Addition of Two Matrices in C Language
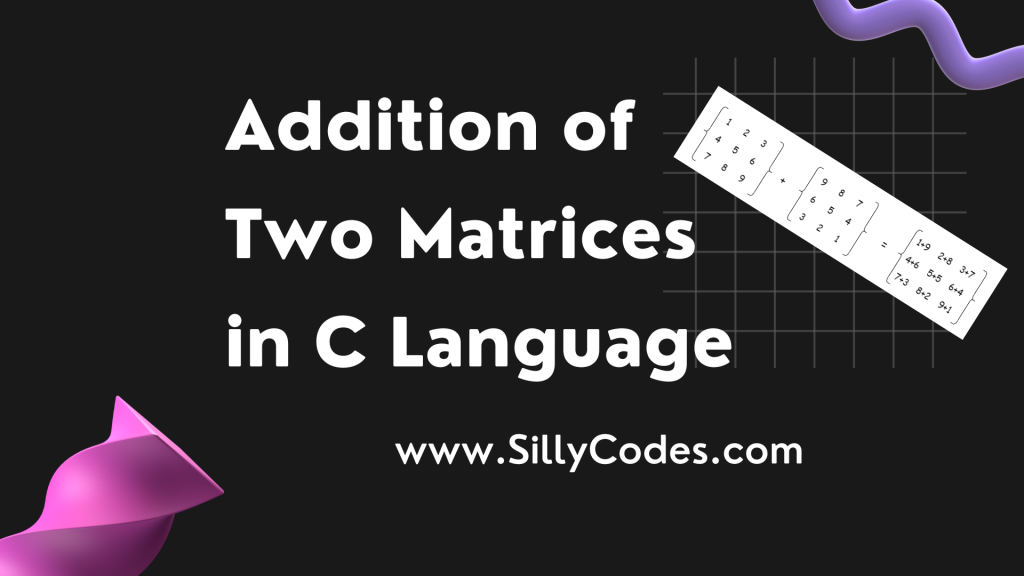
Program Description:
We have looked at the Reading and Printing Matrices and How to Pass Matrices to functions programs in earlier articles, In today’s article, We will look at the Addition of Two Matrices in C programming language. The program should accept Two Matrices from the user, perform the element-wise addition, and return the results.
Prerequisites:
Before looking at the example input and output of the program, Let’s understand how to Add Two Matrices.
How to Add Two Matrices ?:
Let’s say we have two matrices X and Y with the size of the row as rows and column size as columns
We can only Add matrices if they have the same number of rowsand columns. So we need to make sure both matrices X and Y should have the same size( Order)
So Addition of Two Matrices can be calculated by adding corresponding elements from both matrices. (Element-wise Addition)
If Matrix X = [xij] and Y = [yij] are two matrices with the same rows size and column size.
Then the Addition of matrices X and Y is: X+Y = [xij] + [yij]
Example – Addition of Two Matrices:
Matrix – X:
1 2 3 |
1 2 3 4 5 6 7 8 9 |
Matrix – Y:
1 2 3 |
9 8 7 6 5 4 3 2 1 |
Addition of Two Matrices X + Y is:
1 2 3 |
10 10 10 10 10 10 10 10 10 |
Let’s look at the graphical representation of the Matrices Addition operation.
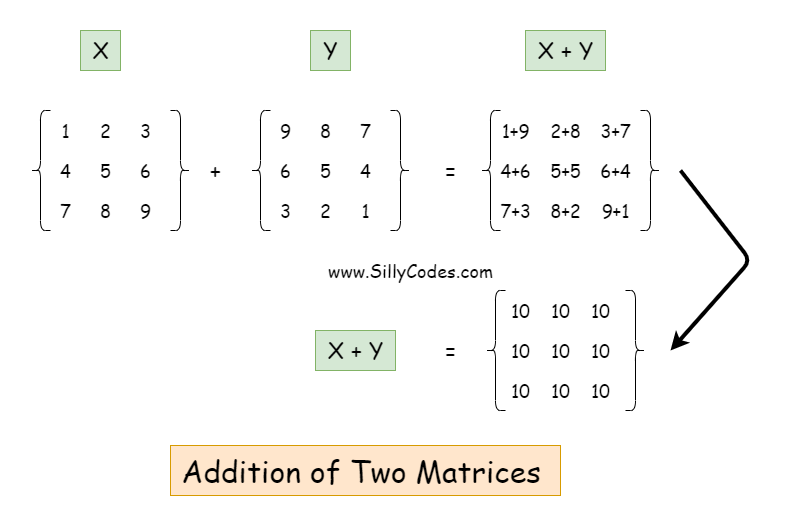
Let’s look at the program to perform the Addition of Two Matrices in the C programming language.
Addition of Two Matrices in C Program Explanation:
Here is the step-by-step explanation of the Addition of Two Matrices Program.
- Start the Program by declaring three Matrices (2D Arrays). They are X matrix, Y matrix, and Z Matrix. The max number of rows and columns in the array are 100 ( change this if you need to use larger arrays). We can also use a Macro or Constant to store the 100 value.
- Prompt the user to provide the desired Row size and Column Size, and store them in the variables rows and columns respectively.
- Check for array bounds using the (rows >= 100 || rows <= 0 || columns >= 100|| columns <= 0) condition, And display an error message if the row/column sizeis beyond the present limits.
- Take the user input for Matrix-X, We need to create two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the columns
- Create the First For Loop ( Outer Loop), Start the iteration from i=0 and go till the i<rows (i.e for(i=0; i<rows; i++) ). The Outer Loop will iterate over the
rows and At Each iteration of the Outer Loop,
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). The Inner Loop will iterate over the columns. At each iteration of the Inner Loop
- Prompt the user to provide the Matrix or 2D Array element and Read the element using the scanf function and Update the values[i][j] element.
- Repeat the above step for all elements in the 2D array or matrix
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). The Inner Loop will iterate over the columns. At each iteration of the Inner Loop
- Repeat the step-5 to Take the Second Matrix ( Matrix-Y) elements from the user.
- To Calculate the Addition of Two Matrices, We again need to use two nested For loops to iterate over the matrix elements.
- Create Outer For Loop ( iterates over rows) – for(i=0; i<rows; i++)
- Create Inner For loop (iterates over columns) – for(j=0; j<columns; j++)
- Add the X[i][j] element with the Y[i][j] and store the result in Z[i][j]
- which is equal to Z[i][j] = X[i][j] + Y[i][j];
- Create Inner For loop (iterates over columns) – for(j=0; j<columns; j++)
- Once the above step-9 is finished, The Matrix-Z contains the Sum of Matrix-X and Matrix-Y.
- Display the Results( Maxtrix-Z) using another Two For loops.
- Create Outer For Loop. Start from i=0 to i<rows – for(i=0; i<rows; i++)
- Create Inner For Loop, Start from j=0 to j<columns – for(j=0; j<columns; j++)
- Print the 2D Array or Matrix Element Z[i][j] using the printf function.
- printf("%d ", Z[i][j]);
- Create Inner For Loop, Start from j=0 to j<columns – for(j=0; j<columns; j++)
- Stop the Program.
Program for Addition of Two Matrices in C Language using Loops:
Here is the program to Add Two Matrices in C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
/* Program to Add Two Matrices in C SillyCodes.com */ #include <stdio.h> int main() { // declare three matrices. // 'X' & 'Y' are Input matrices // 'Z' stores sum of 'X' and 'Y' matrices // We can also use 'Macro' for '100' int X[100][100], Y[100][100], Z[100][100]; int rows, columns, i, j; // Take the Row size and Column size from user printf("Enter the Row size for Matrices(1-100): "); scanf("%d", &rows); printf("Enter the Column Size for Matrices(1-100): "); scanf("%d", &columns); // check for array bounds if(rows >= 100 || rows <= 0 || columns >= 100 || columns <= 0) { printf("Invalid Row or Column, Please Try again \n"); return 0; } // Take the user input for 1st Matrix - 'X' printf("Enter Elements for the First Matrix(X) of Size %dX%d: \n", rows, columns); for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { printf("X[%d][%d]: ", i, j); scanf("%d", &X[i][j]); } } // Take the user input for 2nd Matrix - 'Y' printf("Enter Elements for the Second Matrix(Y) of Size %dX%d: \n", rows, columns); for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { printf("Y[%d][%d]: ", i, j); scanf("%d", &Y[i][j]); } } // Add two matrices 'X'+'Y' element by element for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // Add elements from 'X' and 'Y' Z[i][j] = X[i][j] + Y[i][j]; } } // Print the Result printf("Sum of Two Matrices (Z=X+Y) is : \n"); for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // Print Elements printf("%d ", Z[i][j]); } printf("\n"); } return 0; } |
Program Output:
Let’s Compile and Run the program using GCC compiler (Any Compiler)
Compile the Program
$ gcc add-two-matrices.c
Test Case 1: Normal Case:
Run the program and Provide the Matrix-X and Matrix-Y elements.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
$ ./a.out Enter the Row size for Matrices(1-100): 3 Enter the Column Size for Matrices(1-100): 3 Enter Elements for the First Matrix(X) of Size 3X3: X[0][0]: 10 X[0][1]: 20 X[0][2]: 30 X[1][0]: 40 X[1][1]: 50 X[1][2]: 60 X[2][0]: 70 X[2][1]: 80 X[2][2]: 90 Enter Elements for the Second Matrix(Y) of Size 3X3: Y[0][0]: 1 Y[0][1]: 2 Y[0][2]: 3 Y[1][0]: 4 Y[1][1]: 5 Y[1][2]: 6 Y[2][0]: 7 Y[2][1]: 8 Y[2][2]: 9 Sum of Two Matrices (Z=X+Y) is : 11 22 33 44 55 66 77 88 99 $ |
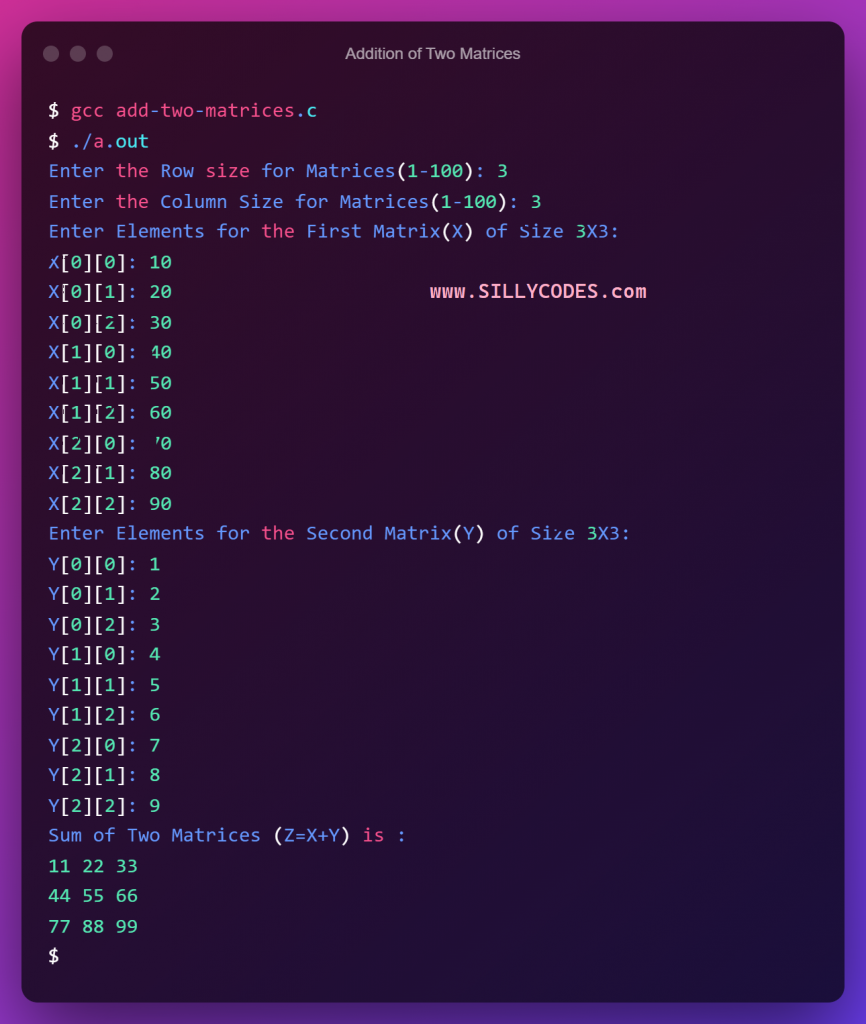
As we can see from the above output, The program initially prompted for the Row Size and Column Size of both matrices and followed by the values for all elements of the two matrices. Finally, Program Calculated and displayed the Sum of Two Matrices on the console.
Let’s look at a few more examples
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ ./a.out Enter the Row size for Matrices(1-100): 1 Enter the Column Size for Matrices(1-100): 3 Enter Elements for the First Matrix(X) of Size 1X3: X[0][0]: 56 X[0][1]: 23 X[0][2]: 67 Enter Elements for the Second Matrix(Y) of Size 1X3: Y[0][0]: 98 Y[0][1]: 76 Y[0][2]: 45 Sum of Two Matrices (Z=X+Y) is : 154 99 112 $ |
Test 2: Negative cases:
Enter the invalid row and column sizes
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter the Row size for Matrices(1-100): 200 Enter the Column Size for Matrices(1-100): 3 Invalid Row or Column, Please Try again $ ./a.out Enter the Row size for Matrices(1-100): 4 Enter the Column Size for Matrices(1-100): 0 Invalid Row or Column, Please Try again $ |
As excepted, The program is displaying the error message if the user enters Invalid Sizes ( Present Limits are 1-100).
Program to perform the Addition of Two Matrices in C using Functions:
In the above program, We have used only a single function to Read the matrix, Display the Matrix, and Calculate the sum of two matrices. So let’s divide the above monolith into smaller functions so that the program becomes clean and elegant.
Here is the rewritten version of the above program, Where we defined three extra functions (except the main() function).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 |
/* Program to Add Two Matrices in C SillyCodes.com */ #include <stdio.h> void addMatrices(int rows, int columns, int X[rows][columns], int Y[rows][columns], int Z[rows][columns]); /** * @brief - Read Matrix from the user and update 'A' * * @param rows - Rows in Matrix(2Darray) * @param columns - Columns in 'A' * @param A - 'A' is Matrix or 2D array */ void readMatrix(int rows, int columns, int A[rows][columns]) { int i, j; for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { //printf("A[%d][%d]: ", i, j); scanf("%d", &A[i][j]); } } } /** * @brief - Print the elements of the Matrix * * @param rows - Rows in Matrix(2Darray) * @param columns - Columns in 'A' * @param A[][] - 'A' is a Matrix or 2D array */ void displayMatrix(int rows, int columns, int A[rows][columns]) { int i, j; for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // Print Elements printf("%d ", A[i][j]); } printf("\n"); } } int main() { // declare three matrices. // 'X' & 'Y' are Input matrices // 'Z' stores sum of 'X' and 'Y' matrices // We can also use 'Macro' for '100' int X[100][100], Y[100][100], Z[100][100]; int rows, columns, i, j; // Take the Rows size and Columns size from user printf("Enter the Rows size for Matrices(1-100): "); scanf("%d", &rows); printf("Enter the Columns Size for Matrices(1-100): "); scanf("%d", &columns); // check for array bounds if(rows >= 100 || rows <= 0 || columns >= 100 || columns <= 0) { printf("Invalid Rows or Columns, Please Try again \n"); return 0; } // Take the user input for 1st Matrix - 'X' printf("Enter Elements for the First Matrix(X) of Size %dX%d: \n", rows, columns); readMatrix(rows, columns, X); // Take the user input for 2nd Matrix - 'Y' printf("Enter Elements for the Second Matrix(Y) of Size %dX%d: \n", rows, columns); readMatrix(rows, columns, Y); // Add two matrices 'X'+'Y' element by element addMatrices(rows, columns, X, Y, Z); // Print the Result printf("Sum of Two Matrices (Z=X+Y) is : \n"); displayMatrix(rows, columns, Z); return 0; } /** * @brief - Add Matrices pointed by 'X' and 'Y' and Store the result in 'Z' * * @param rows - Rows in the Matrices * @param columns - Columns in the Matrices * @param X - First Matrix * @param Y - Second Matrix * @param Z - Resultant Matrix * @return void */ void addMatrices(int rows, int columns, int X[rows][columns], int Y[rows][columns], int Z[rows][columns]) { int i, j; for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // Add elements from 'X' and 'Y' // Assign to 'z' Z[i][j] = X[i][j] + Y[i][j]; } } } |
In the above program, We have defined three user-defined functions (except the main), They are.
- The
readMatrix() function – Used to read the elements from the user and update the given matrix
- Prototype of readMatrix function – void readMatrix(int rows, int columns, int A[rows][columns])
- The readMatrix() function takes three formal arguments which are rows, columns, and 2D Matrix A.
- This function uses two For loops to iterate over the given matrix A and updates its elements by using the scanf function – scanf(“%d”, &A[i][j]);
- The readMatrix() function doesn’t return anything back to the caller. So return type is void.
- As the arrays are passed by the address/reference, All the changes made to the Matrix-A in the readMatrix() function will be visible in the Caller Function (i.e main() function).
- The
displayMatrix() function is used to print the Matrix elements on the Standard Output.
- The prototype of displayMatrix function is void displayMatrix(int rows, int columns, int A[rows][columns])
- Similar to the readMatrix() function, The displayMatrix() function also takes three formal arguments. i.e rows, columns, and A[][] (2D Array)
- The displayMatrix() function uses Two For loops to iterate over the A matrix and print the elements of the matrix. – printf("%d ", A[i][j]);
- The
addMatrices() function – This is the function where we actually perform the addition of the
Matrix-X and
Matrix-Y
- Prototype – void addMatrices(int rows, int columns, int X[rows][columns], int Y[rows][columns], int Z[rows][columns])
- As you can see from the above prototype, This function takes Five Formal Arguments which are rows, columns, matrix X, matrix Y, and matrix Z.
- The addMatrices() function uses two for loops to iterate over the elements of the Matrices X and Y and Performs the element-wise addition and stores the results in the Matrix-Z – Z[i][j] = X[i][j] + Y[i][j];
Program Output:
Compile and Run the Program.
Test 1:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$ gcc add-two-matrices-func.c $ ./a.out Enter the Rows size for Matrices(1-100): 3 Enter the Columns Size for Matrices(1-100): 3 Enter Elements for the First Matrix(X) of Size 3X3: 1 2 3 4 5 6 7 8 9 Enter Elements for the Second Matrix(Y) of Size 3X3: 9 8 7 6 5 4 3 2 1 Sum of Two Matrices (Z=X+Y) is : 10 10 10 10 10 10 10 10 10 $ |
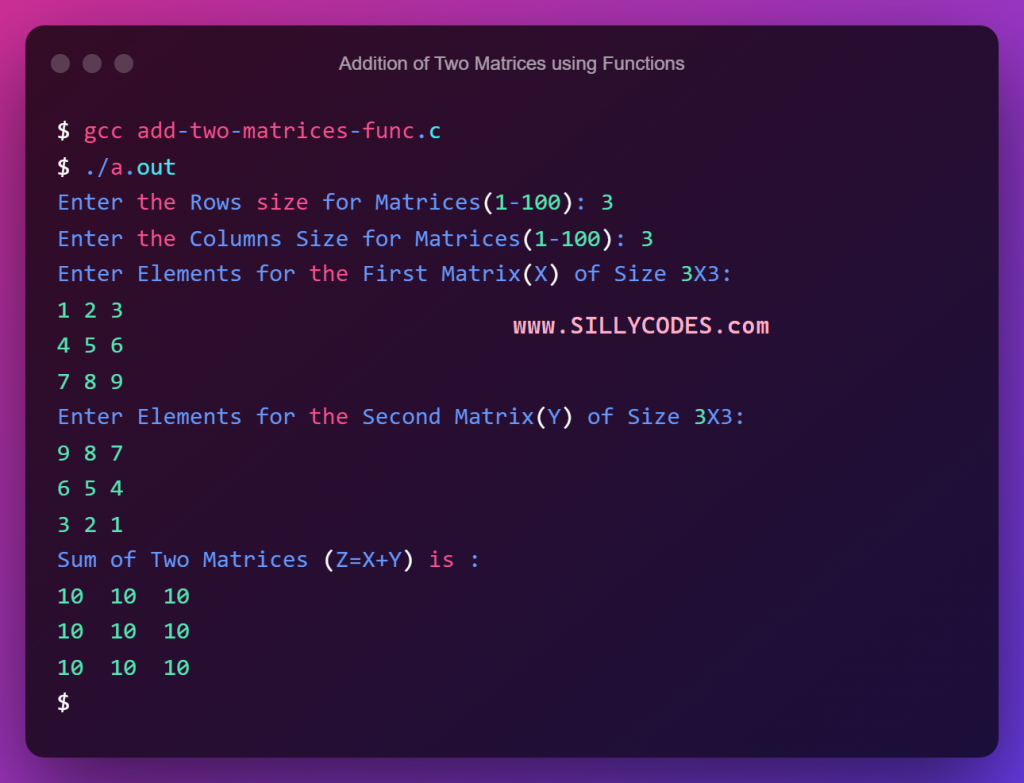
Let’s try a few more examples and observe the output
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
$ gcc add-two-matrices-func.c $ ./a.out Enter the Rows size for Matrices(1-100): 4 Enter the Columns Size for Matrices(1-100): 2 Enter Elements for the First Matrix(X) of Size 4X2: 1 2 3 4 5 6 7 8 Enter Elements for the Second Matrix(Y) of Size 4X2: 10 20 30 40 50 60 70 80 Sum of Two Matrices (Z=X+Y) is : 11 22 33 44 55 66 77 88 $ |
Test 2: Negative Tests:
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter the Rows size for Matrices(1-100): 1000 Enter the Columns Size for Matrices(1-100): 34 Invalid Rows or Columns, Please Try again $ $ ./a.out Enter the Rows size for Matrices(1-100): 22 Enter the Columns Size for Matrices(1-100): 945 Invalid Rows or Columns, Please Try again $ |
As we can see from the above output, The program is properly calculating the sum of two matrices and displaying the error message whenever the row/column sizes go out of the present limits.
Related Array Programs:
- C Program to Insert an element in Array
- C Program to Delete an Element in Array
- C Program to Find Unique Elements in an Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
2 Responses
[…] C Program to Add Two Matrices […]
[…] C Program to Add Two Matrices […]