Find Minimum Element in Array in C Language
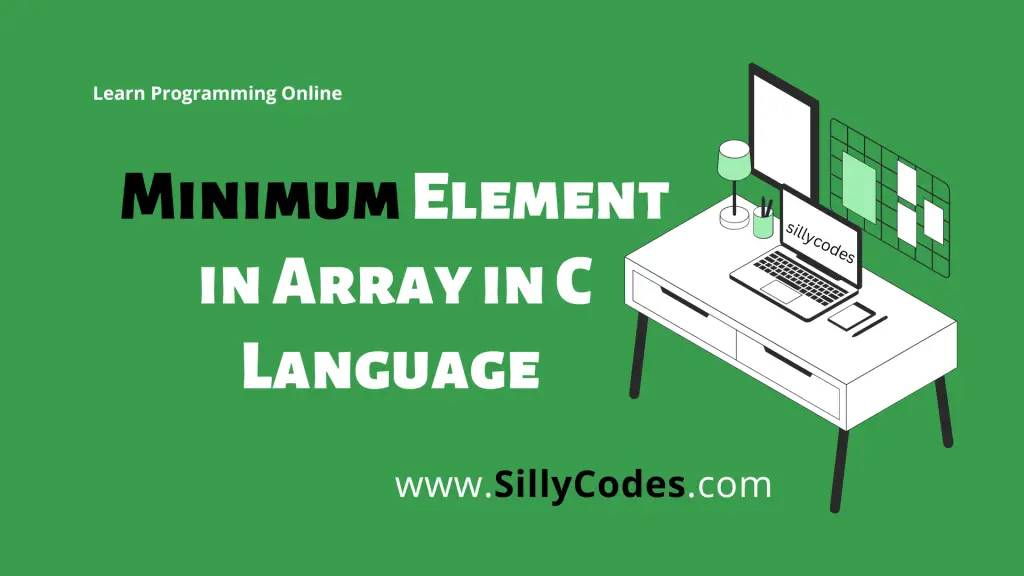
Find Minimum element in Array in C Program Description:
Write a Program to Find Minimum element in Array in C programming language. The program should accept the user input, update the array, and calculate the minimum element in the array.
Example Input and Output:
Input:
1 2 3 4 5 6 |
Please enter array elements data[0] : 4 data[1] : 6 data[2] : 9 data[3] : 2 data[4] : 4 |
Output:
Minimum element in array is : 2
Prerequisites:
It is recommended to know the basics of the Arrays in C and functions in c. We have already covered the array and functions in our earlier posts, Please check them.
- Arrays in C with example programs
- Functions in C – how to declare, define, and call functions.
- Passing Arrays to functions in C
Find Minimum element in Array in C Program Explanation:
- Start the program by declaring an integer array named data. The data array holds 5 elements ( size of the array) and also declare a variable i to traverse the array.
- Take the input from the user and update the array. Use a For Loop to iterate over the array and take the input from the user using standard input and output functions ( printf and scanf)
- Create a variable called min. Initialize the min with INT_MAX. The value of the INT_MAX is 2147483647. Learn more about the INT_MAX at Size and Limits of datatypes
- To find the minimum element we need to traverse the array and update the min if any element is smaller than the min. data[i] < min
- Once the above loop is completed the variable min contain the minimum element in the array.
- Display the results on the console.
The minimum element in Array in C Program Algorithm:
- Declare array int data[5]; and int i
- Prompt the user to input the values for the array
- FORÂ
i = 0Â toÂ
SIZE-1Â ( Loop )
- Read the value for  data[i]
- Initialize min – min = INT_MAX;
- FORÂ
i = 0Â toÂ
SIZE-1Â ( Loop )
- update min( min = data[i]) if the data[i] < min is true
- Print the min value on the console
Program to Find Minimum element in Array in C:
Let’s convert the above algorithm to code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
/*     Program to find minimum number of Array     sillycodes.com */ #include <stdio.h> #include <limits.h>  int main() {     // declare the 'data' array     int data[5];     int i;      // User input for array elements     printf("Please enter array elements\n");     for(i = 0; i < 5; i++)     {         printf("data[%d] : ", i);         scanf("%d", &data[i]);     }         // initialize the 'min' value with 'INT_MAX' from limits.h file     // Value of INT_MAX = 2147483647      // Look at - https://sillycodes.com/size-and-limits-datatypes-in-c-programming/ for info.     int min = INT_MAX;      // find the minimum of array elements     for(i = 0; i < 5; i++)     {         // check for 'min'         if( data[i] < min)         {             // update the 'min' value             min = data[i];         }     }      // print the result     printf("Minimum element in array is : %d\n", min);      return 0; } |
Program Output:
Compile and Run the program using GCC (any compiler)
1 2 3 4 5 6 7 8 9 10 |
$ gcc min-array.c $ ./a.out Please enter array elements data[0] : 4 data[1] : 6 data[2] : 9 data[3] : 2 data[4] : 4 Minimum element in array is : 2 $ |
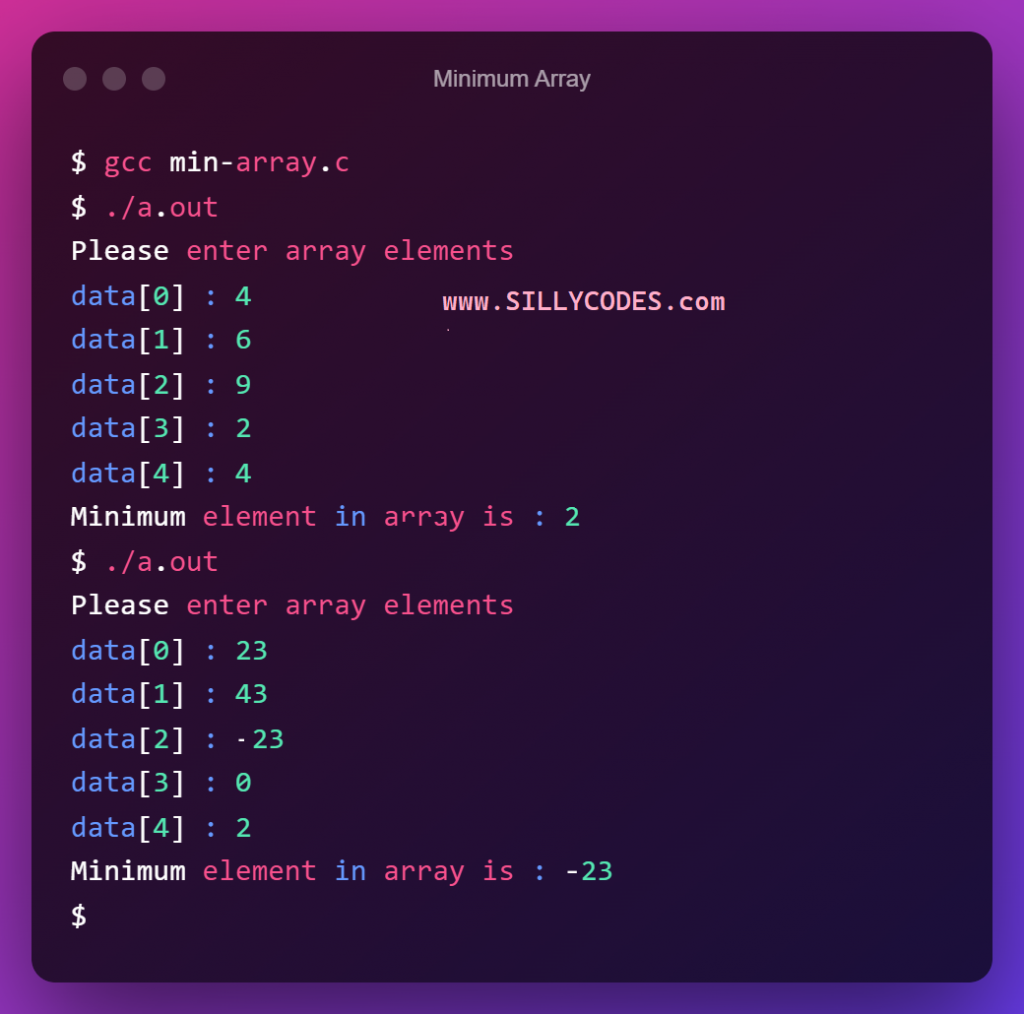
With negative numbers
1 2 3 4 5 6 7 8 9 |
$ ./a.out Please enter array elements data[0] : 23 data[1] : 43 data[2] : -23 data[3] : 0 data[4] : 2 Minimum element in array is : -23 $ |
Find Minimum element in Array in C using Functions:
In the above program, we have used only the main() function. So let’s divide the above program into the functions So that we can re-use the code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 |
/*     Program to find minimum number of Array using function     sillycodes.com */ #include <stdio.h> #include <limits.h>  /** * @brief  - Read the elements from INPUT * * @param data - 'data' Array * @param size - size of the array */ void read(int *data, int size) {     int i;     printf("Please enter array elements\n");     // Read the input from the user     for(i = 0; i < size; i++)     {         printf("data[%d] : ", i);         scanf("%d", &data[i]);     } }  /** * @brief  - Take the array as input and return Min of all array elements * * @param data  - Array * @param size  - Size of the array * @return int  - Minimum element in the array */  int minimum(int data[], int size) {     // initialize the 'min' value with 'INT_MAX' from limits.h file     // Value of INT_MAX = 2147483647      // Look at - https://sillycodes.com/size-and-limits-datatypes-in-c-programming/ for info.     int i, min = INT_MAX;      // find the minimum of array elements     for(i = 0; i < size; i++)     {         // check for 'min'         if( data[i] < min)         {             // update the 'min' value             min = data[i];         }     }      return min; }  int main() {     // declare the 'data' array     int data[5], min;         // Take the user Input and update the array     read(data, 5);      // Find the minimum element in 'data'     min = minimum(data, 5);      // print the result     printf("Minimum element in array is : %d\n", min);      return 0; } |
We have defined two functions here
- read() function – The
read() function is used to read the elements of the array.
- Prototype of read function
- void read(int *data, int size);
-
minimum function – The
minimum function finds the minimum element in the array and returns it.
- Prototype of minimum function
- int minimum(int data[], int size);
📢We can use the pointer or array as the formal argument of the functions.
Program Output:
Now, Let’s compile and run the program and observe the output
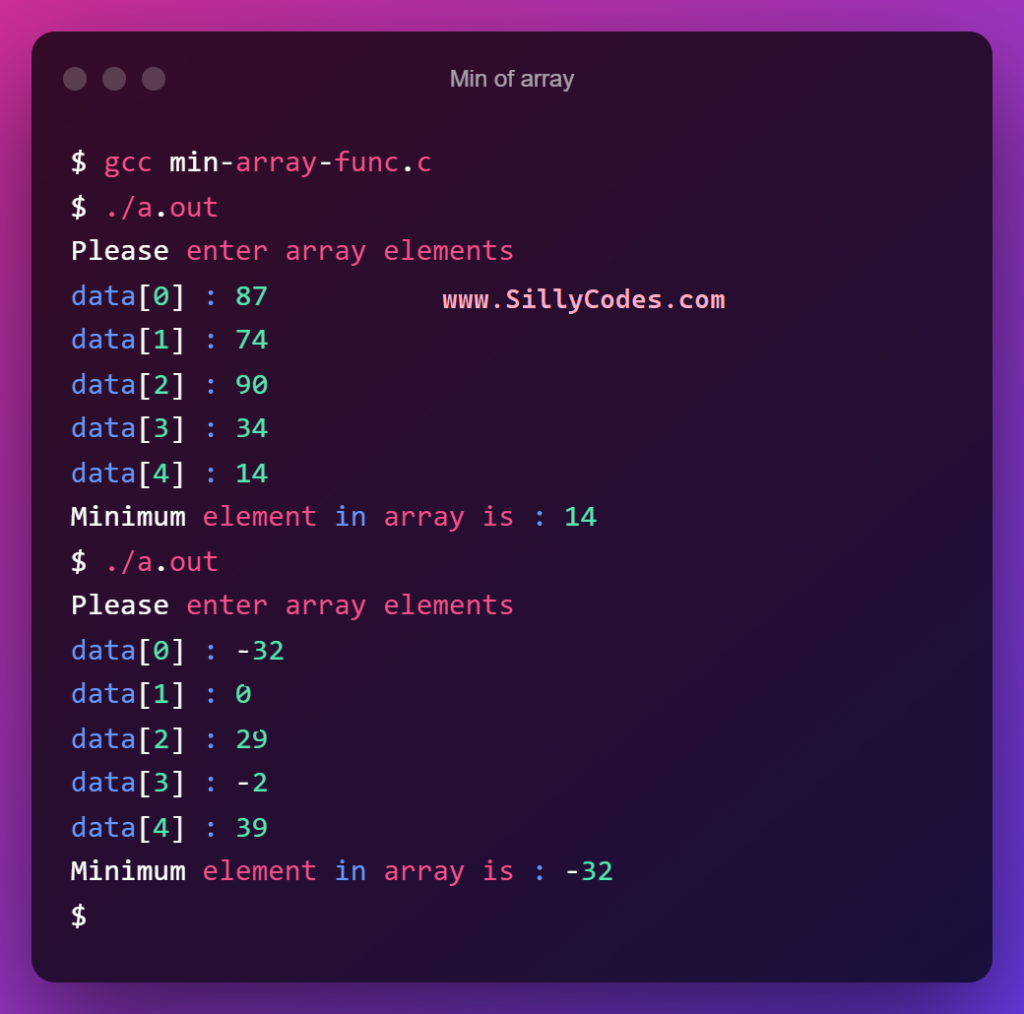
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
$ gcc min-array-func.c $ ./a.out Please enter array elements data[0] : 87 data[1] : 74 data[2] : 90 data[3] : 34 data[4] : 14 Minimum element in array is : 14 $ ./a.out Please enter array elements data[0] : -32 data[1] : 0 data[2] : 29 data[3] : -2 data[4] : 39 Minimum element in array is : -32 $ |
As we can see from the above output, We are getting the desired results.
Related Array Programs:
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
Related Functions and Recursion Programs:
- C Program to Perform Arithmetic Operations using functions
- Add Two Numbers using Functions in C
- C Program to calculate Fibonacci Series using function
- C Program to find Factorial of a Number using functions
- C Program to find Minimum and Maximum numbers using Functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
1 Response
[…] to find the Second Largest Element in Array in C programming language. We have looked at the Minimum number in the array and Maximum Element in Array programs in earlier articles, In today’s article, We will look at […]