Convert String from Uppercase to Lowercase in C Language
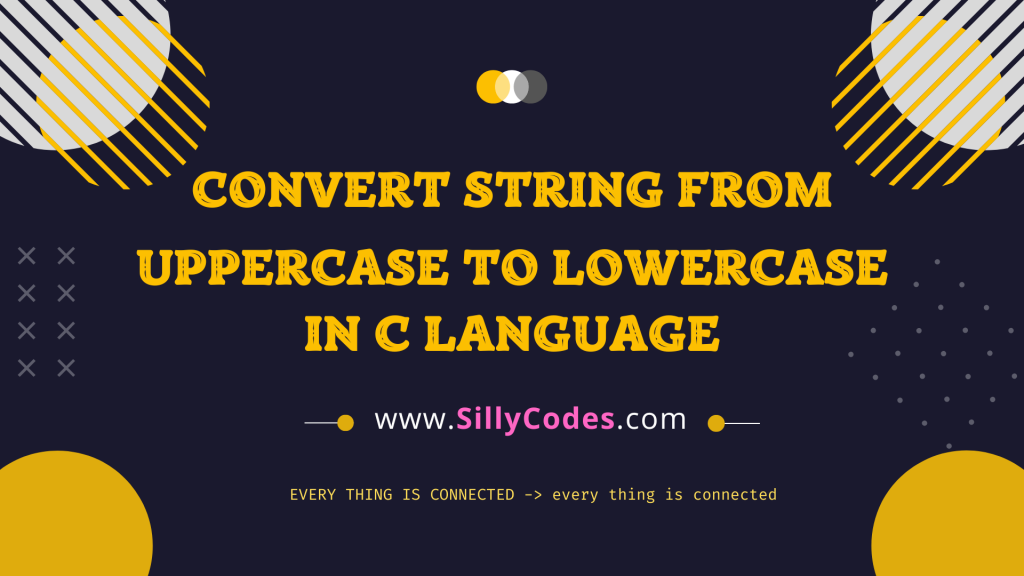
- Algorithm to Convert String From Uppercase to Lowercase in C:
- Method 1: Convert the String from Uppercase to Lowercase in C without using string functions:
- Method 2: UpperCase string to LowerCase string using user-defined functions in C:
- Method 3: Convert Uppercase to Lowercase string using tolower() function in C:
- Method 4: Convert uppercase string to lowercase using strlwr function:
Program Description:
Write a Program to convert a string from uppercase to lowercase in c programming language. The program will accept a string from the user and converts all upper-case characters in the given string to lower-case characters. If the string contains lowercase characters, then we should not change them.
📢 This Program is part of the C String Practice Programs series
Here are the program input and output
Example Input and Output:
Input:
Enter a string : PROGRAMMING IS FUN
Output:
Converted String: programming is fun
Program Prerequisites:
It is good to know the basics of the C-Strings, C-Arrays, and C-Functions. Please read the following articles to learn more about these topics.
- Strings in C Language
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – Declare, Define, and Call Functions
In this article, we are going to look at a few methods to convert the string from Lower case to Upper case in C language. Before looking at the program. Let’s understand the logic to convert the string from Uppercase to Lowercase.
Algorithm to Convert String From Uppercase to Lowercase in C:
All the characters in the C Language are stored in the memory as an ASCII numbers.
For example, The character upper case A‘s ASCII Value is 65. Similarly lower case a‘s ASCII Value is 97. So the C language store these characters based on their ASCII values.
You can check the list of ASCII Values by looking at the following post
So, To convert any Uppercase character to a Lowercase character, We can Add the number 32 to the ASCII number of the uppercase character.
For example,
- Uppercase ‘C’ – ASCII Value – 67.
- To convert Uppercase to a Lowercase character – Add 32 to the Uppercase character. i.e 67 + 32 = 99
- The ASCII value 99 is equal to the Lowercase character ‘c’
Here is the Algorithm of the program
- Declare a string named inputStr with size SIZE( i.e SIZE is a global constant)
- Prompt the user to input a string and store it in the inputStr string. We are using the gets function. use it with caution as it won’t check boundaries.
- Now Iterate over the string character by character and if the character is an Uppercase character, Then Add the number 32 to the ASCII value of the Uppercase character to make it a Lowercase character.
- Create a For Loop, Start from
i=0 and Go till the terminating
NULLcharacter. ( for(i = 0; inputStr[i]; i++) )
- At each iteration, Check if the inputStr[i] is an Uppercase character using if(inputStr[i] >= ‘A’ && inputStr[i] <= ‘Z’) condition. We can also use is isupper() function from ctype.h header file.
- If the inputStr[i] is an uppercase character, Then Add the number 32 to the ASCII value of character – inputStr[i] = inputStr[i] + 32;
- Repeat the above steps until we reach the terminating NULL Character ( \0) of the inputStr.
- Once the above loop is completed, All the Uppercase characters in the given string will be converted into LowerCase characters.
- Print the results on the console using the printf function.
Method 1: Convert the String from Uppercase to Lowercase in C without using string functions:
Here is the string conversion program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
/*     program to convert upper case string to lower case string     sillycodes.com */  #include<stdio.h>  // Max Size of string const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     int i;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);       // Iterate over the string     for(i = 0; inputStr[i]; i++)     {          // check if the character is a upper case alphabet         if(inputStr[i] >= 'A' && inputStr[i] <= 'Z')         {             // Ascii value of 'a' = 97             // Ascii value of 'A' = 65             // To covert Upper to lower, Add 32 to upper case alphabet ASCII value.             // Learn More - https://sillycodes.com/c-program-to-print-all-ascii-characters/             inputStr[i] = inputStr[i] + 32;         }     }      // print the resultant string     printf("Converted String: %s\n", inputStr);      return 0; } |
Program Output:
Let’s compile and Run the program using GCC compiler(Any of your favorite compilers)
1 2 3 4 5 6 7 8 |
$ gcc upper-to-lower.c $ ./a.out Enter a string : PROGRAMMING IS FUN Converted String: programming is fun $ ./a.out Enter a string : Learn PROGRAMMING at SILLYCODES.com Converted String: learn programming at sillycodes.com $ |
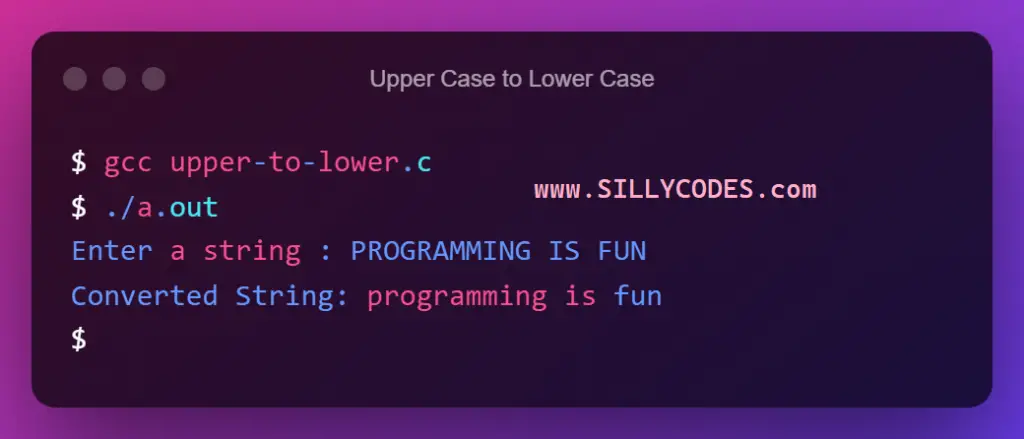
The program is properly converting the upper case character to lower case characters.
Method 2: UpperCase string to LowerCase string using user-defined functions in C:
We are going to define two user-defined functions(except the main() function). They are isUpperCaseChar and toLowerCaseChar.
The isUpperCaseChar() function takes a character as input and checks if it is an uppercase character. This function returns One(1) if the given character is upper case, Otherwise it returns Zero(0). This function is similar to isupper() library function.
Similarly, The toLowerCaseChar() function takes an Uppercase character as input and Converts the given character into a Lowercase character and returns the converted string’s ASCII Code. This function is similar to the tolower() string library function.
Here is the program to convert an uppercase string to a lowercase string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 |
/*     program to convert upper case string to lower case string using user defined functions     sillycodes.com */  #include<stdio.h>  // Max Size of string const int SIZE = 100;  /** * @brief  - Check if the 'ch' is upper case character * * @param ch  - input character * @return int - 1 if 'ch' is upper case. Otherwise, Zero(0). */ int isUpperCaseChar(char ch) {     // check if the character is a upper case alphabet     if(ch >= 'A' && ch <= 'Z')     {         // ch is upper case character         return 1;     }      return 0; }  /** * @brief  - Convert Upper case 'ch' to Lower case character and return it * * @param ch  - input character * @return int - lower case character ascii value */ int toLowerCaseChar(char ch) {     // To covert Upper to lower, Add 32 to upper case alphabet ASCII value.     // Learn More - https://sillycodes.com/c-program-to-print-all-ascii-characters/     return ch + 32; }  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     int i;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);       // Iterate over the string     for(i = 0; inputStr[i]; i++)     {          // Call 'isUpperCaseChar' to check if the character is a upper case alphabet         if(isUpperCaseChar(inputStr[i]))         {             // call 'toLowerCaseChar' function to convert the string             inputStr[i] = toLowerCaseChar(inputStr[i]);         }      }      // print the resultant string     printf("Resultant String: %s\n", inputStr);      return 0; } |
Program Output:
Compile and Run the program.
1 2 3 4 5 |
$ gcc u-to-l-func.c $ ./a.out Enter a string : EVERY THING IS CONNECTED Resultant String: every thing is connected $ |
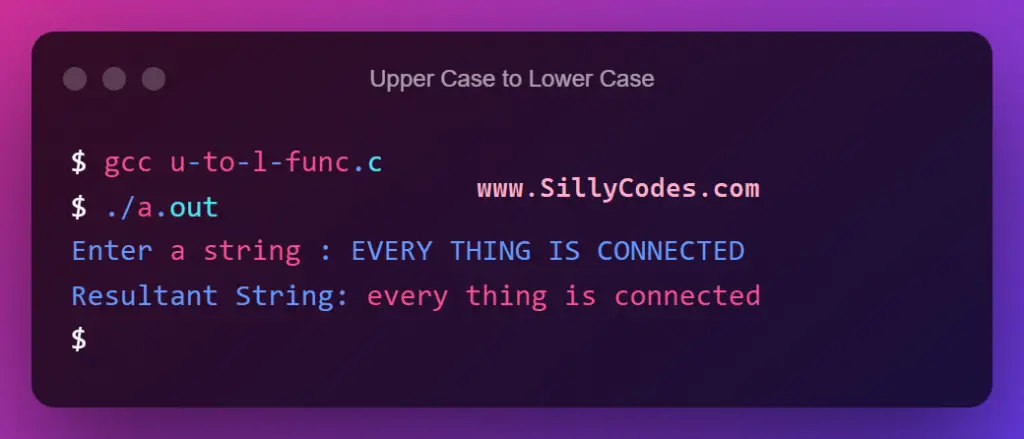
Method 3: Convert Uppercase to Lowercase string using tolower() function in C:
In this method, We are going to use the two library functions isupper() and tolower() to convert an uppercase string to a lowercase string in C programming language.
The isupper() and tolower() functions are available as part of the ctype.h header file.
Here is the syntax of the isupper() library function.
1 |
int isupper(int c); |
The isupper() function checks if the character c is an Uppercase character. It returns a Non-Zero value if c is an Uppercase character, Otherwise, It returns Zero.
Syntax of tolower() function
1 |
int tolower(int c); |
The tolower() function converts the given character c to lowercase characters and returns, its ASCII value.
Here is the program to convert string from uppercase to lowercase characters using C Standard library functions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
/*     program to convert upper case string to lower case string     sillycodes.com */  #include<stdio.h> #include<ctype.h>  // Max Size of string const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     int i;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);       // Iterate over the string     for(i = 0; inputStr[i]; i++)     {          // use 'isupper()' library function to check if the 'ch' is upper case         if(isupper(inputStr[i]))         {             // call the 'tolower' library function.             inputStr[i] = tolower(inputStr[i]);         }     }      // print the resultant string     printf("Converted String: %s\n", inputStr);      return 0; } |
Program Output:
Compile and Run the program.
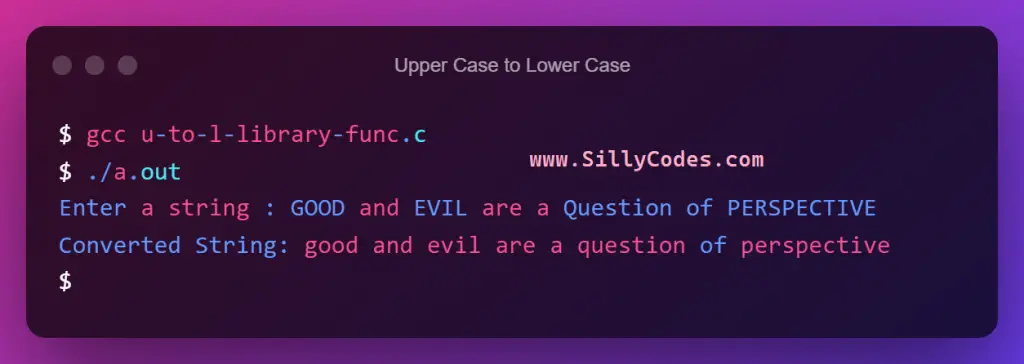
1 2 3 4 5 |
$ gcc u-to-l-library-func.c $ ./a.out Enter a string : GOOD and EVIL are a Question of PERSPECTIVE Converted String: good and evil are a question of perspective $ |
Method 4: Convert uppercase string to lowercase using strlwr function:
There is another function called strlwrto convert the string to a lower case string. But strlwrfunction is not a C standard function. It is a non-standard function from Microsoft’s C-Lang library. So it might not work on all implementations (compilers/environments)
1 |
char *strlwr(char *string); |
If your implementation supports you can use the strlwr function like below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
/*     program to convert upper case string to lower case string     sillycodes.com */  #include<stdio.h> #include<string.h>  // Max Size of string const int SIZE = 1000;  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     int i;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);      // call 'strlwr' to conver the string to 'lower case'     printf("Converted String: %s\n", strlwr(inputStr));      return 0; } |
If strlwr function doesn’t work, Then try the _strlwr() function
2 Responses
[…] we already discussed earlier ( Lowercase to Uppercase conversion, Uppercase to lowercase character conversion programs), characters in C language are stored in the memory with their ASCII […]
[…] C Program to Convert Uppercase string to Lowercase string […]