Delete Duplicates from Sorted Array in C Language
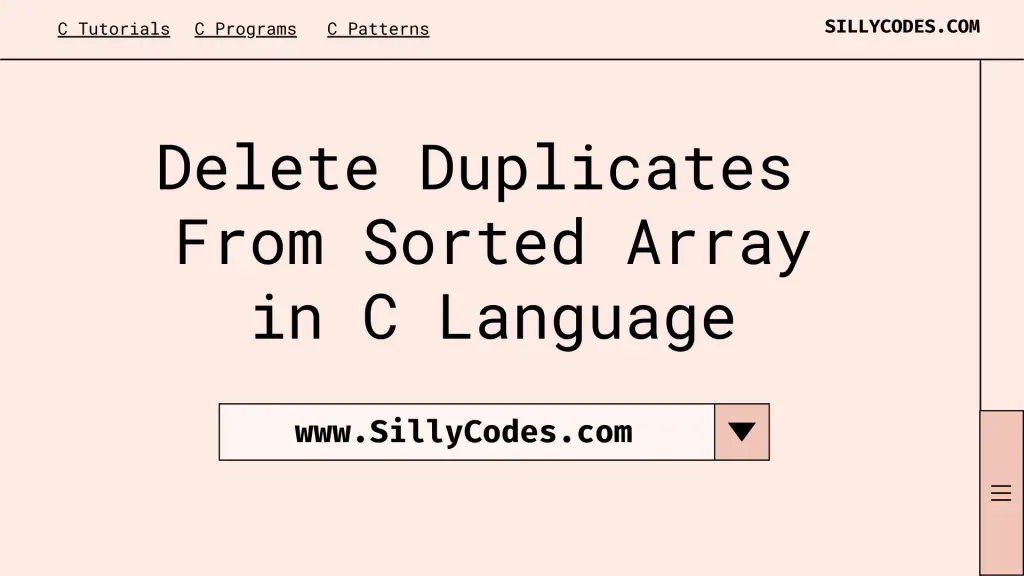
Delete Duplicates from Sorted Array in C Program Description:
We have written the count number of unique elements program in our earlier article, In today’s article, We are going to look at the program to Delete Duplicates from Sorted Array in C programming language. The program should accept a Sorted Array from the user, The input array may contain duplicate elements but the array should be in sorted order, So the program will delete all duplicate elements from the given array.
📢This program is part of the Array Practice Programs in C Language
Excepted Input and Output of the Program:
Input:
Enter desired array size(1-1000): 8
Please enter array elements(8) in ascending order : 1 1 1 1 2 3 4 4
Output:
Original Array (Sorted): 1 1 1 1 2 3 4 4
Array After removing Duplicates: 1 2 3 4
If you observe the above input and output, The user provided a sorted array 1 1 1 1 2 3 4 4, the array has few duplicate and the program removed all duplicate elements and returned the result as 1 2 3 4 array.
Program Prerequisites:
We are going to use arrays and functions, So it is a good idea to know about Arrays in C, Functions in C, and Passing Arrays to Functions
Let’s look at the step-by-step explanation of the Delete Duplicate elements from an array C program.
Delete Duplicates from Sorted Array in C Explanation:
- Declare an array named values with the size of 1000. ( Change the 1000, if you want to use large arrays). We can use a Macro or Const Integer to hold the 1000 but we are directly using it.
- Take the desired array size from the user and update the size variable.
- Check if the size is out of bounds using if(size >= 1000 || size <= 0) condition and display an error message if the size is out of bounds.
- Now, We need to prompt the user to provide the input array. Update the input array elements with user-provided values.
- Use a For Loop to iterate over the array and update the values[i] element.
- Print the Original Array i.e numbers array on the console for comparison. ( Use another for loop and traverse the array and print elements)
- Now we need to delete the duplicate elements from the sorted array, As the array is already sorted, The similar elements(numbers) will be adjacent to each other. So if the present element and the next element in the array are equal, Then skip the element and go to the next iteration
- Initialize variables
i=0,
j=0. Start a for loop from
i=0 to i<size-1, At each iteration,
- Check if the values[i] is not equal to the values[i+1], If both are not equal, Then Only update the array values array.
- So if (values[i] != values[i+1]) is true, Then update values[j++] = values[i];
- Continue the loop till the i reaches size-2.
- Finally, update the values[j++] = values[size-1];
- Initialize variables
i=0,
j=0. Start a for loop from
i=0 to i<size-1, At each iteration,
- Once the above steps are completed, Then the values array will only contain the distinct or unique elements.
- Display the resultant array on the console using another for loop.
Program to Delete Duplicates from Sorted Array in C Language:
Here is the C Program to Delete Duplicates from a sorted array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
/* Program to delete duplicate elements from sorted array in C Author: SillyCodes.com */ #include <stdio.h> int main() { // declare the 'values' array int values[1000]; int i, j, size; printf("Enter desired array size(1-1000): "); scanf("%d", &size); // check for array bounds if(size >= 1000 || size <= 0) { printf("Invalid Size, Try again \n"); return 0; } // User input for array elements printf("Please enter array elements(%d) in ascending order : ", size); for(i = 0; i < size; i++) { // printf("values[%d] : ", i); scanf("%d", &values[i]); } // Print the original array printf("Original Array (Sorted): "); for(i = 0; i < size; i++) { printf("%d ", values[i]); } printf("\n"); // Remove the duplicate elements j = 0; for (i=0; i < size-1; i++) { if (values[i] != values[i+1]) { values[j++] = values[i]; } } values[j++] = values[size-1]; // Print the Modified array // "Note": Loop only till 'j' printf("Array After removing Duplicates: "); for(i = 0; i < j; i++) { printf("%d ", values[i]); } printf("\n"); return 0; } |
Program Output:
Let’s compile and Run the program using your favorite compiler (we are using GCC compiler on ubuntu linux)
Compile the Program
$ gcc delete-dups-sorted-arr.c
Run the executable file
Test 1: Normal Cases.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
$ ./a.out Enter desired array size(1-1000): 10 Please enter array elements(10) in ascending order : 1 2 2 3 3 3 6 6 9 9 Original Array (Sorted): 1 2 2 3 3 3 6 6 9 9 Array After removing Duplicates: 1 2 3 6 9 $ ./a.out Enter desired array size(1-1000): 8 Please enter array elements(8) in ascending order : 1 1 1 1 2 3 4 4 Original Array (Sorted): 1 1 1 1 2 3 4 4 Array After removing Duplicates: 1 2 3 4 $ ./a.out Enter desired array size(1-1000): 5 Please enter array elements(5) in ascending order : 6 6 7 7 7 Original Array (Sorted): 6 6 7 7 7 Array After removing Duplicates: 6 7 $ |
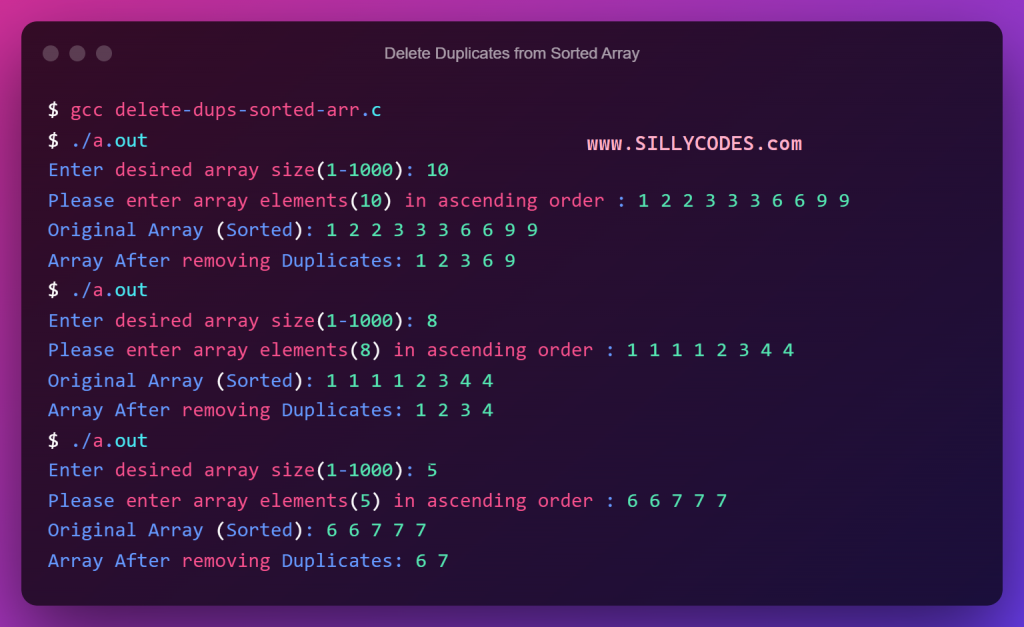
The program is properly removing the duplicate elements from the sorted array.
For example, When we provided the 6 6 7 7 7 as the input array, The program removed all duplicate elements and returned the 6 7 Array as output.
Test 2: Negative Cases
1 2 3 4 5 6 7 |
$ ./a.out Enter desired array size(1-1000): 1200 Invalid Size, Try again $ ./a.out Enter desired array size(1-1000): 0 Invalid Size, Try again $ |
As we can see from the above output, The program is providing an error message(i.e Invalid Size, Try again) if the user enters the wrong size.
Related Array Programs:
- C Program to Find Maximum Element in Array
- C Program to Find Second Largest Element in Array
- C Program to Insert an element in Array
- C Program to Delete an Element in Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
2 Responses
[…] our previous article, We have looked at the program to Delete All duplicate elements in a sorted array. In today’s article, We are going to write a Program to Merge Two Arrays in C programming […]
[…] C Program to Delete Duplicate Elements from Sorted Array […]