Count Number of Alphabets, Digits, and Special characters in string in C
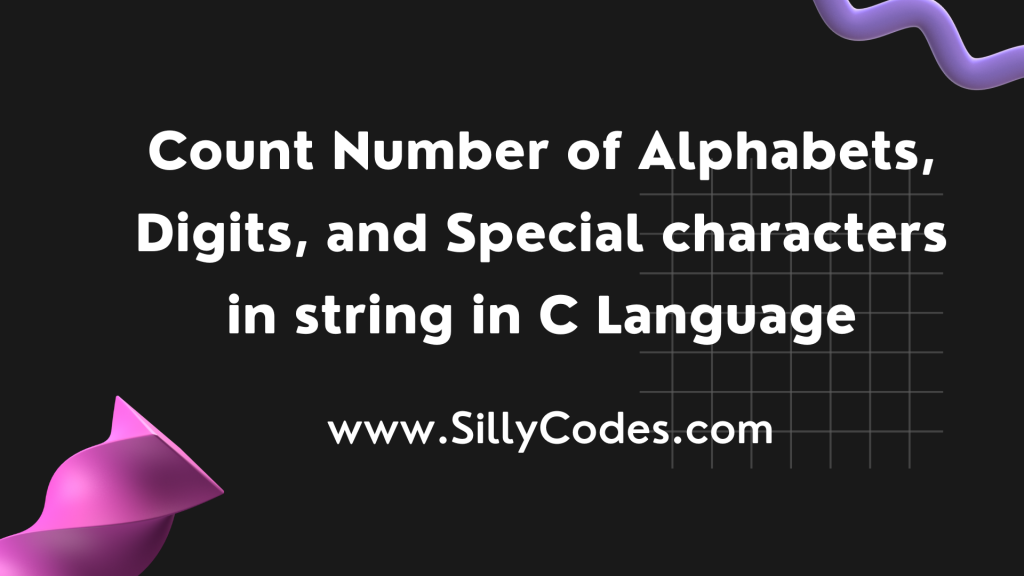
Program Description:
Write a Program to Count Number of Alphabets, Digits, and Special characters in string in C programming language. The program should accept a string from the user and count the number of Alphabets, Digits, White spaces, and Other characters/special characters in the given string.
📢 This Program is part of the C String Practice Programs series
Excepted Input and Output:
Input:
Enter the first string : This is @ll Fantasy 2000
Output:
Number of Alphabets: 15
Number of Digits: 4
Number of WhiteSpaces: 4
Number of Other Characters: 1
Prerequisites:
It is recommended to go through the following articles to better understand this program.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
Count Number of Alphabets, Digits, and Special characters in a string in C Program Explanation:
- Start the program by declaring a string and naming it as inputStr with the size of 100 characters (We are using a global constant to store the SIZE)
- Take the input string from the user and update the inputStr string variable.
- Initialize the variables to store the total number of Alphabets( nAlpha), Digits( nDigits), White spaces( nWhiteSpaces), and Special Characters( nSpecialChars) with Zeros(0).
- The Logic to count the Number of Alphabets, Digits, and Special characters in a String is to Traverse through the string character by character and check if the character is Alphabet, Digit, white space, or special character. We are going to use the functions from the ctype.h header file to verify the characters.
- Start a For Loop and Iterate over the string. – for(i = 0; inputStr[i]; i++). At each iteration,
- Check if the
inputStr[i] is an Alphabet:
- Call the isaplha(inputStr[i]) function with the inputStr[i]. The isalpha() function checks the given character and returns a non-zero value if it is an Alphabet. So If the inputStr[i] is an alphabet, Then Increment the nAlpha variable.
- Check if the
inputStr[i] is a Digit:
- Call the isdigit(inpuStr[i]) function with the input string element. The isdigit() function checks the given character and returns a non-zero value if the inputStr[i] is a Digit. So increment the nDigits variable if the inputStr[i] is a Digit.
- Check if the
inputStr[i] is a White Space:
- Similarly, Call the isspace(inputStr[i]) function and increment the nWhitespaces variable if isspace() function returns non-zero value.
- If none of the above conditions are met, Then the character is a other character / special character. Increment the nSpecialChars variable.
- Check if the
inputStr[i] is an Alphabet:
- Once the above loop is completed, The nAlpha, nDigits, nWhiteSpaces, and nSpecialChars contains the number of Alphabets, Digits, White space, and Special characters respectively.
- Display the results on Console.
Program to Count Number of Alphabets, Digits, and Special characters in string in C:
Here is the C Program to count Alphabets, Digits, Whitespaces, and Special Characters.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
/*     program to count the number of alphabets, Digits, white space, and spacial characters in a string     sillycodes.com */  #include<stdio.h> #include<ctype.h>  const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     int i;      // get the string from the user     printf("Enter the first string : ");     // scanf("%s", inputStr);     gets(inputStr);         // Init the 'nAlpha' and 'nDigits' with Zero     int nAlpha = 0;     int nDigits = 0;     int nWhiteSpaces = 0;     int nSpecialChars = 0;      for(i = 0; inputStr[i]; i++)     {                if(isalpha(inputStr[i]))         {             // Alphabet             nAlpha++;         }         else if(isdigit(inputStr[i]))         {             // Digits.             nDigits++;         }         else if(isspace(inputStr[i]))         {             // White Space             nWhiteSpaces++;         }         else         {             // Other, Might be special character             nSpecialChars++;         }      }         // print the results     printf("Number of Alphabets: %d\n", nAlpha);     printf("Number of Digits: %d\n", nDigits);     printf("Number of WhiteSpaces: %d\n", nWhiteSpaces);     printf("Number of Other Characters: %d\n", nSpecialChars);      return 0; } |
📢Don’t forget to include the ctype.h header file
Program Output:
Let’s compile and Run the program using GCC compiler (Any compiler)
Test 1:
1 2 3 4 5 6 7 8 |
$ gcc alpha.c $ ./a.out Enter the first string : This is @ll Fantasy 2000Â Â Â Â Number of Alphabets: 15 Number of Digits: 4 Number of WhiteSpaces: 4 Number of Other Characters: 1 $ |
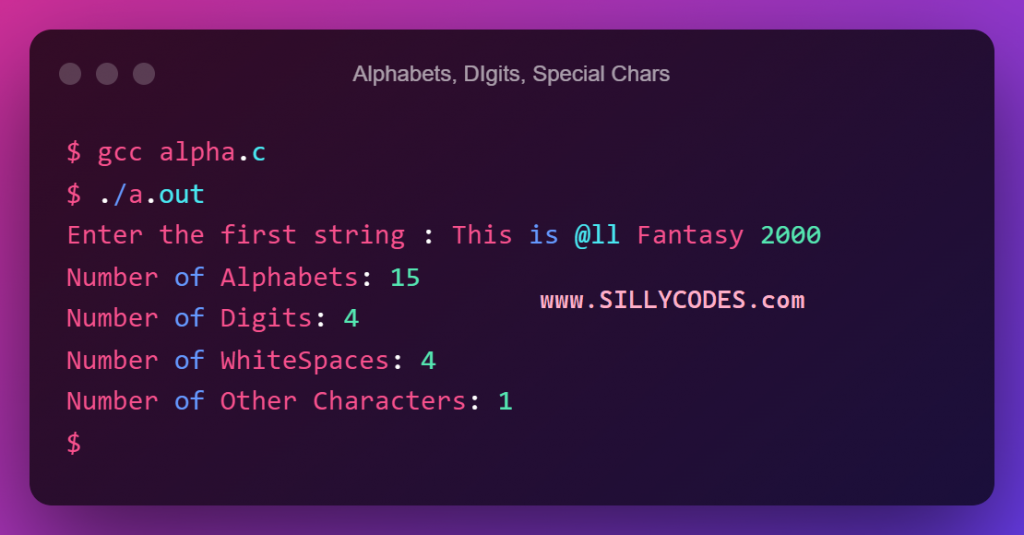
As we can see from the above output, The input string This is @ll Fantasy 2000. We have 15 Alphabets, 4 Digits, 4 White spaces, and 1 Special character.
Let’s try a few more examples.
Test 2:
1 2 3 4 5 6 7 |
$ ./a.out Enter the first string : Learn Programming at SillyCodes.com Number of Alphabets: 31 Number of Digits: 0 Number of WhiteSpaces: 4 Number of Other Characters: 1 $ |
In this case, We have 31 Alphabets, Zero digits, 4 Whitespaces, and 1 Special character in Learn Programming at SillyCodes.com input string.
Test 3:
1 2 3 4 5 6 7 |
$ ./a.out Enter the first string : C-Lang 7482 #*$& Python Number of Alphabets: 11 Number of Digits: 4 Number of WhiteSpaces: 3 Number of Other Characters: 5 $ |
As we can see from the above input and output, The program is properly counting the number of Alphabets, Digits, Whitespaces, and Special Characters in the input string.
1 Response
[…] C Program to Count Alphabets, Digits, Whitespaces in String […]