Factorial program in C using function
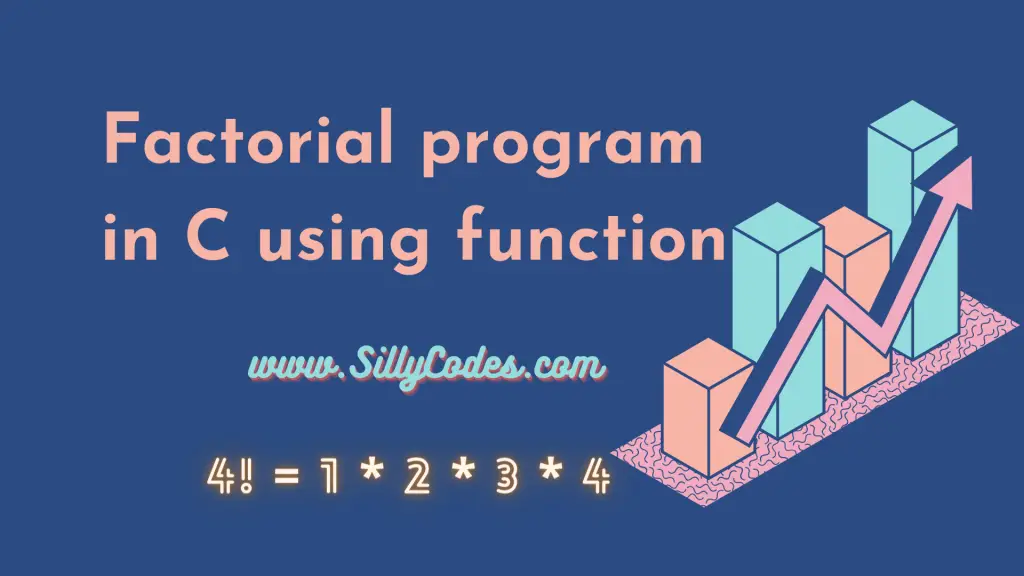
Program Description:
We are going to look at the Factorial program in C using function in this article, The program will accept an Integer number from the user and display the factorial of a given number. The program shouldn’t accept negative numbers.
Factorial of a Number:
The Factorial is the product of all positive integers less than or equal to n. ( product of all numbers from 1 to n )
For example, a Factorial of a number 4 is
4! = 1 * 2 * 3 * 4 = 24
Example Input and Output:
Example 1: Input:
Enter a Number: 9
Output:
Factorial of 9 number is : 362880
Example 2: Input:
Enter a Number: 6
Output:
Factorial of 6 number is : 720
Pre-Requisites:
It is recommended to know the basics of the C functions and loops. Please go through the following articles to familiarize yourself with the above concepts
Related Program: Factorial of Number using Loops
Factorial program in C using function Algorithm:
- Take a integer number from the user and store it in variable num1.
- We won’t allow the negative numbers for factorial, So display an error message to the user and prompt for the input again.
- Create a function named
factorial. The
factorial function takes one integer number as input and calculates the factorial of a given number. Here is the prototype of the factorial function.
- int factorial(int);
- function_name: factorial
- argument_list : int
- return_type : int
- Call the factorial function by passing the num1. As the factorial function returns an integer variable, collect the return value using the variable result.
- The factorial function uses a Loop to calculate the factorial.
- Create two variables i and fact. Initialize the fact variable with 1.
- The loop starts from 1 and goes all the way up to the given number num1 ( We use i to control the loop)
- At each iteration, We multiply the fact variable with loop counter i. – i.e fact *= i;
- Once all iterations are completed, The fact variable contains the factorial value of num1. So return the fact variable.
- Display the result on console.
Factorial program in C using function
Let’s convert above algorithm in to the C program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
/* Program: Factorial program in c using function Author: SillyCodes.com */ #include<stdio.h> /* Description: 'factorial' function calculates the factorial of 'num1' arguments_list: (int) return_type: (int) */ int factorial(int num1) { // init variables int i, fact = 1; // calculate the factorial for(i = 1; i <= num1; i++) { fact = fact * i; // fact *= i; } // return the results return fact; } int main() { // Take user input int num1, result; START: // goto statemetn label. printf("Enter a Number: "); scanf("%d", &num1); if(num1 < 0) { printf("Error: Please enter a positive number\n"); // take back to input stage goto START; } // call the factorial function, Store return value in 'result' result = factorial(num1); // print the result printf("Factorial of %d number is : %d \n", num1, result); return 0; } |
Program Output – Factorial program in C using function:
Let’s compile the program using the GCC compiler.
gcc factorial-func.c
The above command generates the executable file called a.out. Run the executable file.
Test Case 1: Postive Numbers:
1 2 3 4 |
$ ./a.out Enter a Number: 4 Factorial of 4 number is : 24 $ |
1 2 3 4 |
$ ./a.out Enter a Number: 9 Factorial of 9 number is : 362880 $ |
1 2 3 4 |
$ ./a.out Enter a Number: 6 Factorial of 6 number is : 720 $ |
The program is calculating the factorial value correctly.
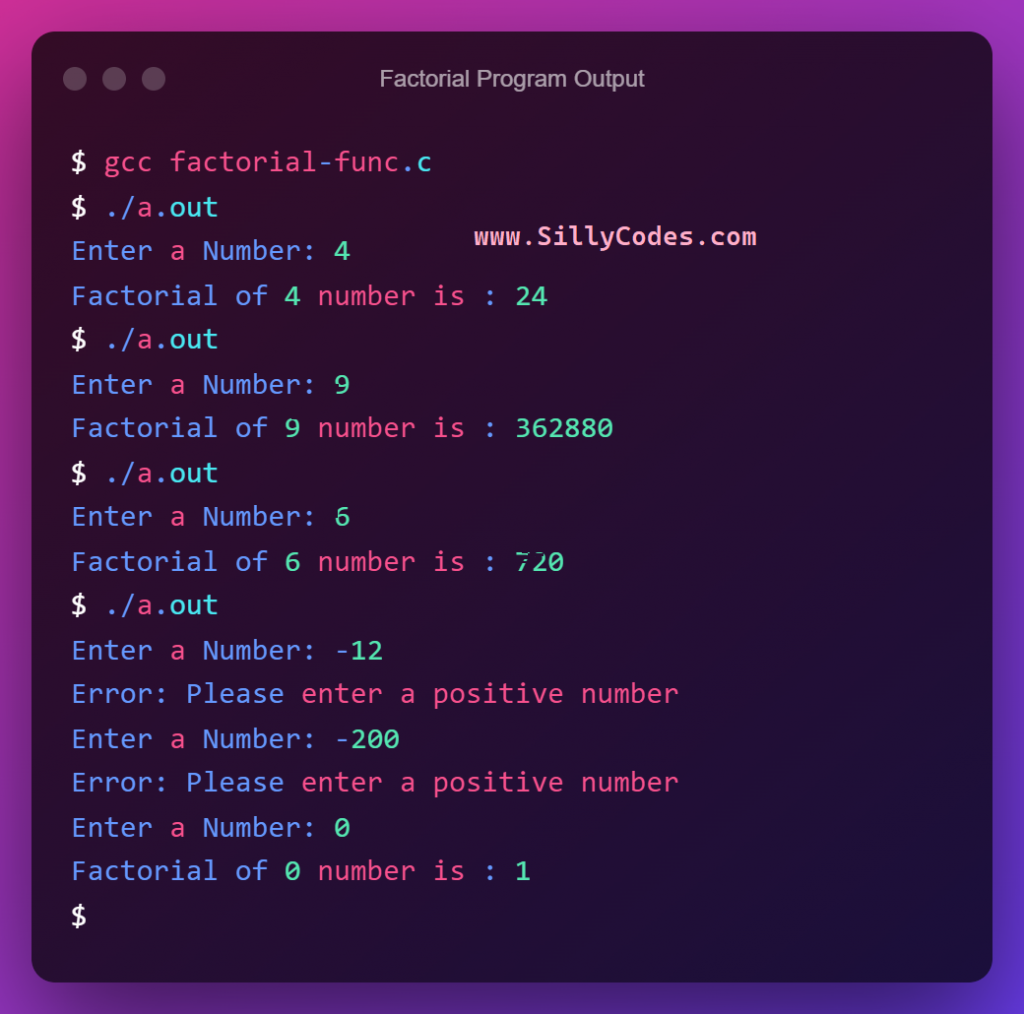
Test Case 2: Negative numbers:
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Number: -12 Error: Please enter a positive number Enter a Number: -200 Error: Please enter a positive number Enter a Number: 0 Factorial of 0 number is : 1 $ |
If the user enters a negative number, As expected, The program is displaying the error message ( Error: Please enter a positive number) and prompting for the input again. The program will prompt the user until user provide a valid number.
We used the Goto statement to prompt the user for input until a valid number is entered.
Related Program:
- All C Program List [Index]
- C Program to Print Numbers in Reverse Order
- C Program to Calculate Sum of First N natural numbers
- C Program to Reverse a Number
- C Program to Calculate Sum of Digits of a Number
- C Program to Calculate Product of Digits of a Number
- C Program to Count the number of Digits of a Number
- C Program to Multiply without using Multiplication operator (*)
- C Program to generate Fibonacci Series up to a given number
- C Program to Print First n Fibonacci numbers
- C Program to get Nth Fibonacci number
- C Program to Check number is Prime Number or not?
3 Responses
[…] Factorial of a Number using functions […]
[…] Factorial of a Number using functions […]
[…] Factorial of a Number using functions […]