Calculate Product of Digits using Recursion in C
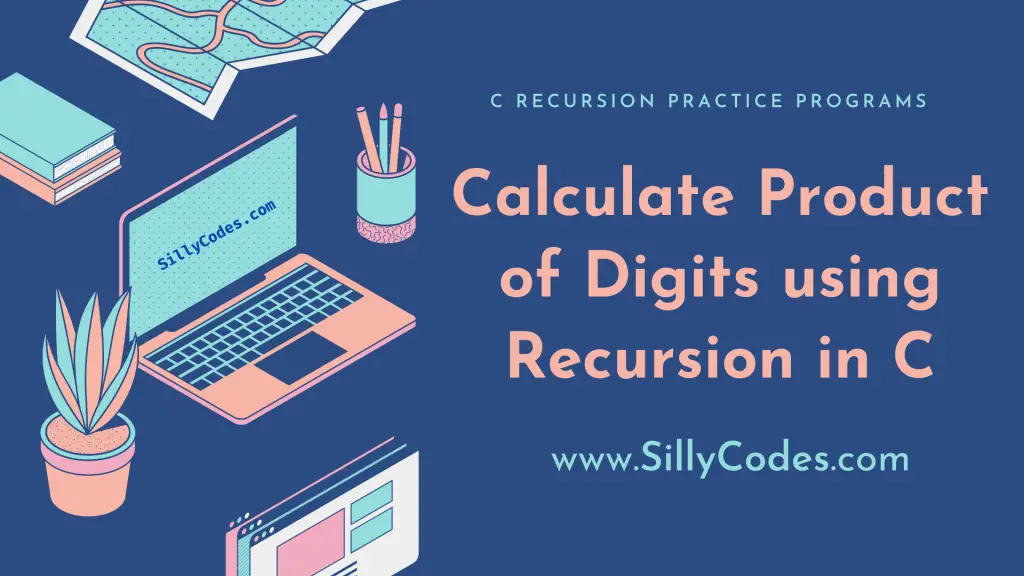
Product of Digits using Recursion in C Program Description:
Write a Program to find the Product of Digits of a number using Recursion in C programming language, The program should take an integer number as input and calculate the product of its digits.
Excepted Input and Output:
Input:
Enter a Number : 1245
Output:
Product of digits of number 1245 is : 40
Pre-Requisites:
It is recommended to have basic knowledge of functions and recursion in C language. Please check out the following articles.
- How to Define, Declare and call functions in C Langauge with Example Programs
- How Recursion works in Programming with Step by step explanation
Product of Digits using Recursion in C Program Algorithm/Explanation:
- Start the program by taking the user input. Store the input value in a variable called num.
- Check for negative numbers using the if(num <= 0) condition. Use Goto Statement to ask for the input again.
- Create a function called
prodOfDigits. The
prodOfDigits function takes an integer variable as input and calculates the product of digits of the given number using recursion and returns the result back to the caller.
- The prodOfDigits uses recursion to calculate the product of each digit. So we need to have a base condition for recursion. which is num == 0. If we reach the base condition, return 1.
- If the
num is not equal to zero.
- Get the last digit of the num using Modulus Operator ( num % 10)
- Make a recursive call to prodOfDigits(num/10) function and get the return value.
- Multiply the above two results. – ( (num % 10) * prodOfDigits(num / 10) )
- Note that we are using num/10 in a recursive call to prodOfDigits function, The division operation ( num/10) removes the last digit from the num, As we already used the last digit in the calculation of the product.
- The above recursive calls continue till we reach the base condition.
- Call the prodOfDigits function from the main function and pass the input number num. Store the return value in product variable.
- Display the results on the console.
▶️Related Program: Product of Digits of a Number using Iterative Method
Product of Digits using Recursion in C Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
/* Program: Product of Digits of a number using Recursion */ #include<stdio.h> /** * @brief - Calculate the sum of digits of number 'n' * * @param num - input number * @return int - sum of digits of number */ int prodOfDigits(int num) { // Our Base Condition if(num == 0) return 1; /* Get the last number using modulus operator Then call the prodOfDigits by removing last number by using division operator. */ return ( (num % 10) * prodOfDigits(num / 10) ); } // main function int main() { int num; START: // goto label // Take Input printf("Enter a Number : "); scanf("%d", &num); // check for negative number if(num <= 0) { printf("Invalid Input, Please try again \n"); goto START; } // function call int product = prodOfDigits(num); // Print Results printf("Product of digits of number %d is : %d \n", num, product); return 0; } |
📢 If you are testing with large numbers, Please use the long and long long int datatype for the num, prod variables. so that the product won’t reach integer limits.
Program Output:
Compile the program
$ gcc product-of-digits-recursion.c
Run the Program
Test Case 1: Positive Numbers
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter a Number : 546 Product of digits of number 546 is : 120 $ ./a.out Enter a Number : 1245 Product of digits of number 1245 is : 40 $ ./a.out Enter a Number : 1 Product of digits of number 1 is : 1 |
Test Case 2: Numbers with Zeros
1 2 3 4 5 6 7 |
// Number contains zero $ ./a.out Enter a Number : 10 Product of digits of number 10 is : 0 $ ./a.out Enter a Number : 243590 Product of digits of number 243590 is : 0 |
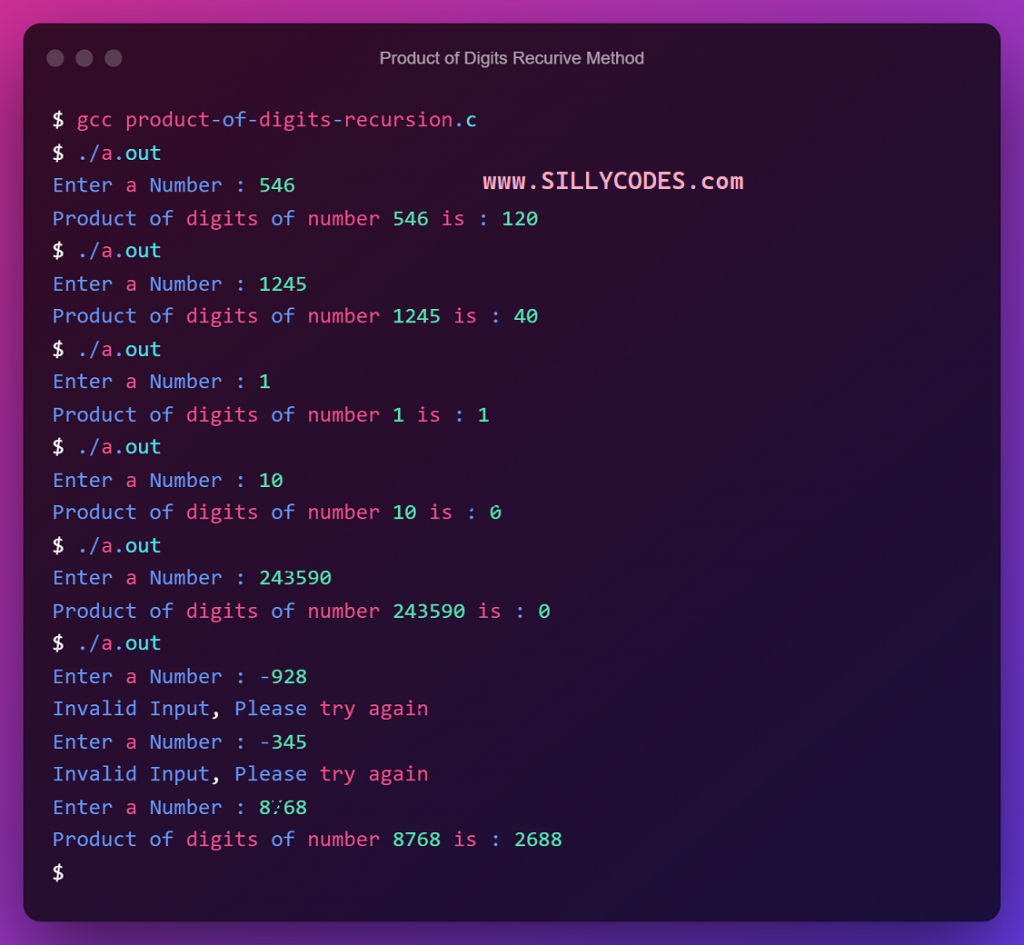
As we can see from the above output, We are getting the desired results.
Test Case 3: Negative Numbers
1 2 3 4 5 6 7 8 9 |
// Negative Number $ ./a.out Enter a Number : -928 Invalid Input, Please try again Enter a Number : -345 Invalid Input, Please try again Enter a Number : 8768 Product of digits of number 8768 is : 2688 $ |
The user will be prompted by the application until a correct input number is given.
More Recursion Practice Programs:
- C Program to Find Factorial of Number using Recursion
- C Program to Generate Fibonacci Number using Recursion.
- C Program to calculate the sum of Digits of Number using Recursion
- C Program to Print First N Natural Numbers using Recursion
- C Program to find sum of Fist N Natural Number using Recursion
- Product of N natural Number using Recursion C Program
Related Functions Practice Programs:
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
- 100+ C Practice Programs
- C Tutorials Index
2 Responses
[…] C Program to Product the Number of digits in a Number using Recursion […]
[…] C Program to Product the Number of digits in a Number using Recursion […]