Remove Last Occurrence of Character in String in C
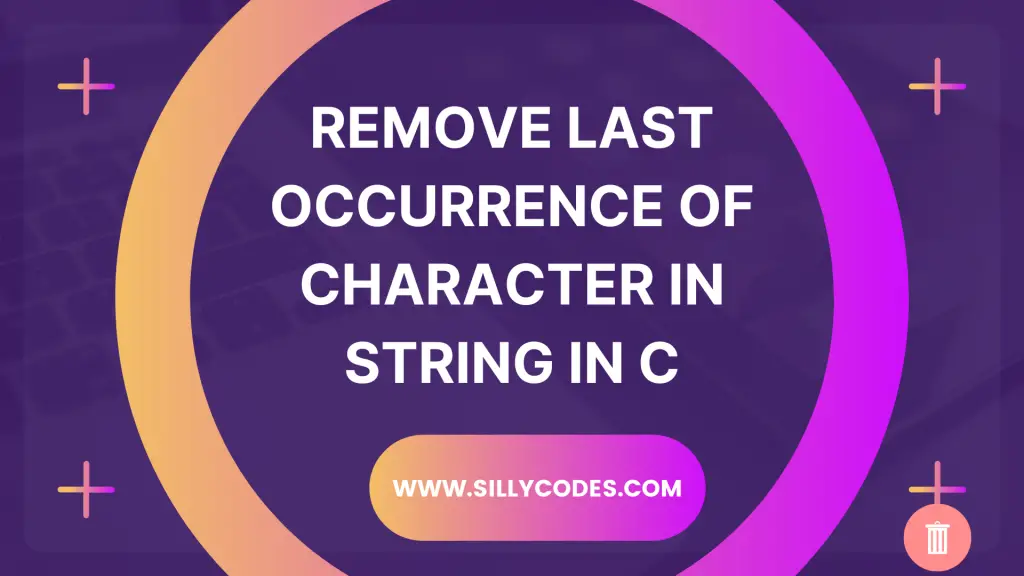
Program Description:
Write a Program to remove last occurrence of character in string in c programming language. The program will accept a string and a character from the user and search and removes the last occurrence of the given character in the string.
📢The program is case-sensitive. So the Uppercase characters and Lowercase characters are different.
We will look at two methods to Search and Remove the First occurrence of a character in the string in C programming. Each method will contain a step-by-step explanation of the program with program output. We also included the instructions to compile and run the program using your compiler.
📢 This Program is part of the C String Practice Programs series
Let’s look at the example input and output of the program to better understand the program requirements.
Excepted Input and Output:
Input:
Enter a string : red lorry blue lorry
Enter character to remove from the string: o
Output:
String Before Removing Last Occurance of character('o') : red lorry blue lorry
String After Removing last Occurance of character : red lorry blue lrry
If you notice the above input and output, The character o last occurrence has been removed.
Prerequisites:
It is recommended to know the C-Strings, C-Arrays, and Functions in C to better understand the following program.
We will look at the two different methods to Remove the character in a string in C Language.
- Iterative Method to Remove character from string
- User-defined Functions to Remove character from string
Remove Last Occurrence of Character in String in C Program Explanation:
Here are the step-by-step instructions for the program.
- Start the program by creating a macro named SIZE. This macro holds the max size of the string- #define SIZE 100
- Declare the input string( inputStr) and character to search( ch).
- Also initialize the variable lastIdx with value -1. The lastIdx will store the index of the last occurrence of a character( ch) in the string.
- Take the input string and character to search from the user and update the inputStr and ch variables respectively. We use the gets function to read the string.
- Now, To Remove the last occurrence of the character( ch) in a string( inputStr), Traverse over the input string, and find the position or index( lastIdx) of the last occurrence of the character in the string. Once we find the last index( lastIdx) move all characters from the right side of the index lastIdx to one position left. ( i.e shift all characters to one place left)
- Create a Loop to find the last position of the character in input string. The For loop starts by initializing variable i to 0. It will continue looping as long as the present character (
inputStr[i]) is not a
NULLcharacter (
'\0').
- At each iteration, Check if the current character is equal( inputStr) to target character( ch). – i.e if(inputStr[i] == ch)
- If the above condition is true, Then update the lastIdx variable with the current index value( i) – i.e lastIdx = i;
- Otherwise, i.e condition is false, Then continue to the next iteration.
- Once the above loop is completed, The variable
lastIdx will contain the index of the last occurrence of character(
ch) in input string. Move all characters from the right side of the
lastIdx index to one position left. To do this, Create a While loop and run it till the lastIdx < strlen(inputStr) condition is
true.
- At each iteration of the while loop, Copy the character at index lastIdx+1 to index lastIdx – i.e inputStr[lastIdx] = inputStr[lastIdx+1];
- Increment the index lastIdx by 1. – lastIdx++;
- Once the above Step 7 is completed, The last occurrence of the character ch will be removed from the input string inputStr. Display the results on the console.
- Stop the program.
Program to Remove First Occurrence of Character in String in C – Iterative Method:
Program to remove a character from the string in the c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
/* program to remove a last occurance character from a string in C sillycodes.com */ #include <stdio.h> #include <string.h> // Max Size of string #define SIZE 100 int main() { // declare a string with 'SIZE' char inputStr[SIZE]; char ch; int i; // initialize 'lastIdx' int lastIdx = -1; // Take the string from the user printf("Enter a string : "); gets(inputStr); // Also take the search character printf("Enter character to remove from the string: "); scanf(" %c", &ch); printf("String Before Removing Last Occurance of character('%c') : %s \n", ch, inputStr); // Iterate over the string and find the last occurance index for(i = 0; inputStr[i]; i++) { // check if the current character is equal to target('ch') character if(inputStr[i] == ch) { // update the 'lastIdx' with 'i' lastIdx = i; } } if(lastIdx == -1) { printf("NOTFOUND: character '%c' is not found in given string \n", ch); return 0; } else { // Character is found // last occurance index is 'lastIdx' // Move all characters from right side of 'lastIdx' to one position 'left' while (lastIdx < strlen(inputStr)) { // copy 'i+1' to 'i' index inputStr[lastIdx] = inputStr[lastIdx+1]; lastIdx++; } printf("String After Removing last Occurance of character : %s \n", inputStr); } return 0; } |
As we are using the strlen() function, We need to include the string.h header file. If you don’t want to use the library function, Then you can look at this article, Where we implemented the custom string length (strlen) function in C.
Program Output:
Compile and Run the program using GCC (Any Compiler). Here are the instructions to compile and run the program using the GCC compiler.
Example 1:
1 2 3 4 5 6 7 |
$ gcc remove-last.c $ ./a.out Enter a string : red lorry blue lorry Enter character to remove from the string: o String Before Removing Last Occurance of character('o') : red lorry blue lorry String After Removing last Occurance of character : red lorry blue lrry $ |
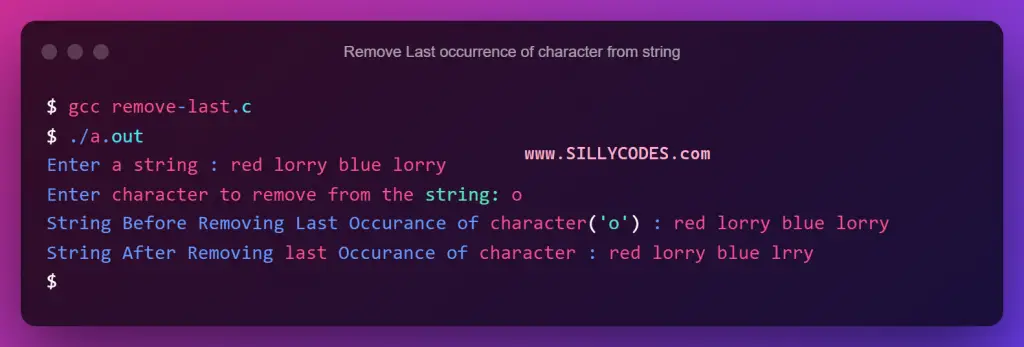
In the above example, The last occurrence of the character o has been deleted.
Example 2:
1 2 3 4 5 6 |
$ ./a.out Enter a string : ABBBBBCDEF Enter character to remove from the string: B String Before Removing Last Occurance of character('B') : ABBBBBCDEF String After Removing first Occurance of character : ABBBBCDEF $ |
In this example, The input string is ABBBBBCDEF and the character to remove is B. So the program removed the last occurrence of the B in the input string and returned the resultant string which is equal to ABBBBCDEF
Remove Last Occurrence of Character in String using a user-defined function in C:
Let’s use the C functions to divide the above program into sub-functions, Where a function does a specific task. So let’s create a function to remove the last occurrence of the specified character in the input string. By using the functions we can define a function once and can call it as many times as we want. Functions also increase the readability of the program and make it easier to debug issues.
In the following program, we are going to create a user-defined function called removeLastCh to remove the last occurrence of the character in the string.
Here is the prototype of the removeLastCh function
1 |
int removeLastCh(char * inputStr, char ch) |
This function takes two formal arguments, They are a character pointer ( char *) pointing to the input string( inputStr) and the character variable(ch) which holds the character to be searched in the input string.
The removeLastCh() function also returns an integer variable back to the user. It returns
- -1 if the character ch is not found in the input string inputStr
- If the character is found, Then it will return a value greater than Zero(0) ( > 0 ). To be precise this function returns the last find index of the character ( lastIdx )
Here is the modified version of the program with the removeLastCh function to remove the character from the string
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
/* program to remove a last occurance character from a string in C using functions sillycodes.com */ #include <stdio.h> #include <string.h> // Max Size of string #define SIZE 100 /** * @brief - Remove last occurance of character in string * * @param inputStr - Input String * @param ch - Character * @return int - -1 if the 'ch' is not found in 'inputStr', Otherwise > 0. */ int removeLastCh(char * inputStr, char ch) { // initialize 'lastIdx' int lastIdx = -1, i; // Iterate over the string and find the last occurance index for(i = 0; inputStr[i]; i++) { // check if the current character is equal to target('ch') character if(inputStr[i] == ch) { // update the 'lastIdx' with 'i' lastIdx = i; } } if(lastIdx != -1) { // Character is found // last occurance index is 'lastIdx' // Move all characters from right side of 'lastIdx' to one position 'left' while (lastIdx < strlen(inputStr)) { // copy 'i+1' to 'i' index inputStr[lastIdx] = inputStr[lastIdx+1]; lastIdx++; } } return lastIdx; } int main() { // declare a string with 'SIZE' char inputStr[SIZE]; char ch; int i; // Take the string from the user printf("Enter a string : "); gets(inputStr); // Also take the search character printf("Enter character to remove from the string: "); scanf(" %c", &ch); printf("String Before Removing Last Occurance of character('%c') : %s \n", ch, inputStr); int result = removeLastCh(inputStr, ch); if(result == -1) printf("NOTFOUND: character '%c' is not found in given string \n", ch); else printf("String After Removing Last Occurance of character : %s \n", inputStr); return 0; } |
Call the removeLastCh function from the main() function with the inputStr and ch as the arguments. Grab the function return value in the result variable.
int result = removeLastCh(inputStr, ch);
Finally, display the output on the console based on the result variable.
Program Output:
Let’s compile and run the program
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ gcc remove-last-fun.c $ ./a.out Enter a string : rotator Enter character to remove from the string: t String Before Removing Last Occurance of character('t') : rotator String After Removing Last Occurance of character : rotaor $ $ ./a.out Enter a string : coroutine Enter character to remove from the string: o String Before Removing Last Occurance of character('o') : coroutine String After Removing Last Occurance of character : corutine $ |
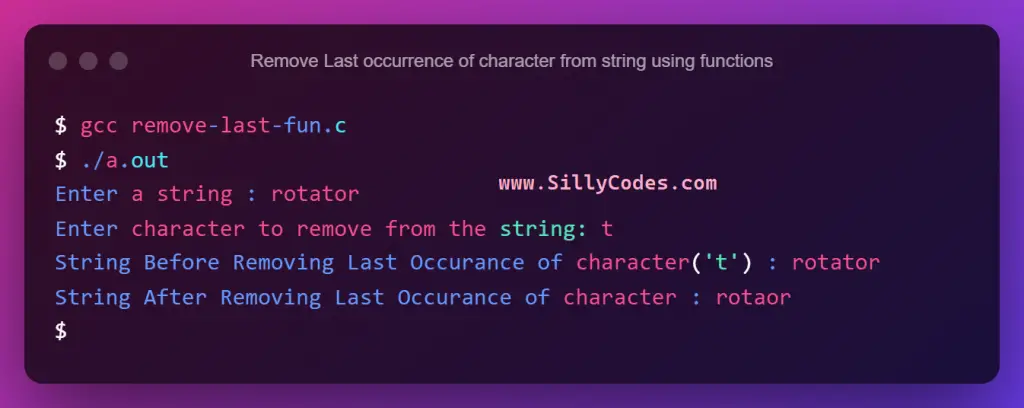
As we can see from the above output, The program is properly deleting the last occurrence of the character from the string.
Related Programs:
- C Tutorials Index
- C Programs Index
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
- C Program to Count Frequencies of each character in a string
- C Program to Find Highest Frequency character in a String
- C Program to Find Lowest Frequency Character in a String