: 3 Character : 'r' - Frequency: 4 Character : 'w' - Frequency: 1 Character : 'y' - Frequency: 3 Character 'l' is the High Frequency Character(Frequency: 4) in the given string $ $ ./a.out Enter a string : MMMMMMM GGGSSS III KK VVV Frequency of Characters in given string : Character : ' ' - Frequency: 4 Character : 'G' - Frequency: 3 Character : 'I' - Frequency: 3 Character : 'K' - Frequency: 2 Character : 'M' - Frequency: 7 Character : 'S' - Frequency: 3 Character : 'V' - Frequency: 3 Character 'M' is the High Frequency Character(Frequency: 7) in the given string $ |
We are getting the desired results. Program is able to find the most repeated character in the string.
Related String Programs:
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
- C Program to Count Frequencies of each character in a string
- C Tutorials Index
- C Programs Index
3 Responses
[…] C Program to Find Highest Frequency character in a String […]
[…] C Program to Find Highest Frequency character in a String […]
[…] C Program to Find Highest Frequency character in a String […]