Count Frequency of Characters in String in C Language
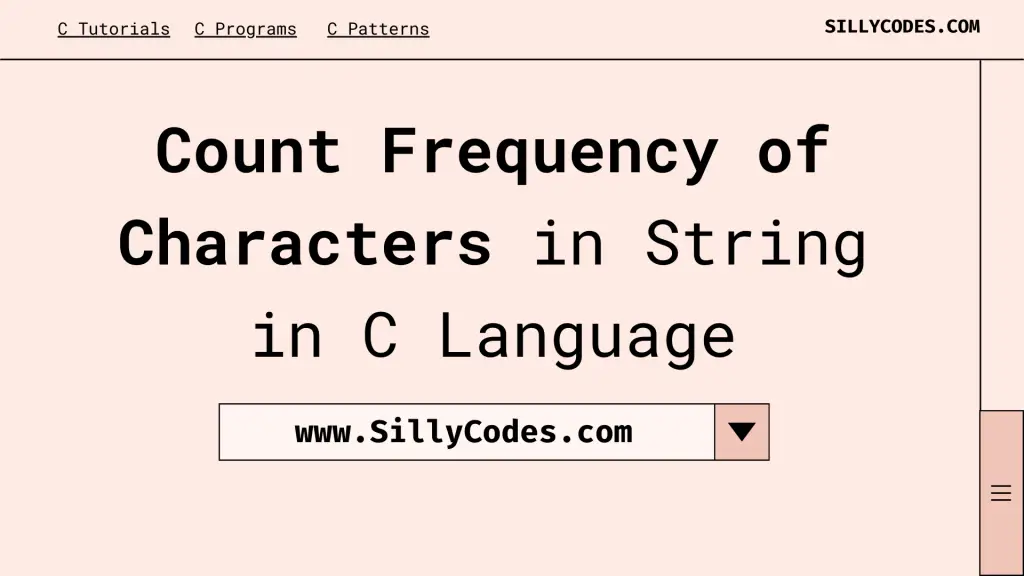
Program Description:
Write a Program to Count Frequency of Characters in String in C Programming language. The program should accept a string from the user and count the frequency of each character in the given string. This program is used to know how many times each character is present in the string.
We are going to look at the few methods to count the character frequencies and each program will be followed by a detailed step-by-step explanation of the program and program output. We also included the instructions to compile and run the program.
📢 This Program is part of the C String Practice Programs series
Example Input and Output:
Input:
Enter a string : www.SillyCodes.com
Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
Frequency of Characters in given string : Character : '.' - Frequency: 2 Character : 'C' - Frequency: 1 Character : 'S' - Frequency: 1 Character : 'c' - Frequency: 1 Character : 'd' - Frequency: 1 Character : 'e' - Frequency: 1 Character : 'i' - Frequency: 1 Character : 'l' - Frequency: 2 Character : 'm' - Frequency: 1 Character : 'o' - Frequency: 2 Character : 's' - Frequency: 1 Character : 'w' - Frequency: 3 Character : 'y' - Frequency: 1 |
The program will also count the frequency of the special characters.
Prerequisites:
It is recommended to know the C-Strings, C-Arrays, and Functions in C to better understand the following program.
We will look at the two different methods to count the frequency of characters in a string in c.
- Iterative Method
- Using User-defined Functions
Algorithm to Count Frequency of Characters in String in C:
The main idea to count the frequency of characters in a string is to maintain a frequencyarray/frequency string. The frequency string will have 256 characters. Why 256 characters? because it is the number of ASCII values. i.e – frequency[256]
Iterate through the all characters of the Input string and update the frequencyarray.
frequency[inputStr[i]]++;
For example, If the input string has a character a, Then we will update the frequency array at the index 97.
frequency[‘a’]
The ASCII equivalent of the ‘a’ is 97. So the count of the character ‘a’ will be stored at the 97th index of the frequencyarray.
frequency[97]
Initially, Frequency array elements are initialized with zeroes. The character index (i.e 97th here) will be incremented based on the input string characters.
frequency[97]++;
This way each character frequency will be updated in the frequency array. Finally, Display the frequency of each character on the console.
Let’s look at the step-by-step explanation of the program.
Count Frequency of Characters in a String in C Program Explanation:
- Start the Program by defining Two Macros named SIZE and ASCIISIZE with 100 and 256 values respectively.
- Declare two string to store the input string( inputStr) and Frequency of characters( frequency) string. – i.e char inputStr[SIZE]; and char frequency[ASCIISIZE] = {0};
- Read the input string from the user using the gets function and store it in inputStr string.
- To count the frequency of characters in a string, Iterate over the string and update the frequency array based on the characters.
- Create a For loop to Iterate over the string. Start the loop from i=0 and go till the terminating NULL character. i.e
for(i = 0; inputStr[i]; i++)
- At each iteration, update the frequency array based on the ASCII value of the inputStr[i] – i.e frequency[inputStr[i]]++;
- Once the above loop is completed, The frequency array will contain the frequency of each character in the given string.
- Iterate over the frequency array and print the frequencies using another loop
- Stop the program.
Program to Count Frequency of Characters in String in C Iterative Method:
Here is the program to check the frequency of each character in a string
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
/* program to Count Frequency of characters in a String in c sillycodes.com */ #include<stdio.h> // Max Size of string #define SIZE 100 #define ASCIISIZE 256 int main() { // declare a string with 'SIZE' char inputStr[SIZE]; int i; // Create a frequency string - Stores count based on ASCII number of characters char frequency[ASCIISIZE] = {0}; // get the string from the user printf("Enter a string : "); gets(inputStr); // Iterate through all characters of string // update the frequency array for(i = 0; inputStr[i]; i++) { // Update the frequency Array. // frequency['a'] = frequency[97] = element at 97th index will be incremented. frequency[inputStr[i]]++; } // Print the Frequency of characters printf("Frequency of Characters in given string : \n"); for(i = 0; i<ASCIISIZE; i++) { if(frequency[i] != 0) { printf("Character : '%c' - Frequency: %d\n", i, frequency[i]); } } return 0; } |
Program Output:
Let’s compile and Run the Program using your compiler. We are using GCC compiler in Ubuntu Linux OS.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
$ gcc count-frequency.c $ ./a.out Enter a string : www.SillyCodes.com Frequency of Characters in given string : Character : '.' - Frequency: 2 Character : 'C' - Frequency: 1 Character : 'S' - Frequency: 1 Character : 'c' - Frequency: 1 Character : 'd' - Frequency: 1 Character : 'e' - Frequency: 1 Character : 'i' - Frequency: 1 Character : 'l' - Frequency: 2 Character : 'm' - Frequency: 1 Character : 'o' - Frequency: 2 Character : 's' - Frequency: 1 Character : 'w' - Frequency: 3 Character : 'y' - Frequency: 1 $ |
Example 2:
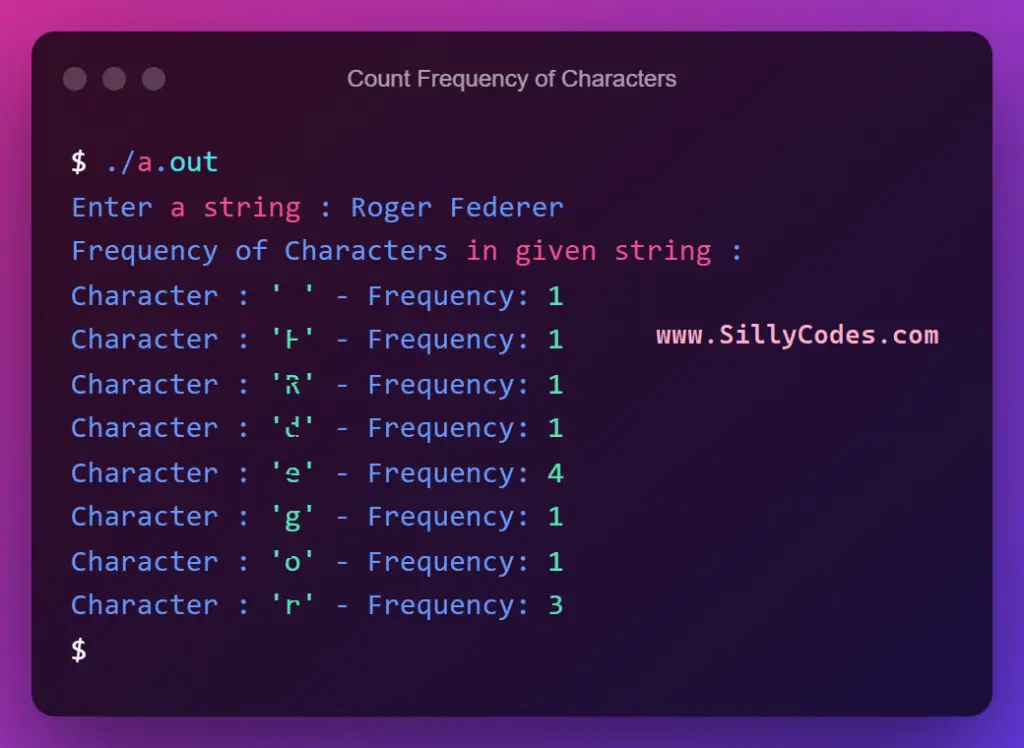
1 2 3 4 5 6 7 8 9 10 11 12 |
$ ./a.out Enter a string : Roger Federer Frequency of Characters in given string : Character : ' ' - Frequency: 1 Character : 'F' - Frequency: 1 Character : 'R' - Frequency: 1 Character : 'd' - Frequency: 1 Character : 'e' - Frequency: 4 Character : 'g' - Frequency: 1 Character : 'o' - Frequency: 1 Character : 'r' - Frequency: 3 $ |
As we can see from the above output, The program is properly counting the frequencies of the characters in the given string.
Count Frequency of Characters in String in C using a user-defined function:
In this method, We are going to move the frequency counting logic to a user-defined function, So that we can reuse the code and also functions improve the readability of the program.
Let’s rewrite the above C program and use a function to print the frequencies of the character in a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
/* program to Count Frequency of characters in a String in c sillycodes.com */ #include<stdio.h> // Max Size of string #define SIZE 100 #define ASCIISIZE 256 /** * @brief - Print the frequencies of each characters in a string ('str') * * @param str - Input String */ void printFrequencies(char *str) { int i; // Create a frequency string - Stores count based on ASCII number of characters char frequency[ASCIISIZE] = {0}; // Iterate through all characters of string // update the frequency array for(i = 0; str[i]; i++) { // Update the frequency Array. // frequency['a'] = frequency[97] = element at 97th index will be incremented. frequency[str[i]]++; } // Print the Frequency of characters printf("Frequency of Characters in given string : \n"); for(i = 0; i<ASCIISIZE; i++) { if(frequency[i] != 0) { printf("Character : '%c' - Frequency: %d\n", i, frequency[i]); } } } int main() { // declare a string with 'SIZE' char inputStr[SIZE]; // get the string from the user printf("Enter a string : "); gets(inputStr); // call the printFrequencies funciton. printFrequencies(inputStr); return 0; } |
In the above program, We have defined a function named printFrequencies. The printFrequencies function takes a string as formal argument and counts the character frequencies of the input string.
Here is the prototype of the printFrequencies() function.
void printFrequencies(char *str)
In the main() function, Get the input string from the user and store it in inputStr string. Then call the printFrequencies() function with the inputStr to print the frequencies of each character in the inputStrstring.
printFrequencies(inputStr);
This printFrequencies function doesn’t return anything. So return type of the function is void
Program Output:
Compile and Run the program.
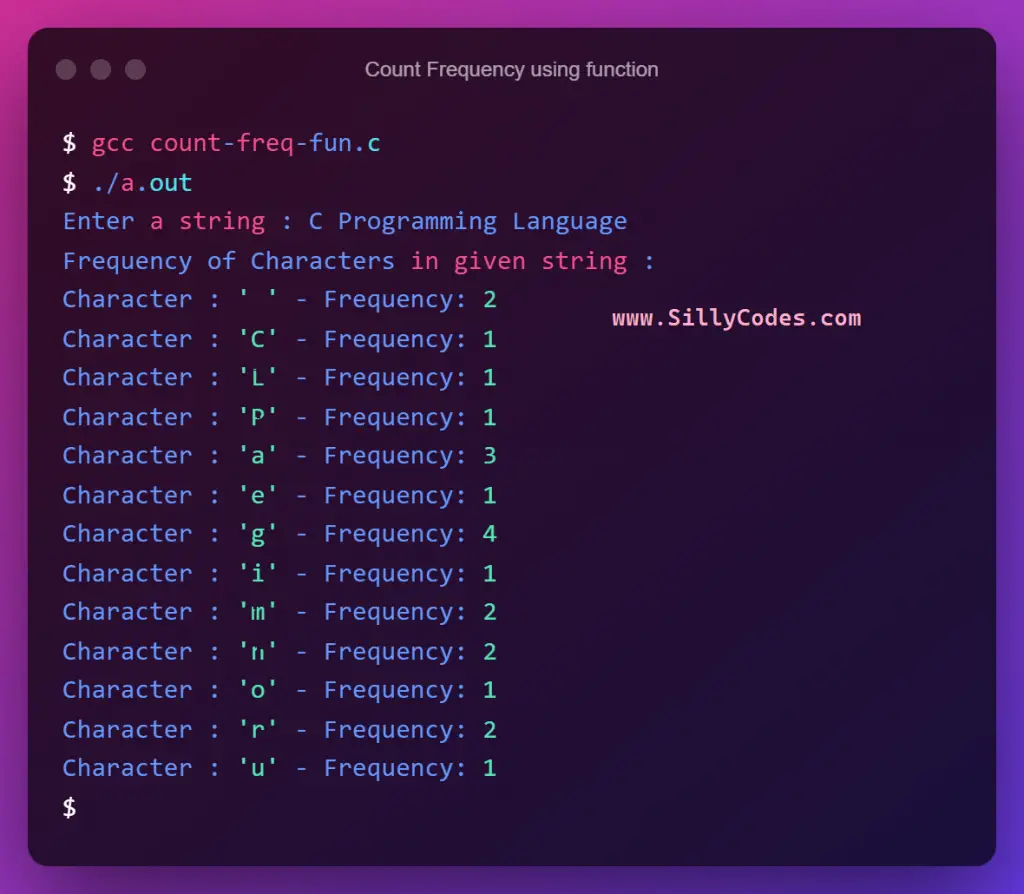
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
$ gcc count-freq-fun.c $ ./a.out Enter a string : C Programming Language Frequency of Characters in given string : Character : ' ' - Frequency: 2 Character : 'C' - Frequency: 1 Character : 'L' - Frequency: 1 Character : 'P' - Frequency: 1 Character : 'a' - Frequency: 3 Character : 'e' - Frequency: 1 Character : 'g' - Frequency: 4 Character : 'i' - Frequency: 1 Character : 'm' - Frequency: 2 Character : 'n' - Frequency: 2 Character : 'o' - Frequency: 1 Character : 'r' - Frequency: 2 Character : 'u' - Frequency: 1 $ |
We have provided the input string as C Programming Language and the program calculated the frequencies of each character and printed on the console.
Related String Programs:
- C Tutorials Index
- C Programs Index
- C Program to Calculate Length of the string
- C Program to Copy a String to new string
- C Program to Compare Two Strings
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
2 Responses
[…] C Program to Count Frequencies of each character in a string […]
[…] C Program to Count Frequencies of each character in a string […]