Transpose of a Matrix in C Program
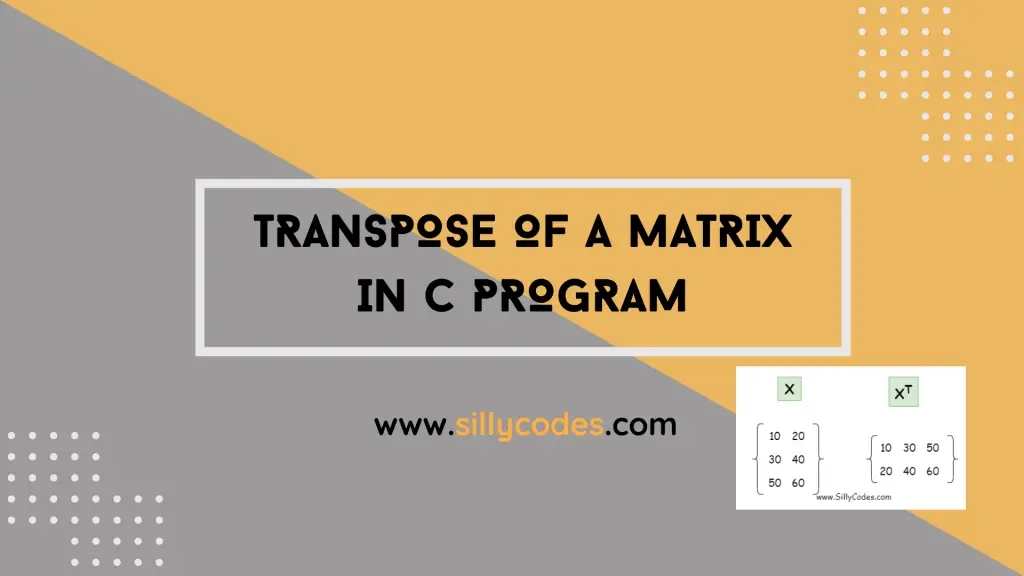
Program Description:
Write a Program to Calculate the Transpose of a Matrix in C programming language. The program should accept a Matrix from the user and calculate and display the transpose of the input matrix.
Before going any further let’s look at what is the Transpose of Matrix.
Transpose of Matrix:
The Transpose of a Matrix can be created by interchanging the original matrix rows into the columns and columns into the rows. (Exchange the Rows with Columns of original Array)
For Example, We have Matrix X with the m rows and n columns. Then Transpose of the matrix X contains the n rows and m columns Such that the i-th column of Orignal Matrix is the i-th row of Trasnpose Matrix.
Transpose of Matrix X can be denoted by XT
If X is an m x n matrix, then its transpose XT is an n x m matrix. The elements of XT are obtained by reflecting the elements of X across the main diagonal
Matrix Transpose Example:
Here is the example.
Original Matrix X:
1 2 3 |
10 20 30 40 50 60 |
Transpose of X ( XT):
1 2 |
10 30 50 20 40 60 |
Here is the graphical representation of the Transpose of a Matrix
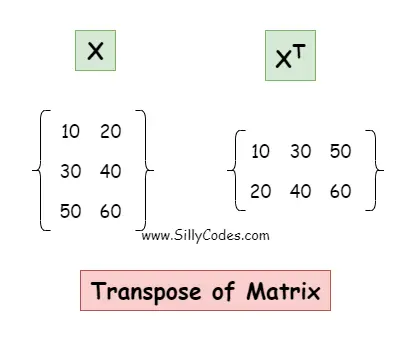
Program Prerequisites:
It is recommended to know the basics of the C Arrays, Functions in C, and Mutli-dimentional Arrays or Matrices in C langauge.
Transpose of a Matrix in C Program Explanation/Algorithm:
Let’s look at the step by step explanation of the Matrix Transpose Program in C.
- Create two integer constants named ROWS and COLUMNS, Which holds the max number of rows and columns in the Matrix. Please change this number if you want to play with the large arrays.
- Start the Program by declaring two Matrices (2D Arrays). They are X matrix, and XTranspose Matrix. The row size and column size of the matrices are ROWS and COLUMNS constants respectively.
- Prompt the user to provide the desired Row size and Column Size, and store them in the variables rows and columns respectively. (Note, These rows and columns are small letters – C is Case Sensitive language)
- Check for array bounds using the (rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) condition, And display an error message if the row/column sizeis beyond the present limits.
- Take the user input for Input Matrix i.e Matrix-X, We need to use two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the columns
- Create the First For Loop ( Outer Loop), Start the iteration from i=0 and go till the i<rows (that is for(i=0; i<rows; i++) ). At Each iteration of the Outer Loop,
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). The Inner Loop will iterate over the columns. At each iteration of the Inner Loop
- Prompt the user to provide the Matrix element and Read the element using the scanf function and Update the X[i][j] element.
- Repeat the above step for all elements in the Matrix-X
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). The Inner Loop will iterate over the columns. At each iteration of the Inner Loop
- Now, To Calculate the Transpose of the X, We need to use two more for loops.
- Create Outer For Loop ( Iterates over
rows) – for(i=0; i<rows; i++)
- Then Create the Inner For loop (Iterates over
columns) – for(j=0; j<columns; j++)
- Copy the value of the X[i][j] element to the XTranspose[j][i]. Note that, i and j are interchanged.
- i.e XTranspose[j][i] = X[i][j];
- Then Create the Inner For loop (Iterates over
columns) – for(j=0; j<columns; j++)
- Once the step-8 is complted, The XTranspose Matrix contains the Transpose of the X (Matrix).
- Display the XTranspose Matrix on the console. ( We need to use two for loops)
📢This program is part of the Array Practice Programs Series.
Program to Calculate the Transpose of a Matrix in C Language:
Here is the program to Calculate the Transpose of the Matrix in C Programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 |
/* Program to Calculate Transpose of Matrix in C SillyCodes.com */ #include <stdio.h> // Define MAX ROWS and COLUMNS const int ROWS=100; const int COLUMNS=100; int main() { // Declare two matrices. // 'X' is Input matrice // 'XTranspose' stores Transpose of 'X' int X[ROWS][COLUMNS], XTranspose[ROWS][COLUMNS]; int rows, columns, i, j; // Take the Row size and Column size from user printf("Enter the Row size for Matrix(1-%d): ", ROWS); scanf("%d", &rows); printf("Enter the Column Size for Matrix(1-%d): ", COLUMNS); scanf("%d", &columns); // check for array bounds if(rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) { printf("Invalid Rows or Columns size, Please Try again \n"); return 0; } // Take the user input for 1st Matrix - 'X' printf("Enter Elements for the Matrix(X) of Size %dX%d: \n", rows, columns); for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // printf("X[%d][%d]: ", i, j); scanf("%d", &X[i][j]); } } // Transport the Matrix 'X' and store the result in `XTranspose' for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // Transpose X. Note, 'i' and 'j' are interchanged XTranspose[j][i] = X[i][j]; } } // Print the Result printf("Transpose of Maxtrix X(XTranspose = X^T) is : \n"); // Note, That we are iterating over columns and then rows for(i=0; i<columns; i++) { for(j=0; j<rows; j++) { // Print Elements printf("%d ", XTranspose[i][j]); } printf("\n"); } return 0; } |
Program Output:
Compile the Program using GCC (Any compiler)
$ gcc transpose.c
Run the Program. ( ./a.out)
Test Case 1: When the Matrix Rows and Columns are of Same size:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
$ ./a.out Enter the Row size for Matrix(1-100): 5 Enter the Column Size for Matrix(1-100): 5 Enter Elements for the Matrix(X) of Size 5X5: 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38 40 42 44 46 48 50 52 54 56 58 Transpose of Maxtrix X(XTranspose = X^T) is : 10 20 30 40 50 12 22 32 42 52 14 24 34 44 54 16 26 36 46 56 18 28 38 48 58 $ $ ./a.out Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 3X3: 1 2 3 4 5 6 7 8 9 Transpose of Maxtrix X(XTranspose = X^T) is : 1 4 7 2 5 8 3 6 9 $ |
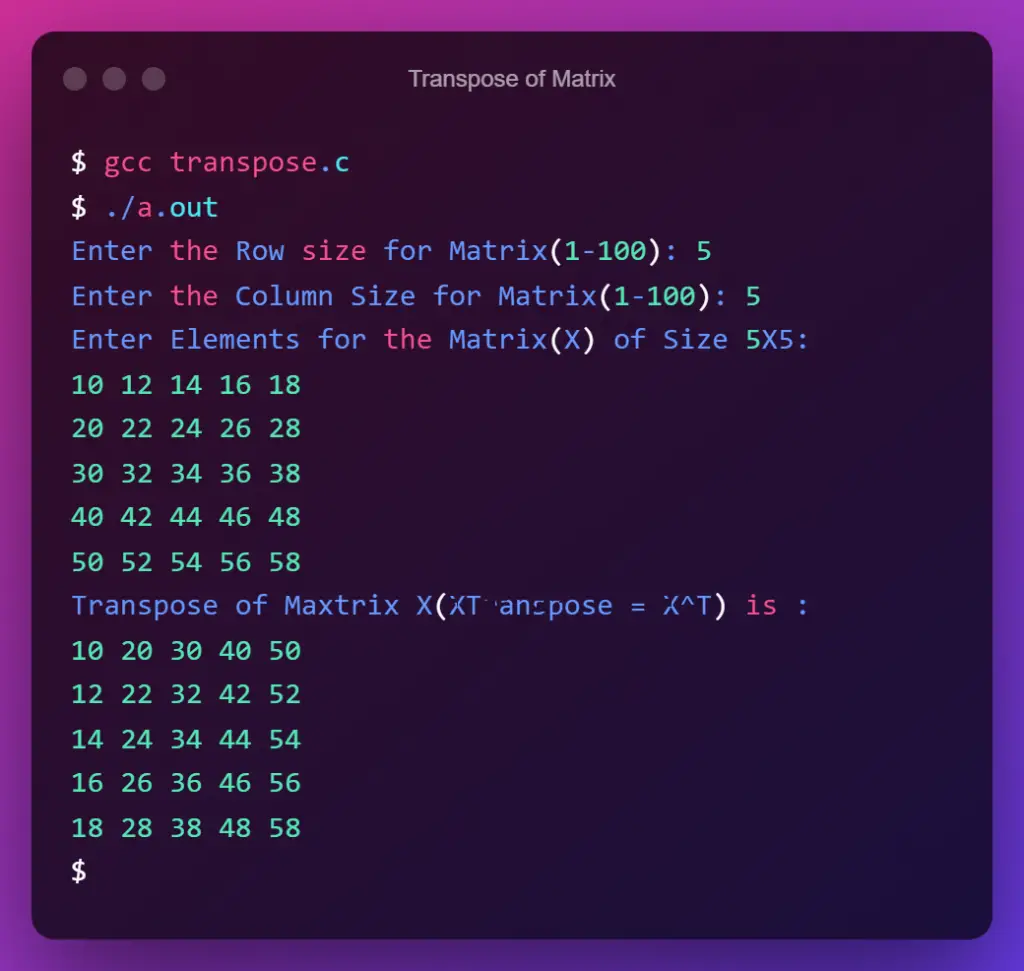
As we can see from the above output, The program is providing the desired results.
Test Case 2: When the Matrix Row size is not equal to Column Size:
1 2 3 4 5 6 7 8 9 10 11 |
$ ./a.out Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 2 Enter Elements for the Matrix(X) of Size 3X2: 10 20 30 40 50 60 Transpose of Maxtrix X(XTranspose = X^T) is : 10 30 50 20 40 60 $ |
As excepted, When the user provided the 3X2 matrix(X), The program calculated the Transpose of the Matrix(XT), which is of 2X3 size.
Test Case 3: When the size is out of limits:
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter the Row size for Matrix(1-100): 999 Enter the Column Size for Matrix(1-100): 0 Invalid Rows or Columns size, Please Try again $ ./a.out Enter the Row size for Matrix(1-100): 23 Enter the Column Size for Matrix(1-100): 873 Invalid Rows or Columns size, Please Try again $ |
The program displays an error messge Invalid Rows or Columns size, Please Try again when the size goes out of the limits .
Transpose of a Matrix using Functions in C Programming:
Let’s rewrite the above program to use the user-defined functions for reading the matrix, printing the matrix and calcluating the transpose of matrix.
Here is the Transpose of Matrix with functions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 |
/* Program to Calculate Transpose of Matrix in C using functions SillyCodes.com */ #include <stdio.h> // Define MAX ROWS and COLUMNS Constants const int ROWS=100; const int COLUMNS=100; // function declaration 'calculateTranspose' function void calculateTranspose(int rows, int columns, int X[rows][columns], int XTranspose[columns][rows]); /** * @brief - Read Matrix from the user and update 'X' * * @param rows - Rows in Matrix(2Darray) * @param columns - Columns in 'X' * @param X - 'X' is Matrix or 2D array */ void readMatrix(int rows, int columns, int X[rows][columns]) { int i, j; for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { //printf("X[%d][%d]: ", i, j); scanf("%d", &X[i][j]); } } } /** * @brief - Print the elements of the Matrix * * @param rows - Rows in Matrix(2Darray) * @param columns - Columns in 'X' * @param X[][] - 'X' is a Matrix or 2D array */ void displayMatrix(int rows, int columns, int X[rows][columns]) { int i, j; for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // Print Elements printf("%d ", X[i][j]); } printf("\n"); } } int main() { // Declare two matrices. // 'X' is Input matrice // 'XTranspose' stores Transpose of 'X' int X[ROWS][COLUMNS], XTranspose[ROWS][COLUMNS]; int rows, columns, i, j; // Take the Row size and Column size from user printf("Enter the Row size for Matrix(1-%d): ", ROWS); scanf("%d", &rows); printf("Enter the Column Size for Matrix(1-%d): ", COLUMNS); scanf("%d", &columns); // check for array bounds if(rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) { printf("Invalid Rows or Columns size, Please Try again \n"); return 0; } // Take the user input for Matrix - 'X' printf("Enter Elements for the Matrix(X) of Size %dX%d: \n", rows, columns); readMatrix(rows, columns, X); // Calculate Transpose of 'X' Matrix and Store it in 'XTranspose' Matrix calculateTranspose(rows, columns, X, XTranspose); // Print the Result printf("Transpose of Maxtrix X(XTranspose = X^T) is : \n"); // Note, That we are iterating over columns and then rows displayMatrix(columns, rows, XTranspose); return 0; } /** * @brief - Calculate Transpose of 'X' Matrix and Store it in 'XTranspose' Matrix * * @param rows - Rows in the Matrices * @param columns - Columns in the Matrices * @param X - Matrix * @param XTranspose - Transpose of 'X' Matrix * @return void */ void calculateTranspose(int rows, int columns, int X[rows][columns], int XTranspose[columns][rows]) { int i, j; // Transport the Matrix 'X' and store the result in `XTranspose' for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // Transpose X. Note, 'i' and 'j' are interchanged XTranspose[j][i] = X[i][j]; } } } |
We have defined three functions in the above program (except main() function)
- The
readMatrix() function – Used to read the elements from the user and update the Matrix
X
- Function Prototype – void readMatrix(int rows, int columns, int X[rows][columns])
- The readMatrix() function takes three formal arguments which are rows, columns, and Matrix X.
- This function uses Two For loops to iterate over the given matrix A and updates its elements by using the scanf function – scanf(“%d”, &X[i][j]);
- The readMatrix() function doesn’t return anything back to the caller. So return type is void.
- As the arrays are passed by the address/reference, All the changes made to the Matrix-X in the readMatrix() function will be visible in the Caller Function (i.e main() function).
- The
displayMatrix() function is used to print the Matrix elements on the Standard Output.
- The prototype of displayMatrix function is void displayMatrix(int rows, int columns, int X[rows][columns])
- Similar to the readMatrix() function, The displayMatrix() function also takes three formal arguments. i.e rows, columns, and X[][] (2D Array)
- The displayMatrix() function uses Two For loops to iterate over the X matrix and print the elements of the matrix. – printf("%d ", X[i][j]);
- The
calculateTranspose() function – This function used to calculate the Transpose of the given matrix
- Function Prototype – void calculateTranspose(int rows, int columns, int X[rows][columns], int XTranspose[columns][rows])
- As you can see from the above prototype, This function takes four Formal Arguments which are rows, columns, matrix X, matrix XTranspose.
- The addMatrices() function uses two for loops to iterate over the elements of the Matrices X and calculates the Transpose of X by interchanging the matrix elements – XTranspose[j][i] = X[i][j];
Program Output:
Let’s compile and Run the program and observ the output
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc transpose-func.c $ ./a.out Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 4 Enter Elements for the Matrix(X) of Size 3X4: 1 2 3 4 5 6 7 8 9 10 11 12 Transpose of Maxtrix X(XTranspose = X^T) is : 1 5 9 2 6 10 3 7 11 4 8 12 $ |
Let’s try a few more examples.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
$ ./a.out Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 3X3: 11 22 33 44 55 66 77 88 99 Transpose of Maxtrix X(XTranspose = X^T) is : 11 44 77 22 55 88 33 66 99 $ $ ./a.out Enter the Row size for Matrix(1-100): 1 Enter the Column Size for Matrix(1-100): 5 Enter Elements for the Matrix(X) of Size 1X5: 1000 2000 3000 4000 5000 Transpose of Maxtrix X(XTranspose = X^T) is : 1000 2000 3000 4000 5000 $ |
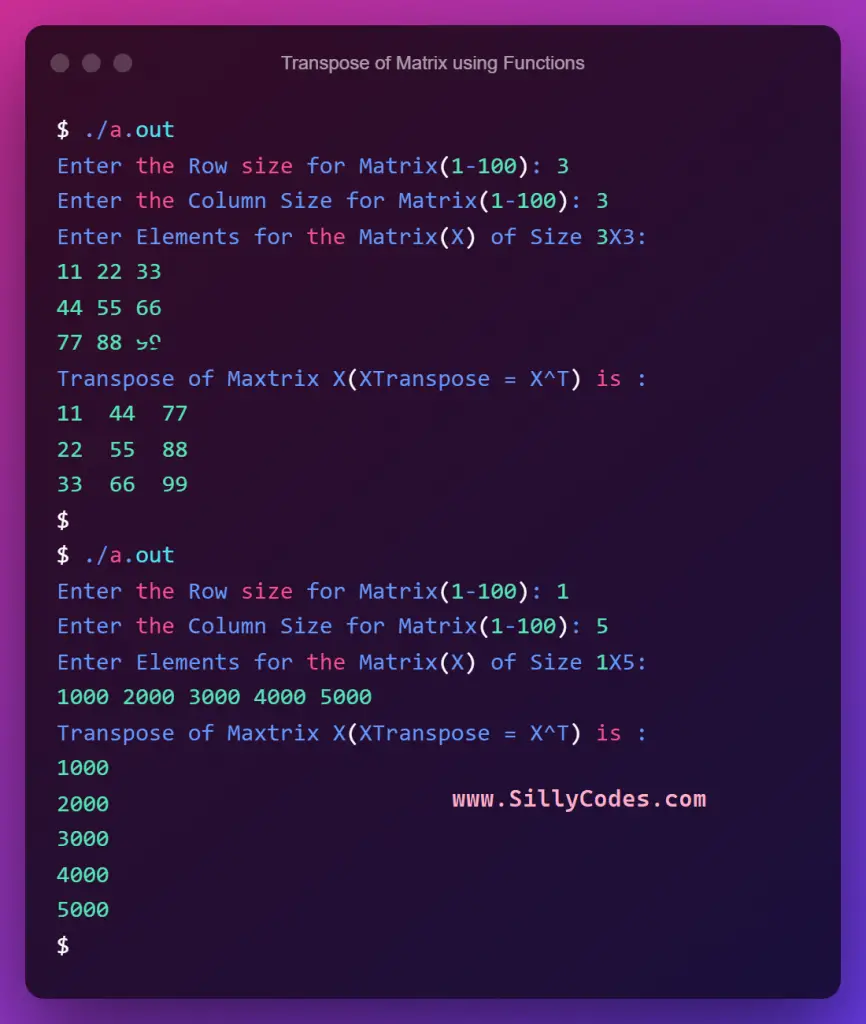
As you can see from the above output, The program is generating the desired results.
For example, When user provided the 1X5 Input matrix 1000 2000 3000 4000 5000, The program generated the 5X1 transpose matrix.
1 2 3 4 5 |
1000 2000 3000 4000 5000 |
6 Responses
[…] have looked at the Matrix Transpose Program in earlier articles, In today’s article, We will look at the Multiplication of Two Matrices in C […]
[…] C Program to Calculate the Transpose of Matrix […]
[…] C Program to Calculate the Transpose of Matrix […]
[…] C Program to Calculate the Transpose of Matrix […]
[…] 1: Calculate the Transpose of the Matrix […]
[…] C Program to Calculate the Transpose of Matrix […]