Program to Copy String in C Language
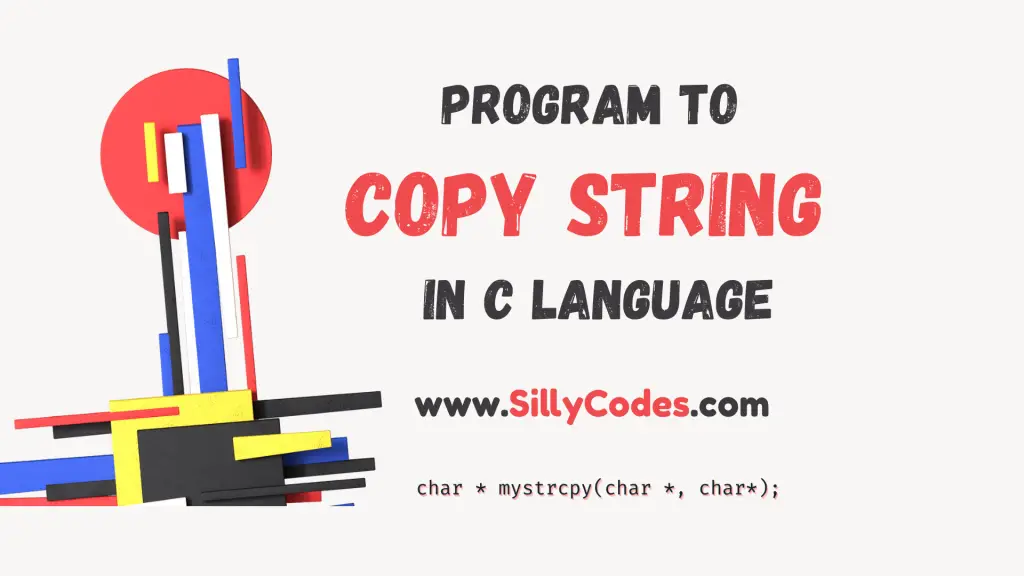
Program Description:
Write a Program to Copy String in C programming language. The program should accept a string (character array) from the user and copy it to new string.
Example Input and Output:
Input:
Enter a string : sillycodes.com
Output:
Original String: sillycodes.com
Copied String : sillycodes.com
Prerequisites:
Please go through the following articles to better understand this program.
We are going to look at three different methods to copy a string in C programming
- Iterative Method: Manually Copying the elements of the original string to a new string.
- User-Defined Function: Using a user-defined function(without strcpy) to copy the string.
- strcpy library function: Using the strcpy library function.
Copy String in C without using strcpy function (Iterative Method):
We can copy a string to a new string by traversing the original string element by element and copying each element to the new string.
Here is the algorithm of the manual string copy program.
Program Algorithm:
- Create Two strings named origStr and dupStr with size of SIZE ( i.e SIZE = 100). The dupStr should have enough size to hold the origStr.
- Prompt the user to provide the original string( origStr) and update the origStr variable.
- To Copy the original string to a new string, Iterate over the Original String using a For Loop and Copy elements from
origStr to
dupStr
- FOR Loop – Start with i=0 and continue till origStr[i] != '\0'.
- At each iteration copy origStr[i] to dupStr[i] – i.e dupStr[i] = origStr[i];
- Finally, add the terminating NULL character to dupStr string. – dupStr[i] = '\0';
- Display the results on the console.
📢 This Program is part of the C String Practice Programs series
Program to Copy String to a new string:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/*     program to copy string in C     sillycodes.com */  #include<stdio.h>  const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char origStr[SIZE], dupStr[SIZE];     int i;      // get the string from the user     printf("Enter a string : ");     // scanf("%s", mainStr);     gets(origStr);             // Iterate through the string and copy character by character     for(i=0; origStr[i] != '\0'; i++)     {         // copy the character from orig to dup         dupStr[i] = origStr[i];     }      // Finally add the terminating NULL character     dupStr[i] = '\0';      // Print the result     printf("Original String: %s\n", origStr);     printf("Copied String : %s\n", dupStr);      return 0; } |
Program Output:
Compile and Run the program ( We are using GCC compiler on Ubuntu Linux OS)
1 2 3 4 5 6 |
$ gcc string-copy.c $ ./a.out Enter a string : sillycodes.com Original String: sillycodes.com Copied String : sillycodes.com $ |
Let’s try a few more examples.
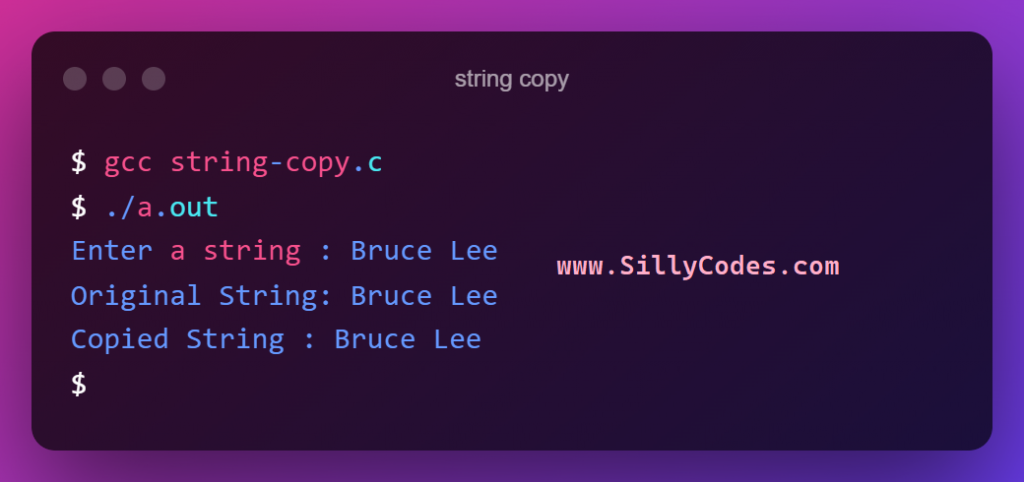
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a string : Bruce Lee Original String: Bruce Lee Copied String : Bruce Lee $ $ ./a.out Enter a string : Indian Ocean Original String: Indian Ocean Copied String : Indian Ocean $ |
The program is properly copying the original string to the new string.
Program to Copy string using a user-defined function in C:
Let’s look at the second method to copy a string to a new string. In this method, We are going to create a user-defined function to copy the string.
In the following program, We have defined a user-defined function called mystrcpy
char * mystrcpy(char *dest, char *src)
The mystrcpy() function takes two character pointers as the formal arguments. The first argument is dest and second argument is src. The mystrcpy() function copies the characters from the src string to the dest string. This function also returns a character pointer pointing to the dest string (i.e resultant string).
Duplicate string using custom strcpy function C Program:
Here is the program to duplicate a string without using the strcpy function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
/*     program to copy string in C using user-defined function     sillycodes.com */  #include<stdio.h> const int SIZE = 100;  /** * @brief  - Copy the 'src' string to 'dest' string * * @param dest - destination string(pointer) * @param src  - Pointer pointing to source string * @return char* - Copied String ('dest' string) */  char * mystrcpy(char *dest, char *src) {     int i = 0;      // Iterate through the 'src' string and copy character by character to 'dest'     for(i=0; src[i] != '\0'; i++)     {         // copy the character from 'src' to 'dest'         dest[i] = src[i];     }      // Finally add the terminating NULL character     dest[i] = '\0';      // return the 'dest' string     return dest; }  int main() {      // declare a string with 'SIZE'     char origStr[SIZE], dupStr[SIZE];     int i;      // get the string from the user     printf("Enter a string : ");     // scanf("%s", mainStr);     gets(origStr);         // call the 'mystrcpy' function to copy strings     mystrcpy(dupStr, origStr);         // Print the result     printf("Original String: %s\n", origStr);     printf("Copied String : %s\n", dupStr);      return 0; } |
The mystrcpy() function also uses a for loop to iterate over the src string and copies the elements to dest string.
Program Output:
Let’s compile and run the program.
1 2 3 4 5 6 7 8 9 10 |
$ gcc mystrcpy.c $ ./a.out Enter a string : VS Code Original String: VS Code Copied String : VS Code $ ./a.out Enter a string : GCC Compiler Original String: GCC Compiler Copied String : GCC Compiler $ |
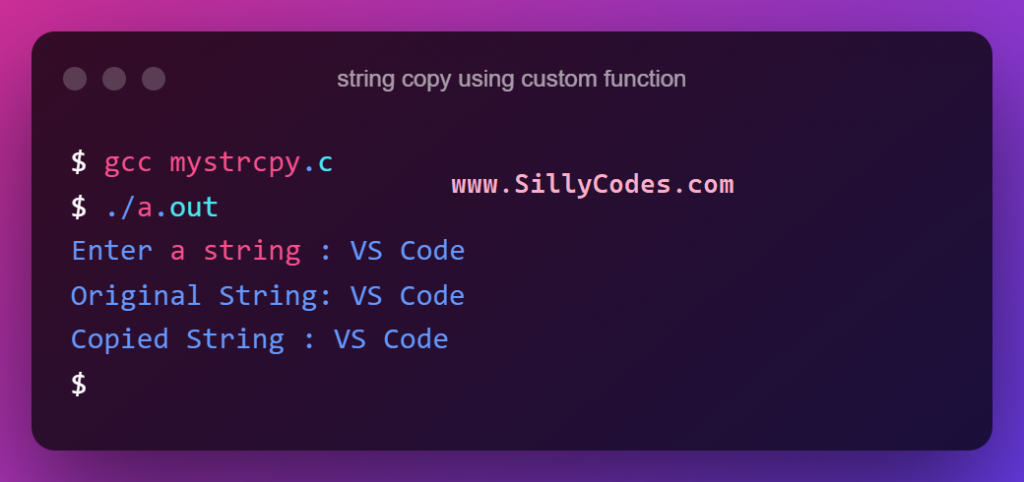
In the first example, User provided the VS Code as the original string( origStr) and mystrcpy() function copied it to the new string ( dupStr).
Copy string using the strcpy function in C Language:
The C Standard library also provides strcpy library function to copy a string to new string. So let’s use this function to create a duplicate string.
The strcpy() function is available as part of the string.h header file. So we need to include the string.h header file to use the strcpy() function.
📢We have covered the strcpy() library function in detail in the following article
Program to Copy String using strcpy function:
Here is the program to copy a string using the strcpy library function
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
/*     program to copy string in C using strcpy function     sillycodes.com */  #include <stdio.h> #include <string.h>  // For 'strcpy' function  // Max size of the string const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char origStr[SIZE], dupStr[SIZE];     int i;      // get the string from the user     printf("Enter a string : ");     // scanf("%s", mainStr);     gets(origStr);         // copy string using 'strcpy'     strcpy(dupStr, origStr);      // Print the result     printf("Original String: %s\n", origStr);     printf("Copied String : %s\n", dupStr);      return 0; } |
Program Output:
Compile and run the program and observe the output.
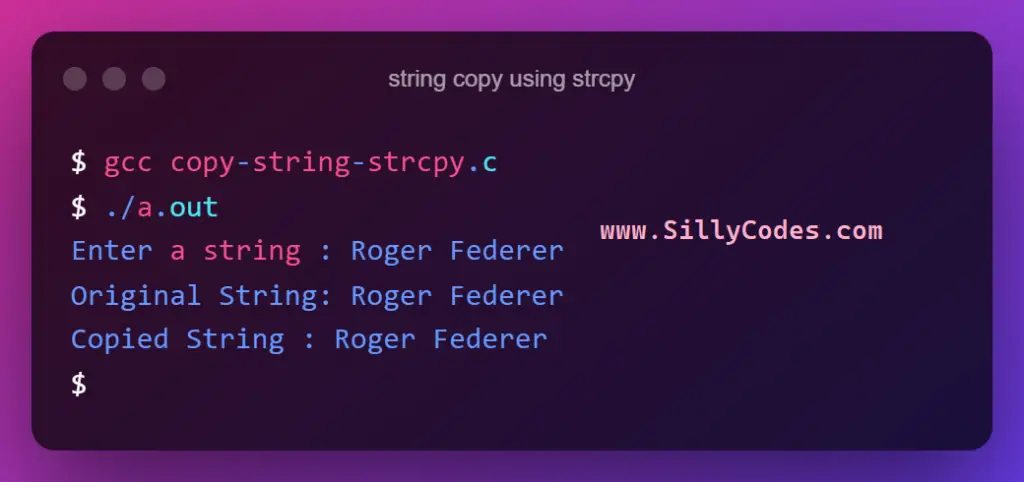
1 2 3 4 5 6 7 8 9 10 |
$ gcc copy-string-strcpy.c $ ./a.out Enter a string : Roger Federer Original String: Roger Federer Copied String : Roger Federer $ ./a.out Enter a string : Everything has beauty, but not everyone sees it. Original String: Everything has beauty, but not everyone sees it. Copied String : Everything has beauty, but not everyone sees it. $ |
As expected program is properly copying the source string to destination string using strcpy function.
📢It is recommended to use the strcpy() Library function to copy strings instead of using the custom implementations. As library functions are fast and more efficient.
Related Programs:
- C Tutorials Index
- C Programs Index
- C Program to Count Even and Odd numbers in Array
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
1 Response
[…] C Program to Copy a String to New String […]