Program to Check Sparse Matrix in C Language
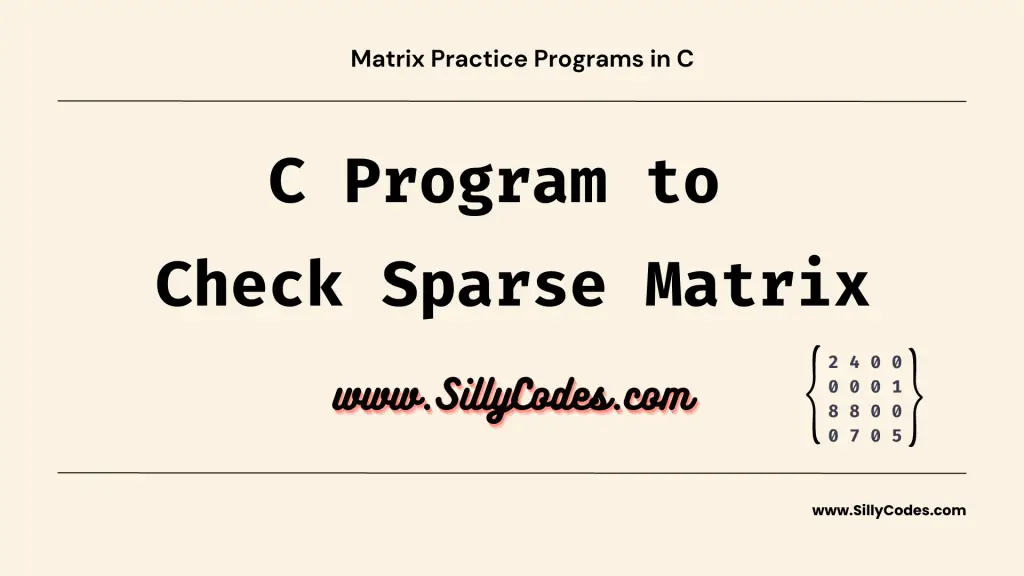
Program Description:
Write a Program to Check Sparse Matrix in C programming language. The program should accept a Matrix (2D array) from the user and check whether the matrix is a Sparse Matrix or not.
Prerequisites:
It is recommended to know how to create arrays and multi-dimensional arrays(Matrices) in C. Please go through the following articles to learn more
- Multi-Dimensional Arrays in C
- Read and Print 2D Arrays in C
- Passing 2D Arrays to Functions
- One dimensional Arrays in C
before looking at the example inputs and output of the program, Let’s understand what is a sparse matrix.
What is a Sparse Matrix?
A sparse Matrix is a Matrix that contains a very small number of non-zero elements. So The elements of the sparse matrix is dominated by the Zeros.
We can call a matrix a Sparse Matrix, If the total number of elements with a value equal to zero is greater than the ((rows * columns)/2) value.
For example, We have a Matrix X with the dimensions rows x columns. Where the rows is the total number of rows in the Matrix-X and columns is the total number of Columns in the Matrix-X.
Let’s say the variable nZeros holding the total number of zeros in the Matrix-X
Then We can call the Matrix-X a Sparse Matrix, If nZeros > ((rows * columns)/2) condition is satisfied.
Example Input and Output:
Input:
1 2 3 4 5 6 7 8 |
$ ./a.out Enter the Row size for Matrix(1-100): 4 Enter the Column Size for Matrix(1-100): 4 Enter Elements for the Matrix(X) of Size 4X4: 2 4 0 0 0 0 0 1 8 8 0 0 0 7 0 5 |
Output:
1 2 |
Given Matrix is a Sparse Matrix Matrix had 9 of Zeroes among the 16 elements |
Let’s look at the program to Check the Sparse Matrix in C programming language.
📢This program is part of the Array Practice Programs Series.
Check Sparse Matrix in C Program Explanation:
Here is a step-by-step explanation of the program.
- Create two integer constants named ROWS and COLUMNS, Which holds the max number of rows and columns in the Matrix. (Modify this number if you want to use large matrices)
- Start the Program by declaring a Matrix (2D Arrays) named X (Matrix-X). The row size and column size of the matrices are ROWS and COLUMNS constants respectively.
- Initialize the nZeros variable with Zero(0).
- Prompt the user to provide the desired Row size and Column Size, and store them in the variables rows and columns respectively. (Note, These rows and columns are small letters – C is Case Sensitive language)
- Check for array bounds using the (rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) condition, And display an error message if the row/column sizeis beyond the present limits.
- Take the user input for Input Matrix i.e Matrix-X, We need to use two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the columns
- Create the First For Loop ( Outer Loop), Start the iteration from i=0 and go till the i<rows (that is for(i=0; i<rows; i++) ). At Each iteration of the Outer Loop,
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). The Inner Loop will iterate over the columns. At each iteration of the Inner Loop
- Prompt the user to provide the Matrix element and Read the element using the scanf function and Update the X[i][j] element.
- Check if the X[i][j] element is equal to zero ( use (X[i][j] == 0)), If the element is equal to Zero(0), Then increment the nZeros variable – nZeros++
- Repeat the above step for all elements in the Matrix-X
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). The Inner Loop will iterate over the columns. At each iteration of the Inner Loop
- Once the above Step 7 is completed, The nZero variable contains the total number of Zeroes in the given Matrix.
- Now, To Check if Matrix X is a Sparse Matrix, We need to check the
(nZeros > ((rows * columns) / 2)) condition.
- If the above condition is true, Then the input matrix (Matrix-X) is a Sparse Matrix.
- If it is false, Then the Matrix-X is not a Sparse Matrix.
- Display the results on the console.
Program to Check Sparse Matrix in C Language:
Let’s convert the above algorithm to C Program
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
/*     Program to Check Sparse Matrix in C     SillyCodes.com */  #include <stdio.h>  // Define MAX ROWS and COLUMNS const int ROWS=100; const int COLUMNS=100;  int main() {     // Declare two matrices.     // 'X' is Input matrice     int X[ROWS][COLUMNS];     int rows, columns, i, j;     // create 'nZeros' and intialize with '0'     int nZeros = 0;      // Take the Row size and Column size from user     printf("Enter the Row size for Matrix(1-%d): ", ROWS);     scanf("%d", &rows);     printf("Enter the Column Size for Matrix(1-%d): ", COLUMNS);     scanf("%d", &columns);      // check for array bounds     if(rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0)     {         printf("Invalid Rows or Columns size, Please Try again \n");         return 0;     }      // Take the user input for 1st Matrix - 'X'     printf("Enter Elements for the Matrix(X) of Size %dX%d: \n", rows, columns);     for(i=0; i<rows; i++)     {         for(j=0; j<columns; j++)         {             // printf("X[%d][%d]: ", i, j);             scanf("%d", &X[i][j]);              // check if the element is Zero             if (X[i][j] == 0)             {                 // increment counter 'nZeros'                 nZeros++;             }         }     }       // check if the Matrix is Sparse Matrix     if(nZeros > ((rows * columns) / 2))     {         printf("Given Matrix is a Sparse Matrix\n");         printf("Matrix had %d of Zeroes among the %d elements\n", nZeros, rows*columns);     }     else     {         printf("Given Matrix is not a Sparse Matrix\n");     }      return 0; } |
Program Output:
Compile and Run the Program using GCC compiler (Any compiler)
To compile the program use following command.
$ gcc check-sparse-mat.c
Run the Program.
Test Case 1: Normal Cases:
1 2 3 4 5 6 7 8 9 10 11 |
$ ./a.out Enter the Row size for Matrix(1-100): 4 Enter the Column Size for Matrix(1-100): 4 Enter Elements for the Matrix(X) of Size 4X4: 2 4 0 0 0 0 0 1 8 8 0 0 0 7 0 5 Given Matrix is a Sparse Matrix Matrix had 9 of Zeroes among the 16 elements $ |
If we observe the output of the above program, The given matrix contains 9 Zeroes out of total 16 elements. The total number of zeroes are greater than the ((rows * columns) / 2). So above matrix is a Sparse Matrix.
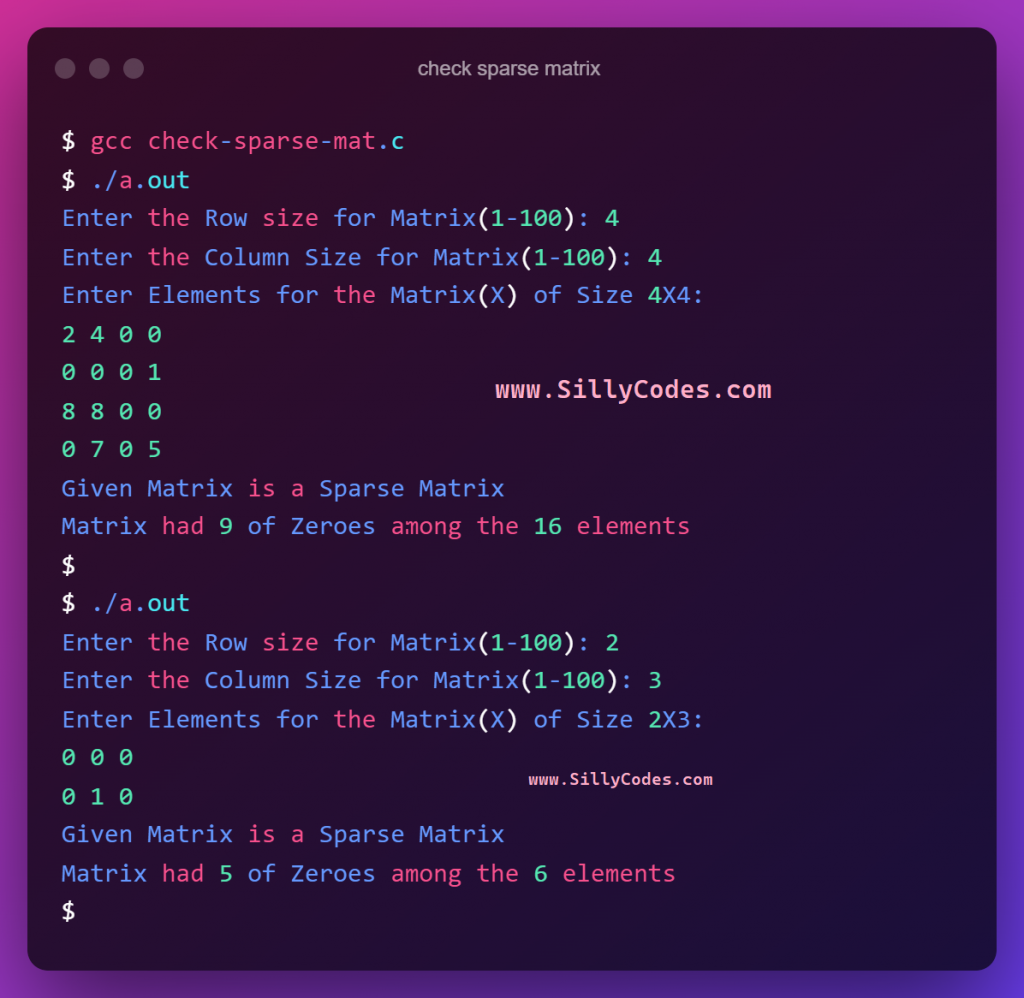
Let’s check few more example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
$ ./a.out Enter the Row size for Matrix(1-100): 2 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 2X3: 0 0 0 0 1 0 Given Matrix is a Sparse Matrix Matrix had 5 of Zeroes among the 6 elements $ $ ./a.out Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 4 Enter Elements for the Matrix(X) of Size 3X4: 1 8 8 4 0 0 2 0 9 4 0 0 Given Matrix is not a Sparse Matrix $ |
As we can see from the above output, The program is generating the desired results.
Test 2: Negative Cases:
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter the Row size for Matrix(1-100): 9000 Enter the Column Size for Matrix(1-100): 3 Invalid Rows or Columns size, Please Try again $ ./a.out Enter the Row size for Matrix(1-100): 0 Enter the Column Size for Matrix(1-100): 0 Invalid Rows or Columns size, Please Try again $ |
As excepted Program is displaying an error message( Invalid Rows or Columns size, Please Try again) if the row or column sizes goes beyond limits
Check Sparse Matrix using Functions in C Program:
In the above program, We have used only the main() function. Let’s divide the program and create a function to take the user input and check the Sparse Matrix.
Here is the program with two user defined functions isSparse to check if the given matrix is a sparse matrix.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 |
/*     Program to Check Sparse Matrix in C     SillyCodes.com */  #include <stdio.h>  // Define MAX ROWS and COLUMNS const int ROWS=100; const int COLUMNS=100;  /** * @brief  - Checks if the "X" is sparse Matrix * * @param rows  - Rows in "X" * @param columns - Columns in "X" * @param X      - Matrix * @return int    - 1 if the "X" is Sparse Matrix, Otherwise 0 */  int isSparse(int rows, int columns, int X[rows][columns]) {     int i, j;     // create 'nZeros' and intialize with '0'     int nZeros = 0;      // Take the user input for 1st Matrix - 'X'     printf("Enter Elements for the Matrix(X) of Size %dX%d: \n", rows, columns);      for(i=0; i<rows; i++)     {         for(j=0; j<columns; j++)         {             // printf("X[%d][%d]: ", i, j);             scanf("%d", &X[i][j]);              // check if the element is Zero             if (X[i][j] == 0)             {                 // increment counter 'nZeros'                 nZeros++;             }         }     }      return (nZeros > ((rows * columns) / 2));  }  int main() {     // Declare two matrices.     // 'X' is Input matrice     int X[ROWS][COLUMNS];     int rows, columns;      // Take the Row size and Column size from user     printf("Enter the Row size for Matrix(1-%d): ", ROWS);     scanf("%d", &rows);     printf("Enter the Column Size for Matrix(1-%d): ", COLUMNS);     scanf("%d", &columns);      // check for array bounds     if(rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0)     {         printf("Invalid Rows or Columns size, Please Try again \n");         return 0;     }      // Call the isSparse() function with Matrix to take user input and check the sparse Matrix     if(isSparse(rows, columns, X))     {         printf("Given Matrix is a Sparse Matrix\n");     }     else     {         printf("Given Matrix is not a Sparse Matrix\n");     }      return 0; } |
We have created a user-defned function called isSparse().
- Prototype of the isSparse() function – int isSparse(int rows, int columns, int X[rows][columns])
- The isSparse() function takes three Formal Arguments they are rows , columns, and X matrix.
- The isSparse() function takes the user input and updates the Matrix-X and check if the Matrix-X is a Sparse Matrix.
- This function reads the user input and also counts the number of zeroes ( nZeros) in the Matrix-X, Finally checks the number of zeroes with the (nZeros > ((rows * columns) / 2)); and returns the results.
- It returns 1, if the given Matrix-X is a Sparse Matrix, Otherwise, it returns Zero(0).
Call the isSparse() function from the main() function to check if the matrix is a Sparse matrix.
Program Output:
Compile and Run the Program and observe the output.
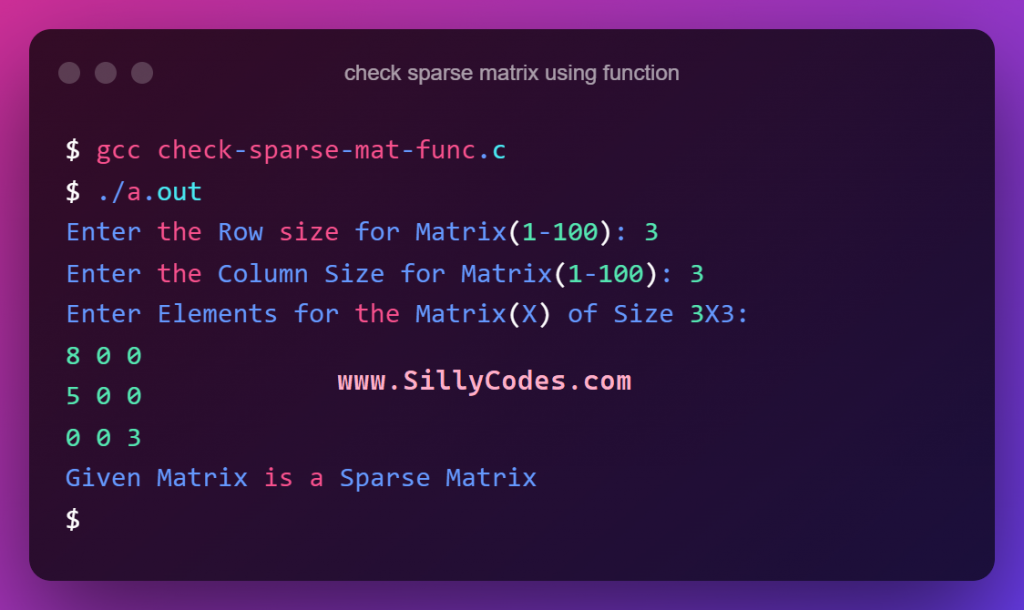
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
$ gcc check-sparse-mat-func.c $ ./a.out Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 3X3: 8 0 0 5 0 0 0 0 3 Given Matrix is a Sparse Matrix $ ./a.out Enter the Row size for Matrix(1-100): 5 Enter the Column Size for Matrix(1-100): 2 Enter Elements for the Matrix(X) of Size 5X2: 1 0 0 0 0 0 0 1 0 0 Given Matrix is a Sparse Matrix $ $ ./a.out Enter the Row size for Matrix(1-100): 4 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 4X3: 2 3 4 5 0 0 8 2 0 0 2 9 Given Matrix is not a Sparse Matrix $ |
The program is properly detecting the sparse matrix.
Related Matrix Programs:
- C Program to Read and Print Matrix or 2D Array
- C Program to demonstrate How to Pass 2D Array to Functions
- C Program to Multiplication of two Matrices
- C Program to Add Two Matrices
- C Program to Subtract Two Matrices
- C Program to Calculate the Transpose of Matrix
- C Program to Check Two Matrices are Equal
- C Program to Check Multiplicability of Two Matrices
1 Response
[…] C Program to Check a Sparse Matrix […]