Program to check Armstrong number in C using function
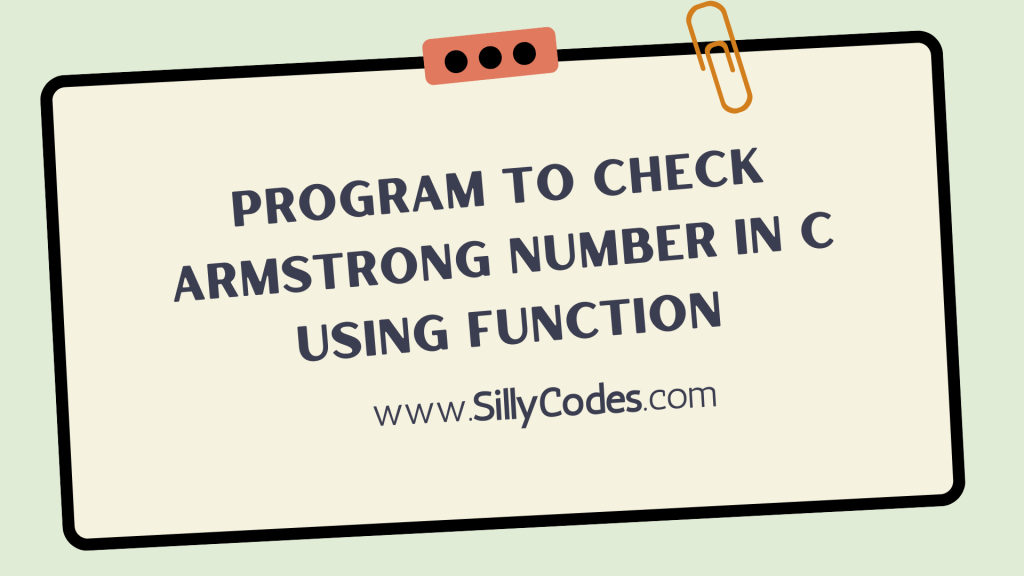
Armstrong Number in C using function Program Description:
Write a C Program to check the Armstrong number in C using function with the program algorithm. The program should accept an integer number from the user and display if it is an Armstrong number or not using a C function.
Example Input:
Enter a Number: 93084
Output:
93084 is an armstrong number
What is an N-Digit Armstrong Number?
A positive number of n digits is said to be an Armstrong number of order n, If the sum of the power of n of each digit in the number is equal to the original number itself.
If the number is abcd is Armstrong number, Then it should satisfy the below equation.
abcd = pow(a, n) + pow(b, n) + pow(c, n) + pow(d, n)
Where n is the number of digits in the given number. here the number is abcd, So the value of n is 4.
Pre-Requisites:
We are going to use the functions to check the Armstrong number, So it is recommended to know the basics of the following topics.
- Functions in C Language with Example Programs
- Loops in C Language – While Loop, For loop
- Modulus Operator in C
- Division Operator in C
Armstrong Number in C using function Program Algorithm:
- Take the input from the user and store it in a variable num.
- Check if the num is a negative number, If so display an error message to the user
- As we discussed earlier, We need to calculate power(eachDigit, nDigit).
- We need to Calculate the Number of digits in the number. Which is used to calculate the power. We used a function countDigits and passed the input number num to calculate the number of digits and countDigits function returns the number of digits in the number( num).
- Then we have defined a function named isArmstrong, The isArmstrong function takes an integer number ( num) as input and returns a boolean. If the given number is an Armstrong number, then isArmstrong function returns true, Otherwise, it returns false.
- To check the Armstrong number, we need to go through each digit of the given number
num using the Loop.
- At Each Iteration,
- Get the digit from the number i.e num – rem = temp %10;
- Calculate the power of rem with nDigits(Number of digits in num). We are using the Power function from the math.h header file – pow(rem, nDigits); to calculate the power. We can also use Custom Power Function.
- Update the arm variable. – arm = arm + <strong>pow</strong>(rem, nDigits);
- Remove the processed digit using the temp/10 operation. – temp = temp/10;
- At Each Iteration,
- Once the above loop is terminated, check the value of
arm number. If the
arm variable is equal to
num, Then given the number
num is N-digit Armstrong Number.
- If arm == num, return true
- otherwise, return false
- In the main function, We are using the if statement to check the result of isArmstrong function and printing the result based on the return value.
Armstrong Number in C using function Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 |
/* Program to check Armstrong Number in C using Function */ #include<stdio.h> #include<math.h> // for 'pow' function #include<stdbool.h> // for 'bool' /** * @brief : Calculate number of digits in 'num' return it * * @param num (integer) * @return int - Number of digits in 'num' */ int countDigits(int num) { // digitCnt holds the number of digits int digitCnt = 0; int temp = num; // Iterate over each digit using division operator. while(temp > 0) { digitCnt++; // remove the last element using division operator. temp = temp / 10; } // got number of digits return it return digitCnt; } /** * @brief : Check if given number 'num' is Armstrong number or not * * @param num (integer) * @return true - if 'num' is an Armstrong number * @return false - if 'num' is not an armstrong number */ bool isArmstrong(int num) { int arm, rem, temp; // Take backup of number 'num' temp = num; // count number of digits in the 'num' // Use - https://sillycodes.com/program-to-count-number-of-digits-in-c/ int nDigits = countDigits(num); // Go through the each digit and calculate the pow(digit, nDigits) while(temp>0) { // get the last digit of number or current digit using modulus rem = temp %10; // calculate the power of 'rem' with 'nDigits' // Use - https://sillycodes.com/program-to-find-power-of-number-in-c/ arm = arm + pow(rem, nDigits); // Remove the last digit by dividing the 'temp' by '10' temp = temp/10; } // After all iterations, The 'arm' variable contains the sum of powers of each digit. // if 'arm' is equal to the input number 'num', Then the 'num' is Armstrong number. if(arm == num) return true; else return false; } int main() { int num; // Take the user input printf("Enter a Number: "); scanf("%d", &num); // Negative number check if(num <= 0) { printf("Error: Please enter a Positive Number\n"); return 0; } // Call the 'isArmstrong()' function pass 'num' if(isArmstrong(num)) printf("%d is an armstrong number\n", num); else printf("%d is not an armstrong number\n", num); return 0; } |
Here are the prototype details of the isArmstrong function
- function_name: isArmstrong
- argument_list: int
- return_type: bool
As we are using the bool type, We need to include the stdbool.h header file. We are also using the pow function, So we need to include the math.h as well.
Program Output:
Compile and run the program, Don’t forget to include the -lm compilation option. ( as we are using the math.h file, we need to include the math library)
Compile the program
$ gcc armstrong-func.c -lm
The above command generates a.out executable file. Let’s run the executable using the ./a.out command.
Test Case 1: Positive Numbers ( Armstrong numbers and non-Armstrong numbers test)
1 2 3 4 |
$ ./a.out Enter a Number: 407 407 is an armstrong number $ |
As we can see from the above output, The number 407 is an Armstrong number. Let’s check a few more numbers
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter a Number: 151 151 is not an armstrong number $ ./a.out Enter a Number: 153 153 is an armstrong number $ ./a.out Enter a Number: 93084 93084 is an armstrong number $ ./a.out Enter a Number: 45667 45667 is not an armstrong number $ |
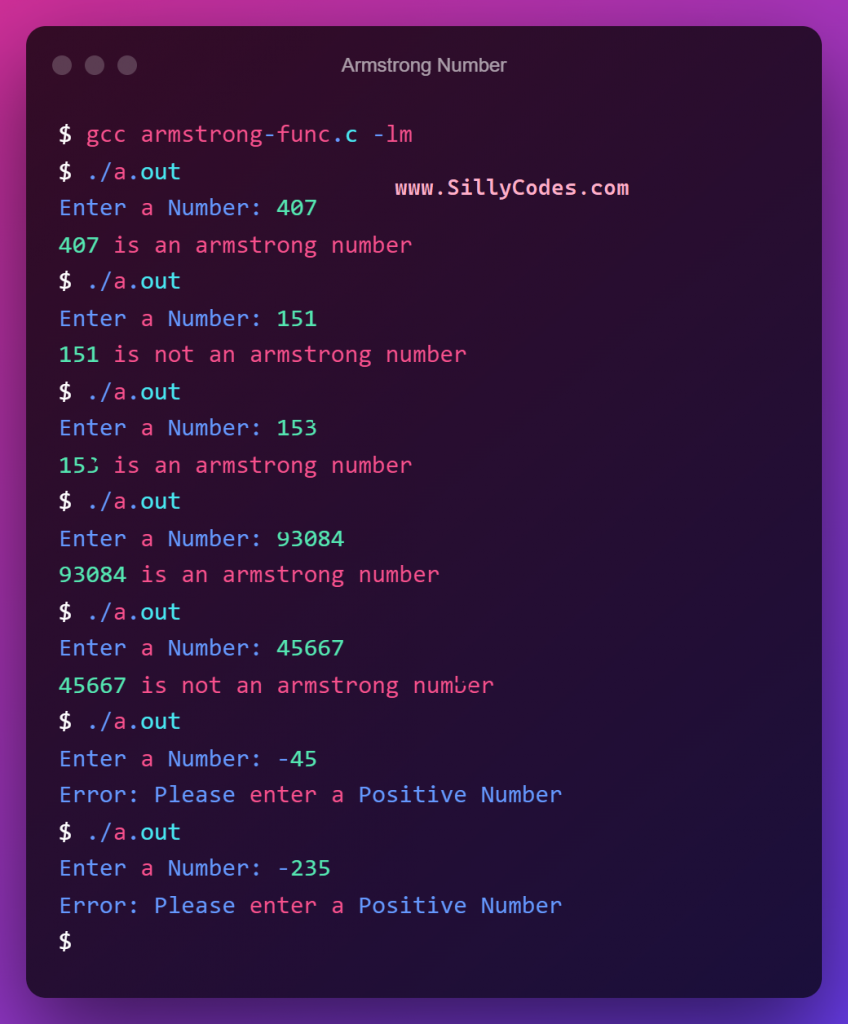
Test Case 2: Pass the Negative Numbers:
1 2 3 4 5 6 7 |
$ ./a.out Enter a Number: -45 Error: Please enter a Positive Number $ ./a.out Enter a Number: -235 Error: Please enter a Positive Number $ |
As expected, The program is displaying the error on negative numbers. Here we are terminating the program if the user enters a negative number, We can use the do while loop or Goto Statement to ask the user input again.
Related Programs:
- Fibonacci series using functions in C
- C Program to Calculate Nth Prime Number
- C Program Check Prime Number [Mutliple Methods]
- C Program to Convert Decimal Number to Binary Number
- C Program to Convert Binary Number to Decimal Number
- C Program to Convert Octal Number to Decimal Number
- C Program to Calculate the Factorial of Number
- C Program to Calculate the Power of Number
- C Program to Check Number is Power of 2
- C Program to find power of number without Loops
- C Program to Find all Factors of a Number
- C Program to find all Prime Factors of number
- C Program to Calculate the GCD or HCF of Two Number
3 Responses
[…] Armstrong Number using Function in C language […]
[…] Armstrong Number using Function in C language […]
[…] Armstrong Number using Function in C language […]