Check Two Matrices are Identical in C | Check Two Matrices are Equal
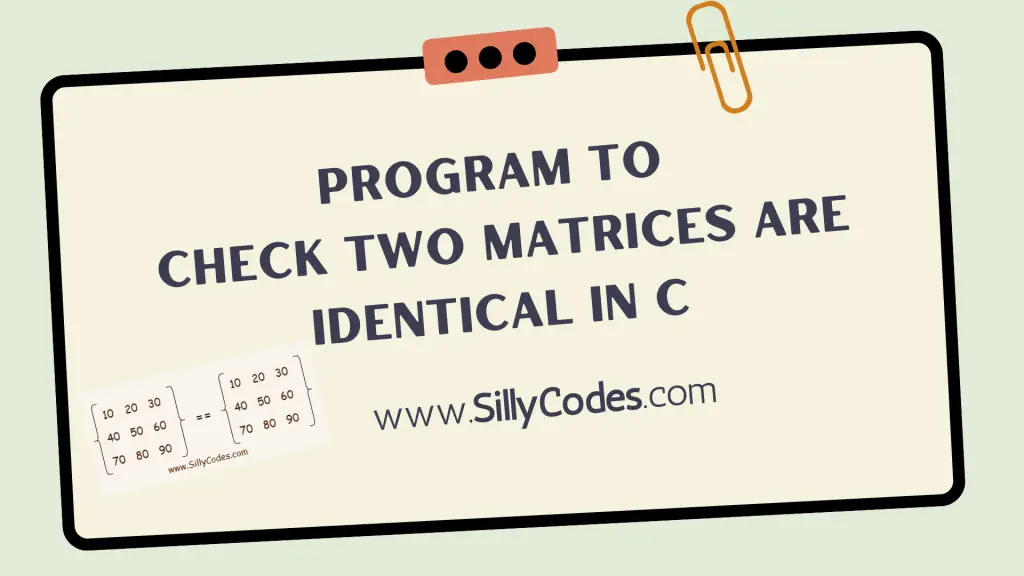
Program Description:
Write a Program to Check Two Matrices are Identical in C programming language. The program should accept two matrices from the user and do the element-by-element cooperation and return if the two matrices are equal/identical.
📢 This Program is part of the Arrays and Matrix Programs Series
Here is the example input and output of the program.
Program Excepted Input and Output:
Input:
1 2 3 4 5 6 7 8 9 10 |
Enter the Row size for Matrices(1-100): 3 Enter the Column Size for Matrices(1-100): 3 Enter Elements for the First Matrix(X) of Size 3X3: 10 20 30 40 50 60 70 80 90 Enter Elements for the Second Matrix(Y) of Size 3X3: 10 20 30 40 50 60 70 80 90 |
Output:
Given Matrices X & Y are Equal
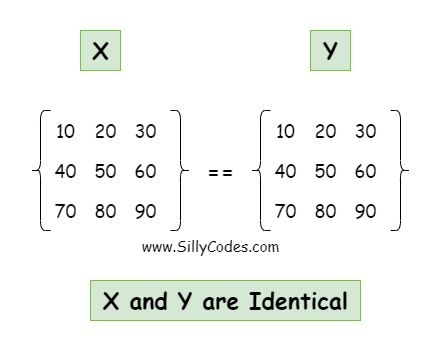
As we can see from the above output, both matrices X and Y are equal to each other.
Program Prerequisites:
We need to know the one-dimensional and multi-dimensional arrays and how to pass multi-dimensional arrays to function.
Check Two Matrices are Identical in C Program Explanation:
Let’s look at the step-by-step instructions to check if two matrices are identical i.e two matrices are equal C Program
- Create two integer constants named ROWS and COLUMNS, Which holds the max number of rows and columns.(Change this number if you want to use large arrays)
- Start the Program by declaring two Matrices (2D Arrays). They are X matrix, Y matrix. The row size and column size of the matrices are ROWS and COLUMNS respectively.
- Prompt the user to provide the desired Row size and Column Size, and store them in the variables rows and columns respectively.
- Check if the array size is going out of present limits ( 1-100) using the (rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) condition, And display an error message if the row/column sizeis beyond the present limits.
- Take the user input for Matrix-X, We need to create two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the columns
- Create the First For Loop ( Outer Loop), Start the iteration from i=0 and go till the i<rows (which is equal to for(i=0; i<rows; i++) ). At Each iteration of the Outer Loop,
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). At each iteration of the Inner Loop
- Prompt the user to provide the Matrix or 2D Array element and Read the element using the scanf function and Update the X[i][j] element.
- Repeat the above step for all elements in the 2D array or matrix
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). At each iteration of the Inner Loop
- Repeat the step-5 to Take the Second Matrix ( Matrix-Y) elements from the user.
- To Check if the two matrices are Identical, The two matrices need to be compared element by element. We can say that two matrices are identical or equal to one another if they both had similar elements at a similar index.
- Create Outer For Loop to iterate over rows – for(i=0; i<rows; i++)
- Create Inner For loop to iterate over columns – for(j=0; j<columns; j++), At Each Iteration,
- Check if the X[i][j] element is equal to Y[i][j] element – if(X[i][j] != Y[i][j])
- If any element pair is not equal, Then Matrix X and Matrix Y are not Identical.
- Create Inner For loop to iterate over columns – for(j=0; j<columns; j++), At Each Iteration,
- Once the above step-9 is finished and Program is not returned, Then the all elements in the Matrix X and Matrix Y are the same, So we can say both matrices are Identical or Equal.
- Display the message on the console.
- Stop the Program.
Program to Check Two Matrices are Identical in C Using Iterative Approach:
Here is the program to check if the two matrices are equal in the C programming.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 |
/* Program to Check if two matrices are equal in C SillyCodes.com */ #include <stdio.h> const int ROWS=100; const int COLUMNS=100; int main() { // Declare two matrices. // 'X' & 'Y' are Input matrices int X[ROWS][COLUMNS], Y[ROWS][COLUMNS]; int rows, columns, i, j; // Take the Row size and Column size from user printf("Enter the Row size for Matrices(1-%d): ", ROWS); scanf("%d", &rows); printf("Enter the Column Size for Matrices(1-%d): ", COLUMNS); scanf("%d", &columns); // check for array bounds if(rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) { printf("Invalid Rows or Columns size, Please Try again \n"); return 0; } // Take the user input for 1st Matrix - 'X' printf("Enter Elements for the First Matrix(X) of Size %dX%d: \n", rows, columns); for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // printf("X[%d][%d]: ", i, j); scanf("%d", &X[i][j]); } } // Take the user input for 2nd Matrix - 'Y' printf("Enter Elements for the Second Matrix(Y) of Size %dX%d: \n", rows, columns); for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // printf("Y[%d][%d]: ", i, j); scanf("%d", &Y[i][j]); } } // Traverse each element in the Arrays and compare the values. // Iterate over rows for(i=0; i<rows; i++) { // Iterate over the columns for(j=0; j<columns; j++) { // check if the values are not equal if(X[i][j] != Y[i][j]) { // Matrices "X" and "Y" are not equal printf("Given Matrices X & Y are Not Equal\n"); return 0; } } } // If we reached till here, Then Matrices are equal. printf("Given Matrices X & Y are Equal\n"); return 0; } |
Program Output:
Compile and Run the above c matrix program.
Test 1: Check Normal Cases:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc check-matrices-equal.c $ ./a.out Enter the Row size for Matrices(1-100): 3 Enter the Column Size for Matrices(1-100): 3 Enter Elements for the First Matrix(X) of Size 3X3: 10 20 30 40 50 60 70 80 90 Enter Elements for the Second Matrix(Y) of Size 3X3: 10 20 30 40 50 60 70 80 90 Given Matrices X & Y are Equal $ |
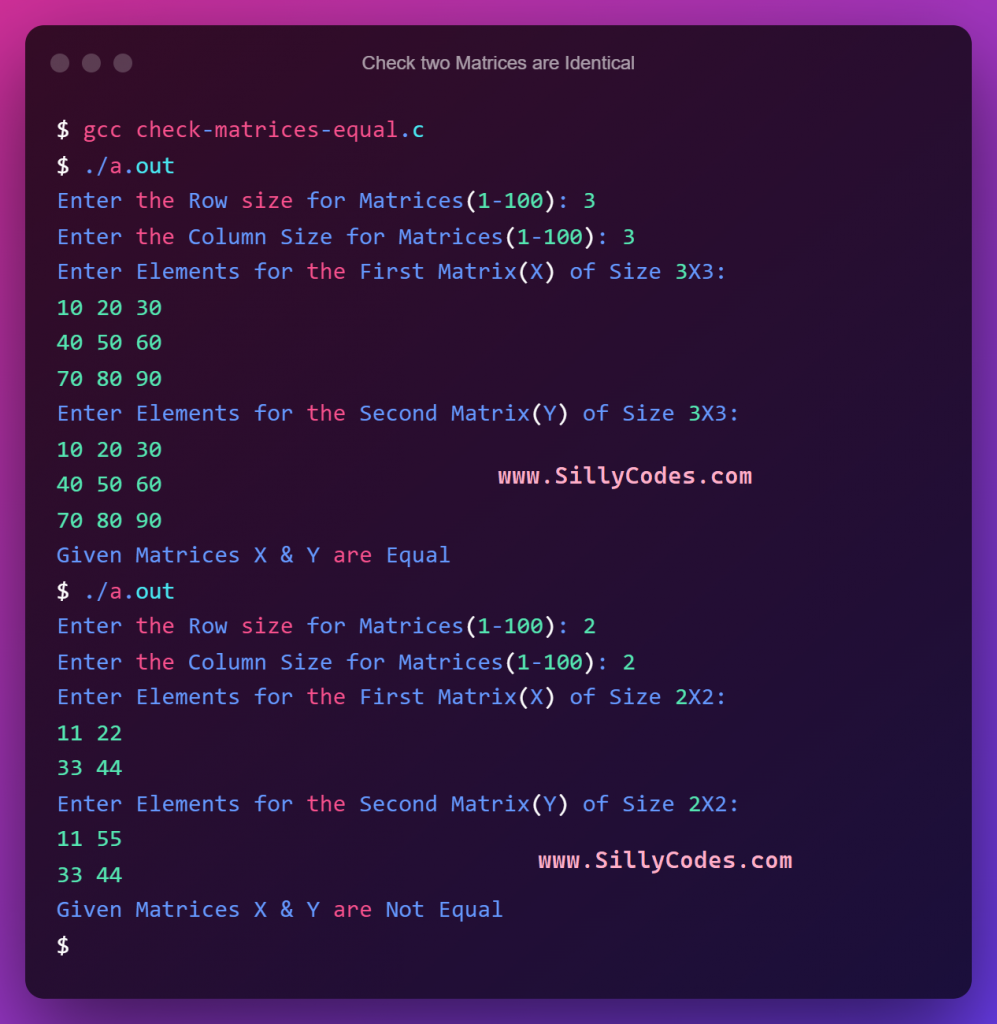
As we can see from the above output, Given Matrices X and Y are Identical.
1 2 3 4 5 6 7 8 9 10 11 |
$ ./a.out Enter the Row size for Matrices(1-100): 2 Enter the Column Size for Matrices(1-100): 2 Enter Elements for the First Matrix(X) of Size 2X2: 11 22 33 44 Enter Elements for the Second Matrix(Y) of Size 2X2: 11 55 33 44 Given Matrices X & Y are Not Equal $ |
If you observe the above output, Element X[0][1] and Y[0][1] are different, So Matrices X and Y are not equal or Not Identical.
Test 2: Negative cases:
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter the Row size for Matrices(1-100): 900 Enter the Column Size for Matrices(1-100): 8 Invalid Rows or Columns size, Please Try again $ ./a.out Enter the Row size for Matrices(1-100): 4 Enter the Column Size for Matrices(1-100): 599 Invalid Rows or Columns size, Please Try again $ |
If the user enters the row size or columns which is beyond the current limit, Then program displays an error message Invalid Rows or Columns size, Please Try again)
Check Two Matrices are Identical using Functions in C:
We have used a single function (i.e main() function) to check if if the two matrices are identical, But it is advised to use the functions to perform a specific task. In the above program, we have a repeating task, reading matrix input. We can create a function to read the matrix elements, and we can call the function as many times as we want. Let’s rewrite the above program and use the functions.
Here is the modified version of the above program with three user-defined functions. Except the main() function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 |
/* Program to Check if the two Matrices are equal using Functions SillyCodes.com */ #include <stdio.h> // ROWS and COLUMNS max size const int ROWS=100; const int COLUMNS=100; // Function declaration int isEqual(int rows, int columns, int X[rows][columns], int Y[rows][columns]); /** * @brief - Read Matrix from the user and update 'A' * * @param rows - Rows in Matrix(2Darray) * @param columns - Columns in 'A' * @param A[][] - 'A' is Matrix or 2D array */ void readMatrix(int rows, int columns, int A[rows][columns]) { int i, j; for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { //printf("A[%d][%d]: ", i, j); scanf("%d", &A[i][j]); } } } /** * @brief - Print the elements of the Matrix * * @param rows - Rows in Matrix(2Darray) * @param columns - Columns in 'A' * @param A[][] - 'A' is a Matrix or 2D array */ void displayMatrix(int rows, int columns, int A[rows][columns]) { int i, j; for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // Print Elements printf("%d ", A[i][j]); } printf("\n"); } } int main() { // Declare two matrices. // 'X' & 'Y' are Input matrices int X[ROWS][COLUMNS], Y[ROWS][COLUMNS]; int rows, columns, i, j; // Take the Row size and Column size from user printf("Enter the Row size for Matrices(1-%d): ", ROWS); scanf("%d", &rows); printf("Enter the Column Size for Matrices(1-%d): ", COLUMNS); scanf("%d", &columns); // check for array bounds if(rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) { printf("Invalid Rows or Columns size, Please Try again \n"); return 0; } // Take the user input for 1st Matrix - 'X' printf("Enter Elements for the First Matrix(X) of Size %dX%d: \n", rows, columns); readMatrix(rows, columns, X); // Take the user input for 2nd Matrix - 'Y' printf("Enter Elements for the Second Matrix(Y) of Size %dX%d: \n", rows, columns); readMatrix(rows, columns, Y); // Check if the Matrix "X" and "Y" are equal int result = isEqual(rows, columns, X, Y); // Print the Result if(result == 0) { printf("Given Matrices X & Y are Not Equal\n"); } else { printf("Given Matrices X & Y are Equal\n"); } return 0; } /** * @brief - CHeck Matrices pointed by 'X' and 'Y' are equal * * @param rows - Rows in the Matrices * @param columns - Columns in the Matrices * @param X - First Matrix * @param Y - Second Matrix * @return int - 1 if 'X' and 'Y' are equal, Otherwise 0 */ int isEqual(int rows, int columns, int X[rows][columns], int Y[rows][columns]) { int i, j; // Traverse each element in the Arrays and compare the values. // Iterate over rows for(i=0; i<rows; i++) { // Iterate over the columns for(j=0; j<columns; j++) { // check if the values are not equal if(X[i][j] != Y[i][j]) { // Matrices "X" and "Y" are not equal return 0; } } } // If we reached till here, Then Matrices are equal. return 1; } |
We have defined three user-defined functions(except the main()) in the above program.
- The
readMatrix() function – Used to read the elements from the user and update the given matrix
- Function Prototype – void readMatrix(int rows, int columns, int A[rows][columns])
- The readMatrix() function takes three formal arguments which are rows, columns, and Matrix A.
- This function uses Two For loops to iterate over the given matrix A and updates its elements by using the scanf function – scanf(“%d”, &A[i][j]);
- The readMatrix() function doesn’t return anything back to the caller. So return type is void.
- As the arrays are passed by the address/reference, All the changes made to the Matrix-A in the readMatrix() function will be visible in the Caller Function (i.e main() function).
- The
displayMatrix() function is used to print the Matrix elements on the Standard Output.
- The prototype of displayMatrix function is void displayMatrix(int rows, int columns, int A[rows][columns])
- Similar to the readMatrix() function, The displayMatrix() function also takes three formal arguments. i.e rows, columns, and A[][] (2D Array)
- The displayMatrix() function uses Two For loops to iterate over the A matrix and print the elements of the matrix. – printf("%d ", A[i][j]);
- The
isEqual() function – This function checks if the
Matrix-X and
Matrix-Y are Identical / Equal.
- Function Prototype – int isEqual(int rows, int columns, int X[rows][columns], int Y[rows][columns])
- This function takes Four Formal Arguments which are rows, columns, matrix X, and matrix Y.
- The isEqual() function uses two for loops to iterate over the elements of the Matrices X and Y and does the element-wise comparison – if(X[i][j] != Y[i][j])
- This function returns an Integer ( int). If the both Matrices X and Y are equal, Then isEqual() function return One(1), Otherwise, it returns Zero(0)
Program Output:
Compile the program
$ gcc check-matrices-equal.c
Run the Program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
$ ./a.out Enter the Row size for Matrices(1-100): 4 Enter the Column Size for Matrices(1-100): 3 Enter Elements for the First Matrix(X) of Size 4X3: 1 2 3 4 5 6 7 8 9 10 11 12 Enter Elements for the Second Matrix(Y) of Size 4X3: 1 2 3 4 5 6 7 8 9 10 11 12 Given Matrices X & Y are Equal $ $ $ ./a.out Enter the Row size for Matrices(1-100): 2 Enter the Column Size for Matrices(1-100): 3 Enter Elements for the First Matrix(X) of Size 2X3: 44 66 88 55 77 99 Enter Elements for the Second Matrix(Y) of Size 2X3: 44 22 88 55 99 77 Given Matrices X & Y are Not Equal $ |
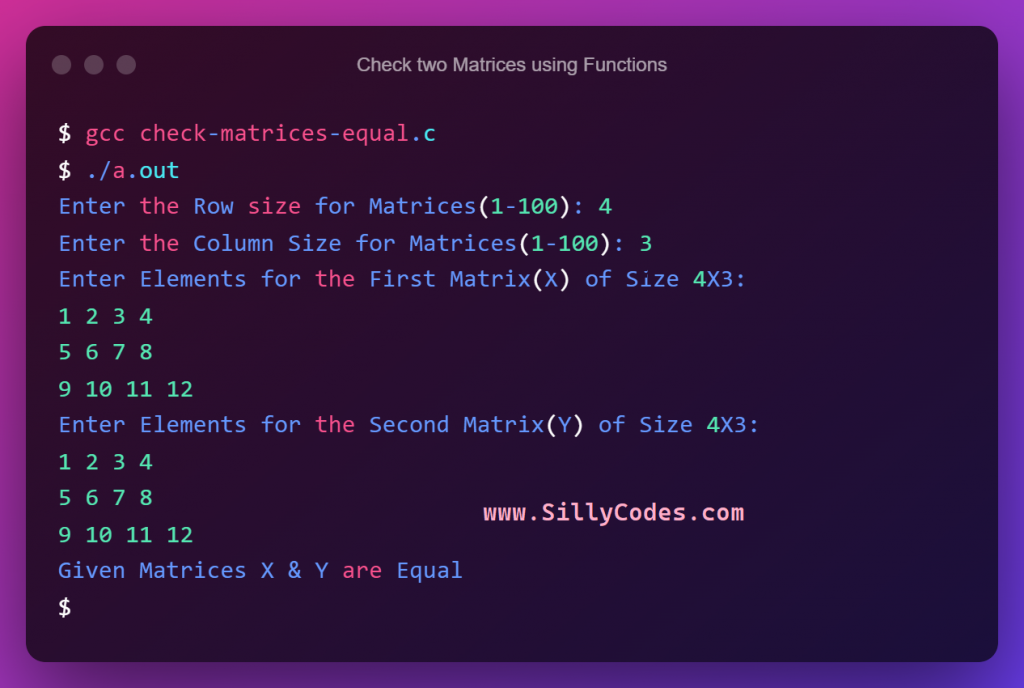
From the above Output, We can see the first attempt where Both matrices are Identical and in the second attempt, Matrices are not equal.
1 2 3 4 5 |
$ ./a.out Enter the Row size for Matrices(1-100): 6000 Enter the Column Size for Matrices(1-100): 5 Invalid Rows or Columns size, Please Try again $ |
Related Matrix Programs:
- C Program to Read and Print Matrix or 2D Array
- C Program to demonstrate How to Pass 2D Array to Functions
- C Program to Multiplication of two Matrices
- C Program to Add Two Matrices
- C Program to Subtract Two Matrices
- C Program to Calculate the Transpose of Matrix
Related Array Programs:
- C Program to Merge Two Arrays
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
- C Program to Delete Duplicate Elements in an Array
- C Program to Left Rotate Array by ‘N’ Times
- C Program to Right Rotate Array Elements by ‘N’ Positions
- Linear Search in C Language with Example Programs
1 Response
[…] C Program to Check Two Matrices are Equal […]