Program to Concatenate Two Strings in C Language
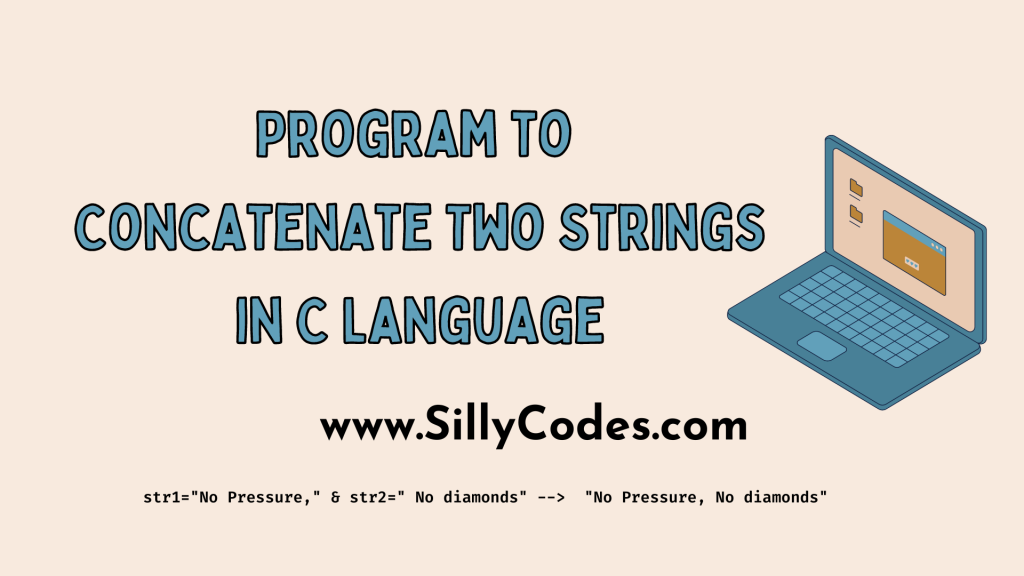
Program Description:
Write a Program to Concatenate Two Strings in C programming language. The program should accept two strings from the user and Concatenate or append the second string at the end of the first string.
Expected Input and Output:
Input:
Enter the first string : All limitations
Enter the second string :Â Â are self-imposed
Output:
String after Concatenation: All limitations are self-imposed
Note, that we also added an extra space at the start of the second string.
Program Prerequisites:
It is recommended to know the basics of Strings and Arrays in C Programming. Please go through the following articles to learn more about these topics.
- Strings in C Language – Declare, Initialize, and Modify Strings
- C Arrays
- Different ways to Read and Print strings in C
We are going to look at three different methods to concatenate two string in C Language in this article, They are
- Iterative Method: Manually concatenating the second string to first string using Loops.
- User-Defined Function: Using a user-defined function(without strcat) to concatenate the string.
- strcat library function: Using the strcat library function.
📢 This Program is part of the C String Practice Programs series
Concatenate Two Strings in C Iterative Method (without using strcat):
In order to concatenate two strings, We need to first calculate the length of the first string, Then use a for loop to iterate over the second string and Append elements from the second string to the first string.
Here is the step-by-step explanation of the program.
Program to Concatenate two string Iterative Method Algorithm:
- Declare two strings str1(i.e first string) and str2(second string). The str1 should have enough capacity to hold the two strings after concatenation.
- Read the two strings from the user and update str1 and str2 respectively.
- Calculate the Length of the First String( str1): To calculate the length of the str1, We can use a strlen function or a loop to count the number of elements. We are using a for loop to calculate the string length. The str1Length variable contains the string str1 length.
- Iterate over the Second String(
str2) and Append elements to
str1: Start a For loop to iterate over the
str2 and append characters of the
str2 to first string
str1. As we are appending to
str1, The starring index will be
str1Length.
- FOR Loop – Start with i=0 and continue till str2[i] != '\0'.
- At each iteration copy str2[i] to str1[str1Length] – i.e str1[str1Length] = str2[i];
- Increment the str1Length variable.
- Finally, Add the Terminating NULL character ( \0) to str1 string.
- Display the result on the console.
Concatenate Two string Iterative Method Program:
Let’s convert the above explanation into the C Code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
/*     program to concatenate two strings in c     sillycodes.com */  #include<stdio.h>  const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char str1[SIZE], str2[SIZE];     int i;      // get the string from the user     printf("Enter the first string : ");     // scanf("%s", str1);     gets(str1);         printf("Enter the second string : ");     gets(str2);          // calculate the length of the 'str1'     int str1Length=0;     while(str1[str1Length] != '\0' )     {         str1Length++;     }      // go through elements of 'str2' and add at the end of 'str1'     for(i=0; str2[i] != '\0'; i++)     {         // add chars of 'str2' to 'str1'         str1[str1Length] = str2[i];         str1Length++;     }      // Finally add the terminating NULL character     str1[str1Length] = '\0';      // Print the result     printf("String after Concatenation: %s \n", str1);      return 0; } |
Program Output:
Compile and Run the program.
1 2 3 4 5 6 |
$ gcc concate-strings.c $ ./a.out Enter the first string : All limitations Enter the second string :Â Â are self-imposed String after Concatenation: All limitations are self-imposed $ |
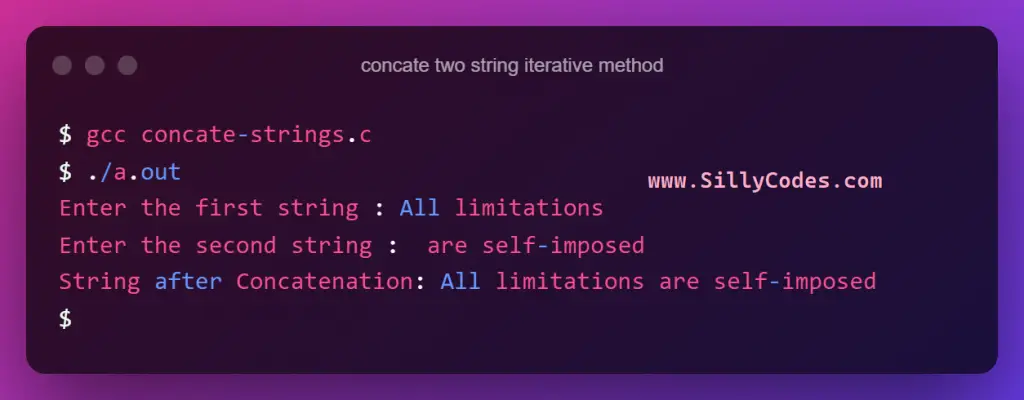
As we can see from the above output, The program is properly appending the str2 to str1.
Concatenate Two Strings in C using a user-defined function (custom strcat function):
Let’s look at another method to concatenate two strings in C language. Which is using a user defined function to concatenate two strings.
In the following program, We defined a custom strcat function named myStrConcate() function. The myStrConcate function takes two character pointers.
char * myStrConcate(char * dest, char * src);
The myStrConcate function concatenates the src string at the end of the dest string. This function also returns the dest string back to the caller.
Here is the program to concatenate two strings using custom strcat function
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
/*     program to concatenate two strings in c using user defined function     sillycodes.com */  #include<stdio.h> const int SIZE = 100; // Function declaration char * myStrConcate(char * dest, char * src);  int main() {      // declare a string with 'SIZE'     char str1[SIZE], str2[SIZE];     int i;      // get the string from the user     printf("Enter the first string : ");     // scanf("%s", str1);     gets(str1);         printf("Enter the second string : ");     gets(str2);      // Call the 'myStrConcate' with 'str1' and 'str2'     myStrConcate(str1, str2);      // Print the result     printf("String after Concatenation: %s \n", str1);      return 0; }  /** * @brief  - Concatenate two string 'dest' and 'src' * * @param dest - 'str1' - Destination string where we append 'src' * @param src  - 'str2' - Source string * @return char* - Appended string. */  char * myStrConcate(char * dest, char * src) {     int i;      // calculate the length of the 'dest'     int destLength=0;     while(dest[destLength] != '\0' )     {         destLength++;     }      // go through elements of 'src' and add at the end of 'dest'     for(i=0; src[i] != '\0'; i++)     {         // add chars of 'src' to 'dest'         dest[destLength] = src[i];         destLength++;     }      // Finally add the terminating NULL character     dest[destLength] = '\0';      // Return the Appended string 'dest'     return dest; } |
Program Output:
Compile and Run the program. We are using GCC compiler.
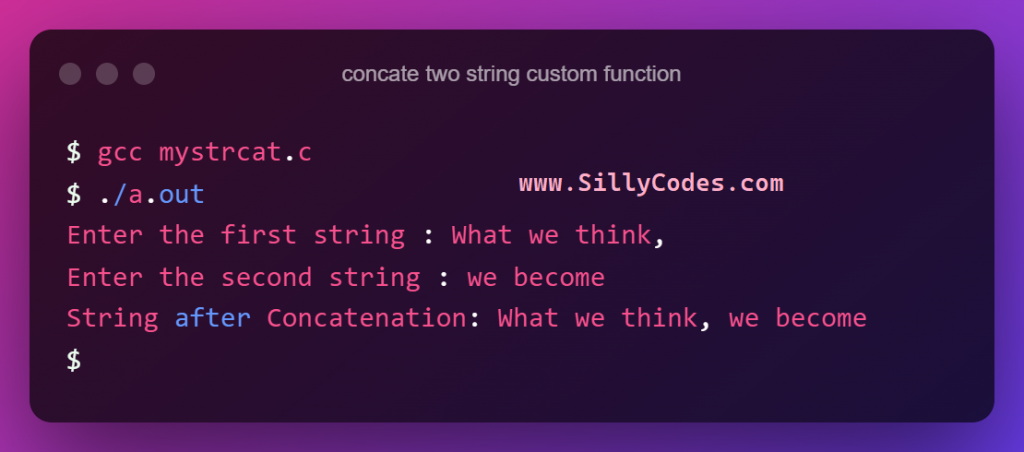
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc mystrcat.c $ ./a.out Enter the first string : What we think, Enter the second string : we become String after Concatenation: What we think, we become $ $ ./a.out Enter the first string : First String, Enter the second string : This is going to be appended String after Concatenation: First String, This is going to be appended $ |
We can further divide the myStrConcatefunction into two functions. One function to calculate the length of the dest(i.e str1) string and other function to do the concatenate operation.
Program to Concatenate Two Strings in C using strcat library function:
Now let’s look at the third method to concatenate two strings in the C language, Which is using the strcat library function.
The C Standard library provides the strcat() function to concatenate strings. The strcat() function is declared in the string.h header file.
📢 Related: strcat function in c
Here is the syntax of the strcat function
char * strcat(char * dest, const char * src);
So Let’s rewrite the program and use the strcat library function to concatenate two strings.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
/*     program to concatenate two strings in c using strcat     sillycodes.com */  #include <stdio.h> #include <string.h>    // for 'strcat' function  const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char str1[SIZE], str2[SIZE];     int i;      // get the string from the user     printf("Enter the first string : ");     // scanf("%s", str1);     gets(str1);         printf("Enter the second string : ");     gets(str2);          // Call the 'strcat' function     strcat(str1, str2);      // Print the result     printf("String after Concatenation: %s \n", str1);      return 0; } |
Don’t forget to include the string.h header file.
📢 It is recommended to use the strcat library function to concatenate two strings.
Program Output:
Compile the program
$ gcc concat-strcat.c
The above command compiles the .c file and generates the executable file. by default, gcc compiler generates the executable file with a.out name. You can change this by passing the -o option to the gcc command.
Run the executable file using the ./.aout command.
1 2 3 4 5 |
$ ./a.out Enter the first string : No Pressure, Enter the second string :Â Â No diamonds String after Concatenation: No Pressure, No diamonds $ |
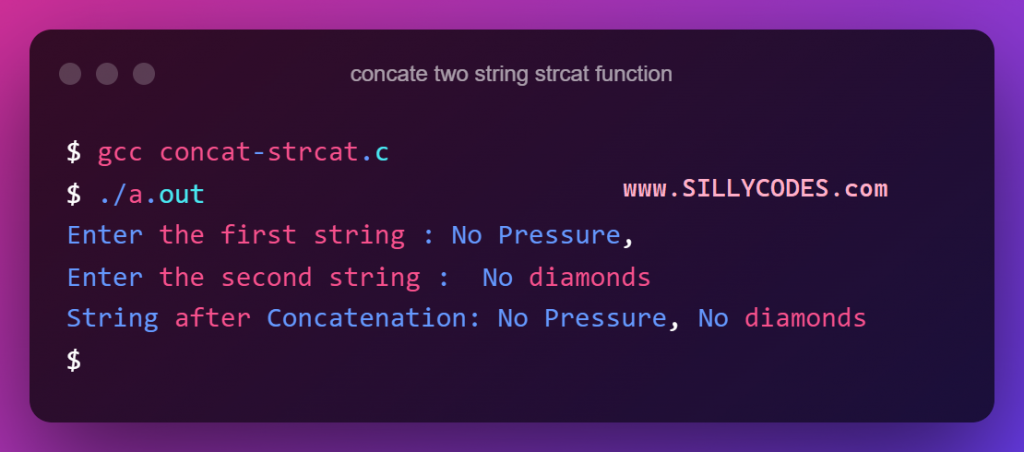
As we can see from the above output, The first string is No Pressure, and the second string is No diamonds. The strcat() function concatenated two strings and generated the resultant string as No Pressure, No diamonds
Related C Practice Programs:
- Index – C Language Practice Programs
- C Tutorials Index – Step by step tutorials to master C Langauge
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
- C Program to Find Maximum Element in Array
- C Program to Find Second Largest Element in Array
- C Program to Insert an element in Array
- C Program to Delete an Element in Array
- C Program to Find Unique Elements in an Array
1 Response
[…] C Program to Concatenate Two Strings […]