Program to Remove extra spaces between the words in C
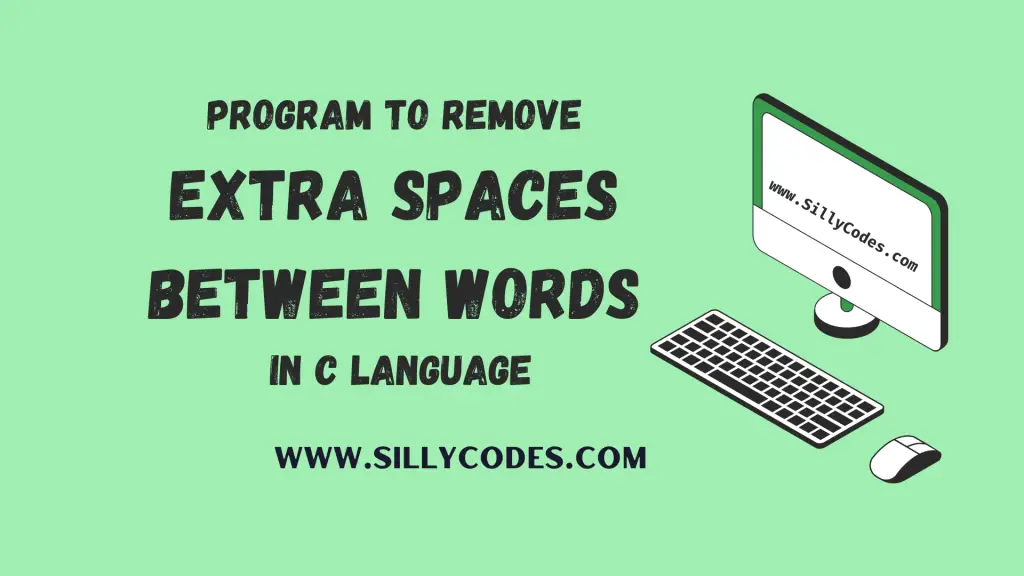
Program Description:
Write a Program to Remove extra spaces between the words in a String using C Programming language. The program should accept a string or sentence from the user, The input string may contain extra whitespace (spaces, tabs, etc) in between the words. The program should remove all extra whitespace between the words and return the resultant sentence. The resultant sentence will have only one space between the words.
📢 This Program is part of the C String Practice Programs series
Here is the program expected input and output
Example Input and Output:
Input:
Enter a string : Learn Programming Online
Output:
Before Removing Whitespaces:Learn Programming Online(Actual string)
After Removing Whitespaces:Learn Programming Online(Resultant string)
If you observe the resultant string only has one space between the words and all extra spaces are removed.
Program Prerequisites:
It is recommended to have knowledge of the string, arrays, and functions in C. Please go through the following articles to learn about these concepts.
- Strings in C Language with Example Programs
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
Let’s look at the step-by-step explanation of the program to remove extra spaces between the words in a sentence.
Remove extra spaces between the words in a String Program Explanation:
- Create a Macro named SIZE to store the maximum number of characters in the input string. We defined the Max size as 100. Please change this value to suit your requirements.
- Declare an input string with the SIZE number of characters. Let’s say this string as inputStr. Also, create a Resultant string named resultStr with SIZE characters.
- Prompt the user to provide the input string and read it using the gets function and update the inputStr string with the given string.
- To Remove Extra spaces between the words in a String, We will use two indices called i and j. The i index will be used to traverse the input string( inputStr) and The j index is used to update the Resultant string( resultStr).
- Traverse the input string and Skip the character if two consecutive characters contain a whitespace character, Otherwise, update the current character in the resultStr string.
- Start a For Loop to traverse the string. Start with
i = 0, j = 0 and continue till we reach the terminating NULL character
inputStr[i] != '\0'
- At each iteration check if two consecutive characters are whitespaces using the isspace() function from the
ctype.h library. We can also manually compare the characters.
- If the above condition is true, The skip the character and continue to the next iteration
- If the above condition is false, Then update the resultStr[j] with inputStr[i]. Increment the both i and j indices. i.e resultStr[j++] = inputStr[i];
- At each iteration check if two consecutive characters are whitespaces using the isspace() function from the
ctype.h library. We can also manually compare the characters.
- Finally, Add the Terminating NULL character to the resultant string at the index j. i.e resultStr[j] = '\0';
- Print the results on the console.
Program to Remove extra spaces between the words in C:
Here is the program to Remove Extra spaces between the words in a given string in the C programming Language.
As we are using the isspace() function, We need to include the ctype.h header file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
/* program to Remove Remove extra spaces between the words in a String in C sillycodes.com */ #include<stdio.h> #include<ctype.h> // Max Size of string #define SIZE 100 int main() { // declare a string with 'SIZE' char inputStr[SIZE]; int i, j; // Create a string to store resultant string char resultStr[SIZE] = {0}; // get the string from the user printf("Enter a string : "); gets(inputStr); // Iterate over the string - Remove extra spaces between the words // index 'i' used for traversing the inputStr // index 'j' used to update the resultStr for(i=0, j=0; inputStr[i]; i++) { // check if we got the whitespace. if(isspace(inputStr[i]) && isspace(inputStr[i-1])) { // Two consecutive white spaces. Skip the 'i'. Continue to next iteration continue; } // Found a valid character, update resultStr. Increment 'j' resultStr[j++] = inputStr[i]; } // Add the terminating NULL character to resultStr resultStr[j] = '\0'; // Print Results printf("Before Removing Whitespaces:%s(Actual string)\n", inputStr); printf("After Removing Whitespaces:%s(Resultant string)\n", resultStr); return 0; } |
Program Output:
Compile and Run the Program using your favorite compiler
1 2 3 4 5 6 7 |
$ gcc remove-extra-space.c $ $ ./a.out Enter a string : Learn Programming Online Before Removing Whitespaces:Learn Programming Online(Actual string) After Removing Whitespaces:Learn Programming Online(Resultant string) $ |
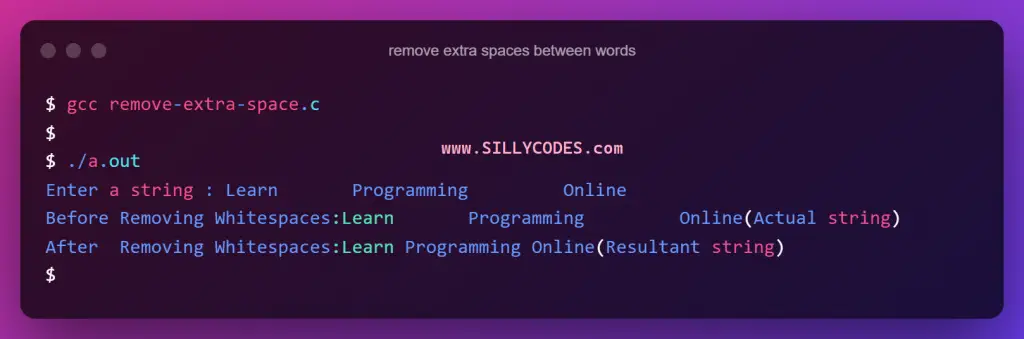
Let’s try a few more examples.
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a string : www. SILLYCODES .com Before Removing Whitespaces:www. SILLYCODES .com(Actual string) After Removing Whitespaces:www. SILLYCODES .com(Resultant string) $ $ ./a.out Enter a string : C Programming Online Before Removing Whitespaces:C Programming Online(Actual string) After Removing Whitespaces:C Programming Online(Resultant string) $ |
As we can see from the above outputs, The input string has white spaces in between the words and the program removed the spaces and returned the string without any extra spaces.
For example, When we provided the string C Programming Online as the input string, Program removed the extra spaces and returned the C Programming Online as the resultant string.
Remove extra spaces between the words in String using User defined function:
Let’s move the logic to remove extra spaces into a dedicated function So that we can reuse the code. In this program, We will create a function named removeWSpacesBWWords to remove the whitespace characters between the words.
Here is the prototype of the removeWSpacesBWWords() function.
void removeWSpacesBWWords(char *inputStr, char * outputStr)
The removeWSpacesBWWords function takes two formal arguments. They are input string( inputStr) and Output String( outputStr). This function removes extra white spaces between the words in the input string and stores the result in the Output string( outputStr).
Here is the program with a user-defined function to remove extra spaces in btw words
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
/* program to Remove Remove extra spaces between the words in a String in C using functions sillycodes.com */ #include<stdio.h> #include<ctype.h> #include<string.h> // Max Size of string #define SIZE 100 /** * @brief - Remove Whitespaces in between words in given string 'inputStr' * * @param inputStr - Input string * @param outputStr - Output String - Resultant string */ void removeWSpacesBWWords(char *inputStr, char * outputStr) { int i, j; // Iterate over the string - Remove extra spaces between the words // index 'i' used for traversing the inputStr // index 'j' used to update the outputStr for(i=0, j=0; inputStr[i]; i++) { // check if we got the whitespace. if(isspace(inputStr[i]) && isspace(inputStr[i-1])) { // Two consecutive white spaces. Skip the 'i'. Continue to next iteration continue; } // Found a valid character, update outputStr. Increment 'j' outputStr[j++] = inputStr[i]; } // Add the terminating NULL character to outputStr outputStr[j] = '\0'; } int main() { // declare a string with 'SIZE' char inputStr[SIZE]; // Create a string to store resultant string char outputStr[SIZE] = {0}; // get the string from the user printf("Enter a string : "); gets(inputStr); // call the 'removeWSpacesBWWords()' with 'inputStr' and 'outputStr' removeWSpacesBWWords(inputStr, outputStr); // Print Results printf("Before Removing Whitespaces:%s(Actual string)\n", inputStr); printf("After Removing Whitespaces:%s(Resultant string)\n", outputStr); return 0; } |
We need to call the removeWSpacesBWWords() function from the main() function with the two strings. i.e inputStr and outputStr.
1 2 |
// call the 'removeWSpacesBWWords()' with 'inputStr' and 'outputStr' removeWSpacesBWWords(inputStr, outputStr); |
Finally, Print the results on the screen using printffunction.
Program Output:
Let’s compile and run the program and observe the output.
1 2 3 4 5 6 7 8 9 10 11 12 |
$ gcc remove-extra-space-func.c $ $ ./a.out Enter a string : Programming is Fun Before Removing Whitespaces:Programming is Fun(Actual string) After Removing Whitespaces:Programming is Fun(Resultant string) $ $ ./a.out Enter a string : Learn Programming at Sillycodes.com Before Removing Whitespaces:Learn Programming at Sillycodes.com(Actual string) After Removing Whitespaces:Learn Programming at Sillycodes.com(Resultant string) $ |
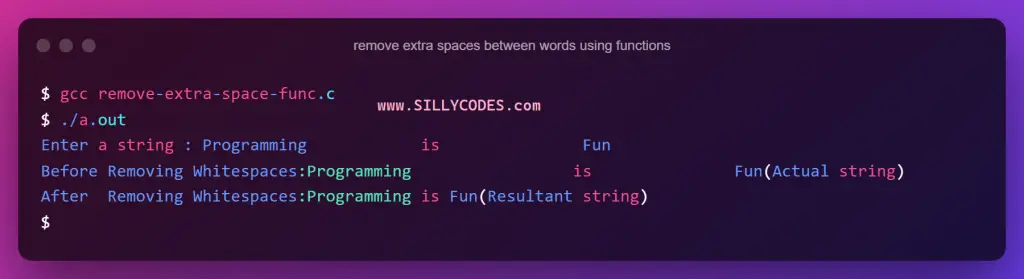
As we can see from the above input and output, The program is properly removing the unnecessary whitespace in between the words in the given string/sentence.
Related Programs:
- C Tutorials Index
- C Programs Index
- C Program to Calculate Length of the string
- C Program to Copy a String to new string
- C Program to Concatenate Two strings
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
4 Responses
[…] Remove extra whitespaces in between words in a String/Sentence. […]
[…] C Program to Remove Extra Spaces between the Words in String […]
[…] C Program to Remove Extra Spaces between the Words in String […]
[…] C Program to Remove Extra Spaces between the Words in String […]